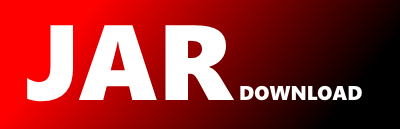
software.amazon.awscdk.services.ecs.Ec2TaskDefinition Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* The details of a task definition run on an EC2 cluster.
*
* Example:
*
*
* // Create a Task Definition for the container to start
* Ec2TaskDefinition taskDefinition = new Ec2TaskDefinition(this, "TaskDef");
* taskDefinition.addContainer("TheContainer", ContainerDefinitionOptions.builder()
* .image(ContainerImage.fromRegistry("example-image"))
* .memoryLimitMiB(256)
* .logging(LogDrivers.splunk(SplunkLogDriverProps.builder()
* .token(SecretValue.secretsManager("my-splunk-token"))
* .url("my-splunk-url")
* .build()))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-28T21:34:28.880Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.Ec2TaskDefinition")
public class Ec2TaskDefinition extends software.amazon.awscdk.services.ecs.TaskDefinition implements software.amazon.awscdk.services.ecs.IEc2TaskDefinition {
protected Ec2TaskDefinition(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Ec2TaskDefinition(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the Ec2TaskDefinition class.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Ec2TaskDefinition(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.Ec2TaskDefinitionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* Constructs a new instance of the Ec2TaskDefinition class.
*
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Ec2TaskDefinition(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Imports a task definition from the specified task definition ARN.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param ec2TaskDefinitionArn This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.IEc2TaskDefinition fromEc2TaskDefinitionArn(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String ec2TaskDefinitionArn) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.ecs.Ec2TaskDefinition.class, "fromEc2TaskDefinitionArn", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.IEc2TaskDefinition.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(ec2TaskDefinitionArn, "ec2TaskDefinitionArn is required") });
}
/**
* Imports an existing Ec2 task definition from its attributes.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param attrs This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.IEc2TaskDefinition fromEc2TaskDefinitionAttributes(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.Ec2TaskDefinitionAttributes attrs) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.ecs.Ec2TaskDefinition.class, "fromEc2TaskDefinitionAttributes", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.IEc2TaskDefinition.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(attrs, "attrs is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.Ec2TaskDefinition}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private software.amazon.awscdk.services.ecs.Ec2TaskDefinitionProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* The name of the IAM task execution role that grants the ECS agent permission to call AWS APIs on your behalf.
*
* The role will be used to retrieve container images from ECR and create CloudWatch log groups.
*
* Default: - An execution role will be automatically created if you use ECR images in your task definition.
*
* @return {@code this}
* @param executionRole The name of the IAM task execution role that grants the ECS agent permission to call AWS APIs on your behalf. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executionRole(final software.amazon.awscdk.services.iam.IRole executionRole) {
this.props().executionRole(executionRole);
return this;
}
/**
* The name of a family that this task definition is registered to.
*
* A family groups multiple versions of a task definition.
*
* Default: - Automatically generated name.
*
* @return {@code this}
* @param family The name of a family that this task definition is registered to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder family(final java.lang.String family) {
this.props().family(family);
return this;
}
/**
* The configuration details for the App Mesh proxy.
*
* Default: - No proxy configuration.
*
* @return {@code this}
* @param proxyConfiguration The configuration details for the App Mesh proxy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder proxyConfiguration(final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration) {
this.props().proxyConfiguration(proxyConfiguration);
return this;
}
/**
* The name of the IAM role that grants containers in the task permission to call AWS APIs on your behalf.
*
* Default: - A task role is automatically created for you.
*
* @return {@code this}
* @param taskRole The name of the IAM role that grants containers in the task permission to call AWS APIs on your behalf. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskRole(final software.amazon.awscdk.services.iam.IRole taskRole) {
this.props().taskRole(taskRole);
return this;
}
/**
* The list of volume definitions for the task.
*
* For more information, see
* Task Definition Parameter Volumes.
*
* Default: - No volumes are passed to the Docker daemon on a container instance.
*
* @return {@code this}
* @param volumes The list of volume definitions for the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder volumes(final java.util.List extends software.amazon.awscdk.services.ecs.Volume> volumes) {
this.props().volumes(volumes);
return this;
}
/**
* The inference accelerators to use for the containers in the task.
*
* Not supported in Fargate.
*
* Default: - No inference accelerators.
*
* @return {@code this}
* @param inferenceAccelerators The inference accelerators to use for the containers in the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder inferenceAccelerators(final java.util.List extends software.amazon.awscdk.services.ecs.InferenceAccelerator> inferenceAccelerators) {
this.props().inferenceAccelerators(inferenceAccelerators);
return this;
}
/**
* The IPC resource namespace to use for the containers in the task.
*
* Not supported in Fargate and Windows containers.
*
* Default: - IpcMode used by the task is not specified
*
* @return {@code this}
* @param ipcMode The IPC resource namespace to use for the containers in the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ipcMode(final software.amazon.awscdk.services.ecs.IpcMode ipcMode) {
this.props().ipcMode(ipcMode);
return this;
}
/**
* The Docker networking mode to use for the containers in the task.
*
* The valid values are NONE, BRIDGE, AWS_VPC, and HOST.
*
* Default: - NetworkMode.BRIDGE for EC2 tasks, AWS_VPC for Fargate tasks.
*
* @return {@code this}
* @param networkMode The Docker networking mode to use for the containers in the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkMode(final software.amazon.awscdk.services.ecs.NetworkMode networkMode) {
this.props().networkMode(networkMode);
return this;
}
/**
* The process namespace to use for the containers in the task.
*
* Not supported in Fargate and Windows containers.
*
* Default: - PidMode used by the task is not specified
*
* @return {@code this}
* @param pidMode The process namespace to use for the containers in the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pidMode(final software.amazon.awscdk.services.ecs.PidMode pidMode) {
this.props().pidMode(pidMode);
return this;
}
/**
* An array of placement constraint objects to use for the task.
*
* You can
* specify a maximum of 10 constraints per task (this limit includes
* constraints in the task definition and those specified at run time).
*
* Default: - No placement constraints.
*
* @return {@code this}
* @param placementConstraints An array of placement constraint objects to use for the task. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementConstraints(final java.util.List extends software.amazon.awscdk.services.ecs.PlacementConstraint> placementConstraints) {
this.props().placementConstraints(placementConstraints);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.Ec2TaskDefinition}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.Ec2TaskDefinition build() {
return new software.amazon.awscdk.services.ecs.Ec2TaskDefinition(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.ecs.Ec2TaskDefinitionProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.ecs.Ec2TaskDefinitionProps.Builder();
}
return this.props;
}
}
}