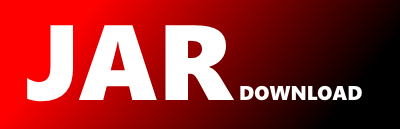
software.amazon.awscdk.services.ecr.IRepository Maven / Gradle / Ivy
package software.amazon.awscdk.services.ecr;
/**
* Represents an ECR repository.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.7.0 (build 179a3a5)", date = "2020-07-01T08:46:46.922Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecr.$Module.class, fqn = "@aws-cdk/aws-ecr.IRepository")
@software.amazon.jsii.Jsii.Proxy(IRepository.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface IRepository extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.core.IResource {
/**
* The ARN of the repository.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRepositoryArn();
/**
* The name of the repository.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRepositoryName();
/**
* The URI of this repository (represents the latest image):.
*
* ACCOUNT.dkr.ecr.REGION.amazonaws.com/REPOSITORY
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRepositoryUri();
/**
* Add a policy statement to the repository's resource policy.
*
* @param statement This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement);
/**
* Grant the given principal identity permissions to perform the actions on this repository.
*
* @param grantee This parameter is required.
* @param actions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grant(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee, final @org.jetbrains.annotations.NotNull java.lang.String... actions);
/**
* Grant the given identity permissions to pull images in this repository.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grantPull(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee);
/**
* Grant the given identity permissions to pull and push images to this repository.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grantPullPush(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee);
/**
* Define a CloudWatch event that triggers when something happens to this repository.
*
* Requires that there exists at least one CloudTrail Trail in your account
* that captures the event. This method will not create the Trail.
*
* @param id The id of the rule. This parameter is required.
* @param options Options for adding the rule.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onCloudTrailEvent(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.events.OnEventOptions options);
/**
* Define a CloudWatch event that triggers when something happens to this repository.
*
* Requires that there exists at least one CloudTrail Trail in your account
* that captures the event. This method will not create the Trail.
*
* @param id The id of the rule. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onCloudTrailEvent(final @org.jetbrains.annotations.NotNull java.lang.String id);
/**
* Defines an AWS CloudWatch event rule that can trigger a target when an image is pushed to this repository.
*
* Requires that there exists at least one CloudTrail Trail in your account
* that captures the event. This method will not create the Trail.
*
* @param id The id of the rule. This parameter is required.
* @param options Options for adding the rule.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onCloudTrailImagePushed(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecr.OnCloudTrailImagePushedOptions options);
/**
* Defines an AWS CloudWatch event rule that can trigger a target when an image is pushed to this repository.
*
* Requires that there exists at least one CloudTrail Trail in your account
* that captures the event. This method will not create the Trail.
*
* @param id The id of the rule. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onCloudTrailImagePushed(final @org.jetbrains.annotations.NotNull java.lang.String id);
/**
* Defines a CloudWatch event rule which triggers for repository events.
*
* Use
* rule.addEventPattern(pattern)
to specify a filter.
*
* @param id This parameter is required.
* @param options
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onEvent(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.events.OnEventOptions options);
/**
* Defines a CloudWatch event rule which triggers for repository events.
*
* Use
* rule.addEventPattern(pattern)
to specify a filter.
*
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onEvent(final @org.jetbrains.annotations.NotNull java.lang.String id);
/**
* Defines an AWS CloudWatch event rule that can trigger a target when the image scan is completed.
*
* @param id The id of the rule. This parameter is required.
* @param options Options for adding the rule.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onImageScanCompleted(final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecr.OnImageScanCompletedOptions options);
/**
* Defines an AWS CloudWatch event rule that can trigger a target when the image scan is completed.
*
* @param id The id of the rule. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.events.Rule onImageScanCompleted(final @org.jetbrains.annotations.NotNull java.lang.String id);
/**
* Returns the URI of the repository for a certain tag. Can be used in `docker push/pull`.
*
* ACCOUNT.dkr.ecr.REGION.amazonaws.com/REPOSITORY[:TAG]
*
* @param tag Image tag to use (tools usually default to "latest" if omitted).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String repositoryUriForTag(final @org.jetbrains.annotations.Nullable java.lang.String tag);
/**
* Returns the URI of the repository for a certain tag. Can be used in `docker push/pull`.
*
* ACCOUNT.dkr.ecr.REGION.amazonaws.com/REPOSITORY[:TAG]
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String repositoryUriForTag();
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.ecr.IRepository {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
/**
* The ARN of the repository.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getRepositoryArn() {
return this.jsiiGet("repositoryArn", java.lang.String.class);
}
/**
* The name of the repository.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getRepositoryName() {
return this.jsiiGet("repositoryName", java.lang.String.class);
}
/**
* The URI of this repository (represents the latest image):.
*
* ACCOUNT.dkr.ecr.REGION.amazonaws.com/REPOSITORY
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getRepositoryUri() {
return this.jsiiGet("repositoryUri", java.lang.String.class);
}
/**
* The stack in which this resource is defined.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Stack getStack() {
return this.jsiiGet("stack", software.amazon.awscdk.core.Stack.class);
}
/**
* The construct tree node for this construct.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.ConstructNode getNode() {
return this.jsiiGet("node", software.amazon.awscdk.core.ConstructNode.class);
}
/**
* Add a policy statement to the repository's resource policy.
*
* @param statement This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement) {
return this.jsiiCall("addToResourcePolicy", software.amazon.awscdk.services.iam.AddToResourcePolicyResult.class, new Object[] { java.util.Objects.requireNonNull(statement, "statement is required") });
}
/**
* Grant the given principal identity permissions to perform the actions on this repository.
*
* @param grantee This parameter is required.
* @param actions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grant(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee, final @org.jetbrains.annotations.NotNull java.lang.String... actions) {
return this.jsiiCall("grant", software.amazon.awscdk.services.iam.Grant.class, java.util.stream.Stream.concat(java.util.Arrays.