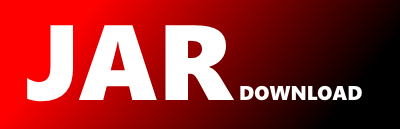
software.amazon.awscdk.services.ecs.FargateTaskDefinitionProps Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* The properties for a task definition.
*
* Example:
*
*
* FargateTaskDefinition fargateTaskDefinition = FargateTaskDefinition.Builder.create(this, "TaskDef")
* .memoryLimitMiB(512)
* .cpu(256)
* .build();
* ContainerDefinition container = fargateTaskDefinition.addContainer("WebContainer", ContainerDefinitionOptions.builder()
* // Use an image from DockerHub
* .image(ContainerImage.fromRegistry("amazon/amazon-ecs-sample"))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-28T21:34:28.929Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.FargateTaskDefinitionProps")
@software.amazon.jsii.Jsii.Proxy(FargateTaskDefinitionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface FargateTaskDefinitionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.CommonTaskDefinitionProps {
/**
* The number of cpu units used by the task.
*
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values,
* which determines your range of valid values for the memory parameter:
*
* 256 (.25 vCPU) - Available memory values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
* 512 (.5 vCPU) - Available memory values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
* 1024 (1 vCPU) - Available memory values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
* 2048 (2 vCPU) - Available memory values: Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
* 4096 (4 vCPU) - Available memory values: Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
* Default: 256
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getCpu() {
return null;
}
/**
* The amount (in GiB) of ephemeral storage to be allocated to the task.
*
* The maximum supported value is 200 GiB.
*
* NOTE: This parameter is only supported for tasks hosted on AWS Fargate using platform version 1.4.0 or later.
*
* Default: 20
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getEphemeralStorageGiB() {
return null;
}
/**
* The amount (in MiB) of memory used by the task.
*
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values, which determines your range of valid values for the cpu parameter:
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu values: 256 (.25 vCPU)
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu values: 512 (.5 vCPU)
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available cpu values: 1024 (1 vCPU)
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu values: 2048 (2 vCPU)
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu values: 4096 (4 vCPU)
*
* Default: 512
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getMemoryLimitMiB() {
return null;
}
/**
* The operating system that your task definitions are running on.
*
* A runtimePlatform is supported only for tasks using the Fargate launch type.
*
* Default: - Undefined.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.RuntimePlatform getRuntimePlatform() {
return null;
}
/**
* @return a {@link Builder} of {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Number cpu;
java.lang.Number ephemeralStorageGiB;
java.lang.Number memoryLimitMiB;
software.amazon.awscdk.services.ecs.RuntimePlatform runtimePlatform;
software.amazon.awscdk.services.iam.IRole executionRole;
java.lang.String family;
software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
software.amazon.awscdk.services.iam.IRole taskRole;
java.util.List volumes;
/**
* Sets the value of {@link FargateTaskDefinitionProps#getCpu}
* @param cpu The number of cpu units used by the task.
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values,
* which determines your range of valid values for the memory parameter:
*
* 256 (.25 vCPU) - Available memory values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
* 512 (.5 vCPU) - Available memory values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
* 1024 (1 vCPU) - Available memory values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
* 2048 (2 vCPU) - Available memory values: Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
* 4096 (4 vCPU) - Available memory values: Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cpu(java.lang.Number cpu) {
this.cpu = cpu;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getEphemeralStorageGiB}
* @param ephemeralStorageGiB The amount (in GiB) of ephemeral storage to be allocated to the task.
* The maximum supported value is 200 GiB.
*
* NOTE: This parameter is only supported for tasks hosted on AWS Fargate using platform version 1.4.0 or later.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ephemeralStorageGiB(java.lang.Number ephemeralStorageGiB) {
this.ephemeralStorageGiB = ephemeralStorageGiB;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getMemoryLimitMiB}
* @param memoryLimitMiB The amount (in MiB) of memory used by the task.
* For tasks using the Fargate launch type,
* this field is required and you must use one of the following values, which determines your range of valid values for the cpu parameter:
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu values: 256 (.25 vCPU)
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu values: 512 (.5 vCPU)
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available cpu values: 1024 (1 vCPU)
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu values: 2048 (2 vCPU)
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu values: 4096 (4 vCPU)
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memoryLimitMiB(java.lang.Number memoryLimitMiB) {
this.memoryLimitMiB = memoryLimitMiB;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getRuntimePlatform}
* @param runtimePlatform The operating system that your task definitions are running on.
* A runtimePlatform is supported only for tasks using the Fargate launch type.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runtimePlatform(software.amazon.awscdk.services.ecs.RuntimePlatform runtimePlatform) {
this.runtimePlatform = runtimePlatform;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getExecutionRole}
* @param executionRole The name of the IAM task execution role that grants the ECS agent permission to call AWS APIs on your behalf.
* The role will be used to retrieve container images from ECR and create CloudWatch log groups.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executionRole(software.amazon.awscdk.services.iam.IRole executionRole) {
this.executionRole = executionRole;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getFamily}
* @param family The name of a family that this task definition is registered to.
* A family groups multiple versions of a task definition.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder family(java.lang.String family) {
this.family = family;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getProxyConfiguration}
* @param proxyConfiguration The configuration details for the App Mesh proxy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder proxyConfiguration(software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getTaskRole}
* @param taskRole The name of the IAM role that grants containers in the task permission to call AWS APIs on your behalf.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskRole(software.amazon.awscdk.services.iam.IRole taskRole) {
this.taskRole = taskRole;
return this;
}
/**
* Sets the value of {@link FargateTaskDefinitionProps#getVolumes}
* @param volumes The list of volume definitions for the task.
* For more information, see
* Task Definition Parameter Volumes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder volumes(java.util.List extends software.amazon.awscdk.services.ecs.Volume> volumes) {
this.volumes = (java.util.List)volumes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FargateTaskDefinitionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public FargateTaskDefinitionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link FargateTaskDefinitionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FargateTaskDefinitionProps {
private final java.lang.Number cpu;
private final java.lang.Number ephemeralStorageGiB;
private final java.lang.Number memoryLimitMiB;
private final software.amazon.awscdk.services.ecs.RuntimePlatform runtimePlatform;
private final software.amazon.awscdk.services.iam.IRole executionRole;
private final java.lang.String family;
private final software.amazon.awscdk.services.ecs.ProxyConfiguration proxyConfiguration;
private final software.amazon.awscdk.services.iam.IRole taskRole;
private final java.util.List volumes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.cpu = software.amazon.jsii.Kernel.get(this, "cpu", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.ephemeralStorageGiB = software.amazon.jsii.Kernel.get(this, "ephemeralStorageGiB", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.memoryLimitMiB = software.amazon.jsii.Kernel.get(this, "memoryLimitMiB", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.runtimePlatform = software.amazon.jsii.Kernel.get(this, "runtimePlatform", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.RuntimePlatform.class));
this.executionRole = software.amazon.jsii.Kernel.get(this, "executionRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.family = software.amazon.jsii.Kernel.get(this, "family", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.proxyConfiguration = software.amazon.jsii.Kernel.get(this, "proxyConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.ProxyConfiguration.class));
this.taskRole = software.amazon.jsii.Kernel.get(this, "taskRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.volumes = software.amazon.jsii.Kernel.get(this, "volumes", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.Volume.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.cpu = builder.cpu;
this.ephemeralStorageGiB = builder.ephemeralStorageGiB;
this.memoryLimitMiB = builder.memoryLimitMiB;
this.runtimePlatform = builder.runtimePlatform;
this.executionRole = builder.executionRole;
this.family = builder.family;
this.proxyConfiguration = builder.proxyConfiguration;
this.taskRole = builder.taskRole;
this.volumes = (java.util.List)builder.volumes;
}
@Override
public final java.lang.Number getCpu() {
return this.cpu;
}
@Override
public final java.lang.Number getEphemeralStorageGiB() {
return this.ephemeralStorageGiB;
}
@Override
public final java.lang.Number getMemoryLimitMiB() {
return this.memoryLimitMiB;
}
@Override
public final software.amazon.awscdk.services.ecs.RuntimePlatform getRuntimePlatform() {
return this.runtimePlatform;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getExecutionRole() {
return this.executionRole;
}
@Override
public final java.lang.String getFamily() {
return this.family;
}
@Override
public final software.amazon.awscdk.services.ecs.ProxyConfiguration getProxyConfiguration() {
return this.proxyConfiguration;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getTaskRole() {
return this.taskRole;
}
@Override
public final java.util.List getVolumes() {
return this.volumes;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCpu() != null) {
data.set("cpu", om.valueToTree(this.getCpu()));
}
if (this.getEphemeralStorageGiB() != null) {
data.set("ephemeralStorageGiB", om.valueToTree(this.getEphemeralStorageGiB()));
}
if (this.getMemoryLimitMiB() != null) {
data.set("memoryLimitMiB", om.valueToTree(this.getMemoryLimitMiB()));
}
if (this.getRuntimePlatform() != null) {
data.set("runtimePlatform", om.valueToTree(this.getRuntimePlatform()));
}
if (this.getExecutionRole() != null) {
data.set("executionRole", om.valueToTree(this.getExecutionRole()));
}
if (this.getFamily() != null) {
data.set("family", om.valueToTree(this.getFamily()));
}
if (this.getProxyConfiguration() != null) {
data.set("proxyConfiguration", om.valueToTree(this.getProxyConfiguration()));
}
if (this.getTaskRole() != null) {
data.set("taskRole", om.valueToTree(this.getTaskRole()));
}
if (this.getVolumes() != null) {
data.set("volumes", om.valueToTree(this.getVolumes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.FargateTaskDefinitionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FargateTaskDefinitionProps.Jsii$Proxy that = (FargateTaskDefinitionProps.Jsii$Proxy) o;
if (this.cpu != null ? !this.cpu.equals(that.cpu) : that.cpu != null) return false;
if (this.ephemeralStorageGiB != null ? !this.ephemeralStorageGiB.equals(that.ephemeralStorageGiB) : that.ephemeralStorageGiB != null) return false;
if (this.memoryLimitMiB != null ? !this.memoryLimitMiB.equals(that.memoryLimitMiB) : that.memoryLimitMiB != null) return false;
if (this.runtimePlatform != null ? !this.runtimePlatform.equals(that.runtimePlatform) : that.runtimePlatform != null) return false;
if (this.executionRole != null ? !this.executionRole.equals(that.executionRole) : that.executionRole != null) return false;
if (this.family != null ? !this.family.equals(that.family) : that.family != null) return false;
if (this.proxyConfiguration != null ? !this.proxyConfiguration.equals(that.proxyConfiguration) : that.proxyConfiguration != null) return false;
if (this.taskRole != null ? !this.taskRole.equals(that.taskRole) : that.taskRole != null) return false;
return this.volumes != null ? this.volumes.equals(that.volumes) : that.volumes == null;
}
@Override
public final int hashCode() {
int result = this.cpu != null ? this.cpu.hashCode() : 0;
result = 31 * result + (this.ephemeralStorageGiB != null ? this.ephemeralStorageGiB.hashCode() : 0);
result = 31 * result + (this.memoryLimitMiB != null ? this.memoryLimitMiB.hashCode() : 0);
result = 31 * result + (this.runtimePlatform != null ? this.runtimePlatform.hashCode() : 0);
result = 31 * result + (this.executionRole != null ? this.executionRole.hashCode() : 0);
result = 31 * result + (this.family != null ? this.family.hashCode() : 0);
result = 31 * result + (this.proxyConfiguration != null ? this.proxyConfiguration.hashCode() : 0);
result = 31 * result + (this.taskRole != null ? this.taskRole.hashCode() : 0);
result = 31 * result + (this.volumes != null ? this.volumes.hashCode() : 0);
return result;
}
}
}