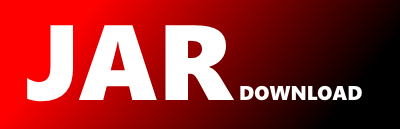
software.amazon.awscdk.services.ecs.CfnClusterCapacityProviderAssociationsProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The CDK Construct Library for AWS::ECS
package software.amazon.awscdk.services.ecs;
/**
* Properties for defining a `CfnClusterCapacityProviderAssociations`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ecs.*;
* CfnClusterCapacityProviderAssociationsProps cfnClusterCapacityProviderAssociationsProps = CfnClusterCapacityProviderAssociationsProps.builder()
* .capacityProviders(List.of("capacityProviders"))
* .cluster("cluster")
* .defaultCapacityProviderStrategy(List.of(CapacityProviderStrategyProperty.builder()
* .capacityProvider("capacityProvider")
* // the properties below are optional
* .base(123)
* .weight(123)
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-20T19:50:05.385Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnClusterCapacityProviderAssociationsProps")
@software.amazon.jsii.Jsii.Proxy(CfnClusterCapacityProviderAssociationsProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnClusterCapacityProviderAssociationsProps extends software.amazon.jsii.JsiiSerializable {
/**
* The capacity providers to associate with the cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getCapacityProviders();
/**
* The cluster the capacity provider association is the target of.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getCluster();
/**
* The default capacity provider strategy to associate with the cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getDefaultCapacityProviderStrategy();
/**
* @return a {@link Builder} of {@link CfnClusterCapacityProviderAssociationsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnClusterCapacityProviderAssociationsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List capacityProviders;
java.lang.String cluster;
java.lang.Object defaultCapacityProviderStrategy;
/**
* Sets the value of {@link CfnClusterCapacityProviderAssociationsProps#getCapacityProviders}
* @param capacityProviders The capacity providers to associate with the cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder capacityProviders(java.util.List capacityProviders) {
this.capacityProviders = capacityProviders;
return this;
}
/**
* Sets the value of {@link CfnClusterCapacityProviderAssociationsProps#getCluster}
* @param cluster The cluster the capacity provider association is the target of. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cluster(java.lang.String cluster) {
this.cluster = cluster;
return this;
}
/**
* Sets the value of {@link CfnClusterCapacityProviderAssociationsProps#getDefaultCapacityProviderStrategy}
* @param defaultCapacityProviderStrategy The default capacity provider strategy to associate with the cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultCapacityProviderStrategy(software.amazon.awscdk.core.IResolvable defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = defaultCapacityProviderStrategy;
return this;
}
/**
* Sets the value of {@link CfnClusterCapacityProviderAssociationsProps#getDefaultCapacityProviderStrategy}
* @param defaultCapacityProviderStrategy The default capacity provider strategy to associate with the cluster. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultCapacityProviderStrategy(java.util.List extends java.lang.Object> defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = defaultCapacityProviderStrategy;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnClusterCapacityProviderAssociationsProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnClusterCapacityProviderAssociationsProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnClusterCapacityProviderAssociationsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnClusterCapacityProviderAssociationsProps {
private final java.util.List capacityProviders;
private final java.lang.String cluster;
private final java.lang.Object defaultCapacityProviderStrategy;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.capacityProviders = software.amazon.jsii.Kernel.get(this, "capacityProviders", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.cluster = software.amazon.jsii.Kernel.get(this, "cluster", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.defaultCapacityProviderStrategy = software.amazon.jsii.Kernel.get(this, "defaultCapacityProviderStrategy", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.capacityProviders = java.util.Objects.requireNonNull(builder.capacityProviders, "capacityProviders is required");
this.cluster = java.util.Objects.requireNonNull(builder.cluster, "cluster is required");
this.defaultCapacityProviderStrategy = java.util.Objects.requireNonNull(builder.defaultCapacityProviderStrategy, "defaultCapacityProviderStrategy is required");
}
@Override
public final java.util.List getCapacityProviders() {
return this.capacityProviders;
}
@Override
public final java.lang.String getCluster() {
return this.cluster;
}
@Override
public final java.lang.Object getDefaultCapacityProviderStrategy() {
return this.defaultCapacityProviderStrategy;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("capacityProviders", om.valueToTree(this.getCapacityProviders()));
data.set("cluster", om.valueToTree(this.getCluster()));
data.set("defaultCapacityProviderStrategy", om.valueToTree(this.getDefaultCapacityProviderStrategy()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnClusterCapacityProviderAssociationsProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnClusterCapacityProviderAssociationsProps.Jsii$Proxy that = (CfnClusterCapacityProviderAssociationsProps.Jsii$Proxy) o;
if (!capacityProviders.equals(that.capacityProviders)) return false;
if (!cluster.equals(that.cluster)) return false;
return this.defaultCapacityProviderStrategy.equals(that.defaultCapacityProviderStrategy);
}
@Override
public final int hashCode() {
int result = this.capacityProviders.hashCode();
result = 31 * result + (this.cluster.hashCode());
result = 31 * result + (this.defaultCapacityProviderStrategy.hashCode());
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy