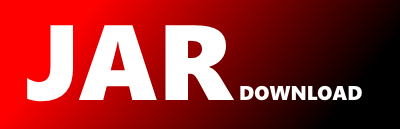
software.amazon.awscdk.services.ecs.ScratchSpace Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The CDK Construct Library for AWS::ECS
package software.amazon.awscdk.services.ecs;
/**
* The temporary disk space mounted to the container.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.20.2 (build faba0be)", date = "2019-11-11T17:18:01.453Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.ScratchSpace")
@software.amazon.jsii.Jsii.Proxy(ScratchSpace.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ScratchSpace extends software.amazon.jsii.JsiiSerializable {
/**
* The path on the container to mount the scratch volume at.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getContainerPath();
/**
* The name of the scratch volume to mount.
*
* Must be a volume name referenced in the name parameter of task definition volume.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getName();
/**
* Specifies whether to give the container read-only access to the scratch volume.
*
* If this value is true, the container has read-only access to the scratch volume.
* If this value is false, then the container can write to the scratch volume.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.Boolean getReadOnly();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getSourcePath();
/**
* @return a {@link Builder} of {@link ScratchSpace}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ScratchSpace}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.String containerPath;
private java.lang.String name;
private java.lang.Boolean readOnly;
private java.lang.String sourcePath;
/**
* Sets the value of ContainerPath
* @param containerPath The path on the container to mount the scratch volume at. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerPath(java.lang.String containerPath) {
this.containerPath = containerPath;
return this;
}
/**
* Sets the value of Name
* @param name The name of the scratch volume to mount. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of ReadOnly
* @param readOnly Specifies whether to give the container read-only access to the scratch volume. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder readOnly(java.lang.Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
/**
* Sets the value of SourcePath
* @param sourcePath the value to be set. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourcePath(java.lang.String sourcePath) {
this.sourcePath = sourcePath;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ScratchSpace}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public ScratchSpace build() {
return new Jsii$Proxy(containerPath, name, readOnly, sourcePath);
}
}
/**
* An implementation for {@link ScratchSpace}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ScratchSpace {
private final java.lang.String containerPath;
private final java.lang.String name;
private final java.lang.Boolean readOnly;
private final java.lang.String sourcePath;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerPath = this.jsiiGet("containerPath", java.lang.String.class);
this.name = this.jsiiGet("name", java.lang.String.class);
this.readOnly = this.jsiiGet("readOnly", java.lang.Boolean.class);
this.sourcePath = this.jsiiGet("sourcePath", java.lang.String.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(final java.lang.String containerPath, final java.lang.String name, final java.lang.Boolean readOnly, final java.lang.String sourcePath) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerPath = java.util.Objects.requireNonNull(containerPath, "containerPath is required");
this.name = java.util.Objects.requireNonNull(name, "name is required");
this.readOnly = java.util.Objects.requireNonNull(readOnly, "readOnly is required");
this.sourcePath = java.util.Objects.requireNonNull(sourcePath, "sourcePath is required");
}
@Override
public java.lang.String getContainerPath() {
return this.containerPath;
}
@Override
public java.lang.String getName() {
return this.name;
}
@Override
public java.lang.Boolean getReadOnly() {
return this.readOnly;
}
@Override
public java.lang.String getSourcePath() {
return this.sourcePath;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("containerPath", om.valueToTree(this.getContainerPath()));
data.set("name", om.valueToTree(this.getName()));
data.set("readOnly", om.valueToTree(this.getReadOnly()));
data.set("sourcePath", om.valueToTree(this.getSourcePath()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.ScratchSpace"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ScratchSpace.Jsii$Proxy that = (ScratchSpace.Jsii$Proxy) o;
if (!containerPath.equals(that.containerPath)) return false;
if (!name.equals(that.name)) return false;
if (!readOnly.equals(that.readOnly)) return false;
return this.sourcePath.equals(that.sourcePath);
}
@Override
public int hashCode() {
int result = this.containerPath.hashCode();
result = 31 * result + (this.name.hashCode());
result = 31 * result + (this.readOnly.hashCode());
result = 31 * result + (this.sourcePath.hashCode());
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy