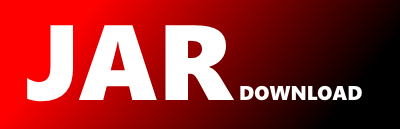
software.amazon.awscdk.services.ecs.FargateServiceProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.ecs;
/**
* The properties for defining a service using the Fargate launch type.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:18:01.436Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.FargateServiceProps")
@software.amazon.jsii.Jsii.Proxy(FargateServiceProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface FargateServiceProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.BaseServiceOptions {
/**
* The task definition to use for tasks in the service.
*
* [disable-awslint:ref-via-interface]
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
software.amazon.awscdk.services.ecs.TaskDefinition getTaskDefinition();
/**
* Specifies whether the task's elastic network interface receives a public IP address.
*
* If true, each task will receive a public IP address.
*
* Default: - Use subnet default.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Boolean getAssignPublicIp() {
return null;
}
/**
* The platform version on which to run your service.
*
* If one is not specified, the LATEST platform version is used by default. For more information, see
* [AWS Fargate Platform Versions](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/platform_versions.html)
* in the Amazon Elastic Container Service Developer Guide.
*
* Default: Latest
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default software.amazon.awscdk.services.ecs.FargatePlatformVersion getPlatformVersion() {
return null;
}
/**
* Specifies whether to propagate the tags from the task definition or the service to the tasks in the service. Tags can only be propagated to the tasks within the service during service creation.
*
* Default: PropagatedTagSource.NONE
*
* @deprecated Use `propagateTags` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
default software.amazon.awscdk.services.ecs.PropagatedTagSource getPropagateTaskTagsFrom() {
return null;
}
/**
* The security groups to associate with the service.
*
* If you do not specify a security group, the default security group for the VPC is used.
*
* Default: - A new security group is created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return null;
}
/**
* The subnets to associate with the service.
*
* Default: - Private subnets.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return null;
}
/**
* @return a {@link Builder} of {@link FargateServiceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FargateServiceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private software.amazon.awscdk.services.ecs.TaskDefinition taskDefinition;
private java.lang.Boolean assignPublicIp;
private software.amazon.awscdk.services.ecs.FargatePlatformVersion platformVersion;
private software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTaskTagsFrom;
private software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
private software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
private software.amazon.awscdk.services.ecs.ICluster cluster;
private software.amazon.awscdk.services.ecs.CloudMapOptions cloudMapOptions;
private java.lang.Number desiredCount;
private java.lang.Boolean enableEcsManagedTags;
private software.amazon.awscdk.core.Duration healthCheckGracePeriod;
private java.lang.Number maxHealthyPercent;
private java.lang.Number minHealthyPercent;
private software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTags;
private java.lang.String serviceName;
/**
* Sets the value of TaskDefinition
* @param taskDefinition The task definition to use for tasks in the service. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskDefinition(software.amazon.awscdk.services.ecs.TaskDefinition taskDefinition) {
this.taskDefinition = taskDefinition;
return this;
}
/**
* Sets the value of AssignPublicIp
* @param assignPublicIp Specifies whether the task's elastic network interface receives a public IP address.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assignPublicIp(java.lang.Boolean assignPublicIp) {
this.assignPublicIp = assignPublicIp;
return this;
}
/**
* Sets the value of PlatformVersion
* @param platformVersion The platform version on which to run your service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder platformVersion(software.amazon.awscdk.services.ecs.FargatePlatformVersion platformVersion) {
this.platformVersion = platformVersion;
return this;
}
/**
* Sets the value of PropagateTaskTagsFrom
* @param propagateTaskTagsFrom Specifies whether to propagate the tags from the task definition or the service to the tasks in the service. Tags can only be propagated to the tasks within the service during service creation.
* @return {@code this}
* @deprecated Use `propagateTags` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder propagateTaskTagsFrom(software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTaskTagsFrom) {
this.propagateTaskTagsFrom = propagateTaskTagsFrom;
return this;
}
/**
* Sets the value of SecurityGroup
* @param securityGroup The security groups to associate with the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityGroup(software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup) {
this.securityGroup = securityGroup;
return this;
}
/**
* Sets the value of VpcSubnets
* @param vpcSubnets The subnets to associate with the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Sets the value of Cluster
* @param cluster The name of the cluster that hosts the service. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cluster(software.amazon.awscdk.services.ecs.ICluster cluster) {
this.cluster = cluster;
return this;
}
/**
* Sets the value of CloudMapOptions
* @param cloudMapOptions The options for configuring an Amazon ECS service to use service discovery.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudMapOptions(software.amazon.awscdk.services.ecs.CloudMapOptions cloudMapOptions) {
this.cloudMapOptions = cloudMapOptions;
return this;
}
/**
* Sets the value of DesiredCount
* @param desiredCount The desired number of instantiations of the task definition to keep running on the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder desiredCount(java.lang.Number desiredCount) {
this.desiredCount = desiredCount;
return this;
}
/**
* Sets the value of EnableEcsManagedTags
* @param enableEcsManagedTags Specifies whether to enable Amazon ECS managed tags for the tasks within the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableEcsManagedTags(java.lang.Boolean enableEcsManagedTags) {
this.enableEcsManagedTags = enableEcsManagedTags;
return this;
}
/**
* Sets the value of HealthCheckGracePeriod
* @param healthCheckGracePeriod The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing target health checks after a task has first started.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckGracePeriod(software.amazon.awscdk.core.Duration healthCheckGracePeriod) {
this.healthCheckGracePeriod = healthCheckGracePeriod;
return this;
}
/**
* Sets the value of MaxHealthyPercent
* @param maxHealthyPercent The maximum number of tasks, specified as a percentage of the Amazon ECS service's DesiredCount value, that can run in a service during a deployment.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxHealthyPercent(java.lang.Number maxHealthyPercent) {
this.maxHealthyPercent = maxHealthyPercent;
return this;
}
/**
* Sets the value of MinHealthyPercent
* @param minHealthyPercent The minimum number of tasks, specified as a percentage of the Amazon ECS service's DesiredCount value, that must continue to run and remain healthy during a deployment.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder minHealthyPercent(java.lang.Number minHealthyPercent) {
this.minHealthyPercent = minHealthyPercent;
return this;
}
/**
* Sets the value of PropagateTags
* @param propagateTags Specifies whether to propagate the tags from the task definition or the service to the tasks in the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder propagateTags(software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTags) {
this.propagateTags = propagateTags;
return this;
}
/**
* Sets the value of ServiceName
* @param serviceName The name of the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceName(java.lang.String serviceName) {
this.serviceName = serviceName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FargateServiceProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public FargateServiceProps build() {
return new Jsii$Proxy(taskDefinition, assignPublicIp, platformVersion, propagateTaskTagsFrom, securityGroup, vpcSubnets, cluster, cloudMapOptions, desiredCount, enableEcsManagedTags, healthCheckGracePeriod, maxHealthyPercent, minHealthyPercent, propagateTags, serviceName);
}
}
/**
* An implementation for {@link FargateServiceProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FargateServiceProps {
private final software.amazon.awscdk.services.ecs.TaskDefinition taskDefinition;
private final java.lang.Boolean assignPublicIp;
private final software.amazon.awscdk.services.ecs.FargatePlatformVersion platformVersion;
private final software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTaskTagsFrom;
private final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
private final software.amazon.awscdk.services.ecs.ICluster cluster;
private final software.amazon.awscdk.services.ecs.CloudMapOptions cloudMapOptions;
private final java.lang.Number desiredCount;
private final java.lang.Boolean enableEcsManagedTags;
private final software.amazon.awscdk.core.Duration healthCheckGracePeriod;
private final java.lang.Number maxHealthyPercent;
private final java.lang.Number minHealthyPercent;
private final software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTags;
private final java.lang.String serviceName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.taskDefinition = this.jsiiGet("taskDefinition", software.amazon.awscdk.services.ecs.TaskDefinition.class);
this.assignPublicIp = this.jsiiGet("assignPublicIp", java.lang.Boolean.class);
this.platformVersion = this.jsiiGet("platformVersion", software.amazon.awscdk.services.ecs.FargatePlatformVersion.class);
this.propagateTaskTagsFrom = this.jsiiGet("propagateTaskTagsFrom", software.amazon.awscdk.services.ecs.PropagatedTagSource.class);
this.securityGroup = this.jsiiGet("securityGroup", software.amazon.awscdk.services.ec2.ISecurityGroup.class);
this.vpcSubnets = this.jsiiGet("vpcSubnets", software.amazon.awscdk.services.ec2.SubnetSelection.class);
this.cluster = this.jsiiGet("cluster", software.amazon.awscdk.services.ecs.ICluster.class);
this.cloudMapOptions = this.jsiiGet("cloudMapOptions", software.amazon.awscdk.services.ecs.CloudMapOptions.class);
this.desiredCount = this.jsiiGet("desiredCount", java.lang.Number.class);
this.enableEcsManagedTags = this.jsiiGet("enableECSManagedTags", java.lang.Boolean.class);
this.healthCheckGracePeriod = this.jsiiGet("healthCheckGracePeriod", software.amazon.awscdk.core.Duration.class);
this.maxHealthyPercent = this.jsiiGet("maxHealthyPercent", java.lang.Number.class);
this.minHealthyPercent = this.jsiiGet("minHealthyPercent", java.lang.Number.class);
this.propagateTags = this.jsiiGet("propagateTags", software.amazon.awscdk.services.ecs.PropagatedTagSource.class);
this.serviceName = this.jsiiGet("serviceName", java.lang.String.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final software.amazon.awscdk.services.ecs.TaskDefinition taskDefinition, final java.lang.Boolean assignPublicIp, final software.amazon.awscdk.services.ecs.FargatePlatformVersion platformVersion, final software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTaskTagsFrom, final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup, final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets, final software.amazon.awscdk.services.ecs.ICluster cluster, final software.amazon.awscdk.services.ecs.CloudMapOptions cloudMapOptions, final java.lang.Number desiredCount, final java.lang.Boolean enableEcsManagedTags, final software.amazon.awscdk.core.Duration healthCheckGracePeriod, final java.lang.Number maxHealthyPercent, final java.lang.Number minHealthyPercent, final software.amazon.awscdk.services.ecs.PropagatedTagSource propagateTags, final java.lang.String serviceName) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.taskDefinition = java.util.Objects.requireNonNull(taskDefinition, "taskDefinition is required");
this.assignPublicIp = assignPublicIp;
this.platformVersion = platformVersion;
this.propagateTaskTagsFrom = propagateTaskTagsFrom;
this.securityGroup = securityGroup;
this.vpcSubnets = vpcSubnets;
this.cluster = java.util.Objects.requireNonNull(cluster, "cluster is required");
this.cloudMapOptions = cloudMapOptions;
this.desiredCount = desiredCount;
this.enableEcsManagedTags = enableEcsManagedTags;
this.healthCheckGracePeriod = healthCheckGracePeriod;
this.maxHealthyPercent = maxHealthyPercent;
this.minHealthyPercent = minHealthyPercent;
this.propagateTags = propagateTags;
this.serviceName = serviceName;
}
@Override
public software.amazon.awscdk.services.ecs.TaskDefinition getTaskDefinition() {
return this.taskDefinition;
}
@Override
public java.lang.Boolean getAssignPublicIp() {
return this.assignPublicIp;
}
@Override
public software.amazon.awscdk.services.ecs.FargatePlatformVersion getPlatformVersion() {
return this.platformVersion;
}
@Override
public software.amazon.awscdk.services.ecs.PropagatedTagSource getPropagateTaskTagsFrom() {
return this.propagateTaskTagsFrom;
}
@Override
public software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return this.securityGroup;
}
@Override
public software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
public software.amazon.awscdk.services.ecs.ICluster getCluster() {
return this.cluster;
}
@Override
public software.amazon.awscdk.services.ecs.CloudMapOptions getCloudMapOptions() {
return this.cloudMapOptions;
}
@Override
public java.lang.Number getDesiredCount() {
return this.desiredCount;
}
@Override
public java.lang.Boolean getEnableEcsManagedTags() {
return this.enableEcsManagedTags;
}
@Override
public software.amazon.awscdk.core.Duration getHealthCheckGracePeriod() {
return this.healthCheckGracePeriod;
}
@Override
public java.lang.Number getMaxHealthyPercent() {
return this.maxHealthyPercent;
}
@Override
public java.lang.Number getMinHealthyPercent() {
return this.minHealthyPercent;
}
@Override
public software.amazon.awscdk.services.ecs.PropagatedTagSource getPropagateTags() {
return this.propagateTags;
}
@Override
public java.lang.String getServiceName() {
return this.serviceName;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("taskDefinition", om.valueToTree(this.getTaskDefinition()));
if (this.getAssignPublicIp() != null) {
data.set("assignPublicIp", om.valueToTree(this.getAssignPublicIp()));
}
if (this.getPlatformVersion() != null) {
data.set("platformVersion", om.valueToTree(this.getPlatformVersion()));
}
if (this.getPropagateTaskTagsFrom() != null) {
data.set("propagateTaskTagsFrom", om.valueToTree(this.getPropagateTaskTagsFrom()));
}
if (this.getSecurityGroup() != null) {
data.set("securityGroup", om.valueToTree(this.getSecurityGroup()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
data.set("cluster", om.valueToTree(this.getCluster()));
if (this.getCloudMapOptions() != null) {
data.set("cloudMapOptions", om.valueToTree(this.getCloudMapOptions()));
}
if (this.getDesiredCount() != null) {
data.set("desiredCount", om.valueToTree(this.getDesiredCount()));
}
if (this.getEnableEcsManagedTags() != null) {
data.set("enableECSManagedTags", om.valueToTree(this.getEnableEcsManagedTags()));
}
if (this.getHealthCheckGracePeriod() != null) {
data.set("healthCheckGracePeriod", om.valueToTree(this.getHealthCheckGracePeriod()));
}
if (this.getMaxHealthyPercent() != null) {
data.set("maxHealthyPercent", om.valueToTree(this.getMaxHealthyPercent()));
}
if (this.getMinHealthyPercent() != null) {
data.set("minHealthyPercent", om.valueToTree(this.getMinHealthyPercent()));
}
if (this.getPropagateTags() != null) {
data.set("propagateTags", om.valueToTree(this.getPropagateTags()));
}
if (this.getServiceName() != null) {
data.set("serviceName", om.valueToTree(this.getServiceName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.FargateServiceProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FargateServiceProps.Jsii$Proxy that = (FargateServiceProps.Jsii$Proxy) o;
if (!taskDefinition.equals(that.taskDefinition)) return false;
if (this.assignPublicIp != null ? !this.assignPublicIp.equals(that.assignPublicIp) : that.assignPublicIp != null) return false;
if (this.platformVersion != null ? !this.platformVersion.equals(that.platformVersion) : that.platformVersion != null) return false;
if (this.propagateTaskTagsFrom != null ? !this.propagateTaskTagsFrom.equals(that.propagateTaskTagsFrom) : that.propagateTaskTagsFrom != null) return false;
if (this.securityGroup != null ? !this.securityGroup.equals(that.securityGroup) : that.securityGroup != null) return false;
if (this.vpcSubnets != null ? !this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets != null) return false;
if (!cluster.equals(that.cluster)) return false;
if (this.cloudMapOptions != null ? !this.cloudMapOptions.equals(that.cloudMapOptions) : that.cloudMapOptions != null) return false;
if (this.desiredCount != null ? !this.desiredCount.equals(that.desiredCount) : that.desiredCount != null) return false;
if (this.enableEcsManagedTags != null ? !this.enableEcsManagedTags.equals(that.enableEcsManagedTags) : that.enableEcsManagedTags != null) return false;
if (this.healthCheckGracePeriod != null ? !this.healthCheckGracePeriod.equals(that.healthCheckGracePeriod) : that.healthCheckGracePeriod != null) return false;
if (this.maxHealthyPercent != null ? !this.maxHealthyPercent.equals(that.maxHealthyPercent) : that.maxHealthyPercent != null) return false;
if (this.minHealthyPercent != null ? !this.minHealthyPercent.equals(that.minHealthyPercent) : that.minHealthyPercent != null) return false;
if (this.propagateTags != null ? !this.propagateTags.equals(that.propagateTags) : that.propagateTags != null) return false;
return this.serviceName != null ? this.serviceName.equals(that.serviceName) : that.serviceName == null;
}
@Override
public int hashCode() {
int result = this.taskDefinition.hashCode();
result = 31 * result + (this.assignPublicIp != null ? this.assignPublicIp.hashCode() : 0);
result = 31 * result + (this.platformVersion != null ? this.platformVersion.hashCode() : 0);
result = 31 * result + (this.propagateTaskTagsFrom != null ? this.propagateTaskTagsFrom.hashCode() : 0);
result = 31 * result + (this.securityGroup != null ? this.securityGroup.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
result = 31 * result + (this.cluster.hashCode());
result = 31 * result + (this.cloudMapOptions != null ? this.cloudMapOptions.hashCode() : 0);
result = 31 * result + (this.desiredCount != null ? this.desiredCount.hashCode() : 0);
result = 31 * result + (this.enableEcsManagedTags != null ? this.enableEcsManagedTags.hashCode() : 0);
result = 31 * result + (this.healthCheckGracePeriod != null ? this.healthCheckGracePeriod.hashCode() : 0);
result = 31 * result + (this.maxHealthyPercent != null ? this.maxHealthyPercent.hashCode() : 0);
result = 31 * result + (this.minHealthyPercent != null ? this.minHealthyPercent.hashCode() : 0);
result = 31 * result + (this.propagateTags != null ? this.propagateTags.hashCode() : 0);
result = 31 * result + (this.serviceName != null ? this.serviceName.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy