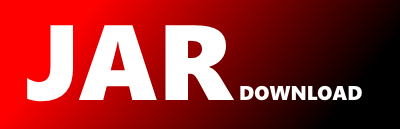
software.amazon.awscdk.services.ecs.FargateTaskDefinitionProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The CDK Construct Library for AWS::ECS
package software.amazon.awscdk.services.ecs;
/**
* The properties for a task definition.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:18:01.447Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.FargateTaskDefinitionProps")
@software.amazon.jsii.Jsii.Proxy(FargateTaskDefinitionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface FargateTaskDefinitionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.CommonTaskDefinitionProps {
/**
* The number of cpu units used by the task.
*
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values,
* which determines your range of valid values for the memory parameter:
*
* 256 (.25 vCPU) - Available memory values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
* 512 (.5 vCPU) - Available memory values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
* 1024 (1 vCPU) - Available memory values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
* 2048 (2 vCPU) - Available memory values: Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
* 4096 (4 vCPU) - Available memory values: Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
* Default: 256
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Number getCpu() {
return null;
}
/**
* The amount (in MiB) of memory used by the task.
*
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values, which determines your range of valid values for the cpu parameter:
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu values: 256 (.25 vCPU)
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu values: 512 (.5 vCPU)
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available cpu values: 1024 (1 vCPU)
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu values: 2048 (2 vCPU)
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu values: 4096 (4 vCPU)
*
* Default: 512
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Number getMemoryLimitMiB() {
return null;
}
/**
* @return a {@link Builder} of {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.Number cpu;
private java.lang.Number memoryLimitMiB;
private software.amazon.awscdk.services.iam.IRole executionRole;
private java.lang.String family;
private software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
private software.amazon.awscdk.services.iam.IRole taskRole;
private java.util.List volumes;
/**
* Sets the value of Cpu
* @param cpu The number of cpu units used by the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cpu(java.lang.Number cpu) {
this.cpu = cpu;
return this;
}
/**
* Sets the value of MemoryLimitMiB
* @param memoryLimitMiB The amount (in MiB) of memory used by the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memoryLimitMiB(java.lang.Number memoryLimitMiB) {
this.memoryLimitMiB = memoryLimitMiB;
return this;
}
/**
* Sets the value of ExecutionRole
* @param executionRole The name of the IAM task execution role that grants the ECS agent to call AWS APIs on your behalf.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executionRole(software.amazon.awscdk.services.iam.IRole executionRole) {
this.executionRole = executionRole;
return this;
}
/**
* Sets the value of Family
* @param family The name of a family that this task definition is registered to.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder family(java.lang.String family) {
this.family = family;
return this;
}
/**
* Sets the value of ProxyConfiguration
* @param proxyConfiguration The configuration details for the App Mesh proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder proxyConfiguration(software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
return this;
}
/**
* Sets the value of TaskRole
* @param taskRole The name of the IAM role that grants containers in the task permission to call AWS APIs on your behalf.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskRole(software.amazon.awscdk.services.iam.IRole taskRole) {
this.taskRole = taskRole;
return this;
}
/**
* Sets the value of Volumes
* @param volumes The list of volume definitions for the task.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder volumes(java.util.List volumes) {
this.volumes = volumes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FargateTaskDefinitionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public FargateTaskDefinitionProps build() {
return new Jsii$Proxy(cpu, memoryLimitMiB, executionRole, family, proxyConfiguration, taskRole, volumes);
}
}
/**
* An implementation for {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FargateTaskDefinitionProps {
private final java.lang.Number cpu;
private final java.lang.Number memoryLimitMiB;
private final software.amazon.awscdk.services.iam.IRole executionRole;
private final java.lang.String family;
private final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
private final software.amazon.awscdk.services.iam.IRole taskRole;
private final java.util.List volumes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.cpu = this.jsiiGet("cpu", java.lang.Number.class);
this.memoryLimitMiB = this.jsiiGet("memoryLimitMiB", java.lang.Number.class);
this.executionRole = this.jsiiGet("executionRole", software.amazon.awscdk.services.iam.IRole.class);
this.family = this.jsiiGet("family", java.lang.String.class);
this.proxyConfiguration = this.jsiiGet("proxyConfiguration", software.amazon.awscdk.services.ecs.ProxyConfiguration.class);
this.taskRole = this.jsiiGet("taskRole", software.amazon.awscdk.services.iam.IRole.class);
this.volumes = this.jsiiGet("volumes", java.util.List.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.Number cpu, final java.lang.Number memoryLimitMiB, final software.amazon.awscdk.services.iam.IRole executionRole, final java.lang.String family, final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration, final software.amazon.awscdk.services.iam.IRole taskRole, final java.util.List volumes) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.cpu = cpu;
this.memoryLimitMiB = memoryLimitMiB;
this.executionRole = executionRole;
this.family = family;
this.proxyConfiguration = proxyConfiguration;
this.taskRole = taskRole;
this.volumes = volumes;
}
@Override
public java.lang.Number getCpu() {
return this.cpu;
}
@Override
public java.lang.Number getMemoryLimitMiB() {
return this.memoryLimitMiB;
}
@Override
public software.amazon.awscdk.services.iam.IRole getExecutionRole() {
return this.executionRole;
}
@Override
public java.lang.String getFamily() {
return this.family;
}
@Override
public software.amazon.awscdk.services.ecs.ProxyConfiguration getProxyConfiguration() {
return this.proxyConfiguration;
}
@Override
public software.amazon.awscdk.services.iam.IRole getTaskRole() {
return this.taskRole;
}
@Override
public java.util.List getVolumes() {
return this.volumes;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCpu() != null) {
data.set("cpu", om.valueToTree(this.getCpu()));
}
if (this.getMemoryLimitMiB() != null) {
data.set("memoryLimitMiB", om.valueToTree(this.getMemoryLimitMiB()));
}
if (this.getExecutionRole() != null) {
data.set("executionRole", om.valueToTree(this.getExecutionRole()));
}
if (this.getFamily() != null) {
data.set("family", om.valueToTree(this.getFamily()));
}
if (this.getProxyConfiguration() != null) {
data.set("proxyConfiguration", om.valueToTree(this.getProxyConfiguration()));
}
if (this.getTaskRole() != null) {
data.set("taskRole", om.valueToTree(this.getTaskRole()));
}
if (this.getVolumes() != null) {
data.set("volumes", om.valueToTree(this.getVolumes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.FargateTaskDefinitionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FargateTaskDefinitionProps.Jsii$Proxy that = (FargateTaskDefinitionProps.Jsii$Proxy) o;
if (this.cpu != null ? !this.cpu.equals(that.cpu) : that.cpu != null) return false;
if (this.memoryLimitMiB != null ? !this.memoryLimitMiB.equals(that.memoryLimitMiB) : that.memoryLimitMiB != null) return false;
if (this.executionRole != null ? !this.executionRole.equals(that.executionRole) : that.executionRole != null) return false;
if (this.family != null ? !this.family.equals(that.family) : that.family != null) return false;
if (this.proxyConfiguration != null ? !this.proxyConfiguration.equals(that.proxyConfiguration) : that.proxyConfiguration != null) return false;
if (this.taskRole != null ? !this.taskRole.equals(that.taskRole) : that.taskRole != null) return false;
return this.volumes != null ? this.volumes.equals(that.volumes) : that.volumes == null;
}
@Override
public int hashCode() {
int result = this.cpu != null ? this.cpu.hashCode() : 0;
result = 31 * result + (this.memoryLimitMiB != null ? this.memoryLimitMiB.hashCode() : 0);
result = 31 * result + (this.executionRole != null ? this.executionRole.hashCode() : 0);
result = 31 * result + (this.family != null ? this.family.hashCode() : 0);
result = 31 * result + (this.proxyConfiguration != null ? this.proxyConfiguration.hashCode() : 0);
result = 31 * result + (this.taskRole != null ? this.taskRole.hashCode() : 0);
result = 31 * result + (this.volumes != null ? this.volumes.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy