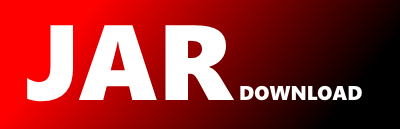
software.amazon.awscdk.services.ecs.FirelensLogRouter Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* Firelens log router.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.29.0 (build 41df200)", date = "2021-05-25T18:24:57.055Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.FirelensLogRouter")
public class FirelensLogRouter extends software.amazon.awscdk.services.ecs.ContainerDefinition {
protected FirelensLogRouter(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected FirelensLogRouter(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the FirelensLogRouter class.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public FirelensLogRouter(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.FirelensLogRouterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Render this container definition to a CloudFormation object.
*
* @param _taskDefinition
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.CfnTaskDefinition.ContainerDefinitionProperty renderContainerDefinition(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.TaskDefinition _taskDefinition) {
return software.amazon.jsii.Kernel.call(this, "renderContainerDefinition", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.CfnTaskDefinition.ContainerDefinitionProperty.class), new Object[] { _taskDefinition });
}
/**
* Render this container definition to a CloudFormation object.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.CfnTaskDefinition.ContainerDefinitionProperty renderContainerDefinition() {
return software.amazon.jsii.Kernel.call(this, "renderContainerDefinition", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.CfnTaskDefinition.ContainerDefinitionProperty.class));
}
/**
* Firelens configuration.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.FirelensConfig getFirelensConfig() {
return software.amazon.jsii.Kernel.get(this, "firelensConfig", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.FirelensConfig.class));
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.FirelensLogRouter}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.ecs.FirelensLogRouterProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.ecs.FirelensLogRouterProps.Builder();
}
/**
* The image used to start a container.
*
* This string is passed directly to the Docker daemon.
* Images in the Docker Hub registry are available by default.
* Other repositories are specified with either repository-url/image:tag or repository-url/image@digest.
* TODO: Update these to specify using classes of IContainerImage
*
* @return {@code this}
* @param image The image used to start a container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder image(final software.amazon.awscdk.services.ecs.ContainerImage image) {
this.props.image(image);
return this;
}
/**
* The command that is passed to the container.
*
* If you provide a shell command as a single string, you have to quote command-line arguments.
*
* Default: - CMD value built into container image.
*
* @return {@code this}
* @param command The command that is passed to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder command(final java.util.List command) {
this.props.command(command);
return this;
}
/**
* The name of the container.
*
* Default: - id of node associated with ContainerDefinition.
*
* @return {@code this}
* @param containerName The name of the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerName(final java.lang.String containerName) {
this.props.containerName(containerName);
return this;
}
/**
* The minimum number of CPU units to reserve for the container.
*
* Default: - No minimum CPU units reserved.
*
* @return {@code this}
* @param cpu The minimum number of CPU units to reserve for the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cpu(final java.lang.Number cpu) {
this.props.cpu(cpu);
return this;
}
/**
* Specifies whether networking is disabled within the container.
*
* When this parameter is true, networking is disabled within the container.
*
* Default: false
*
* @return {@code this}
* @param disableNetworking Specifies whether networking is disabled within the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder disableNetworking(final java.lang.Boolean disableNetworking) {
this.props.disableNetworking(disableNetworking);
return this;
}
/**
* A list of DNS search domains that are presented to the container.
*
* Default: - No search domains.
*
* @return {@code this}
* @param dnsSearchDomains A list of DNS search domains that are presented to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dnsSearchDomains(final java.util.List dnsSearchDomains) {
this.props.dnsSearchDomains(dnsSearchDomains);
return this;
}
/**
* A list of DNS servers that are presented to the container.
*
* Default: - Default DNS servers.
*
* @return {@code this}
* @param dnsServers A list of DNS servers that are presented to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dnsServers(final java.util.List dnsServers) {
this.props.dnsServers(dnsServers);
return this;
}
/**
* A key/value map of labels to add to the container.
*
* Default: - No labels.
*
* @return {@code this}
* @param dockerLabels A key/value map of labels to add to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dockerLabels(final java.util.Map dockerLabels) {
this.props.dockerLabels(dockerLabels);
return this;
}
/**
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
* Default: - No security labels.
*
* @return {@code this}
* @param dockerSecurityOptions A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dockerSecurityOptions(final java.util.List dockerSecurityOptions) {
this.props.dockerSecurityOptions(dockerSecurityOptions);
return this;
}
/**
* The ENTRYPOINT value to pass to the container.
*
* Default: - Entry point configured in container.
*
* @return {@code this}
* @see https://docs.docker.com/engine/reference/builder/#entrypoint
* @param entryPoint The ENTRYPOINT value to pass to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder entryPoint(final java.util.List entryPoint) {
this.props.entryPoint(entryPoint);
return this;
}
/**
* The environment variables to pass to the container.
*
* Default: - No environment variables.
*
* @return {@code this}
* @param environment The environment variables to pass to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(final java.util.Map environment) {
this.props.environment(environment);
return this;
}
/**
* The environment files to pass to the container.
*
* Default: - No environment files.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AmazonECS/latest/developerguide/taskdef-envfiles.html
* @param environmentFiles The environment files to pass to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environmentFiles(final java.util.List extends software.amazon.awscdk.services.ecs.EnvironmentFile> environmentFiles) {
this.props.environmentFiles(environmentFiles);
return this;
}
/**
* Specifies whether the container is marked essential.
*
* If the essential parameter of a container is marked as true, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped.
* If the essential parameter of a container is marked as false, then its failure does not
* affect the rest of the containers in a task. All tasks must have at least one essential container.
*
* If this parameter is omitted, a container is assumed to be essential.
*
* Default: true
*
* @return {@code this}
* @param essential Specifies whether the container is marked essential. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder essential(final java.lang.Boolean essential) {
this.props.essential(essential);
return this;
}
/**
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
* Default: - No extra hosts.
*
* @return {@code this}
* @param extraHosts A list of hostnames and IP address mappings to append to the /etc/hosts file on the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder extraHosts(final java.util.Map extraHosts) {
this.props.extraHosts(extraHosts);
return this;
}
/**
* The number of GPUs assigned to the container.
*
* Default: - No GPUs assigned.
*
* @return {@code this}
* @param gpuCount The number of GPUs assigned to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gpuCount(final java.lang.Number gpuCount) {
this.props.gpuCount(gpuCount);
return this;
}
/**
* The health check command and associated configuration parameters for the container.
*
* Default: - Health check configuration from container.
*
* @return {@code this}
* @param healthCheck The health check command and associated configuration parameters for the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheck(final software.amazon.awscdk.services.ecs.HealthCheck healthCheck) {
this.props.healthCheck(healthCheck);
return this;
}
/**
* The hostname to use for your container.
*
* Default: - Automatic hostname.
*
* @return {@code this}
* @param hostname The hostname to use for your container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder hostname(final java.lang.String hostname) {
this.props.hostname(hostname);
return this;
}
/**
* The inference accelerators referenced by the container.
*
* Default: - No inference accelerators assigned.
*
* @return {@code this}
* @param inferenceAcceleratorResources The inference accelerators referenced by the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceAcceleratorResources(final java.util.List inferenceAcceleratorResources) {
this.props.inferenceAcceleratorResources(inferenceAcceleratorResources);
return this;
}
/**
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*
* For more information see KernelCapabilities.
*
* Default: - No Linux parameters.
*
* @return {@code this}
* @param linuxParameters Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder linuxParameters(final software.amazon.awscdk.services.ecs.LinuxParameters linuxParameters) {
this.props.linuxParameters(linuxParameters);
return this;
}
/**
* The log configuration specification for the container.
*
* Default: - Containers use the same logging driver that the Docker daemon uses.
*
* @return {@code this}
* @param logging The log configuration specification for the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logging(final software.amazon.awscdk.services.ecs.LogDriver logging) {
this.props.logging(logging);
return this;
}
/**
* The amount (in MiB) of memory to present to the container.
*
* If your container attempts to exceed the allocated memory, the container
* is terminated.
*
* At least one of memoryLimitMiB and memoryReservationMiB is required for non-Fargate services.
*
* Default: - No memory limit.
*
* @return {@code this}
* @param memoryLimitMiB The amount (in MiB) of memory to present to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memoryLimitMiB(final java.lang.Number memoryLimitMiB) {
this.props.memoryLimitMiB(memoryLimitMiB);
return this;
}
/**
* The soft limit (in MiB) of memory to reserve for the container.
*
* When system memory is under heavy contention, Docker attempts to keep the
* container memory to this soft limit. However, your container can consume more
* memory when it needs to, up to either the hard limit specified with the memory
* parameter (if applicable), or all of the available memory on the container
* instance, whichever comes first.
*
* At least one of memoryLimitMiB and memoryReservationMiB is required for non-Fargate services.
*
* Default: - No memory reserved.
*
* @return {@code this}
* @param memoryReservationMiB The soft limit (in MiB) of memory to reserve for the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memoryReservationMiB(final java.lang.Number memoryReservationMiB) {
this.props.memoryReservationMiB(memoryReservationMiB);
return this;
}
/**
* The port mappings to add to the container definition.
*
* Default: - No ports are mapped.
*
* @return {@code this}
* @param portMappings The port mappings to add to the container definition. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder portMappings(final java.util.List extends software.amazon.awscdk.services.ecs.PortMapping> portMappings) {
this.props.portMappings(portMappings);
return this;
}
/**
* Specifies whether the container is marked as privileged.
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar to the root user).
*
* Default: false
*
* @return {@code this}
* @param privileged Specifies whether the container is marked as privileged. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder privileged(final java.lang.Boolean privileged) {
this.props.privileged(privileged);
return this;
}
/**
* When this parameter is true, the container is given read-only access to its root file system.
*
* Default: false
*
* @return {@code this}
* @param readonlyRootFilesystem When this parameter is true, the container is given read-only access to its root file system. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder readonlyRootFilesystem(final java.lang.Boolean readonlyRootFilesystem) {
this.props.readonlyRootFilesystem(readonlyRootFilesystem);
return this;
}
/**
* The secret environment variables to pass to the container.
*
* Default: - No secret environment variables.
*
* @return {@code this}
* @param secrets The secret environment variables to pass to the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secrets(final java.util.Map secrets) {
this.props.secrets(secrets);
return this;
}
/**
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container.
*
* Default: - none
*
* @return {@code this}
* @param startTimeout Time duration (in seconds) to wait before giving up on resolving dependencies for a container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder startTimeout(final software.amazon.awscdk.core.Duration startTimeout) {
this.props.startTimeout(startTimeout);
return this;
}
/**
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its own.
*
* Default: - none
*
* @return {@code this}
* @param stopTimeout Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its own. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stopTimeout(final software.amazon.awscdk.core.Duration stopTimeout) {
this.props.stopTimeout(stopTimeout);
return this;
}
/**
* The user name to use inside the container.
*
* Default: root
*
* @return {@code this}
* @param user The user name to use inside the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder user(final java.lang.String user) {
this.props.user(user);
return this;
}
/**
* The working directory in which to run commands inside the container.
*
* Default: /
*
* @return {@code this}
* @param workingDirectory The working directory in which to run commands inside the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workingDirectory(final java.lang.String workingDirectory) {
this.props.workingDirectory(workingDirectory);
return this;
}
/**
* The name of the task definition that includes this container definition.
*
* [disable-awslint:ref-via-interface]
*
* @return {@code this}
* @param taskDefinition The name of the task definition that includes this container definition. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskDefinition(final software.amazon.awscdk.services.ecs.TaskDefinition taskDefinition) {
this.props.taskDefinition(taskDefinition);
return this;
}
/**
* Firelens configuration.
*
* @return {@code this}
* @param firelensConfig Firelens configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder firelensConfig(final software.amazon.awscdk.services.ecs.FirelensConfig firelensConfig) {
this.props.firelensConfig(firelensConfig);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.FirelensLogRouter}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.FirelensLogRouter build() {
return new software.amazon.awscdk.services.ecs.FirelensLogRouter(
this.scope,
this.id,
this.props.build()
);
}
}
}