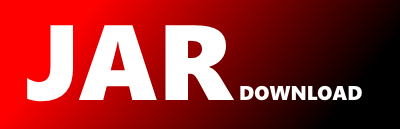
software.amazon.awscdk.services.ecs.AddCapacityOptions Maven / Gradle / Ivy
package software.amazon.awscdk.services.ecs;
/**
* The properties for adding instance capacity to an AutoScalingGroup.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.31.0 (build 6fa403d)", date = "2021-07-21T15:56:51.140Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.AddCapacityOptions")
@software.amazon.jsii.Jsii.Proxy(AddCapacityOptions.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AddCapacityOptions extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.AddAutoScalingGroupCapacityOptions, software.amazon.awscdk.services.autoscaling.CommonAutoScalingGroupProps {
/**
* The EC2 instance type to use when launching instances into the AutoScalingGroup.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.InstanceType getInstanceType();
/**
* The ECS-optimized AMI variant to use.
*
* For more information, see
* Amazon ECS-optimized AMIs.
* You must define either machineImage
or machineImageType
, not both.
*
* Default: - Amazon Linux 2
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.IMachineImage getMachineImage() {
return null;
}
/**
* @return a {@link Builder} of {@link AddCapacityOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AddCapacityOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private software.amazon.awscdk.services.ec2.InstanceType instanceType;
private software.amazon.awscdk.services.ec2.IMachineImage machineImage;
private java.lang.Boolean canContainersAccessInstanceRole;
private software.amazon.awscdk.services.ecs.MachineImageType machineImageType;
private java.lang.Boolean spotInstanceDraining;
private software.amazon.awscdk.core.Duration taskDrainTime;
private software.amazon.awscdk.services.kms.IKey topicEncryptionKey;
private java.lang.Boolean allowAllOutbound;
private java.lang.Boolean associatePublicIpAddress;
private java.lang.String autoScalingGroupName;
private java.util.List blockDevices;
private software.amazon.awscdk.core.Duration cooldown;
private java.lang.Number desiredCapacity;
private java.util.List groupMetrics;
private software.amazon.awscdk.services.autoscaling.HealthCheck healthCheck;
private java.lang.Boolean ignoreUnmodifiedSizeProperties;
private software.amazon.awscdk.services.autoscaling.Monitoring instanceMonitoring;
private java.lang.String keyName;
private java.lang.Number maxCapacity;
private software.amazon.awscdk.core.Duration maxInstanceLifetime;
private java.lang.Number minCapacity;
private java.lang.Boolean newInstancesProtectedFromScaleIn;
private java.util.List notifications;
private software.amazon.awscdk.services.sns.ITopic notificationsTopic;
private java.lang.Number replacingUpdateMinSuccessfulInstancesPercent;
private java.lang.Number resourceSignalCount;
private software.amazon.awscdk.core.Duration resourceSignalTimeout;
private software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration rollingUpdateConfiguration;
private software.amazon.awscdk.services.autoscaling.Signals signals;
private java.lang.String spotPrice;
private software.amazon.awscdk.services.autoscaling.UpdatePolicy updatePolicy;
private software.amazon.awscdk.services.autoscaling.UpdateType updateType;
private software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link AddCapacityOptions#getInstanceType}
* @param instanceType The EC2 instance type to use when launching instances into the AutoScalingGroup. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceType(software.amazon.awscdk.services.ec2.InstanceType instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getMachineImage}
* @param machineImage The ECS-optimized AMI variant to use.
* For more information, see
* Amazon ECS-optimized AMIs.
* You must define either machineImage
or machineImageType
, not both.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder machineImage(software.amazon.awscdk.services.ec2.IMachineImage machineImage) {
this.machineImage = machineImage;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getCanContainersAccessInstanceRole}
* @param canContainersAccessInstanceRole Specifies whether the containers can access the container instance role.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder canContainersAccessInstanceRole(java.lang.Boolean canContainersAccessInstanceRole) {
this.canContainersAccessInstanceRole = canContainersAccessInstanceRole;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getMachineImageType}
* @param machineImageType Specify the machine image type.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder machineImageType(software.amazon.awscdk.services.ecs.MachineImageType machineImageType) {
this.machineImageType = machineImageType;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getSpotInstanceDraining}
* @param spotInstanceDraining Specify whether to enable Automated Draining for Spot Instances running Amazon ECS Services.
* For more information, see Using Spot Instances.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder spotInstanceDraining(java.lang.Boolean spotInstanceDraining) {
this.spotInstanceDraining = spotInstanceDraining;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getTaskDrainTime}
* @param taskDrainTime The time period to wait before force terminating an instance that is draining.
* This creates a Lambda function that is used by a lifecycle hook for the
* AutoScalingGroup that will delay instance termination until all ECS tasks
* have drained from the instance. Set to 0 to disable task draining.
*
* Set to 0 to disable task draining.
* @return {@code this}
* @deprecated The lifecycle draining hook is not configured if using the EC2 Capacity Provider. Enable managed termination protection instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder taskDrainTime(software.amazon.awscdk.core.Duration taskDrainTime) {
this.taskDrainTime = taskDrainTime;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getTopicEncryptionKey}
* @param topicEncryptionKey If {@link AddAutoScalingGroupCapacityOptions.taskDrainTime} is non-zero, then the ECS cluster creates an SNS Topic to as part of a system to drain instances of tasks when the instance is being shut down. If this property is provided, then this key will be used to encrypt the contents of that SNS Topic. See [SNS Data Encryption](https://docs.aws.amazon.com/sns/latest/dg/sns-data-encryption.html) for more information.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder topicEncryptionKey(software.amazon.awscdk.services.kms.IKey topicEncryptionKey) {
this.topicEncryptionKey = topicEncryptionKey;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getAllowAllOutbound}
* @param allowAllOutbound Whether the instances can initiate connections to anywhere by default.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder allowAllOutbound(java.lang.Boolean allowAllOutbound) {
this.allowAllOutbound = allowAllOutbound;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getAssociatePublicIpAddress}
* @param associatePublicIpAddress Whether instances in the Auto Scaling Group should have public IP addresses associated with them.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder associatePublicIpAddress(java.lang.Boolean associatePublicIpAddress) {
this.associatePublicIpAddress = associatePublicIpAddress;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getAutoScalingGroupName}
* @param autoScalingGroupName The name of the Auto Scaling group.
* This name must be unique per Region per account.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoScalingGroupName(java.lang.String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getBlockDevices}
* @param blockDevices Specifies how block devices are exposed to the instance. You can specify virtual devices and EBS volumes.
* Each instance that is launched has an associated root device volume,
* either an Amazon EBS volume or an instance store volume.
* You can use block device mappings to specify additional EBS volumes or
* instance store volumes to attach to an instance when it is launched.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder blockDevices(java.util.List extends software.amazon.awscdk.services.autoscaling.BlockDevice> blockDevices) {
this.blockDevices = (java.util.List)blockDevices;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getCooldown}
* @param cooldown Default scaling cooldown for this AutoScalingGroup.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cooldown(software.amazon.awscdk.core.Duration cooldown) {
this.cooldown = cooldown;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getDesiredCapacity}
* @param desiredCapacity Initial amount of instances in the fleet.
* If this is set to a number, every deployment will reset the amount of
* instances to this number. It is recommended to leave this value blank.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder desiredCapacity(java.lang.Number desiredCapacity) {
this.desiredCapacity = desiredCapacity;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getGroupMetrics}
* @param groupMetrics Enable monitoring for group metrics, these metrics describe the group rather than any of its instances.
* To report all group metrics use GroupMetrics.all()
* Group metrics are reported in a granularity of 1 minute at no additional charge.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder groupMetrics(java.util.List extends software.amazon.awscdk.services.autoscaling.GroupMetrics> groupMetrics) {
this.groupMetrics = (java.util.List)groupMetrics;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getHealthCheck}
* @param healthCheck Configuration for health checks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheck(software.amazon.awscdk.services.autoscaling.HealthCheck healthCheck) {
this.healthCheck = healthCheck;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getIgnoreUnmodifiedSizeProperties}
* @param ignoreUnmodifiedSizeProperties If the ASG has scheduled actions, don't reset unchanged group sizes.
* Only used if the ASG has scheduled actions (which may scale your ASG up
* or down regardless of cdk deployments). If true, the size of the group
* will only be reset if it has been changed in the CDK app. If false, the
* sizes will always be changed back to what they were in the CDK app
* on deployment.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreUnmodifiedSizeProperties(java.lang.Boolean ignoreUnmodifiedSizeProperties) {
this.ignoreUnmodifiedSizeProperties = ignoreUnmodifiedSizeProperties;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getInstanceMonitoring}
* @param instanceMonitoring Controls whether instances in this group are launched with detailed or basic monitoring.
* When detailed monitoring is enabled, Amazon CloudWatch generates metrics every minute and your account
* is charged a fee. When you disable detailed monitoring, CloudWatch generates metrics every 5 minutes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceMonitoring(software.amazon.awscdk.services.autoscaling.Monitoring instanceMonitoring) {
this.instanceMonitoring = instanceMonitoring;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getKeyName}
* @param keyName Name of SSH keypair to grant access to instances.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder keyName(java.lang.String keyName) {
this.keyName = keyName;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getMaxCapacity}
* @param maxCapacity Maximum number of instances in the fleet.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxCapacity(java.lang.Number maxCapacity) {
this.maxCapacity = maxCapacity;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getMaxInstanceLifetime}
* @param maxInstanceLifetime The maximum amount of time that an instance can be in service.
* The maximum duration applies
* to all current and future instances in the group. As an instance approaches its maximum duration,
* it is terminated and replaced, and cannot be used again.
*
* You must specify a value of at least 604,800 seconds (7 days). To clear a previously set value,
* leave this property undefined.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxInstanceLifetime(software.amazon.awscdk.core.Duration maxInstanceLifetime) {
this.maxInstanceLifetime = maxInstanceLifetime;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getMinCapacity}
* @param minCapacity Minimum number of instances in the fleet.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder minCapacity(java.lang.Number minCapacity) {
this.minCapacity = minCapacity;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getNewInstancesProtectedFromScaleIn}
* @param newInstancesProtectedFromScaleIn Whether newly-launched instances are protected from termination by Amazon EC2 Auto Scaling when scaling in.
* By default, Auto Scaling can terminate an instance at any time after launch
* when scaling in an Auto Scaling Group, subject to the group's termination
* policy. However, you may wish to protect newly-launched instances from
* being scaled in if they are going to run critical applications that should
* not be prematurely terminated.
*
* This flag must be enabled if the Auto Scaling Group will be associated with
* an ECS Capacity Provider with managed termination protection.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder newInstancesProtectedFromScaleIn(java.lang.Boolean newInstancesProtectedFromScaleIn) {
this.newInstancesProtectedFromScaleIn = newInstancesProtectedFromScaleIn;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getNotifications}
* @param notifications Configure autoscaling group to send notifications about fleet changes to an SNS topic(s).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder notifications(java.util.List extends software.amazon.awscdk.services.autoscaling.NotificationConfiguration> notifications) {
this.notifications = (java.util.List)notifications;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getNotificationsTopic}
* @param notificationsTopic SNS topic to send notifications about fleet changes.
* @return {@code this}
* @deprecated use `notifications`
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder notificationsTopic(software.amazon.awscdk.services.sns.ITopic notificationsTopic) {
this.notificationsTopic = notificationsTopic;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getReplacingUpdateMinSuccessfulInstancesPercent}
* @param replacingUpdateMinSuccessfulInstancesPercent Configuration for replacing updates.
* Only used if updateType == UpdateType.ReplacingUpdate. Specifies how
* many instances must signal success for the update to succeed.
* @return {@code this}
* @deprecated Use `signals` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder replacingUpdateMinSuccessfulInstancesPercent(java.lang.Number replacingUpdateMinSuccessfulInstancesPercent) {
this.replacingUpdateMinSuccessfulInstancesPercent = replacingUpdateMinSuccessfulInstancesPercent;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getResourceSignalCount}
* @param resourceSignalCount How many ResourceSignal calls CloudFormation expects before the resource is considered created.
* @return {@code this}
* @deprecated Use `signals` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder resourceSignalCount(java.lang.Number resourceSignalCount) {
this.resourceSignalCount = resourceSignalCount;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getResourceSignalTimeout}
* @param resourceSignalTimeout The length of time to wait for the resourceSignalCount.
* The maximum value is 43200 (12 hours).
* @return {@code this}
* @deprecated Use `signals` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder resourceSignalTimeout(software.amazon.awscdk.core.Duration resourceSignalTimeout) {
this.resourceSignalTimeout = resourceSignalTimeout;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getRollingUpdateConfiguration}
* @param rollingUpdateConfiguration Configuration for rolling updates.
* Only used if updateType == UpdateType.RollingUpdate.
* @return {@code this}
* @deprecated Use `updatePolicy` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder rollingUpdateConfiguration(software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration rollingUpdateConfiguration) {
this.rollingUpdateConfiguration = rollingUpdateConfiguration;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getSignals}
* @param signals Configure waiting for signals during deployment.
* Use this to pause the CloudFormation deployment to wait for the instances
* in the AutoScalingGroup to report successful startup during
* creation and updates. The UserData script needs to invoke cfn-signal
* with a success or failure code after it is done setting up the instance.
*
* Without waiting for signals, the CloudFormation deployment will proceed as
* soon as the AutoScalingGroup has been created or updated but before the
* instances in the group have been started.
*
* For example, to have instances wait for an Elastic Load Balancing health check before
* they signal success, add a health-check verification by using the
* cfn-init helper script. For an example, see the verify_instance_health
* command in the Auto Scaling rolling updates sample template:
*
* https://github.com/awslabs/aws-cloudformation-templates/blob/master/aws/services/AutoScaling/AutoScalingRollingUpdates.yaml
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder signals(software.amazon.awscdk.services.autoscaling.Signals signals) {
this.signals = signals;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getSpotPrice}
* @param spotPrice The maximum hourly price (in USD) to be paid for any Spot Instance launched to fulfill the request.
* Spot Instances are
* launched when the price you specify exceeds the current Spot market price.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder spotPrice(java.lang.String spotPrice) {
this.spotPrice = spotPrice;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getUpdatePolicy}
* @param updatePolicy What to do when an AutoScalingGroup's instance configuration is changed.
* This is applied when any of the settings on the ASG are changed that
* affect how the instances should be created (VPC, instance type, startup
* scripts, etc.). It indicates how the existing instances should be
* replaced with new instances matching the new config. By default, nothing
* is done and only new instances are launched with the new config.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder updatePolicy(software.amazon.awscdk.services.autoscaling.UpdatePolicy updatePolicy) {
this.updatePolicy = updatePolicy;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getUpdateType}
* @param updateType What to do when an AutoScalingGroup's instance configuration is changed.
* This is applied when any of the settings on the ASG are changed that
* affect how the instances should be created (VPC, instance type, startup
* scripts, etc.). It indicates how the existing instances should be
* replaced with new instances matching the new config. By default, nothing
* is done and only new instances are launched with the new config.
* @return {@code this}
* @deprecated Use `updatePolicy` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder updateType(software.amazon.awscdk.services.autoscaling.UpdateType updateType) {
this.updateType = updateType;
return this;
}
/**
* Sets the value of {@link AddCapacityOptions#getVpcSubnets}
* @param vpcSubnets Where to place instances within the VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AddCapacityOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AddCapacityOptions build() {
return new Jsii$Proxy(instanceType, machineImage, canContainersAccessInstanceRole, machineImageType, spotInstanceDraining, taskDrainTime, topicEncryptionKey, allowAllOutbound, associatePublicIpAddress, autoScalingGroupName, blockDevices, cooldown, desiredCapacity, groupMetrics, healthCheck, ignoreUnmodifiedSizeProperties, instanceMonitoring, keyName, maxCapacity, maxInstanceLifetime, minCapacity, newInstancesProtectedFromScaleIn, notifications, notificationsTopic, replacingUpdateMinSuccessfulInstancesPercent, resourceSignalCount, resourceSignalTimeout, rollingUpdateConfiguration, signals, spotPrice, updatePolicy, updateType, vpcSubnets);
}
}
/**
* An implementation for {@link AddCapacityOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AddCapacityOptions {
private final software.amazon.awscdk.services.ec2.InstanceType instanceType;
private final software.amazon.awscdk.services.ec2.IMachineImage machineImage;
private final java.lang.Boolean canContainersAccessInstanceRole;
private final software.amazon.awscdk.services.ecs.MachineImageType machineImageType;
private final java.lang.Boolean spotInstanceDraining;
private final software.amazon.awscdk.core.Duration taskDrainTime;
private final software.amazon.awscdk.services.kms.IKey topicEncryptionKey;
private final java.lang.Boolean allowAllOutbound;
private final java.lang.Boolean associatePublicIpAddress;
private final java.lang.String autoScalingGroupName;
private final java.util.List blockDevices;
private final software.amazon.awscdk.core.Duration cooldown;
private final java.lang.Number desiredCapacity;
private final java.util.List groupMetrics;
private final software.amazon.awscdk.services.autoscaling.HealthCheck healthCheck;
private final java.lang.Boolean ignoreUnmodifiedSizeProperties;
private final software.amazon.awscdk.services.autoscaling.Monitoring instanceMonitoring;
private final java.lang.String keyName;
private final java.lang.Number maxCapacity;
private final software.amazon.awscdk.core.Duration maxInstanceLifetime;
private final java.lang.Number minCapacity;
private final java.lang.Boolean newInstancesProtectedFromScaleIn;
private final java.util.List notifications;
private final software.amazon.awscdk.services.sns.ITopic notificationsTopic;
private final java.lang.Number replacingUpdateMinSuccessfulInstancesPercent;
private final java.lang.Number resourceSignalCount;
private final software.amazon.awscdk.core.Duration resourceSignalTimeout;
private final software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration rollingUpdateConfiguration;
private final software.amazon.awscdk.services.autoscaling.Signals signals;
private final java.lang.String spotPrice;
private final software.amazon.awscdk.services.autoscaling.UpdatePolicy updatePolicy;
private final software.amazon.awscdk.services.autoscaling.UpdateType updateType;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.instanceType = software.amazon.jsii.Kernel.get(this, "instanceType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.InstanceType.class));
this.machineImage = software.amazon.jsii.Kernel.get(this, "machineImage", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IMachineImage.class));
this.canContainersAccessInstanceRole = software.amazon.jsii.Kernel.get(this, "canContainersAccessInstanceRole", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.machineImageType = software.amazon.jsii.Kernel.get(this, "machineImageType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.MachineImageType.class));
this.spotInstanceDraining = software.amazon.jsii.Kernel.get(this, "spotInstanceDraining", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.taskDrainTime = software.amazon.jsii.Kernel.get(this, "taskDrainTime", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.topicEncryptionKey = software.amazon.jsii.Kernel.get(this, "topicEncryptionKey", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.kms.IKey.class));
this.allowAllOutbound = software.amazon.jsii.Kernel.get(this, "allowAllOutbound", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.associatePublicIpAddress = software.amazon.jsii.Kernel.get(this, "associatePublicIpAddress", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.autoScalingGroupName = software.amazon.jsii.Kernel.get(this, "autoScalingGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.blockDevices = software.amazon.jsii.Kernel.get(this, "blockDevices", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.BlockDevice.class)));
this.cooldown = software.amazon.jsii.Kernel.get(this, "cooldown", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.desiredCapacity = software.amazon.jsii.Kernel.get(this, "desiredCapacity", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.groupMetrics = software.amazon.jsii.Kernel.get(this, "groupMetrics", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.GroupMetrics.class)));
this.healthCheck = software.amazon.jsii.Kernel.get(this, "healthCheck", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.HealthCheck.class));
this.ignoreUnmodifiedSizeProperties = software.amazon.jsii.Kernel.get(this, "ignoreUnmodifiedSizeProperties", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.instanceMonitoring = software.amazon.jsii.Kernel.get(this, "instanceMonitoring", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.Monitoring.class));
this.keyName = software.amazon.jsii.Kernel.get(this, "keyName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.maxCapacity = software.amazon.jsii.Kernel.get(this, "maxCapacity", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.maxInstanceLifetime = software.amazon.jsii.Kernel.get(this, "maxInstanceLifetime", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.minCapacity = software.amazon.jsii.Kernel.get(this, "minCapacity", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.newInstancesProtectedFromScaleIn = software.amazon.jsii.Kernel.get(this, "newInstancesProtectedFromScaleIn", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.notifications = software.amazon.jsii.Kernel.get(this, "notifications", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.NotificationConfiguration.class)));
this.notificationsTopic = software.amazon.jsii.Kernel.get(this, "notificationsTopic", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.sns.ITopic.class));
this.replacingUpdateMinSuccessfulInstancesPercent = software.amazon.jsii.Kernel.get(this, "replacingUpdateMinSuccessfulInstancesPercent", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.resourceSignalCount = software.amazon.jsii.Kernel.get(this, "resourceSignalCount", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.resourceSignalTimeout = software.amazon.jsii.Kernel.get(this, "resourceSignalTimeout", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.rollingUpdateConfiguration = software.amazon.jsii.Kernel.get(this, "rollingUpdateConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration.class));
this.signals = software.amazon.jsii.Kernel.get(this, "signals", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.Signals.class));
this.spotPrice = software.amazon.jsii.Kernel.get(this, "spotPrice", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.updatePolicy = software.amazon.jsii.Kernel.get(this, "updatePolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.UpdatePolicy.class));
this.updateType = software.amazon.jsii.Kernel.get(this, "updateType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.autoscaling.UpdateType.class));
this.vpcSubnets = software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final software.amazon.awscdk.services.ec2.InstanceType instanceType, final software.amazon.awscdk.services.ec2.IMachineImage machineImage, final java.lang.Boolean canContainersAccessInstanceRole, final software.amazon.awscdk.services.ecs.MachineImageType machineImageType, final java.lang.Boolean spotInstanceDraining, final software.amazon.awscdk.core.Duration taskDrainTime, final software.amazon.awscdk.services.kms.IKey topicEncryptionKey, final java.lang.Boolean allowAllOutbound, final java.lang.Boolean associatePublicIpAddress, final java.lang.String autoScalingGroupName, final java.util.List extends software.amazon.awscdk.services.autoscaling.BlockDevice> blockDevices, final software.amazon.awscdk.core.Duration cooldown, final java.lang.Number desiredCapacity, final java.util.List extends software.amazon.awscdk.services.autoscaling.GroupMetrics> groupMetrics, final software.amazon.awscdk.services.autoscaling.HealthCheck healthCheck, final java.lang.Boolean ignoreUnmodifiedSizeProperties, final software.amazon.awscdk.services.autoscaling.Monitoring instanceMonitoring, final java.lang.String keyName, final java.lang.Number maxCapacity, final software.amazon.awscdk.core.Duration maxInstanceLifetime, final java.lang.Number minCapacity, final java.lang.Boolean newInstancesProtectedFromScaleIn, final java.util.List extends software.amazon.awscdk.services.autoscaling.NotificationConfiguration> notifications, final software.amazon.awscdk.services.sns.ITopic notificationsTopic, final java.lang.Number replacingUpdateMinSuccessfulInstancesPercent, final java.lang.Number resourceSignalCount, final software.amazon.awscdk.core.Duration resourceSignalTimeout, final software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration rollingUpdateConfiguration, final software.amazon.awscdk.services.autoscaling.Signals signals, final java.lang.String spotPrice, final software.amazon.awscdk.services.autoscaling.UpdatePolicy updatePolicy, final software.amazon.awscdk.services.autoscaling.UpdateType updateType, final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.instanceType = java.util.Objects.requireNonNull(instanceType, "instanceType is required");
this.machineImage = machineImage;
this.canContainersAccessInstanceRole = canContainersAccessInstanceRole;
this.machineImageType = machineImageType;
this.spotInstanceDraining = spotInstanceDraining;
this.taskDrainTime = taskDrainTime;
this.topicEncryptionKey = topicEncryptionKey;
this.allowAllOutbound = allowAllOutbound;
this.associatePublicIpAddress = associatePublicIpAddress;
this.autoScalingGroupName = autoScalingGroupName;
this.blockDevices = (java.util.List)blockDevices;
this.cooldown = cooldown;
this.desiredCapacity = desiredCapacity;
this.groupMetrics = (java.util.List)groupMetrics;
this.healthCheck = healthCheck;
this.ignoreUnmodifiedSizeProperties = ignoreUnmodifiedSizeProperties;
this.instanceMonitoring = instanceMonitoring;
this.keyName = keyName;
this.maxCapacity = maxCapacity;
this.maxInstanceLifetime = maxInstanceLifetime;
this.minCapacity = minCapacity;
this.newInstancesProtectedFromScaleIn = newInstancesProtectedFromScaleIn;
this.notifications = (java.util.List)notifications;
this.notificationsTopic = notificationsTopic;
this.replacingUpdateMinSuccessfulInstancesPercent = replacingUpdateMinSuccessfulInstancesPercent;
this.resourceSignalCount = resourceSignalCount;
this.resourceSignalTimeout = resourceSignalTimeout;
this.rollingUpdateConfiguration = rollingUpdateConfiguration;
this.signals = signals;
this.spotPrice = spotPrice;
this.updatePolicy = updatePolicy;
this.updateType = updateType;
this.vpcSubnets = vpcSubnets;
}
@Override
public final software.amazon.awscdk.services.ec2.InstanceType getInstanceType() {
return this.instanceType;
}
@Override
public final software.amazon.awscdk.services.ec2.IMachineImage getMachineImage() {
return this.machineImage;
}
@Override
public final java.lang.Boolean getCanContainersAccessInstanceRole() {
return this.canContainersAccessInstanceRole;
}
@Override
public final software.amazon.awscdk.services.ecs.MachineImageType getMachineImageType() {
return this.machineImageType;
}
@Override
public final java.lang.Boolean getSpotInstanceDraining() {
return this.spotInstanceDraining;
}
@Override
public final software.amazon.awscdk.core.Duration getTaskDrainTime() {
return this.taskDrainTime;
}
@Override
public final software.amazon.awscdk.services.kms.IKey getTopicEncryptionKey() {
return this.topicEncryptionKey;
}
@Override
public final java.lang.Boolean getAllowAllOutbound() {
return this.allowAllOutbound;
}
@Override
public final java.lang.Boolean getAssociatePublicIpAddress() {
return this.associatePublicIpAddress;
}
@Override
public final java.lang.String getAutoScalingGroupName() {
return this.autoScalingGroupName;
}
@Override
public final java.util.List getBlockDevices() {
return this.blockDevices;
}
@Override
public final software.amazon.awscdk.core.Duration getCooldown() {
return this.cooldown;
}
@Override
public final java.lang.Number getDesiredCapacity() {
return this.desiredCapacity;
}
@Override
public final java.util.List getGroupMetrics() {
return this.groupMetrics;
}
@Override
public final software.amazon.awscdk.services.autoscaling.HealthCheck getHealthCheck() {
return this.healthCheck;
}
@Override
public final java.lang.Boolean getIgnoreUnmodifiedSizeProperties() {
return this.ignoreUnmodifiedSizeProperties;
}
@Override
public final software.amazon.awscdk.services.autoscaling.Monitoring getInstanceMonitoring() {
return this.instanceMonitoring;
}
@Override
public final java.lang.String getKeyName() {
return this.keyName;
}
@Override
public final java.lang.Number getMaxCapacity() {
return this.maxCapacity;
}
@Override
public final software.amazon.awscdk.core.Duration getMaxInstanceLifetime() {
return this.maxInstanceLifetime;
}
@Override
public final java.lang.Number getMinCapacity() {
return this.minCapacity;
}
@Override
public final java.lang.Boolean getNewInstancesProtectedFromScaleIn() {
return this.newInstancesProtectedFromScaleIn;
}
@Override
public final java.util.List getNotifications() {
return this.notifications;
}
@Override
public final software.amazon.awscdk.services.sns.ITopic getNotificationsTopic() {
return this.notificationsTopic;
}
@Override
public final java.lang.Number getReplacingUpdateMinSuccessfulInstancesPercent() {
return this.replacingUpdateMinSuccessfulInstancesPercent;
}
@Override
public final java.lang.Number getResourceSignalCount() {
return this.resourceSignalCount;
}
@Override
public final software.amazon.awscdk.core.Duration getResourceSignalTimeout() {
return this.resourceSignalTimeout;
}
@Override
public final software.amazon.awscdk.services.autoscaling.RollingUpdateConfiguration getRollingUpdateConfiguration() {
return this.rollingUpdateConfiguration;
}
@Override
public final software.amazon.awscdk.services.autoscaling.Signals getSignals() {
return this.signals;
}
@Override
public final java.lang.String getSpotPrice() {
return this.spotPrice;
}
@Override
public final software.amazon.awscdk.services.autoscaling.UpdatePolicy getUpdatePolicy() {
return this.updatePolicy;
}
@Override
public final software.amazon.awscdk.services.autoscaling.UpdateType getUpdateType() {
return this.updateType;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("instanceType", om.valueToTree(this.getInstanceType()));
if (this.getMachineImage() != null) {
data.set("machineImage", om.valueToTree(this.getMachineImage()));
}
if (this.getCanContainersAccessInstanceRole() != null) {
data.set("canContainersAccessInstanceRole", om.valueToTree(this.getCanContainersAccessInstanceRole()));
}
if (this.getMachineImageType() != null) {
data.set("machineImageType", om.valueToTree(this.getMachineImageType()));
}
if (this.getSpotInstanceDraining() != null) {
data.set("spotInstanceDraining", om.valueToTree(this.getSpotInstanceDraining()));
}
if (this.getTaskDrainTime() != null) {
data.set("taskDrainTime", om.valueToTree(this.getTaskDrainTime()));
}
if (this.getTopicEncryptionKey() != null) {
data.set("topicEncryptionKey", om.valueToTree(this.getTopicEncryptionKey()));
}
if (this.getAllowAllOutbound() != null) {
data.set("allowAllOutbound", om.valueToTree(this.getAllowAllOutbound()));
}
if (this.getAssociatePublicIpAddress() != null) {
data.set("associatePublicIpAddress", om.valueToTree(this.getAssociatePublicIpAddress()));
}
if (this.getAutoScalingGroupName() != null) {
data.set("autoScalingGroupName", om.valueToTree(this.getAutoScalingGroupName()));
}
if (this.getBlockDevices() != null) {
data.set("blockDevices", om.valueToTree(this.getBlockDevices()));
}
if (this.getCooldown() != null) {
data.set("cooldown", om.valueToTree(this.getCooldown()));
}
if (this.getDesiredCapacity() != null) {
data.set("desiredCapacity", om.valueToTree(this.getDesiredCapacity()));
}
if (this.getGroupMetrics() != null) {
data.set("groupMetrics", om.valueToTree(this.getGroupMetrics()));
}
if (this.getHealthCheck() != null) {
data.set("healthCheck", om.valueToTree(this.getHealthCheck()));
}
if (this.getIgnoreUnmodifiedSizeProperties() != null) {
data.set("ignoreUnmodifiedSizeProperties", om.valueToTree(this.getIgnoreUnmodifiedSizeProperties()));
}
if (this.getInstanceMonitoring() != null) {
data.set("instanceMonitoring", om.valueToTree(this.getInstanceMonitoring()));
}
if (this.getKeyName() != null) {
data.set("keyName", om.valueToTree(this.getKeyName()));
}
if (this.getMaxCapacity() != null) {
data.set("maxCapacity", om.valueToTree(this.getMaxCapacity()));
}
if (this.getMaxInstanceLifetime() != null) {
data.set("maxInstanceLifetime", om.valueToTree(this.getMaxInstanceLifetime()));
}
if (this.getMinCapacity() != null) {
data.set("minCapacity", om.valueToTree(this.getMinCapacity()));
}
if (this.getNewInstancesProtectedFromScaleIn() != null) {
data.set("newInstancesProtectedFromScaleIn", om.valueToTree(this.getNewInstancesProtectedFromScaleIn()));
}
if (this.getNotifications() != null) {
data.set("notifications", om.valueToTree(this.getNotifications()));
}
if (this.getNotificationsTopic() != null) {
data.set("notificationsTopic", om.valueToTree(this.getNotificationsTopic()));
}
if (this.getReplacingUpdateMinSuccessfulInstancesPercent() != null) {
data.set("replacingUpdateMinSuccessfulInstancesPercent", om.valueToTree(this.getReplacingUpdateMinSuccessfulInstancesPercent()));
}
if (this.getResourceSignalCount() != null) {
data.set("resourceSignalCount", om.valueToTree(this.getResourceSignalCount()));
}
if (this.getResourceSignalTimeout() != null) {
data.set("resourceSignalTimeout", om.valueToTree(this.getResourceSignalTimeout()));
}
if (this.getRollingUpdateConfiguration() != null) {
data.set("rollingUpdateConfiguration", om.valueToTree(this.getRollingUpdateConfiguration()));
}
if (this.getSignals() != null) {
data.set("signals", om.valueToTree(this.getSignals()));
}
if (this.getSpotPrice() != null) {
data.set("spotPrice", om.valueToTree(this.getSpotPrice()));
}
if (this.getUpdatePolicy() != null) {
data.set("updatePolicy", om.valueToTree(this.getUpdatePolicy()));
}
if (this.getUpdateType() != null) {
data.set("updateType", om.valueToTree(this.getUpdateType()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.AddCapacityOptions"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AddCapacityOptions.Jsii$Proxy that = (AddCapacityOptions.Jsii$Proxy) o;
if (!instanceType.equals(that.instanceType)) return false;
if (this.machineImage != null ? !this.machineImage.equals(that.machineImage) : that.machineImage != null) return false;
if (this.canContainersAccessInstanceRole != null ? !this.canContainersAccessInstanceRole.equals(that.canContainersAccessInstanceRole) : that.canContainersAccessInstanceRole != null) return false;
if (this.machineImageType != null ? !this.machineImageType.equals(that.machineImageType) : that.machineImageType != null) return false;
if (this.spotInstanceDraining != null ? !this.spotInstanceDraining.equals(that.spotInstanceDraining) : that.spotInstanceDraining != null) return false;
if (this.taskDrainTime != null ? !this.taskDrainTime.equals(that.taskDrainTime) : that.taskDrainTime != null) return false;
if (this.topicEncryptionKey != null ? !this.topicEncryptionKey.equals(that.topicEncryptionKey) : that.topicEncryptionKey != null) return false;
if (this.allowAllOutbound != null ? !this.allowAllOutbound.equals(that.allowAllOutbound) : that.allowAllOutbound != null) return false;
if (this.associatePublicIpAddress != null ? !this.associatePublicIpAddress.equals(that.associatePublicIpAddress) : that.associatePublicIpAddress != null) return false;
if (this.autoScalingGroupName != null ? !this.autoScalingGroupName.equals(that.autoScalingGroupName) : that.autoScalingGroupName != null) return false;
if (this.blockDevices != null ? !this.blockDevices.equals(that.blockDevices) : that.blockDevices != null) return false;
if (this.cooldown != null ? !this.cooldown.equals(that.cooldown) : that.cooldown != null) return false;
if (this.desiredCapacity != null ? !this.desiredCapacity.equals(that.desiredCapacity) : that.desiredCapacity != null) return false;
if (this.groupMetrics != null ? !this.groupMetrics.equals(that.groupMetrics) : that.groupMetrics != null) return false;
if (this.healthCheck != null ? !this.healthCheck.equals(that.healthCheck) : that.healthCheck != null) return false;
if (this.ignoreUnmodifiedSizeProperties != null ? !this.ignoreUnmodifiedSizeProperties.equals(that.ignoreUnmodifiedSizeProperties) : that.ignoreUnmodifiedSizeProperties != null) return false;
if (this.instanceMonitoring != null ? !this.instanceMonitoring.equals(that.instanceMonitoring) : that.instanceMonitoring != null) return false;
if (this.keyName != null ? !this.keyName.equals(that.keyName) : that.keyName != null) return false;
if (this.maxCapacity != null ? !this.maxCapacity.equals(that.maxCapacity) : that.maxCapacity != null) return false;
if (this.maxInstanceLifetime != null ? !this.maxInstanceLifetime.equals(that.maxInstanceLifetime) : that.maxInstanceLifetime != null) return false;
if (this.minCapacity != null ? !this.minCapacity.equals(that.minCapacity) : that.minCapacity != null) return false;
if (this.newInstancesProtectedFromScaleIn != null ? !this.newInstancesProtectedFromScaleIn.equals(that.newInstancesProtectedFromScaleIn) : that.newInstancesProtectedFromScaleIn != null) return false;
if (this.notifications != null ? !this.notifications.equals(that.notifications) : that.notifications != null) return false;
if (this.notificationsTopic != null ? !this.notificationsTopic.equals(that.notificationsTopic) : that.notificationsTopic != null) return false;
if (this.replacingUpdateMinSuccessfulInstancesPercent != null ? !this.replacingUpdateMinSuccessfulInstancesPercent.equals(that.replacingUpdateMinSuccessfulInstancesPercent) : that.replacingUpdateMinSuccessfulInstancesPercent != null) return false;
if (this.resourceSignalCount != null ? !this.resourceSignalCount.equals(that.resourceSignalCount) : that.resourceSignalCount != null) return false;
if (this.resourceSignalTimeout != null ? !this.resourceSignalTimeout.equals(that.resourceSignalTimeout) : that.resourceSignalTimeout != null) return false;
if (this.rollingUpdateConfiguration != null ? !this.rollingUpdateConfiguration.equals(that.rollingUpdateConfiguration) : that.rollingUpdateConfiguration != null) return false;
if (this.signals != null ? !this.signals.equals(that.signals) : that.signals != null) return false;
if (this.spotPrice != null ? !this.spotPrice.equals(that.spotPrice) : that.spotPrice != null) return false;
if (this.updatePolicy != null ? !this.updatePolicy.equals(that.updatePolicy) : that.updatePolicy != null) return false;
if (this.updateType != null ? !this.updateType.equals(that.updateType) : that.updateType != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public final int hashCode() {
int result = this.instanceType.hashCode();
result = 31 * result + (this.machineImage != null ? this.machineImage.hashCode() : 0);
result = 31 * result + (this.canContainersAccessInstanceRole != null ? this.canContainersAccessInstanceRole.hashCode() : 0);
result = 31 * result + (this.machineImageType != null ? this.machineImageType.hashCode() : 0);
result = 31 * result + (this.spotInstanceDraining != null ? this.spotInstanceDraining.hashCode() : 0);
result = 31 * result + (this.taskDrainTime != null ? this.taskDrainTime.hashCode() : 0);
result = 31 * result + (this.topicEncryptionKey != null ? this.topicEncryptionKey.hashCode() : 0);
result = 31 * result + (this.allowAllOutbound != null ? this.allowAllOutbound.hashCode() : 0);
result = 31 * result + (this.associatePublicIpAddress != null ? this.associatePublicIpAddress.hashCode() : 0);
result = 31 * result + (this.autoScalingGroupName != null ? this.autoScalingGroupName.hashCode() : 0);
result = 31 * result + (this.blockDevices != null ? this.blockDevices.hashCode() : 0);
result = 31 * result + (this.cooldown != null ? this.cooldown.hashCode() : 0);
result = 31 * result + (this.desiredCapacity != null ? this.desiredCapacity.hashCode() : 0);
result = 31 * result + (this.groupMetrics != null ? this.groupMetrics.hashCode() : 0);
result = 31 * result + (this.healthCheck != null ? this.healthCheck.hashCode() : 0);
result = 31 * result + (this.ignoreUnmodifiedSizeProperties != null ? this.ignoreUnmodifiedSizeProperties.hashCode() : 0);
result = 31 * result + (this.instanceMonitoring != null ? this.instanceMonitoring.hashCode() : 0);
result = 31 * result + (this.keyName != null ? this.keyName.hashCode() : 0);
result = 31 * result + (this.maxCapacity != null ? this.maxCapacity.hashCode() : 0);
result = 31 * result + (this.maxInstanceLifetime != null ? this.maxInstanceLifetime.hashCode() : 0);
result = 31 * result + (this.minCapacity != null ? this.minCapacity.hashCode() : 0);
result = 31 * result + (this.newInstancesProtectedFromScaleIn != null ? this.newInstancesProtectedFromScaleIn.hashCode() : 0);
result = 31 * result + (this.notifications != null ? this.notifications.hashCode() : 0);
result = 31 * result + (this.notificationsTopic != null ? this.notificationsTopic.hashCode() : 0);
result = 31 * result + (this.replacingUpdateMinSuccessfulInstancesPercent != null ? this.replacingUpdateMinSuccessfulInstancesPercent.hashCode() : 0);
result = 31 * result + (this.resourceSignalCount != null ? this.resourceSignalCount.hashCode() : 0);
result = 31 * result + (this.resourceSignalTimeout != null ? this.resourceSignalTimeout.hashCode() : 0);
result = 31 * result + (this.rollingUpdateConfiguration != null ? this.rollingUpdateConfiguration.hashCode() : 0);
result = 31 * result + (this.signals != null ? this.signals.hashCode() : 0);
result = 31 * result + (this.spotPrice != null ? this.spotPrice.hashCode() : 0);
result = 31 * result + (this.updatePolicy != null ? this.updatePolicy.hashCode() : 0);
result = 31 * result + (this.updateType != null ? this.updateType.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}