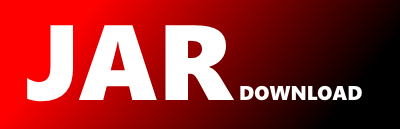
software.amazon.awscdk.services.ecs.CloudMapOptions Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* The options to enabling AWS Cloud Map for an Amazon ECS service.
*
* Example:
*
*
* TaskDefinition taskDefinition;
* Cluster cluster;
* Ec2Service service = Ec2Service.Builder.create(this, "Service")
* .cluster(cluster)
* .taskDefinition(taskDefinition)
* .cloudMapOptions(CloudMapOptions.builder()
* // Create A records - useful for AWSVPC network mode.
* .dnsRecordType(DnsRecordType.A)
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-28T21:34:28.845Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CloudMapOptions")
@software.amazon.jsii.Jsii.Proxy(CloudMapOptions.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CloudMapOptions extends software.amazon.jsii.JsiiSerializable {
/**
* The service discovery namespace for the Cloud Map service to attach to the ECS service.
*
* Default: - the defaultCloudMapNamespace associated to the cluster
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.servicediscovery.INamespace getCloudMapNamespace() {
return null;
}
/**
* The container to point to for a SRV record.
*
* Default: - the task definition's default container
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.ContainerDefinition getContainer() {
return null;
}
/**
* The port to point to for a SRV record.
*
* Default: - the default port of the task definition's default container
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getContainerPort() {
return null;
}
/**
* The DNS record type that you want AWS Cloud Map to create.
*
* The supported record types are A or SRV.
*
* Default: - DnsRecordType.A if TaskDefinition.networkMode = AWS_VPC, otherwise DnsRecordType.SRV
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.servicediscovery.DnsRecordType getDnsRecordType() {
return null;
}
/**
* The amount of time that you want DNS resolvers to cache the settings for this record.
*
* Default: Duration.minutes(1)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getDnsTtl() {
return null;
}
/**
* The number of 30-second intervals that you want Cloud Map to wait after receiving an UpdateInstanceCustomHealthStatus request before it changes the health status of a service instance.
*
* NOTE: This is used for HealthCheckCustomConfig
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getFailureThreshold() {
return null;
}
/**
* The name of the Cloud Map service to attach to the ECS service.
*
* Default: CloudFormation-generated name
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getName() {
return null;
}
/**
* @return a {@link Builder} of {@link CloudMapOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CloudMapOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.servicediscovery.INamespace cloudMapNamespace;
software.amazon.awscdk.services.ecs.ContainerDefinition container;
java.lang.Number containerPort;
software.amazon.awscdk.services.servicediscovery.DnsRecordType dnsRecordType;
software.amazon.awscdk.core.Duration dnsTtl;
java.lang.Number failureThreshold;
java.lang.String name;
/**
* Sets the value of {@link CloudMapOptions#getCloudMapNamespace}
* @param cloudMapNamespace The service discovery namespace for the Cloud Map service to attach to the ECS service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudMapNamespace(software.amazon.awscdk.services.servicediscovery.INamespace cloudMapNamespace) {
this.cloudMapNamespace = cloudMapNamespace;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getContainer}
* @param container The container to point to for a SRV record.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder container(software.amazon.awscdk.services.ecs.ContainerDefinition container) {
this.container = container;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getContainerPort}
* @param containerPort The port to point to for a SRV record.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerPort(java.lang.Number containerPort) {
this.containerPort = containerPort;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getDnsRecordType}
* @param dnsRecordType The DNS record type that you want AWS Cloud Map to create.
* The supported record types are A or SRV.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dnsRecordType(software.amazon.awscdk.services.servicediscovery.DnsRecordType dnsRecordType) {
this.dnsRecordType = dnsRecordType;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getDnsTtl}
* @param dnsTtl The amount of time that you want DNS resolvers to cache the settings for this record.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dnsTtl(software.amazon.awscdk.core.Duration dnsTtl) {
this.dnsTtl = dnsTtl;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getFailureThreshold}
* @param failureThreshold The number of 30-second intervals that you want Cloud Map to wait after receiving an UpdateInstanceCustomHealthStatus request before it changes the health status of a service instance.
* NOTE: This is used for HealthCheckCustomConfig
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder failureThreshold(java.lang.Number failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
/**
* Sets the value of {@link CloudMapOptions#getName}
* @param name The name of the Cloud Map service to attach to the ECS service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CloudMapOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CloudMapOptions build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CloudMapOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CloudMapOptions {
private final software.amazon.awscdk.services.servicediscovery.INamespace cloudMapNamespace;
private final software.amazon.awscdk.services.ecs.ContainerDefinition container;
private final java.lang.Number containerPort;
private final software.amazon.awscdk.services.servicediscovery.DnsRecordType dnsRecordType;
private final software.amazon.awscdk.core.Duration dnsTtl;
private final java.lang.Number failureThreshold;
private final java.lang.String name;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.cloudMapNamespace = software.amazon.jsii.Kernel.get(this, "cloudMapNamespace", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.servicediscovery.INamespace.class));
this.container = software.amazon.jsii.Kernel.get(this, "container", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.ContainerDefinition.class));
this.containerPort = software.amazon.jsii.Kernel.get(this, "containerPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.dnsRecordType = software.amazon.jsii.Kernel.get(this, "dnsRecordType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.servicediscovery.DnsRecordType.class));
this.dnsTtl = software.amazon.jsii.Kernel.get(this, "dnsTtl", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.failureThreshold = software.amazon.jsii.Kernel.get(this, "failureThreshold", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.cloudMapNamespace = builder.cloudMapNamespace;
this.container = builder.container;
this.containerPort = builder.containerPort;
this.dnsRecordType = builder.dnsRecordType;
this.dnsTtl = builder.dnsTtl;
this.failureThreshold = builder.failureThreshold;
this.name = builder.name;
}
@Override
public final software.amazon.awscdk.services.servicediscovery.INamespace getCloudMapNamespace() {
return this.cloudMapNamespace;
}
@Override
public final software.amazon.awscdk.services.ecs.ContainerDefinition getContainer() {
return this.container;
}
@Override
public final java.lang.Number getContainerPort() {
return this.containerPort;
}
@Override
public final software.amazon.awscdk.services.servicediscovery.DnsRecordType getDnsRecordType() {
return this.dnsRecordType;
}
@Override
public final software.amazon.awscdk.core.Duration getDnsTtl() {
return this.dnsTtl;
}
@Override
public final java.lang.Number getFailureThreshold() {
return this.failureThreshold;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCloudMapNamespace() != null) {
data.set("cloudMapNamespace", om.valueToTree(this.getCloudMapNamespace()));
}
if (this.getContainer() != null) {
data.set("container", om.valueToTree(this.getContainer()));
}
if (this.getContainerPort() != null) {
data.set("containerPort", om.valueToTree(this.getContainerPort()));
}
if (this.getDnsRecordType() != null) {
data.set("dnsRecordType", om.valueToTree(this.getDnsRecordType()));
}
if (this.getDnsTtl() != null) {
data.set("dnsTtl", om.valueToTree(this.getDnsTtl()));
}
if (this.getFailureThreshold() != null) {
data.set("failureThreshold", om.valueToTree(this.getFailureThreshold()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CloudMapOptions"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CloudMapOptions.Jsii$Proxy that = (CloudMapOptions.Jsii$Proxy) o;
if (this.cloudMapNamespace != null ? !this.cloudMapNamespace.equals(that.cloudMapNamespace) : that.cloudMapNamespace != null) return false;
if (this.container != null ? !this.container.equals(that.container) : that.container != null) return false;
if (this.containerPort != null ? !this.containerPort.equals(that.containerPort) : that.containerPort != null) return false;
if (this.dnsRecordType != null ? !this.dnsRecordType.equals(that.dnsRecordType) : that.dnsRecordType != null) return false;
if (this.dnsTtl != null ? !this.dnsTtl.equals(that.dnsTtl) : that.dnsTtl != null) return false;
if (this.failureThreshold != null ? !this.failureThreshold.equals(that.failureThreshold) : that.failureThreshold != null) return false;
return this.name != null ? this.name.equals(that.name) : that.name == null;
}
@Override
public final int hashCode() {
int result = this.cloudMapNamespace != null ? this.cloudMapNamespace.hashCode() : 0;
result = 31 * result + (this.container != null ? this.container.hashCode() : 0);
result = 31 * result + (this.containerPort != null ? this.containerPort.hashCode() : 0);
result = 31 * result + (this.dnsRecordType != null ? this.dnsRecordType.hashCode() : 0);
result = 31 * result + (this.dnsTtl != null ? this.dnsTtl.hashCode() : 0);
result = 31 * result + (this.failureThreshold != null ? this.failureThreshold.hashCode() : 0);
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
return result;
}
}
}