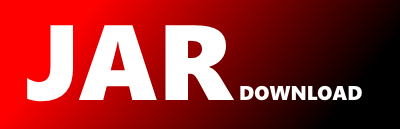
software.amazon.awscdk.services.ec2.alpha.SubnetV2 Maven / Gradle / Ivy
Show all versions of ec2-alpha Show documentation
package software.amazon.awscdk.services.ec2.alpha;
/**
* (experimental) The SubnetV2 class represents a subnet within a VPC (Virtual Private Cloud) in AWS.
*
* It extends the Resource class and implements the ISubnet interface.
*
* Instances of this class can be used to create and manage subnets within a VpcV2 instance.
* Subnets can be configured with specific IP address ranges (IPv4 and IPv6), availability zones,
* and subnet types (e.g., public, private, isolated).
*
* Example:
*
*
* Stack stack = new Stack();
* VpcV2 myVpc = new VpcV2(this, "Vpc");
* RouteTable routeTable = RouteTable.Builder.create(this, "RouteTable")
* .vpc(myVpc)
* .build();
* SubnetV2 subnet = SubnetV2.Builder.create(this, "Subnet")
* .vpc(myVpc)
* .availabilityZone("eu-west-2a")
* .ipv4CidrBlock(new IpCidr("10.0.0.0/24"))
* .subnetType(SubnetType.PUBLIC)
* .build();
* myVpc.addInternetGateway();
* myVpc.addNatGateway(NatGatewayOptions.builder()
* .subnet(subnet)
* .connectivityType(NatConnectivityType.PUBLIC)
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.103.1 (build bef2dea)", date = "2024-10-11T15:56:07.927Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ec2.alpha.$Module.class, fqn = "@aws-cdk/aws-ec2-alpha.SubnetV2")
public class SubnetV2 extends software.amazon.awscdk.Resource implements software.amazon.awscdk.services.ec2.alpha.ISubnetV2 {
protected SubnetV2(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected SubnetV2(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* (experimental) Constructs a new SubnetV2 instance.
*
* @param scope The parent Construct that this resource will be part of. This parameter is required.
* @param id The unique identifier for this resource. This parameter is required.
* @param props The configuration properties for the subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public SubnetV2(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.alpha.SubnetV2Props props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Associate a Network ACL with this subnet.
*
* @param id The unique identifier for this association. This parameter is required.
* @param networkAcl The Network ACL to associate with this subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public void associateNetworkAcl(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.INetworkAcl networkAcl) {
software.amazon.jsii.Kernel.call(this, "associateNetworkAcl", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(networkAcl, "networkAcl is required") });
}
/**
* (experimental) The Availability Zone the subnet is located in.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getAvailabilityZone() {
return software.amazon.jsii.Kernel.get(this, "availabilityZone", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) Dependencies for internet connectivity This Property exposes the RouteTable-Subnet association so that other resources can depend on it.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.constructs.IDependable getInternetConnectivityEstablished() {
return software.amazon.jsii.Kernel.get(this, "internetConnectivityEstablished", software.amazon.jsii.NativeType.forClass(software.constructs.IDependable.class));
}
/**
* (experimental) The IPv4 CIDR block for this subnet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getIpv4CidrBlock() {
return software.amazon.jsii.Kernel.get(this, "ipv4CidrBlock", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) Returns the Network ACL associated with this subnet.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.INetworkAcl getNetworkAcl() {
return software.amazon.jsii.Kernel.get(this, "networkAcl", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.INetworkAcl.class));
}
/**
* (experimental) Return the Route Table associated with this subnet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IRouteTable getRouteTable() {
return software.amazon.jsii.Kernel.get(this, "routeTable", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IRouteTable.class));
}
/**
* (experimental) The subnetId for this particular subnet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getSubnetId() {
return software.amazon.jsii.Kernel.get(this, "subnetId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The IPv6 CIDR Block for this subnet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable java.lang.String getIpv6CidrBlock() {
return software.amazon.jsii.Kernel.get(this, "ipv6CidrBlock", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The type of subnet (public or private) that this subnet represents.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetType getSubnetType() {
return software.amazon.jsii.Kernel.get(this, "subnetType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetType.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.ec2.alpha.SubnetV2}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope The parent Construct that this resource will be part of. This parameter is required.
* @param id The unique identifier for this resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.ec2.alpha.SubnetV2Props.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.ec2.alpha.SubnetV2Props.Builder();
}
/**
* (experimental) Custom AZ for the subnet.
*
* @return {@code this}
* @param availabilityZone Custom AZ for the subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder availabilityZone(final java.lang.String availabilityZone) {
this.props.availabilityZone(availabilityZone);
return this;
}
/**
* (experimental) ipv4 cidr to assign to this subnet.
*
* See https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ec2-subnet.html#cfn-ec2-subnet-cidrblock
*
* @return {@code this}
* @param ipv4CidrBlock ipv4 cidr to assign to this subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv4CidrBlock(final software.amazon.awscdk.services.ec2.alpha.IpCidr ipv4CidrBlock) {
this.props.ipv4CidrBlock(ipv4CidrBlock);
return this;
}
/**
* (experimental) The type of Subnet to configure.
*
* The Subnet type will control the ability to route and connect to the
* Internet.
*
* TODO: Add validation check subnetType
when adding resources (e.g. cannot add NatGateway to private)
*
* @return {@code this}
* @param subnetType The type of Subnet to configure. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subnetType(final software.amazon.awscdk.services.ec2.SubnetType subnetType) {
this.props.subnetType(subnetType);
return this;
}
/**
* (experimental) VPC Prop.
*
* @return {@code this}
* @param vpc VPC Prop. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(final software.amazon.awscdk.services.ec2.alpha.IVpcV2 vpc) {
this.props.vpc(vpc);
return this;
}
/**
* (experimental) Indicates whether a network interface created in this subnet receives an IPv6 address.
*
* If you specify AssignIpv6AddressOnCreation, you must also specify Ipv6CidrBlock.
*
* Default: - undefined in case not provided as an input
*
* @return {@code this}
* @param assignIpv6AddressOnCreation Indicates whether a network interface created in this subnet receives an IPv6 address. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder assignIpv6AddressOnCreation(final java.lang.Boolean assignIpv6AddressOnCreation) {
this.props.assignIpv6AddressOnCreation(assignIpv6AddressOnCreation);
return this;
}
/**
* (experimental) Ipv6 CIDR Range for subnet.
*
* Default: - No Ipv6 address
*
* @return {@code this}
* @param ipv6CidrBlock Ipv6 CIDR Range for subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv6CidrBlock(final software.amazon.awscdk.services.ec2.alpha.IpCidr ipv6CidrBlock) {
this.props.ipv6CidrBlock(ipv6CidrBlock);
return this;
}
/**
* (experimental) Custom Route for subnet.
*
* Default: - a default route table created
*
* @return {@code this}
* @param routeTable Custom Route for subnet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder routeTable(final software.amazon.awscdk.services.ec2.IRouteTable routeTable) {
this.props.routeTable(routeTable);
return this;
}
/**
* (experimental) Subnet name.
*
* Default: - provisioned with an autogenerated name by CDK
*
* @return {@code this}
* @param subnetName Subnet name. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subnetName(final java.lang.String subnetName) {
this.props.subnetName(subnetName);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.ec2.alpha.SubnetV2}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.ec2.alpha.SubnetV2 build() {
return new software.amazon.awscdk.services.ec2.alpha.SubnetV2(
this.scope,
this.id,
this.props.build()
);
}
}
}