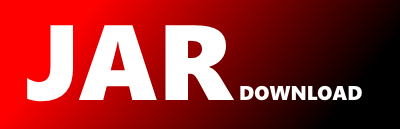
software.amazon.awscdk.services.ec2.alpha.VPNGatewayV2Props Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ec2-alpha Show documentation
Show all versions of ec2-alpha Show documentation
The CDK construct library for VPC V2
package software.amazon.awscdk.services.ec2.alpha;
/**
* (experimental) Properties to define a VPN gateway.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ec2.alpha.*;
* import software.amazon.awscdk.services.ec2.*;
* Subnet subnet;
* SubnetFilter subnetFilter;
* VpcV2 vpcV2;
* VPNGatewayV2Props vPNGatewayV2Props = VPNGatewayV2Props.builder()
* .type(VpnConnectionType.IPSEC_1)
* .vpc(vpcV2)
* // the properties below are optional
* .amazonSideAsn(123)
* .vpnGatewayName("vpnGatewayName")
* .vpnRoutePropagation(List.of(SubnetSelection.builder()
* .availabilityZones(List.of("availabilityZones"))
* .onePerAz(false)
* .subnetFilters(List.of(subnetFilter))
* .subnetGroupName("subnetGroupName")
* .subnets(List.of(subnet))
* .subnetType(SubnetType.PRIVATE_ISOLATED)
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.103.1 (build bef2dea)", date = "2024-10-11T15:56:07.942Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ec2.alpha.$Module.class, fqn = "@aws-cdk/aws-ec2-alpha.VPNGatewayV2Props")
@software.amazon.jsii.Jsii.Proxy(VPNGatewayV2Props.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface VPNGatewayV2Props extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ec2.alpha.VPNGatewayV2Options {
/**
* (experimental) The ID of the VPC for which to create the VPN gateway.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.alpha.IVpcV2 getVpc();
/**
* @return a {@link Builder} of {@link VPNGatewayV2Props}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link VPNGatewayV2Props}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.ec2.alpha.IVpcV2 vpc;
software.amazon.awscdk.services.ec2.VpnConnectionType type;
java.lang.Number amazonSideAsn;
java.lang.String vpnGatewayName;
java.util.List vpnRoutePropagation;
/**
* Sets the value of {@link VPNGatewayV2Props#getVpc}
* @param vpc The ID of the VPC for which to create the VPN gateway. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(software.amazon.awscdk.services.ec2.alpha.IVpcV2 vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link VPNGatewayV2Props#getType}
* @param type The type of VPN connection the virtual private gateway supports. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder type(software.amazon.awscdk.services.ec2.VpnConnectionType type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link VPNGatewayV2Props#getAmazonSideAsn}
* @param amazonSideAsn The private Autonomous System Number (ASN) for the Amazon side of a BGP session.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder amazonSideAsn(java.lang.Number amazonSideAsn) {
this.amazonSideAsn = amazonSideAsn;
return this;
}
/**
* Sets the value of {@link VPNGatewayV2Props#getVpnGatewayName}
* @param vpnGatewayName The resource name of the VPN gateway.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpnGatewayName(java.lang.String vpnGatewayName) {
this.vpnGatewayName = vpnGatewayName;
return this;
}
/**
* Sets the value of {@link VPNGatewayV2Props#getVpnRoutePropagation}
* @param vpnRoutePropagation Subnets where the route propagation should be added.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder vpnRoutePropagation(java.util.List extends software.amazon.awscdk.services.ec2.SubnetSelection> vpnRoutePropagation) {
this.vpnRoutePropagation = (java.util.List)vpnRoutePropagation;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link VPNGatewayV2Props}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public VPNGatewayV2Props build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link VPNGatewayV2Props}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements VPNGatewayV2Props {
private final software.amazon.awscdk.services.ec2.alpha.IVpcV2 vpc;
private final software.amazon.awscdk.services.ec2.VpnConnectionType type;
private final java.lang.Number amazonSideAsn;
private final java.lang.String vpnGatewayName;
private final java.util.List vpnRoutePropagation;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.vpc = software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.alpha.IVpcV2.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.VpnConnectionType.class));
this.amazonSideAsn = software.amazon.jsii.Kernel.get(this, "amazonSideAsn", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.vpnGatewayName = software.amazon.jsii.Kernel.get(this, "vpnGatewayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpnRoutePropagation = software.amazon.jsii.Kernel.get(this, "vpnRoutePropagation", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.vpc = java.util.Objects.requireNonNull(builder.vpc, "vpc is required");
this.type = java.util.Objects.requireNonNull(builder.type, "type is required");
this.amazonSideAsn = builder.amazonSideAsn;
this.vpnGatewayName = builder.vpnGatewayName;
this.vpnRoutePropagation = (java.util.List)builder.vpnRoutePropagation;
}
@Override
public final software.amazon.awscdk.services.ec2.alpha.IVpcV2 getVpc() {
return this.vpc;
}
@Override
public final software.amazon.awscdk.services.ec2.VpnConnectionType getType() {
return this.type;
}
@Override
public final java.lang.Number getAmazonSideAsn() {
return this.amazonSideAsn;
}
@Override
public final java.lang.String getVpnGatewayName() {
return this.vpnGatewayName;
}
@Override
public final java.util.List getVpnRoutePropagation() {
return this.vpnRoutePropagation;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("vpc", om.valueToTree(this.getVpc()));
data.set("type", om.valueToTree(this.getType()));
if (this.getAmazonSideAsn() != null) {
data.set("amazonSideAsn", om.valueToTree(this.getAmazonSideAsn()));
}
if (this.getVpnGatewayName() != null) {
data.set("vpnGatewayName", om.valueToTree(this.getVpnGatewayName()));
}
if (this.getVpnRoutePropagation() != null) {
data.set("vpnRoutePropagation", om.valueToTree(this.getVpnRoutePropagation()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ec2-alpha.VPNGatewayV2Props"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
VPNGatewayV2Props.Jsii$Proxy that = (VPNGatewayV2Props.Jsii$Proxy) o;
if (!vpc.equals(that.vpc)) return false;
if (!type.equals(that.type)) return false;
if (this.amazonSideAsn != null ? !this.amazonSideAsn.equals(that.amazonSideAsn) : that.amazonSideAsn != null) return false;
if (this.vpnGatewayName != null ? !this.vpnGatewayName.equals(that.vpnGatewayName) : that.vpnGatewayName != null) return false;
return this.vpnRoutePropagation != null ? this.vpnRoutePropagation.equals(that.vpnRoutePropagation) : that.vpnRoutePropagation == null;
}
@Override
public final int hashCode() {
int result = this.vpc.hashCode();
result = 31 * result + (this.type.hashCode());
result = 31 * result + (this.amazonSideAsn != null ? this.amazonSideAsn.hashCode() : 0);
result = 31 * result + (this.vpnGatewayName != null ? this.vpnGatewayName.hashCode() : 0);
result = 31 * result + (this.vpnRoutePropagation != null ? this.vpnRoutePropagation.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy