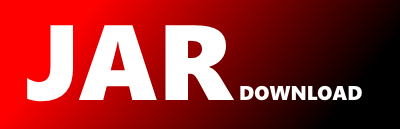
software.amazon.awscdk.services.ecs.SyslogLogDriver Maven / Gradle / Ivy
package software.amazon.awscdk.services.ecs;
/**
* A log driver that sends log information to syslog Logs.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.31.0 (build 6fa403d)", date = "2021-07-21T15:56:51.254Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.SyslogLogDriver")
public class SyslogLogDriver extends software.amazon.awscdk.services.ecs.LogDriver {
protected SyslogLogDriver(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected SyslogLogDriver(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the SyslogLogDriver class.
*
* @param props the syslog log driver configuration options.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public SyslogLogDriver(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.SyslogLogDriverProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { props });
}
/**
* Constructs a new instance of the SyslogLogDriver class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public SyslogLogDriver() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Called when the log driver is configured on a container.
*
* @param _scope This parameter is required.
* @param _containerDefinition This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.LogDriverConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.ContainerDefinition _containerDefinition) {
return software.amazon.jsii.Kernel.call(this, "bind", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.LogDriverConfig.class), new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_containerDefinition, "_containerDefinition is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.SyslogLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private software.amazon.awscdk.services.ecs.SyslogLogDriverProps.Builder props;
private Builder() {
}
/**
* The env option takes an array of keys.
*
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
*
* Default: - No env
*
* @return {@code this}
* @param env The env option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(final java.util.List env) {
this.props().env(env);
return this;
}
/**
* The env-regex option is similar to and compatible with env.
*
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
*
* Default: - No envRegex
*
* @return {@code this}
* @param envRegex The env-regex option is similar to and compatible with env. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(final java.lang.String envRegex) {
this.props().envRegex(envRegex);
return this;
}
/**
* The labels option takes an array of keys.
*
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
*
* Default: - No labels
*
* @return {@code this}
* @param labels The labels option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(final java.util.List labels) {
this.props().labels(labels);
return this;
}
/**
* By default, Docker uses the first 12 characters of the container ID to tag log messages.
*
* Refer to the log tag option documentation for customizing the
* log tag format.
*
* Default: - The first 12 characters of the container ID
*
* @return {@code this}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(final java.lang.String tag) {
this.props().tag(tag);
return this;
}
/**
* The address of an external syslog server.
*
* The URI specifier may be
* [tcp|udp|tcp+tls]://host:port, unix://path, or unixgram://path.
*
* Default: - If the transport is tcp, udp, or tcp+tls, the default port is 514.
*
* @return {@code this}
* @param address The address of an external syslog server. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder address(final java.lang.String address) {
this.props().address(address);
return this;
}
/**
* The syslog facility to use.
*
* Can be the number or name for any valid
* syslog facility. See the syslog documentation:
* https://tools.ietf.org/html/rfc5424#section-6.2.1.
*
* Default: - facility not set
*
* @return {@code this}
* @param facility The syslog facility to use. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder facility(final java.lang.String facility) {
this.props().facility(facility);
return this;
}
/**
* The syslog message format to use.
*
* If not specified the local UNIX syslog
* format is used, without a specified hostname. Specify rfc3164 for the RFC-3164
* compatible format, rfc5424 for RFC-5424 compatible format, or rfc5424micro
* for RFC-5424 compatible format with microsecond timestamp resolution.
*
* Default: - format not set
*
* @return {@code this}
* @param format The syslog message format to use. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder format(final java.lang.String format) {
this.props().format(format);
return this;
}
/**
* The absolute path to the trust certificates signed by the CA.
*
* Ignored
* if the address protocol is not tcp+tls.
*
* Default: - tlsCaCert not set
*
* @return {@code this}
* @param tlsCaCert The absolute path to the trust certificates signed by the CA. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tlsCaCert(final java.lang.String tlsCaCert) {
this.props().tlsCaCert(tlsCaCert);
return this;
}
/**
* The absolute path to the TLS certificate file.
*
* Ignored if the address
* protocol is not tcp+tls.
*
* Default: - tlsCert not set
*
* @return {@code this}
* @param tlsCert The absolute path to the TLS certificate file. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tlsCert(final java.lang.String tlsCert) {
this.props().tlsCert(tlsCert);
return this;
}
/**
* The absolute path to the TLS key file.
*
* Ignored if the address protocol
* is not tcp+tls.
*
* Default: - tlsKey not set
*
* @return {@code this}
* @param tlsKey The absolute path to the TLS key file. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tlsKey(final java.lang.String tlsKey) {
this.props().tlsKey(tlsKey);
return this;
}
/**
* If set to true, TLS verification is skipped when connecting to the syslog daemon.
*
* Ignored if the address protocol is not tcp+tls.
*
* Default: - false
*
* @return {@code this}
* @param tlsSkipVerify If set to true, TLS verification is skipped when connecting to the syslog daemon. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tlsSkipVerify(final java.lang.Boolean tlsSkipVerify) {
this.props().tlsSkipVerify(tlsSkipVerify);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.SyslogLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.SyslogLogDriver build() {
return new software.amazon.awscdk.services.ecs.SyslogLogDriver(
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.ecs.SyslogLogDriverProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.ecs.SyslogLogDriverProps.Builder();
}
return this.props;
}
}
}