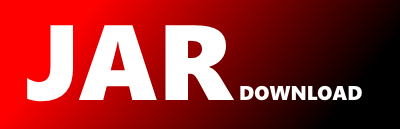
software.amazon.awscdk.services.ecs.JsonFileLogDriver Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A log driver that sends log information to json-file Logs.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ecs.*;
* JsonFileLogDriver jsonFileLogDriver = JsonFileLogDriver.Builder.create()
* .compress(false)
* .env(List.of("env"))
* .envRegex("envRegex")
* .labels(List.of("labels"))
* .maxFile(123)
* .maxSize("maxSize")
* .tag("tag")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-20T19:50:05.644Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.JsonFileLogDriver")
public class JsonFileLogDriver extends software.amazon.awscdk.services.ecs.LogDriver {
protected JsonFileLogDriver(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected JsonFileLogDriver(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the JsonFileLogDriver class.
*
* @param props the json-file log driver configuration options.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public JsonFileLogDriver(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.JsonFileLogDriverProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { props });
}
/**
* Constructs a new instance of the JsonFileLogDriver class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public JsonFileLogDriver() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Called when the log driver is configured on a container.
*
* @param _scope This parameter is required.
* @param _containerDefinition This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.LogDriverConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.ContainerDefinition _containerDefinition) {
return software.amazon.jsii.Kernel.call(this, "bind", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.LogDriverConfig.class), new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_containerDefinition, "_containerDefinition is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.JsonFileLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private software.amazon.awscdk.services.ecs.JsonFileLogDriverProps.Builder props;
private Builder() {
}
/**
* The env option takes an array of keys.
*
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
*
* Default: - No env
*
* @return {@code this}
* @param env The env option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(final java.util.List env) {
this.props().env(env);
return this;
}
/**
* The env-regex option is similar to and compatible with env.
*
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
*
* Default: - No envRegex
*
* @return {@code this}
* @param envRegex The env-regex option is similar to and compatible with env. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(final java.lang.String envRegex) {
this.props().envRegex(envRegex);
return this;
}
/**
* The labels option takes an array of keys.
*
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
*
* Default: - No labels
*
* @return {@code this}
* @param labels The labels option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(final java.util.List labels) {
this.props().labels(labels);
return this;
}
/**
* By default, Docker uses the first 12 characters of the container ID to tag log messages.
*
* Refer to the log tag option documentation for customizing the
* log tag format.
*
* Default: - The first 12 characters of the container ID
*
* @return {@code this}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(final java.lang.String tag) {
this.props().tag(tag);
return this;
}
/**
* Toggles compression for rotated logs.
*
* Default: - false
*
* @return {@code this}
* @param compress Toggles compression for rotated logs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compress(final java.lang.Boolean compress) {
this.props().compress(compress);
return this;
}
/**
* The maximum number of log files that can be present.
*
* If rolling the logs creates
* excess files, the oldest file is removed. Only effective when max-size is also set.
* A positive integer.
*
* Default: - 1
*
* @return {@code this}
* @param maxFile The maximum number of log files that can be present. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxFile(final java.lang.Number maxFile) {
this.props().maxFile(maxFile);
return this;
}
/**
* The maximum size of the log before it is rolled.
*
* A positive integer plus a modifier
* representing the unit of measure (k, m, or g).
*
* Default: - -1 (unlimited)
*
* @return {@code this}
* @param maxSize The maximum size of the log before it is rolled. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxSize(final java.lang.String maxSize) {
this.props().maxSize(maxSize);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.JsonFileLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.JsonFileLogDriver build() {
return new software.amazon.awscdk.services.ecs.JsonFileLogDriver(
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.ecs.JsonFileLogDriverProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.ecs.JsonFileLogDriverProps.Builder();
}
return this.props;
}
}
}