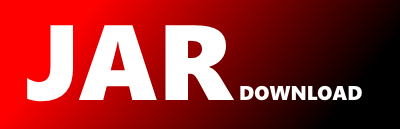
software.amazon.awscdk.services.ecs.GenericLogDriver Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A log driver that sends logs to the specified driver.
*
* Example:
*
*
* // Create a Task Definition for the container to start
* Ec2TaskDefinition taskDefinition = new Ec2TaskDefinition(this, "TaskDef");
* taskDefinition.addContainer("TheContainer", ContainerDefinitionOptions.builder()
* .image(ContainerImage.fromRegistry("example-image"))
* .memoryLimitMiB(256)
* .logging(GenericLogDriver.Builder.create()
* .logDriver("fluentd")
* .options(Map.of(
* "tag", "example-tag"))
* .build())
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-28T21:34:28.951Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.GenericLogDriver")
public class GenericLogDriver extends software.amazon.awscdk.services.ecs.LogDriver {
protected GenericLogDriver(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected GenericLogDriver(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the GenericLogDriver class.
*
* @param props the generic log driver configuration options. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public GenericLogDriver(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.GenericLogDriverProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Called when the log driver is configured on a container.
*
* @param _scope This parameter is required.
* @param _containerDefinition This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.LogDriverConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.ContainerDefinition _containerDefinition) {
return software.amazon.jsii.Kernel.call(this, "bind", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.LogDriverConfig.class), new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_containerDefinition, "_containerDefinition is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.GenericLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.ecs.GenericLogDriverProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.ecs.GenericLogDriverProps.Builder();
}
/**
* The log driver to use for the container.
*
* The valid values listed for this parameter are log drivers
* that the Amazon ECS container agent can communicate with by default.
*
* For tasks using the Fargate launch type, the supported log drivers are awslogs and splunk.
* For tasks using the EC2 launch type, the supported log drivers are awslogs, syslog, gelf, fluentd, splunk, journald, and json-file.
*
* For more information about using the awslogs log driver, see
* Using the awslogs Log Driver
* in the Amazon Elastic Container Service Developer Guide.
*
* @return {@code this}
* @param logDriver The log driver to use for the container. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logDriver(final java.lang.String logDriver) {
this.props.logDriver(logDriver);
return this;
}
/**
* The configuration options to send to the log driver.
*
* Default: - the log driver options.
*
* @return {@code this}
* @param options The configuration options to send to the log driver. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder options(final java.util.Map options) {
this.props.options(options);
return this;
}
/**
* The secrets to pass to the log configuration.
*
* Default: - no secret options provided.
*
* @return {@code this}
* @param secretOptions The secrets to pass to the log configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secretOptions(final java.util.Map secretOptions) {
this.props.secretOptions(secretOptions);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.GenericLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.GenericLogDriver build() {
return new software.amazon.awscdk.services.ecs.GenericLogDriver(
this.props.build()
);
}
}
}