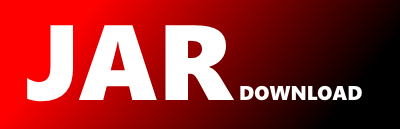
software.amazon.awscdk.services.ecs.GelfLogDriverProps Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* Specifies the journald log driver configuration options.
*
* Source
*
* Example:
*
*
* // Create a Task Definition for the container to start
* Ec2TaskDefinition taskDefinition = new Ec2TaskDefinition(this, "TaskDef");
* taskDefinition.addContainer("TheContainer", ContainerDefinitionOptions.builder()
* .image(ContainerImage.fromRegistry("example-image"))
* .memoryLimitMiB(256)
* .logging(LogDrivers.gelf(GelfLogDriverProps.builder().address("my-gelf-address").build()))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-28T21:34:28.949Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.GelfLogDriverProps")
@software.amazon.jsii.Jsii.Proxy(GelfLogDriverProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface GelfLogDriverProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.BaseLogDriverProps {
/**
* The address of the GELF server.
*
* tcp and udp are the only supported URI
* specifier and you must specify the port.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAddress();
/**
* UDP Only The level of compression when gzip or zlib is the gelf-compression-type.
*
* An integer in the range of -1 to 9 (BestCompression). Higher levels provide more
* compression at lower speed. Either -1 or 0 disables compression.
*
* Default: - 1
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getCompressionLevel() {
return null;
}
/**
* UDP Only The type of compression the GELF driver uses to compress each log message.
*
* Allowed values are gzip, zlib and none.
*
* Default: - gzip
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.GelfCompressionType getCompressionType() {
return null;
}
/**
* TCP Only The maximum number of reconnection attempts when the connection drop.
*
* A positive integer.
*
* Default: - 3
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getTcpMaxReconnect() {
return null;
}
/**
* TCP Only The number of seconds to wait between reconnection attempts.
*
* A positive integer.
*
* Default: - 1
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getTcpReconnectDelay() {
return null;
}
/**
* @return a {@link Builder} of {@link GelfLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link GelfLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String address;
java.lang.Number compressionLevel;
software.amazon.awscdk.services.ecs.GelfCompressionType compressionType;
java.lang.Number tcpMaxReconnect;
software.amazon.awscdk.core.Duration tcpReconnectDelay;
java.util.List env;
java.lang.String envRegex;
java.util.List labels;
java.lang.String tag;
/**
* Sets the value of {@link GelfLogDriverProps#getAddress}
* @param address The address of the GELF server. This parameter is required.
* tcp and udp are the only supported URI
* specifier and you must specify the port.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder address(java.lang.String address) {
this.address = address;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getCompressionLevel}
* @param compressionLevel UDP Only The level of compression when gzip or zlib is the gelf-compression-type.
* An integer in the range of -1 to 9 (BestCompression). Higher levels provide more
* compression at lower speed. Either -1 or 0 disables compression.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compressionLevel(java.lang.Number compressionLevel) {
this.compressionLevel = compressionLevel;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getCompressionType}
* @param compressionType UDP Only The type of compression the GELF driver uses to compress each log message.
* Allowed values are gzip, zlib and none.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compressionType(software.amazon.awscdk.services.ecs.GelfCompressionType compressionType) {
this.compressionType = compressionType;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getTcpMaxReconnect}
* @param tcpMaxReconnect TCP Only The maximum number of reconnection attempts when the connection drop.
* A positive integer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tcpMaxReconnect(java.lang.Number tcpMaxReconnect) {
this.tcpMaxReconnect = tcpMaxReconnect;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getTcpReconnectDelay}
* @param tcpReconnectDelay TCP Only The number of seconds to wait between reconnection attempts.
* A positive integer.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tcpReconnectDelay(software.amazon.awscdk.core.Duration tcpReconnectDelay) {
this.tcpReconnectDelay = tcpReconnectDelay;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getEnv}
* @param env The env option takes an array of keys.
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(java.util.List env) {
this.env = env;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getEnvRegex}
* @param envRegex The env-regex option is similar to and compatible with env.
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(java.lang.String envRegex) {
this.envRegex = envRegex;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getLabels}
* @param labels The labels option takes an array of keys.
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(java.util.List labels) {
this.labels = labels;
return this;
}
/**
* Sets the value of {@link GelfLogDriverProps#getTag}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages.
* Refer to the log tag option documentation for customizing the
* log tag format.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(java.lang.String tag) {
this.tag = tag;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link GelfLogDriverProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public GelfLogDriverProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link GelfLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements GelfLogDriverProps {
private final java.lang.String address;
private final java.lang.Number compressionLevel;
private final software.amazon.awscdk.services.ecs.GelfCompressionType compressionType;
private final java.lang.Number tcpMaxReconnect;
private final software.amazon.awscdk.core.Duration tcpReconnectDelay;
private final java.util.List env;
private final java.lang.String envRegex;
private final java.util.List labels;
private final java.lang.String tag;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.address = software.amazon.jsii.Kernel.get(this, "address", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.compressionLevel = software.amazon.jsii.Kernel.get(this, "compressionLevel", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.compressionType = software.amazon.jsii.Kernel.get(this, "compressionType", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.GelfCompressionType.class));
this.tcpMaxReconnect = software.amazon.jsii.Kernel.get(this, "tcpMaxReconnect", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.tcpReconnectDelay = software.amazon.jsii.Kernel.get(this, "tcpReconnectDelay", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.env = software.amazon.jsii.Kernel.get(this, "env", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.envRegex = software.amazon.jsii.Kernel.get(this, "envRegex", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.labels = software.amazon.jsii.Kernel.get(this, "labels", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.tag = software.amazon.jsii.Kernel.get(this, "tag", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.address = java.util.Objects.requireNonNull(builder.address, "address is required");
this.compressionLevel = builder.compressionLevel;
this.compressionType = builder.compressionType;
this.tcpMaxReconnect = builder.tcpMaxReconnect;
this.tcpReconnectDelay = builder.tcpReconnectDelay;
this.env = builder.env;
this.envRegex = builder.envRegex;
this.labels = builder.labels;
this.tag = builder.tag;
}
@Override
public final java.lang.String getAddress() {
return this.address;
}
@Override
public final java.lang.Number getCompressionLevel() {
return this.compressionLevel;
}
@Override
public final software.amazon.awscdk.services.ecs.GelfCompressionType getCompressionType() {
return this.compressionType;
}
@Override
public final java.lang.Number getTcpMaxReconnect() {
return this.tcpMaxReconnect;
}
@Override
public final software.amazon.awscdk.core.Duration getTcpReconnectDelay() {
return this.tcpReconnectDelay;
}
@Override
public final java.util.List getEnv() {
return this.env;
}
@Override
public final java.lang.String getEnvRegex() {
return this.envRegex;
}
@Override
public final java.util.List getLabels() {
return this.labels;
}
@Override
public final java.lang.String getTag() {
return this.tag;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("address", om.valueToTree(this.getAddress()));
if (this.getCompressionLevel() != null) {
data.set("compressionLevel", om.valueToTree(this.getCompressionLevel()));
}
if (this.getCompressionType() != null) {
data.set("compressionType", om.valueToTree(this.getCompressionType()));
}
if (this.getTcpMaxReconnect() != null) {
data.set("tcpMaxReconnect", om.valueToTree(this.getTcpMaxReconnect()));
}
if (this.getTcpReconnectDelay() != null) {
data.set("tcpReconnectDelay", om.valueToTree(this.getTcpReconnectDelay()));
}
if (this.getEnv() != null) {
data.set("env", om.valueToTree(this.getEnv()));
}
if (this.getEnvRegex() != null) {
data.set("envRegex", om.valueToTree(this.getEnvRegex()));
}
if (this.getLabels() != null) {
data.set("labels", om.valueToTree(this.getLabels()));
}
if (this.getTag() != null) {
data.set("tag", om.valueToTree(this.getTag()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.GelfLogDriverProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GelfLogDriverProps.Jsii$Proxy that = (GelfLogDriverProps.Jsii$Proxy) o;
if (!address.equals(that.address)) return false;
if (this.compressionLevel != null ? !this.compressionLevel.equals(that.compressionLevel) : that.compressionLevel != null) return false;
if (this.compressionType != null ? !this.compressionType.equals(that.compressionType) : that.compressionType != null) return false;
if (this.tcpMaxReconnect != null ? !this.tcpMaxReconnect.equals(that.tcpMaxReconnect) : that.tcpMaxReconnect != null) return false;
if (this.tcpReconnectDelay != null ? !this.tcpReconnectDelay.equals(that.tcpReconnectDelay) : that.tcpReconnectDelay != null) return false;
if (this.env != null ? !this.env.equals(that.env) : that.env != null) return false;
if (this.envRegex != null ? !this.envRegex.equals(that.envRegex) : that.envRegex != null) return false;
if (this.labels != null ? !this.labels.equals(that.labels) : that.labels != null) return false;
return this.tag != null ? this.tag.equals(that.tag) : that.tag == null;
}
@Override
public final int hashCode() {
int result = this.address.hashCode();
result = 31 * result + (this.compressionLevel != null ? this.compressionLevel.hashCode() : 0);
result = 31 * result + (this.compressionType != null ? this.compressionType.hashCode() : 0);
result = 31 * result + (this.tcpMaxReconnect != null ? this.tcpMaxReconnect.hashCode() : 0);
result = 31 * result + (this.tcpReconnectDelay != null ? this.tcpReconnectDelay.hashCode() : 0);
result = 31 * result + (this.env != null ? this.env.hashCode() : 0);
result = 31 * result + (this.envRegex != null ? this.envRegex.hashCode() : 0);
result = 31 * result + (this.labels != null ? this.labels.hashCode() : 0);
result = 31 * result + (this.tag != null ? this.tag.hashCode() : 0);
return result;
}
}
}