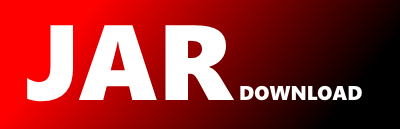
software.amazon.awscdk.services.ecs.FluentdLogDriverProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The CDK Construct Library for AWS::ECS
package software.amazon.awscdk.services.ecs;
/**
* Specifies the fluentd log driver configuration options.
*
* [Source](https://docs.docker.com/config/containers/logging/fluentd/)
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:18:01.448Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.FluentdLogDriverProps")
@software.amazon.jsii.Jsii.Proxy(FluentdLogDriverProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface FluentdLogDriverProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.BaseLogDriverProps {
/**
* By default, the logging driver connects to localhost:24224.
*
* Supply the
* address option to connect to a different address. tcp(default) and unix
* sockets are supported.
*
* Default: - address not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.String getAddress() {
return null;
}
/**
* Docker connects to Fluentd in the background.
*
* Messages are buffered until
* the connection is established.
*
* Default: - false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Boolean getAsyncConnect() {
return null;
}
/**
* The amount of data to buffer before flushing to disk.
*
* Default: - The amount of RAM available to the container.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Number getBufferLimit() {
return null;
}
/**
* The maximum number of retries.
*
* Default: - 4294967295 (2**32 - 1).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Number getMaxRetries() {
return null;
}
/**
* How long to wait between retries.
*
* Default: - 1 second
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default software.amazon.awscdk.core.Duration getRetryWait() {
return null;
}
/**
* Generates event logs in nanosecond resolution.
*
* Default: - false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default java.lang.Boolean getSubSecondPrecision() {
return null;
}
/**
* @return a {@link Builder} of {@link FluentdLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FluentdLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.String address;
private java.lang.Boolean asyncConnect;
private java.lang.Number bufferLimit;
private java.lang.Number maxRetries;
private software.amazon.awscdk.core.Duration retryWait;
private java.lang.Boolean subSecondPrecision;
private java.util.List env;
private java.lang.String envRegex;
private java.util.List labels;
private java.lang.String tag;
/**
* Sets the value of Address
* @param address By default, the logging driver connects to localhost:24224.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder address(java.lang.String address) {
this.address = address;
return this;
}
/**
* Sets the value of AsyncConnect
* @param asyncConnect Docker connects to Fluentd in the background.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder asyncConnect(java.lang.Boolean asyncConnect) {
this.asyncConnect = asyncConnect;
return this;
}
/**
* Sets the value of BufferLimit
* @param bufferLimit The amount of data to buffer before flushing to disk.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bufferLimit(java.lang.Number bufferLimit) {
this.bufferLimit = bufferLimit;
return this;
}
/**
* Sets the value of MaxRetries
* @param maxRetries The maximum number of retries.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxRetries(java.lang.Number maxRetries) {
this.maxRetries = maxRetries;
return this;
}
/**
* Sets the value of RetryWait
* @param retryWait How long to wait between retries.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder retryWait(software.amazon.awscdk.core.Duration retryWait) {
this.retryWait = retryWait;
return this;
}
/**
* Sets the value of SubSecondPrecision
* @param subSecondPrecision Generates event logs in nanosecond resolution.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subSecondPrecision(java.lang.Boolean subSecondPrecision) {
this.subSecondPrecision = subSecondPrecision;
return this;
}
/**
* Sets the value of Env
* @param env The env option takes an array of keys.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(java.util.List env) {
this.env = env;
return this;
}
/**
* Sets the value of EnvRegex
* @param envRegex The env-regex option is similar to and compatible with env.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(java.lang.String envRegex) {
this.envRegex = envRegex;
return this;
}
/**
* Sets the value of Labels
* @param labels The labels option takes an array of keys.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(java.util.List labels) {
this.labels = labels;
return this;
}
/**
* Sets the value of Tag
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(java.lang.String tag) {
this.tag = tag;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FluentdLogDriverProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public FluentdLogDriverProps build() {
return new Jsii$Proxy(address, asyncConnect, bufferLimit, maxRetries, retryWait, subSecondPrecision, env, envRegex, labels, tag);
}
}
/**
* An implementation for {@link FluentdLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FluentdLogDriverProps {
private final java.lang.String address;
private final java.lang.Boolean asyncConnect;
private final java.lang.Number bufferLimit;
private final java.lang.Number maxRetries;
private final software.amazon.awscdk.core.Duration retryWait;
private final java.lang.Boolean subSecondPrecision;
private final java.util.List env;
private final java.lang.String envRegex;
private final java.util.List labels;
private final java.lang.String tag;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.address = this.jsiiGet("address", java.lang.String.class);
this.asyncConnect = this.jsiiGet("asyncConnect", java.lang.Boolean.class);
this.bufferLimit = this.jsiiGet("bufferLimit", java.lang.Number.class);
this.maxRetries = this.jsiiGet("maxRetries", java.lang.Number.class);
this.retryWait = this.jsiiGet("retryWait", software.amazon.awscdk.core.Duration.class);
this.subSecondPrecision = this.jsiiGet("subSecondPrecision", java.lang.Boolean.class);
this.env = this.jsiiGet("env", java.util.List.class);
this.envRegex = this.jsiiGet("envRegex", java.lang.String.class);
this.labels = this.jsiiGet("labels", java.util.List.class);
this.tag = this.jsiiGet("tag", java.lang.String.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.String address, final java.lang.Boolean asyncConnect, final java.lang.Number bufferLimit, final java.lang.Number maxRetries, final software.amazon.awscdk.core.Duration retryWait, final java.lang.Boolean subSecondPrecision, final java.util.List env, final java.lang.String envRegex, final java.util.List labels, final java.lang.String tag) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.address = address;
this.asyncConnect = asyncConnect;
this.bufferLimit = bufferLimit;
this.maxRetries = maxRetries;
this.retryWait = retryWait;
this.subSecondPrecision = subSecondPrecision;
this.env = env;
this.envRegex = envRegex;
this.labels = labels;
this.tag = tag;
}
@Override
public java.lang.String getAddress() {
return this.address;
}
@Override
public java.lang.Boolean getAsyncConnect() {
return this.asyncConnect;
}
@Override
public java.lang.Number getBufferLimit() {
return this.bufferLimit;
}
@Override
public java.lang.Number getMaxRetries() {
return this.maxRetries;
}
@Override
public software.amazon.awscdk.core.Duration getRetryWait() {
return this.retryWait;
}
@Override
public java.lang.Boolean getSubSecondPrecision() {
return this.subSecondPrecision;
}
@Override
public java.util.List getEnv() {
return this.env;
}
@Override
public java.lang.String getEnvRegex() {
return this.envRegex;
}
@Override
public java.util.List getLabels() {
return this.labels;
}
@Override
public java.lang.String getTag() {
return this.tag;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAddress() != null) {
data.set("address", om.valueToTree(this.getAddress()));
}
if (this.getAsyncConnect() != null) {
data.set("asyncConnect", om.valueToTree(this.getAsyncConnect()));
}
if (this.getBufferLimit() != null) {
data.set("bufferLimit", om.valueToTree(this.getBufferLimit()));
}
if (this.getMaxRetries() != null) {
data.set("maxRetries", om.valueToTree(this.getMaxRetries()));
}
if (this.getRetryWait() != null) {
data.set("retryWait", om.valueToTree(this.getRetryWait()));
}
if (this.getSubSecondPrecision() != null) {
data.set("subSecondPrecision", om.valueToTree(this.getSubSecondPrecision()));
}
if (this.getEnv() != null) {
data.set("env", om.valueToTree(this.getEnv()));
}
if (this.getEnvRegex() != null) {
data.set("envRegex", om.valueToTree(this.getEnvRegex()));
}
if (this.getLabels() != null) {
data.set("labels", om.valueToTree(this.getLabels()));
}
if (this.getTag() != null) {
data.set("tag", om.valueToTree(this.getTag()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.FluentdLogDriverProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FluentdLogDriverProps.Jsii$Proxy that = (FluentdLogDriverProps.Jsii$Proxy) o;
if (this.address != null ? !this.address.equals(that.address) : that.address != null) return false;
if (this.asyncConnect != null ? !this.asyncConnect.equals(that.asyncConnect) : that.asyncConnect != null) return false;
if (this.bufferLimit != null ? !this.bufferLimit.equals(that.bufferLimit) : that.bufferLimit != null) return false;
if (this.maxRetries != null ? !this.maxRetries.equals(that.maxRetries) : that.maxRetries != null) return false;
if (this.retryWait != null ? !this.retryWait.equals(that.retryWait) : that.retryWait != null) return false;
if (this.subSecondPrecision != null ? !this.subSecondPrecision.equals(that.subSecondPrecision) : that.subSecondPrecision != null) return false;
if (this.env != null ? !this.env.equals(that.env) : that.env != null) return false;
if (this.envRegex != null ? !this.envRegex.equals(that.envRegex) : that.envRegex != null) return false;
if (this.labels != null ? !this.labels.equals(that.labels) : that.labels != null) return false;
return this.tag != null ? this.tag.equals(that.tag) : that.tag == null;
}
@Override
public int hashCode() {
int result = this.address != null ? this.address.hashCode() : 0;
result = 31 * result + (this.asyncConnect != null ? this.asyncConnect.hashCode() : 0);
result = 31 * result + (this.bufferLimit != null ? this.bufferLimit.hashCode() : 0);
result = 31 * result + (this.maxRetries != null ? this.maxRetries.hashCode() : 0);
result = 31 * result + (this.retryWait != null ? this.retryWait.hashCode() : 0);
result = 31 * result + (this.subSecondPrecision != null ? this.subSecondPrecision.hashCode() : 0);
result = 31 * result + (this.env != null ? this.env.hashCode() : 0);
result = 31 * result + (this.envRegex != null ? this.envRegex.hashCode() : 0);
result = 31 * result + (this.labels != null ? this.labels.hashCode() : 0);
result = 31 * result + (this.tag != null ? this.tag.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy