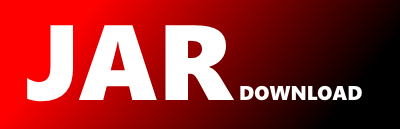
software.amazon.awscdk.services.ecs.Ec2TaskDefinitionProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The CDK Construct Library for AWS::ECS
package software.amazon.awscdk.services.ecs;
/**
* The properties for a task definition run on an EC2 cluster.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:18:01.435Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.Ec2TaskDefinitionProps")
@software.amazon.jsii.Jsii.Proxy(Ec2TaskDefinitionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface Ec2TaskDefinitionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.CommonTaskDefinitionProps {
/**
* The Docker networking mode to use for the containers in the task.
*
* The valid values are none, bridge, awsvpc, and host.
*
* Default: - NetworkMode.Bridge for EC2 tasks, AwsVpc for Fargate tasks.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default software.amazon.awscdk.services.ecs.NetworkMode getNetworkMode() {
return null;
}
/**
* An array of placement constraint objects to use for the task.
*
* You can
* specify a maximum of 10 constraints per task (this limit includes
* constraints in the task definition and those specified at run time).
*
* Default: - No placement constraints.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.util.List getPlacementConstraints() {
return null;
}
/**
* @return a {@link Builder} of {@link Ec2TaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link Ec2TaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private software.amazon.awscdk.services.ecs.NetworkMode networkMode;
private java.util.List placementConstraints;
private software.amazon.awscdk.services.iam.IRole executionRole;
private java.lang.String family;
private software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
private software.amazon.awscdk.services.iam.IRole taskRole;
private java.util.List volumes;
/**
* Sets the value of NetworkMode
* @param networkMode The Docker networking mode to use for the containers in the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkMode(software.amazon.awscdk.services.ecs.NetworkMode networkMode) {
this.networkMode = networkMode;
return this;
}
/**
* Sets the value of PlacementConstraints
* @param placementConstraints An array of placement constraint objects to use for the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementConstraints(java.util.List placementConstraints) {
this.placementConstraints = placementConstraints;
return this;
}
/**
* Sets the value of ExecutionRole
* @param executionRole The name of the IAM task execution role that grants the ECS agent to call AWS APIs on your behalf.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executionRole(software.amazon.awscdk.services.iam.IRole executionRole) {
this.executionRole = executionRole;
return this;
}
/**
* Sets the value of Family
* @param family The name of a family that this task definition is registered to.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder family(java.lang.String family) {
this.family = family;
return this;
}
/**
* Sets the value of ProxyConfiguration
* @param proxyConfiguration The configuration details for the App Mesh proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder proxyConfiguration(software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
return this;
}
/**
* Sets the value of TaskRole
* @param taskRole The name of the IAM role that grants containers in the task permission to call AWS APIs on your behalf.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskRole(software.amazon.awscdk.services.iam.IRole taskRole) {
this.taskRole = taskRole;
return this;
}
/**
* Sets the value of Volumes
* @param volumes The list of volume definitions for the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder volumes(java.util.List volumes) {
this.volumes = volumes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link Ec2TaskDefinitionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Ec2TaskDefinitionProps build() {
return new Jsii$Proxy(networkMode, placementConstraints, executionRole, family, proxyConfiguration, taskRole, volumes);
}
}
/**
* An implementation for {@link Ec2TaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements Ec2TaskDefinitionProps {
private final software.amazon.awscdk.services.ecs.NetworkMode networkMode;
private final java.util.List placementConstraints;
private final software.amazon.awscdk.services.iam.IRole executionRole;
private final java.lang.String family;
private final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
private final software.amazon.awscdk.services.iam.IRole taskRole;
private final java.util.List volumes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.networkMode = this.jsiiGet("networkMode", software.amazon.awscdk.services.ecs.NetworkMode.class);
this.placementConstraints = this.jsiiGet("placementConstraints", java.util.List.class);
this.executionRole = this.jsiiGet("executionRole", software.amazon.awscdk.services.iam.IRole.class);
this.family = this.jsiiGet("family", java.lang.String.class);
this.proxyConfiguration = this.jsiiGet("proxyConfiguration", software.amazon.awscdk.services.ecs.ProxyConfiguration.class);
this.taskRole = this.jsiiGet("taskRole", software.amazon.awscdk.services.iam.IRole.class);
this.volumes = this.jsiiGet("volumes", java.util.List.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final software.amazon.awscdk.services.ecs.NetworkMode networkMode, final java.util.List placementConstraints, final software.amazon.awscdk.services.iam.IRole executionRole, final java.lang.String family, final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration, final software.amazon.awscdk.services.iam.IRole taskRole, final java.util.List volumes) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.networkMode = networkMode;
this.placementConstraints = placementConstraints;
this.executionRole = executionRole;
this.family = family;
this.proxyConfiguration = proxyConfiguration;
this.taskRole = taskRole;
this.volumes = volumes;
}
@Override
public software.amazon.awscdk.services.ecs.NetworkMode getNetworkMode() {
return this.networkMode;
}
@Override
public java.util.List getPlacementConstraints() {
return this.placementConstraints;
}
@Override
public software.amazon.awscdk.services.iam.IRole getExecutionRole() {
return this.executionRole;
}
@Override
public java.lang.String getFamily() {
return this.family;
}
@Override
public software.amazon.awscdk.services.ecs.ProxyConfiguration getProxyConfiguration() {
return this.proxyConfiguration;
}
@Override
public software.amazon.awscdk.services.iam.IRole getTaskRole() {
return this.taskRole;
}
@Override
public java.util.List getVolumes() {
return this.volumes;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getNetworkMode() != null) {
data.set("networkMode", om.valueToTree(this.getNetworkMode()));
}
if (this.getPlacementConstraints() != null) {
data.set("placementConstraints", om.valueToTree(this.getPlacementConstraints()));
}
if (this.getExecutionRole() != null) {
data.set("executionRole", om.valueToTree(this.getExecutionRole()));
}
if (this.getFamily() != null) {
data.set("family", om.valueToTree(this.getFamily()));
}
if (this.getProxyConfiguration() != null) {
data.set("proxyConfiguration", om.valueToTree(this.getProxyConfiguration()));
}
if (this.getTaskRole() != null) {
data.set("taskRole", om.valueToTree(this.getTaskRole()));
}
if (this.getVolumes() != null) {
data.set("volumes", om.valueToTree(this.getVolumes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.Ec2TaskDefinitionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Ec2TaskDefinitionProps.Jsii$Proxy that = (Ec2TaskDefinitionProps.Jsii$Proxy) o;
if (this.networkMode != null ? !this.networkMode.equals(that.networkMode) : that.networkMode != null) return false;
if (this.placementConstraints != null ? !this.placementConstraints.equals(that.placementConstraints) : that.placementConstraints != null) return false;
if (this.executionRole != null ? !this.executionRole.equals(that.executionRole) : that.executionRole != null) return false;
if (this.family != null ? !this.family.equals(that.family) : that.family != null) return false;
if (this.proxyConfiguration != null ? !this.proxyConfiguration.equals(that.proxyConfiguration) : that.proxyConfiguration != null) return false;
if (this.taskRole != null ? !this.taskRole.equals(that.taskRole) : that.taskRole != null) return false;
return this.volumes != null ? this.volumes.equals(that.volumes) : that.volumes == null;
}
@Override
public int hashCode() {
int result = this.networkMode != null ? this.networkMode.hashCode() : 0;
result = 31 * result + (this.placementConstraints != null ? this.placementConstraints.hashCode() : 0);
result = 31 * result + (this.executionRole != null ? this.executionRole.hashCode() : 0);
result = 31 * result + (this.family != null ? this.family.hashCode() : 0);
result = 31 * result + (this.proxyConfiguration != null ? this.proxyConfiguration.hashCode() : 0);
result = 31 * result + (this.taskRole != null ? this.taskRole.hashCode() : 0);
result = 31 * result + (this.volumes != null ? this.volumes.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy