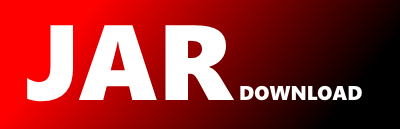
software.amazon.awscdk.services.ecs.SplunkLogDriver Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A log driver that sends log information to splunk Logs.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.9.0 (build 5c646d5)", date = "2020-07-28T21:12:44.632Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.SplunkLogDriver")
public class SplunkLogDriver extends software.amazon.awscdk.services.ecs.LogDriver {
protected SplunkLogDriver(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected SplunkLogDriver(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the SplunkLogDriver class.
*
* @param props the splunk log driver configuration options. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public SplunkLogDriver(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.SplunkLogDriverProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Called when the log driver is configured on a container.
*
* @param _scope This parameter is required.
* @param _containerDefinition This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.LogDriverConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.ContainerDefinition _containerDefinition) {
return this.jsiiCall("bind", software.amazon.awscdk.services.ecs.LogDriverConfig.class, new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_containerDefinition, "_containerDefinition is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.SplunkLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.ecs.SplunkLogDriverProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.ecs.SplunkLogDriverProps.Builder();
}
/**
* The env option takes an array of keys.
*
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
*
* Default: - No env
*
* @return {@code this}
* @param env The env option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(final java.util.List env) {
this.props.env(env);
return this;
}
/**
* The env-regex option is similar to and compatible with env.
*
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
*
* Default: - No envRegex
*
* @return {@code this}
* @param envRegex The env-regex option is similar to and compatible with env. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(final java.lang.String envRegex) {
this.props.envRegex(envRegex);
return this;
}
/**
* The labels option takes an array of keys.
*
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
*
* Default: - No labels
*
* @return {@code this}
* @param labels The labels option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(final java.util.List labels) {
this.props.labels(labels);
return this;
}
/**
* By default, Docker uses the first 12 characters of the container ID to tag log messages.
*
* Refer to the log tag option documentation for customizing the
* log tag format.
*
* Default: - The first 12 characters of the container ID
*
* @return {@code this}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(final java.lang.String tag) {
this.props.tag(tag);
return this;
}
/**
* Splunk HTTP Event Collector token.
*
* @return {@code this}
* @param token Splunk HTTP Event Collector token. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder token(final software.amazon.awscdk.core.SecretValue token) {
this.props.token(token);
return this;
}
/**
* Path to your Splunk Enterprise, self-service Splunk Cloud instance, or Splunk Cloud managed cluster (including port and scheme used by HTTP Event Collector) in one of the following formats: https://your_splunk_instance:8088 or https://input-prd-p-XXXXXXX.cloud.splunk.com:8088 or https://http-inputs-XXXXXXXX.splunkcloud.com.
*
* @return {@code this}
* @param url Path to your Splunk Enterprise, self-service Splunk Cloud instance, or Splunk Cloud managed cluster (including port and scheme used by HTTP Event Collector) in one of the following formats: https://your_splunk_instance:8088 or https://input-prd-p-XXXXXXX.cloud.splunk.com:8088 or https://http-inputs-XXXXXXXX.splunkcloud.com. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder url(final java.lang.String url) {
this.props.url(url);
return this;
}
/**
* Name to use for validating server certificate.
*
* Default: - The hostname of the splunk-url
*
* @return {@code this}
* @param caName Name to use for validating server certificate. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caName(final java.lang.String caName) {
this.props.caName(caName);
return this;
}
/**
* Path to root certificate.
*
* Default: - caPath not set.
*
* @return {@code this}
* @param caPath Path to root certificate. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caPath(final java.lang.String caPath) {
this.props.caPath(caPath);
return this;
}
/**
* Message format.
*
* Can be inline, json or raw.
*
* Default: - inline
*
* @return {@code this}
* @param format Message format. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder format(final software.amazon.awscdk.services.ecs.SplunkLogFormat format) {
this.props.format(format);
return this;
}
/**
* Enable/disable gzip compression to send events to Splunk Enterprise or Splunk Cloud instance.
*
* Default: - false
*
* @return {@code this}
* @param gzip Enable/disable gzip compression to send events to Splunk Enterprise or Splunk Cloud instance. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gzip(final java.lang.Boolean gzip) {
this.props.gzip(gzip);
return this;
}
/**
* Set compression level for gzip.
*
* Valid values are -1 (default), 0 (no compression),
* 1 (best speed) ... 9 (best compression).
*
* Default: - -1 (Default Compression)
*
* @return {@code this}
* @param gzipLevel Set compression level for gzip. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gzipLevel(final java.lang.Number gzipLevel) {
this.props.gzipLevel(gzipLevel);
return this;
}
/**
* Event index.
*
* Default: - index not set.
*
* @return {@code this}
* @param index Event index. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder index(final java.lang.String index) {
this.props.index(index);
return this;
}
/**
* Ignore server certificate validation.
*
* Default: - insecureSkipVerify not set.
*
* @return {@code this}
* @param insecureSkipVerify Ignore server certificate validation. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder insecureSkipVerify(final java.lang.String insecureSkipVerify) {
this.props.insecureSkipVerify(insecureSkipVerify);
return this;
}
/**
* Event source.
*
* Default: - source not set.
*
* @return {@code this}
* @param source Event source. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder source(final java.lang.String source) {
this.props.source(source);
return this;
}
/**
* Event source type.
*
* Default: - sourceType not set.
*
* @return {@code this}
* @param sourceType Event source type. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceType(final java.lang.String sourceType) {
this.props.sourceType(sourceType);
return this;
}
/**
* Verify on start, that docker can connect to Splunk server.
*
* Default: - true
*
* @return {@code this}
* @param verifyConnection Verify on start, that docker can connect to Splunk server. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder verifyConnection(final java.lang.Boolean verifyConnection) {
this.props.verifyConnection(verifyConnection);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.SplunkLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.SplunkLogDriver build() {
return new software.amazon.awscdk.services.ecs.SplunkLogDriver(
this.props.build()
);
}
}
}