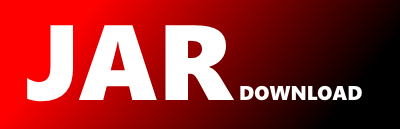
software.amazon.awscdk.services.ec2.alpha.VpcCidrOptions Maven / Gradle / Ivy
Show all versions of ec2-alpha Show documentation
package software.amazon.awscdk.services.ec2.alpha;
/**
* (experimental) Consolidated return parameters to pass to VPC construct.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ec2.alpha.*;
* import software.amazon.awscdk.*;
* CfnResource cfnResource;
* IIpamPool ipamPool;
* VpcCidrOptions vpcCidrOptions = VpcCidrOptions.builder()
* .amazonProvided(false)
* .cidrBlockName("cidrBlockName")
* .dependencies(List.of(cfnResource))
* .ipv4CidrBlock("ipv4CidrBlock")
* .ipv4IpamPool(ipamPool)
* .ipv4NetmaskLength(123)
* .ipv6IpamPool(ipamPool)
* .ipv6NetmaskLength(123)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.103.1 (build bef2dea)", date = "2024-10-11T15:56:07.942Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ec2.alpha.$Module.class, fqn = "@aws-cdk/aws-ec2-alpha.VpcCidrOptions")
@software.amazon.jsii.Jsii.Proxy(VpcCidrOptions.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface VpcCidrOptions extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) Use amazon provided IP range.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAmazonProvided() {
return null;
}
/**
* (experimental) Required to set Secondary cidr block resource name in order to generate unique logical id for the resource.
*
* Default: - no name for primary addresses
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getCidrBlockName() {
return null;
}
/**
* (experimental) Dependency to associate Ipv6 CIDR block.
*
* Default: - No dependency
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getDependencies() {
return null;
}
/**
* (experimental) IPv4 CIDR Block.
*
* Default: '10.0.0.0/16'
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getIpv4CidrBlock() {
return null;
}
/**
* (experimental) Ipv4 IPAM Pool.
*
* Default: - Only required when using IPAM Ipv4
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.alpha.IIpamPool getIpv4IpamPool() {
return null;
}
/**
* (experimental) CIDR Mask for Vpc.
*
* Default: - Only required when using IPAM Ipv4
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getIpv4NetmaskLength() {
return null;
}
/**
* (experimental) Ipv6 IPAM pool id for VPC range, can only be defined under public scope.
*
* Default: - no pool id
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.alpha.IIpamPool getIpv6IpamPool() {
return null;
}
/**
* (experimental) CIDR Mask for Vpc.
*
* Default: - Only required when using AWS Ipam
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getIpv6NetmaskLength() {
return null;
}
/**
* @return a {@link Builder} of {@link VpcCidrOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link VpcCidrOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Boolean amazonProvided;
java.lang.String cidrBlockName;
java.util.List dependencies;
java.lang.String ipv4CidrBlock;
software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv4IpamPool;
java.lang.Number ipv4NetmaskLength;
software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv6IpamPool;
java.lang.Number ipv6NetmaskLength;
/**
* Sets the value of {@link VpcCidrOptions#getAmazonProvided}
* @param amazonProvided Use amazon provided IP range.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder amazonProvided(java.lang.Boolean amazonProvided) {
this.amazonProvided = amazonProvided;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getCidrBlockName}
* @param cidrBlockName Required to set Secondary cidr block resource name in order to generate unique logical id for the resource.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder cidrBlockName(java.lang.String cidrBlockName) {
this.cidrBlockName = cidrBlockName;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getDependencies}
* @param dependencies Dependency to associate Ipv6 CIDR block.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder dependencies(java.util.List extends software.amazon.awscdk.CfnResource> dependencies) {
this.dependencies = (java.util.List)dependencies;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getIpv4CidrBlock}
* @param ipv4CidrBlock IPv4 CIDR Block.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv4CidrBlock(java.lang.String ipv4CidrBlock) {
this.ipv4CidrBlock = ipv4CidrBlock;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getIpv4IpamPool}
* @param ipv4IpamPool Ipv4 IPAM Pool.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv4IpamPool(software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv4IpamPool) {
this.ipv4IpamPool = ipv4IpamPool;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getIpv4NetmaskLength}
* @param ipv4NetmaskLength CIDR Mask for Vpc.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv4NetmaskLength(java.lang.Number ipv4NetmaskLength) {
this.ipv4NetmaskLength = ipv4NetmaskLength;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getIpv6IpamPool}
* @param ipv6IpamPool Ipv6 IPAM pool id for VPC range, can only be defined under public scope.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv6IpamPool(software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv6IpamPool) {
this.ipv6IpamPool = ipv6IpamPool;
return this;
}
/**
* Sets the value of {@link VpcCidrOptions#getIpv6NetmaskLength}
* @param ipv6NetmaskLength CIDR Mask for Vpc.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ipv6NetmaskLength(java.lang.Number ipv6NetmaskLength) {
this.ipv6NetmaskLength = ipv6NetmaskLength;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link VpcCidrOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public VpcCidrOptions build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link VpcCidrOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements VpcCidrOptions {
private final java.lang.Boolean amazonProvided;
private final java.lang.String cidrBlockName;
private final java.util.List dependencies;
private final java.lang.String ipv4CidrBlock;
private final software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv4IpamPool;
private final java.lang.Number ipv4NetmaskLength;
private final software.amazon.awscdk.services.ec2.alpha.IIpamPool ipv6IpamPool;
private final java.lang.Number ipv6NetmaskLength;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.amazonProvided = software.amazon.jsii.Kernel.get(this, "amazonProvided", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.cidrBlockName = software.amazon.jsii.Kernel.get(this, "cidrBlockName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.dependencies = software.amazon.jsii.Kernel.get(this, "dependencies", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.CfnResource.class)));
this.ipv4CidrBlock = software.amazon.jsii.Kernel.get(this, "ipv4CidrBlock", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.ipv4IpamPool = software.amazon.jsii.Kernel.get(this, "ipv4IpamPool", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.alpha.IIpamPool.class));
this.ipv4NetmaskLength = software.amazon.jsii.Kernel.get(this, "ipv4NetmaskLength", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.ipv6IpamPool = software.amazon.jsii.Kernel.get(this, "ipv6IpamPool", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.alpha.IIpamPool.class));
this.ipv6NetmaskLength = software.amazon.jsii.Kernel.get(this, "ipv6NetmaskLength", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.amazonProvided = builder.amazonProvided;
this.cidrBlockName = builder.cidrBlockName;
this.dependencies = (java.util.List)builder.dependencies;
this.ipv4CidrBlock = builder.ipv4CidrBlock;
this.ipv4IpamPool = builder.ipv4IpamPool;
this.ipv4NetmaskLength = builder.ipv4NetmaskLength;
this.ipv6IpamPool = builder.ipv6IpamPool;
this.ipv6NetmaskLength = builder.ipv6NetmaskLength;
}
@Override
public final java.lang.Boolean getAmazonProvided() {
return this.amazonProvided;
}
@Override
public final java.lang.String getCidrBlockName() {
return this.cidrBlockName;
}
@Override
public final java.util.List getDependencies() {
return this.dependencies;
}
@Override
public final java.lang.String getIpv4CidrBlock() {
return this.ipv4CidrBlock;
}
@Override
public final software.amazon.awscdk.services.ec2.alpha.IIpamPool getIpv4IpamPool() {
return this.ipv4IpamPool;
}
@Override
public final java.lang.Number getIpv4NetmaskLength() {
return this.ipv4NetmaskLength;
}
@Override
public final software.amazon.awscdk.services.ec2.alpha.IIpamPool getIpv6IpamPool() {
return this.ipv6IpamPool;
}
@Override
public final java.lang.Number getIpv6NetmaskLength() {
return this.ipv6NetmaskLength;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAmazonProvided() != null) {
data.set("amazonProvided", om.valueToTree(this.getAmazonProvided()));
}
if (this.getCidrBlockName() != null) {
data.set("cidrBlockName", om.valueToTree(this.getCidrBlockName()));
}
if (this.getDependencies() != null) {
data.set("dependencies", om.valueToTree(this.getDependencies()));
}
if (this.getIpv4CidrBlock() != null) {
data.set("ipv4CidrBlock", om.valueToTree(this.getIpv4CidrBlock()));
}
if (this.getIpv4IpamPool() != null) {
data.set("ipv4IpamPool", om.valueToTree(this.getIpv4IpamPool()));
}
if (this.getIpv4NetmaskLength() != null) {
data.set("ipv4NetmaskLength", om.valueToTree(this.getIpv4NetmaskLength()));
}
if (this.getIpv6IpamPool() != null) {
data.set("ipv6IpamPool", om.valueToTree(this.getIpv6IpamPool()));
}
if (this.getIpv6NetmaskLength() != null) {
data.set("ipv6NetmaskLength", om.valueToTree(this.getIpv6NetmaskLength()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ec2-alpha.VpcCidrOptions"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
VpcCidrOptions.Jsii$Proxy that = (VpcCidrOptions.Jsii$Proxy) o;
if (this.amazonProvided != null ? !this.amazonProvided.equals(that.amazonProvided) : that.amazonProvided != null) return false;
if (this.cidrBlockName != null ? !this.cidrBlockName.equals(that.cidrBlockName) : that.cidrBlockName != null) return false;
if (this.dependencies != null ? !this.dependencies.equals(that.dependencies) : that.dependencies != null) return false;
if (this.ipv4CidrBlock != null ? !this.ipv4CidrBlock.equals(that.ipv4CidrBlock) : that.ipv4CidrBlock != null) return false;
if (this.ipv4IpamPool != null ? !this.ipv4IpamPool.equals(that.ipv4IpamPool) : that.ipv4IpamPool != null) return false;
if (this.ipv4NetmaskLength != null ? !this.ipv4NetmaskLength.equals(that.ipv4NetmaskLength) : that.ipv4NetmaskLength != null) return false;
if (this.ipv6IpamPool != null ? !this.ipv6IpamPool.equals(that.ipv6IpamPool) : that.ipv6IpamPool != null) return false;
return this.ipv6NetmaskLength != null ? this.ipv6NetmaskLength.equals(that.ipv6NetmaskLength) : that.ipv6NetmaskLength == null;
}
@Override
public final int hashCode() {
int result = this.amazonProvided != null ? this.amazonProvided.hashCode() : 0;
result = 31 * result + (this.cidrBlockName != null ? this.cidrBlockName.hashCode() : 0);
result = 31 * result + (this.dependencies != null ? this.dependencies.hashCode() : 0);
result = 31 * result + (this.ipv4CidrBlock != null ? this.ipv4CidrBlock.hashCode() : 0);
result = 31 * result + (this.ipv4IpamPool != null ? this.ipv4IpamPool.hashCode() : 0);
result = 31 * result + (this.ipv4NetmaskLength != null ? this.ipv4NetmaskLength.hashCode() : 0);
result = 31 * result + (this.ipv6IpamPool != null ? this.ipv6IpamPool.hashCode() : 0);
result = 31 * result + (this.ipv6NetmaskLength != null ? this.ipv6NetmaskLength.hashCode() : 0);
return result;
}
}
}