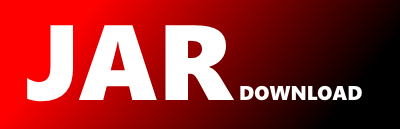
software.amazon.awscdk.services.dms.CfnReplicationTask Maven / Gradle / Ivy
Show all versions of dms Show documentation
package software.amazon.awscdk.services.dms;
/**
* A CloudFormation AWS::DMS::ReplicationTask
.
*
* The AWS::DMS::ReplicationTask
resource creates an AWS DMS replication task.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.dms.*;
* CfnReplicationTask cfnReplicationTask = CfnReplicationTask.Builder.create(this, "MyCfnReplicationTask")
* .migrationType("migrationType")
* .replicationInstanceArn("replicationInstanceArn")
* .sourceEndpointArn("sourceEndpointArn")
* .tableMappings("tableMappings")
* .targetEndpointArn("targetEndpointArn")
* // the properties below are optional
* .cdcStartPosition("cdcStartPosition")
* .cdcStartTime(123)
* .cdcStopPosition("cdcStopPosition")
* .replicationTaskIdentifier("replicationTaskIdentifier")
* .replicationTaskSettings("replicationTaskSettings")
* .resourceIdentifier("resourceIdentifier")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .taskData("taskData")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:29:56.410Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.dms.$Module.class, fqn = "@aws-cdk/aws-dms.CfnReplicationTask")
public class CfnReplicationTask extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnReplicationTask(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnReplicationTask(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.dms.CfnReplicationTask.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new AWS::DMS::ReplicationTask
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnReplicationTask(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.dms.CfnReplicationTaskProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector
- tree inspector to collect and process attributes.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* One or more tags to be assigned to the replication task.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The migration type.
*
* Valid values: full-load
| cdc
| full-load-and-cdc
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getMigrationType() {
return software.amazon.jsii.Kernel.get(this, "migrationType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The migration type.
*
* Valid values: full-load
| cdc
| full-load-and-cdc
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setMigrationType(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "migrationType", java.util.Objects.requireNonNull(value, "migrationType is required"));
}
/**
* The Amazon Resource Name (ARN) of a replication instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getReplicationInstanceArn() {
return software.amazon.jsii.Kernel.get(this, "replicationInstanceArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The Amazon Resource Name (ARN) of a replication instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setReplicationInstanceArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "replicationInstanceArn", java.util.Objects.requireNonNull(value, "replicationInstanceArn is required"));
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the source endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getSourceEndpointArn() {
return software.amazon.jsii.Kernel.get(this, "sourceEndpointArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the source endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSourceEndpointArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "sourceEndpointArn", java.util.Objects.requireNonNull(value, "sourceEndpointArn is required"));
}
/**
* The table mappings for the task, in JSON format.
*
* For more information, see Using Table Mapping to Specify Task Settings in the AWS Database Migration Service User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getTableMappings() {
return software.amazon.jsii.Kernel.get(this, "tableMappings", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The table mappings for the task, in JSON format.
*
* For more information, see Using Table Mapping to Specify Task Settings in the AWS Database Migration Service User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setTableMappings(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "tableMappings", java.util.Objects.requireNonNull(value, "tableMappings is required"));
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the target endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getTargetEndpointArn() {
return software.amazon.jsii.Kernel.get(this, "targetEndpointArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the target endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setTargetEndpointArn(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "targetEndpointArn", java.util.Objects.requireNonNull(value, "targetEndpointArn is required"));
}
/**
* Indicates when you want a change data capture (CDC) operation to start.
*
* Use either CdcStartPosition
or CdcStartTime
to specify when you want a CDC operation to start. Specifying both values results in an error.
*
* The value can be in date, checkpoint, log sequence number (LSN), or system change number (SCN) format.
*
* Here is a date example: --cdc-start-position "2018-03-08T12:12:12"
*
* Here is a checkpoint example: --cdc-start-position "checkpoint:V1#27#mysql-bin-changelog.157832:1975:-1:2002:677883278264080:mysql-bin-changelog.157832:1876#0#0#*#0#93"
*
* Here is an LSN example: --cdc-start-position “mysql-bin-changelog.000024:373”
*
*
*
* When you use this task setting with a source PostgreSQL database, a logical replication slot should already be created and associated with the source endpoint. You can verify this by setting the slotName
extra connection attribute to the name of this logical replication slot. For more information, see Extra Connection Attributes When Using PostgreSQL as a Source for AWS DMS in the AWS Database Migration Service User Guide .
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getCdcStartPosition() {
return software.amazon.jsii.Kernel.get(this, "cdcStartPosition", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Indicates when you want a change data capture (CDC) operation to start.
*
* Use either CdcStartPosition
or CdcStartTime
to specify when you want a CDC operation to start. Specifying both values results in an error.
*
* The value can be in date, checkpoint, log sequence number (LSN), or system change number (SCN) format.
*
* Here is a date example: --cdc-start-position "2018-03-08T12:12:12"
*
* Here is a checkpoint example: --cdc-start-position "checkpoint:V1#27#mysql-bin-changelog.157832:1975:-1:2002:677883278264080:mysql-bin-changelog.157832:1876#0#0#*#0#93"
*
* Here is an LSN example: --cdc-start-position “mysql-bin-changelog.000024:373”
*
*
*
* When you use this task setting with a source PostgreSQL database, a logical replication slot should already be created and associated with the source endpoint. You can verify this by setting the slotName
extra connection attribute to the name of this logical replication slot. For more information, see Extra Connection Attributes When Using PostgreSQL as a Source for AWS DMS in the AWS Database Migration Service User Guide .
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCdcStartPosition(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "cdcStartPosition", value);
}
/**
* Indicates the start time for a change data capture (CDC) operation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Number getCdcStartTime() {
return software.amazon.jsii.Kernel.get(this, "cdcStartTime", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Indicates the start time for a change data capture (CDC) operation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCdcStartTime(final @org.jetbrains.annotations.Nullable java.lang.Number value) {
software.amazon.jsii.Kernel.set(this, "cdcStartTime", value);
}
/**
* Indicates when you want a change data capture (CDC) operation to stop.
*
* The value can be either server time or commit time.
*
* Here is a server time example: --cdc-stop-position "server_time:2018-02-09T12:12:12"
*
* Here is a commit time example: --cdc-stop-position "commit_time: 2018-02-09T12:12:12"
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getCdcStopPosition() {
return software.amazon.jsii.Kernel.get(this, "cdcStopPosition", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Indicates when you want a change data capture (CDC) operation to stop.
*
* The value can be either server time or commit time.
*
* Here is a server time example: --cdc-stop-position "server_time:2018-02-09T12:12:12"
*
* Here is a commit time example: --cdc-stop-position "commit_time: 2018-02-09T12:12:12"
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCdcStopPosition(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "cdcStopPosition", value);
}
/**
* An identifier for the replication task.
*
* Constraints:
*
*
* - Must contain 1-255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getReplicationTaskIdentifier() {
return software.amazon.jsii.Kernel.get(this, "replicationTaskIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* An identifier for the replication task.
*
* Constraints:
*
*
* - Must contain 1-255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setReplicationTaskIdentifier(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "replicationTaskIdentifier", value);
}
/**
* Overall settings for the task, in JSON format.
*
* For more information, see Specifying Task Settings for AWS Database Migration Service Tasks in the AWS Database Migration Service User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getReplicationTaskSettings() {
return software.amazon.jsii.Kernel.get(this, "replicationTaskSettings", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Overall settings for the task, in JSON format.
*
* For more information, see Specifying Task Settings for AWS Database Migration Service Tasks in the AWS Database Migration Service User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setReplicationTaskSettings(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "replicationTaskSettings", value);
}
/**
* A display name for the resource identifier at the end of the EndpointArn
response parameter that is returned in the created Endpoint
object.
*
* The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
.
*
* For example, this value might result in the EndpointArn
value arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a ResourceIdentifier
value, AWS DMS generates a default identifier value for the end of EndpointArn
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getResourceIdentifier() {
return software.amazon.jsii.Kernel.get(this, "resourceIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A display name for the resource identifier at the end of the EndpointArn
response parameter that is returned in the created Endpoint
object.
*
* The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
.
*
* For example, this value might result in the EndpointArn
value arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a ResourceIdentifier
value, AWS DMS generates a default identifier value for the end of EndpointArn
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setResourceIdentifier(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "resourceIdentifier", value);
}
/**
* AWS::DMS::ReplicationTask.TaskData
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getTaskData() {
return software.amazon.jsii.Kernel.get(this, "taskData", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* AWS::DMS::ReplicationTask.TaskData
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setTaskData(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "taskData", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.dms.CfnReplicationTask}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.dms.CfnReplicationTaskProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.dms.CfnReplicationTaskProps.Builder();
}
/**
* The migration type.
*
* Valid values: full-load
| cdc
| full-load-and-cdc
*
* @return {@code this}
* @param migrationType The migration type. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder migrationType(final java.lang.String migrationType) {
this.props.migrationType(migrationType);
return this;
}
/**
* The Amazon Resource Name (ARN) of a replication instance.
*
* @return {@code this}
* @param replicationInstanceArn The Amazon Resource Name (ARN) of a replication instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder replicationInstanceArn(final java.lang.String replicationInstanceArn) {
this.props.replicationInstanceArn(replicationInstanceArn);
return this;
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the source endpoint.
*
* @return {@code this}
* @param sourceEndpointArn An Amazon Resource Name (ARN) that uniquely identifies the source endpoint. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceEndpointArn(final java.lang.String sourceEndpointArn) {
this.props.sourceEndpointArn(sourceEndpointArn);
return this;
}
/**
* The table mappings for the task, in JSON format.
*
* For more information, see Using Table Mapping to Specify Task Settings in the AWS Database Migration Service User Guide .
*
* @return {@code this}
* @param tableMappings The table mappings for the task, in JSON format. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tableMappings(final java.lang.String tableMappings) {
this.props.tableMappings(tableMappings);
return this;
}
/**
* An Amazon Resource Name (ARN) that uniquely identifies the target endpoint.
*
* @return {@code this}
* @param targetEndpointArn An Amazon Resource Name (ARN) that uniquely identifies the target endpoint. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetEndpointArn(final java.lang.String targetEndpointArn) {
this.props.targetEndpointArn(targetEndpointArn);
return this;
}
/**
* Indicates when you want a change data capture (CDC) operation to start.
*
* Use either CdcStartPosition
or CdcStartTime
to specify when you want a CDC operation to start. Specifying both values results in an error.
*
* The value can be in date, checkpoint, log sequence number (LSN), or system change number (SCN) format.
*
* Here is a date example: --cdc-start-position "2018-03-08T12:12:12"
*
* Here is a checkpoint example: --cdc-start-position "checkpoint:V1#27#mysql-bin-changelog.157832:1975:-1:2002:677883278264080:mysql-bin-changelog.157832:1876#0#0#*#0#93"
*
* Here is an LSN example: --cdc-start-position “mysql-bin-changelog.000024:373”
*
*
*
* When you use this task setting with a source PostgreSQL database, a logical replication slot should already be created and associated with the source endpoint. You can verify this by setting the slotName
extra connection attribute to the name of this logical replication slot. For more information, see Extra Connection Attributes When Using PostgreSQL as a Source for AWS DMS in the AWS Database Migration Service User Guide .
*
*
*
* @return {@code this}
* @param cdcStartPosition Indicates when you want a change data capture (CDC) operation to start. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cdcStartPosition(final java.lang.String cdcStartPosition) {
this.props.cdcStartPosition(cdcStartPosition);
return this;
}
/**
* Indicates the start time for a change data capture (CDC) operation.
*
* @return {@code this}
* @param cdcStartTime Indicates the start time for a change data capture (CDC) operation. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cdcStartTime(final java.lang.Number cdcStartTime) {
this.props.cdcStartTime(cdcStartTime);
return this;
}
/**
* Indicates when you want a change data capture (CDC) operation to stop.
*
* The value can be either server time or commit time.
*
* Here is a server time example: --cdc-stop-position "server_time:2018-02-09T12:12:12"
*
* Here is a commit time example: --cdc-stop-position "commit_time: 2018-02-09T12:12:12"
*
* @return {@code this}
* @param cdcStopPosition Indicates when you want a change data capture (CDC) operation to stop. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cdcStopPosition(final java.lang.String cdcStopPosition) {
this.props.cdcStopPosition(cdcStopPosition);
return this;
}
/**
* An identifier for the replication task.
*
* Constraints:
*
*
* - Must contain 1-255 alphanumeric characters or hyphens.
* - First character must be a letter.
* - Cannot end with a hyphen or contain two consecutive hyphens.
*
*
* @return {@code this}
* @param replicationTaskIdentifier An identifier for the replication task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder replicationTaskIdentifier(final java.lang.String replicationTaskIdentifier) {
this.props.replicationTaskIdentifier(replicationTaskIdentifier);
return this;
}
/**
* Overall settings for the task, in JSON format.
*
* For more information, see Specifying Task Settings for AWS Database Migration Service Tasks in the AWS Database Migration Service User Guide .
*
* @return {@code this}
* @param replicationTaskSettings Overall settings for the task, in JSON format. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder replicationTaskSettings(final java.lang.String replicationTaskSettings) {
this.props.replicationTaskSettings(replicationTaskSettings);
return this;
}
/**
* A display name for the resource identifier at the end of the EndpointArn
response parameter that is returned in the created Endpoint
object.
*
* The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
.
*
* For example, this value might result in the EndpointArn
value arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a ResourceIdentifier
value, AWS DMS generates a default identifier value for the end of EndpointArn
.
*
* @return {@code this}
* @param resourceIdentifier A display name for the resource identifier at the end of the EndpointArn
response parameter that is returned in the created Endpoint
object. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder resourceIdentifier(final java.lang.String resourceIdentifier) {
this.props.resourceIdentifier(resourceIdentifier);
return this;
}
/**
* One or more tags to be assigned to the replication task.
*
* @return {@code this}
* @param tags One or more tags to be assigned to the replication task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props.tags(tags);
return this;
}
/**
* AWS::DMS::ReplicationTask.TaskData
.
*
* @return {@code this}
* @param taskData AWS::DMS::ReplicationTask.TaskData
. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskData(final java.lang.String taskData) {
this.props.taskData(taskData);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.dms.CfnReplicationTask}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.dms.CfnReplicationTask build() {
return new software.amazon.awscdk.services.dms.CfnReplicationTask(
this.scope,
this.id,
this.props.build()
);
}
}
}