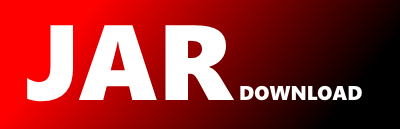
software.amazon.awscdk.services.ecs.AssetImageProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.ecs;
/**
* The properties for building an AssetImage.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.31.0 (build 6fa403d)", date = "2021-07-21T15:56:51.154Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.AssetImageProps")
@software.amazon.jsii.Jsii.Proxy(AssetImageProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AssetImageProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecr.assets.DockerImageAssetOptions {
/**
* @return a {@link Builder} of {@link AssetImageProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AssetImageProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.util.Map buildArgs;
private java.lang.String file;
private java.lang.String repositoryName;
private java.lang.String target;
private java.lang.String extraHash;
private java.util.List exclude;
private software.amazon.awscdk.assets.FollowMode follow;
private software.amazon.awscdk.core.IgnoreMode ignoreMode;
private software.amazon.awscdk.core.SymlinkFollowMode followSymlinks;
/**
* Sets the value of {@link AssetImageProps#getBuildArgs}
* @param buildArgs Build args to pass to the `docker build` command.
* Since Docker build arguments are resolved before deployment, keys and
* values cannot refer to unresolved tokens (such as lambda.functionArn
or
* queue.queueUrl
).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder buildArgs(java.util.Map buildArgs) {
this.buildArgs = buildArgs;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getFile}
* @param file Path to the Dockerfile (relative to the directory).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder file(java.lang.String file) {
this.file = file;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getRepositoryName}
* @param repositoryName ECR repository name.
* Specify this property if you need to statically address the image, e.g.
* from a Kubernetes Pod. Note, this is only the repository name, without the
* registry and the tag parts.
* @return {@code this}
* @deprecated to control the location of docker image assets, please override
`Stack.addDockerImageAsset`. this feature will be removed in future
releases.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder repositoryName(java.lang.String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getTarget}
* @param target Docker target to build to.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder target(java.lang.String target) {
this.target = target;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getExtraHash}
* @param extraHash Extra information to encode into the fingerprint (e.g. build instructions and other inputs).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder extraHash(java.lang.String extraHash) {
this.extraHash = extraHash;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getExclude}
* @param exclude Glob patterns to exclude from the copy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder exclude(java.util.List exclude) {
this.exclude = exclude;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getFollow}
* @param follow A strategy for how to handle symlinks.
* @return {@code this}
* @deprecated use `followSymlinks` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder follow(software.amazon.awscdk.assets.FollowMode follow) {
this.follow = follow;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getIgnoreMode}
* @param ignoreMode The ignore behavior to use for exclude patterns.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ignoreMode(software.amazon.awscdk.core.IgnoreMode ignoreMode) {
this.ignoreMode = ignoreMode;
return this;
}
/**
* Sets the value of {@link AssetImageProps#getFollowSymlinks}
* @param followSymlinks A strategy for how to handle symlinks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder followSymlinks(software.amazon.awscdk.core.SymlinkFollowMode followSymlinks) {
this.followSymlinks = followSymlinks;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AssetImageProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AssetImageProps build() {
return new Jsii$Proxy(buildArgs, file, repositoryName, target, extraHash, exclude, follow, ignoreMode, followSymlinks);
}
}
/**
* An implementation for {@link AssetImageProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AssetImageProps {
private final java.util.Map buildArgs;
private final java.lang.String file;
private final java.lang.String repositoryName;
private final java.lang.String target;
private final java.lang.String extraHash;
private final java.util.List exclude;
private final software.amazon.awscdk.assets.FollowMode follow;
private final software.amazon.awscdk.core.IgnoreMode ignoreMode;
private final software.amazon.awscdk.core.SymlinkFollowMode followSymlinks;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.buildArgs = software.amazon.jsii.Kernel.get(this, "buildArgs", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.file = software.amazon.jsii.Kernel.get(this, "file", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.repositoryName = software.amazon.jsii.Kernel.get(this, "repositoryName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.target = software.amazon.jsii.Kernel.get(this, "target", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.extraHash = software.amazon.jsii.Kernel.get(this, "extraHash", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.exclude = software.amazon.jsii.Kernel.get(this, "exclude", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.follow = software.amazon.jsii.Kernel.get(this, "follow", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.assets.FollowMode.class));
this.ignoreMode = software.amazon.jsii.Kernel.get(this, "ignoreMode", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.IgnoreMode.class));
this.followSymlinks = software.amazon.jsii.Kernel.get(this, "followSymlinks", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.SymlinkFollowMode.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.util.Map buildArgs, final java.lang.String file, final java.lang.String repositoryName, final java.lang.String target, final java.lang.String extraHash, final java.util.List exclude, final software.amazon.awscdk.assets.FollowMode follow, final software.amazon.awscdk.core.IgnoreMode ignoreMode, final software.amazon.awscdk.core.SymlinkFollowMode followSymlinks) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.buildArgs = buildArgs;
this.file = file;
this.repositoryName = repositoryName;
this.target = target;
this.extraHash = extraHash;
this.exclude = exclude;
this.follow = follow;
this.ignoreMode = ignoreMode;
this.followSymlinks = followSymlinks;
}
@Override
public final java.util.Map getBuildArgs() {
return this.buildArgs;
}
@Override
public final java.lang.String getFile() {
return this.file;
}
@Override
public final java.lang.String getRepositoryName() {
return this.repositoryName;
}
@Override
public final java.lang.String getTarget() {
return this.target;
}
@Override
public final java.lang.String getExtraHash() {
return this.extraHash;
}
@Override
public final java.util.List getExclude() {
return this.exclude;
}
@Override
public final software.amazon.awscdk.assets.FollowMode getFollow() {
return this.follow;
}
@Override
public final software.amazon.awscdk.core.IgnoreMode getIgnoreMode() {
return this.ignoreMode;
}
@Override
public final software.amazon.awscdk.core.SymlinkFollowMode getFollowSymlinks() {
return this.followSymlinks;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getBuildArgs() != null) {
data.set("buildArgs", om.valueToTree(this.getBuildArgs()));
}
if (this.getFile() != null) {
data.set("file", om.valueToTree(this.getFile()));
}
if (this.getRepositoryName() != null) {
data.set("repositoryName", om.valueToTree(this.getRepositoryName()));
}
if (this.getTarget() != null) {
data.set("target", om.valueToTree(this.getTarget()));
}
if (this.getExtraHash() != null) {
data.set("extraHash", om.valueToTree(this.getExtraHash()));
}
if (this.getExclude() != null) {
data.set("exclude", om.valueToTree(this.getExclude()));
}
if (this.getFollow() != null) {
data.set("follow", om.valueToTree(this.getFollow()));
}
if (this.getIgnoreMode() != null) {
data.set("ignoreMode", om.valueToTree(this.getIgnoreMode()));
}
if (this.getFollowSymlinks() != null) {
data.set("followSymlinks", om.valueToTree(this.getFollowSymlinks()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.AssetImageProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AssetImageProps.Jsii$Proxy that = (AssetImageProps.Jsii$Proxy) o;
if (this.buildArgs != null ? !this.buildArgs.equals(that.buildArgs) : that.buildArgs != null) return false;
if (this.file != null ? !this.file.equals(that.file) : that.file != null) return false;
if (this.repositoryName != null ? !this.repositoryName.equals(that.repositoryName) : that.repositoryName != null) return false;
if (this.target != null ? !this.target.equals(that.target) : that.target != null) return false;
if (this.extraHash != null ? !this.extraHash.equals(that.extraHash) : that.extraHash != null) return false;
if (this.exclude != null ? !this.exclude.equals(that.exclude) : that.exclude != null) return false;
if (this.follow != null ? !this.follow.equals(that.follow) : that.follow != null) return false;
if (this.ignoreMode != null ? !this.ignoreMode.equals(that.ignoreMode) : that.ignoreMode != null) return false;
return this.followSymlinks != null ? this.followSymlinks.equals(that.followSymlinks) : that.followSymlinks == null;
}
@Override
public final int hashCode() {
int result = this.buildArgs != null ? this.buildArgs.hashCode() : 0;
result = 31 * result + (this.file != null ? this.file.hashCode() : 0);
result = 31 * result + (this.repositoryName != null ? this.repositoryName.hashCode() : 0);
result = 31 * result + (this.target != null ? this.target.hashCode() : 0);
result = 31 * result + (this.extraHash != null ? this.extraHash.hashCode() : 0);
result = 31 * result + (this.exclude != null ? this.exclude.hashCode() : 0);
result = 31 * result + (this.follow != null ? this.follow.hashCode() : 0);
result = 31 * result + (this.ignoreMode != null ? this.ignoreMode.hashCode() : 0);
result = 31 * result + (this.followSymlinks != null ? this.followSymlinks.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy