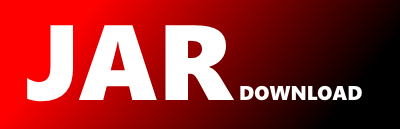
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolRoleMapping Maven / Gradle / Ivy
package software.amazon.awscdk.services.cognito.identitypool;
/**
* (experimental) Map roles to users in the identity pool based on claims from the Identity Provider.
*
* Example:
*
*
* import software.amazon.awscdk.services.cognito.identitypool.IdentityPoolRoleMapping;
* IdentityPool identityPool;
* IdentityPoolRoleMapping myAddedRoleMapping1;
* IdentityPoolRoleMapping myAddedRoleMapping2;
* IdentityPoolRoleMapping myAddedRoleMapping3;
* identityPool.addRoleMappings(myAddedRoleMapping1, myAddedRoleMapping2, myAddedRoleMapping3);
*
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-cognito-identitypoolroleattachment.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-31T18:44:17.062Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cognito.identitypool.$Module.class, fqn = "@aws-cdk/aws-cognito-identitypool.IdentityPoolRoleMapping")
@software.amazon.jsii.Jsii.Proxy(IdentityPoolRoleMapping.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface IdentityPoolRoleMapping extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) The url of the provider of for which the role is mapped.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl getProviderUrl();
/**
* (experimental) Allow for role assumption when results of role mapping are ambiguous.
*
* Default: false - Ambiguous role resolutions will lead to requester being denied
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getResolveAmbiguousRoles() {
return null;
}
/**
* (experimental) The claim and value that must be matched in order to assume the role.
*
* Required if useToken is false
*
* Default: - No Rule Mapping Rule
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getRules() {
return null;
}
/**
* (experimental) If true then mapped roles must be passed through the cognito:roles or cognito:preferred_role claims from identity provider.
*
* Default: false
*
* @see https://docs.aws.amazon.com/cognito/latest/developerguide/role-based-access-control.html#using-tokens-to-assign-roles-to-users
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getUseToken() {
return null;
}
/**
* @return a {@link Builder} of {@link IdentityPoolRoleMapping}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link IdentityPoolRoleMapping}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl providerUrl;
java.lang.Boolean resolveAmbiguousRoles;
java.util.List rules;
java.lang.Boolean useToken;
/**
* Sets the value of {@link IdentityPoolRoleMapping#getProviderUrl}
* @param providerUrl The url of the provider of for which the role is mapped. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder providerUrl(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl providerUrl) {
this.providerUrl = providerUrl;
return this;
}
/**
* Sets the value of {@link IdentityPoolRoleMapping#getResolveAmbiguousRoles}
* @param resolveAmbiguousRoles Allow for role assumption when results of role mapping are ambiguous.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder resolveAmbiguousRoles(java.lang.Boolean resolveAmbiguousRoles) {
this.resolveAmbiguousRoles = resolveAmbiguousRoles;
return this;
}
/**
* Sets the value of {@link IdentityPoolRoleMapping#getRules}
* @param rules The claim and value that must be matched in order to assume the role.
* Required if useToken is false
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder rules(java.util.List extends software.amazon.awscdk.services.cognito.identitypool.RoleMappingRule> rules) {
this.rules = (java.util.List)rules;
return this;
}
/**
* Sets the value of {@link IdentityPoolRoleMapping#getUseToken}
* @param useToken If true then mapped roles must be passed through the cognito:roles or cognito:preferred_role claims from identity provider.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useToken(java.lang.Boolean useToken) {
this.useToken = useToken;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link IdentityPoolRoleMapping}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public IdentityPoolRoleMapping build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link IdentityPoolRoleMapping}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements IdentityPoolRoleMapping {
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl providerUrl;
private final java.lang.Boolean resolveAmbiguousRoles;
private final java.util.List rules;
private final java.lang.Boolean useToken;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.providerUrl = software.amazon.jsii.Kernel.get(this, "providerUrl", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl.class));
this.resolveAmbiguousRoles = software.amazon.jsii.Kernel.get(this, "resolveAmbiguousRoles", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.rules = software.amazon.jsii.Kernel.get(this, "rules", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.RoleMappingRule.class)));
this.useToken = software.amazon.jsii.Kernel.get(this, "useToken", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.providerUrl = java.util.Objects.requireNonNull(builder.providerUrl, "providerUrl is required");
this.resolveAmbiguousRoles = builder.resolveAmbiguousRoles;
this.rules = (java.util.List)builder.rules;
this.useToken = builder.useToken;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviderUrl getProviderUrl() {
return this.providerUrl;
}
@Override
public final java.lang.Boolean getResolveAmbiguousRoles() {
return this.resolveAmbiguousRoles;
}
@Override
public final java.util.List getRules() {
return this.rules;
}
@Override
public final java.lang.Boolean getUseToken() {
return this.useToken;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("providerUrl", om.valueToTree(this.getProviderUrl()));
if (this.getResolveAmbiguousRoles() != null) {
data.set("resolveAmbiguousRoles", om.valueToTree(this.getResolveAmbiguousRoles()));
}
if (this.getRules() != null) {
data.set("rules", om.valueToTree(this.getRules()));
}
if (this.getUseToken() != null) {
data.set("useToken", om.valueToTree(this.getUseToken()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cognito-identitypool.IdentityPoolRoleMapping"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IdentityPoolRoleMapping.Jsii$Proxy that = (IdentityPoolRoleMapping.Jsii$Proxy) o;
if (!providerUrl.equals(that.providerUrl)) return false;
if (this.resolveAmbiguousRoles != null ? !this.resolveAmbiguousRoles.equals(that.resolveAmbiguousRoles) : that.resolveAmbiguousRoles != null) return false;
if (this.rules != null ? !this.rules.equals(that.rules) : that.rules != null) return false;
return this.useToken != null ? this.useToken.equals(that.useToken) : that.useToken == null;
}
@Override
public final int hashCode() {
int result = this.providerUrl.hashCode();
result = 31 * result + (this.resolveAmbiguousRoles != null ? this.resolveAmbiguousRoles.hashCode() : 0);
result = 31 * result + (this.rules != null ? this.rules.hashCode() : 0);
result = 31 * result + (this.useToken != null ? this.useToken.hashCode() : 0);
return result;
}
}
}