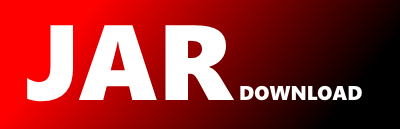
software.amazon.awscdk.services.ecs.CfnService Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A CloudFormation `AWS::ECS::Service`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2021-01-06T15:18:00.548Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService")
public class CfnService extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnService(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnService(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.ecs.CfnService.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::ECS::Service`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnService(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.CfnServiceProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* Create a new `AWS::ECS::Service`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnService(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* (experimental) Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrName() {
return software.amazon.jsii.Kernel.get(this, "attrName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* `AWS::ECS::Service.Tags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-tags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* `AWS::ECS::Service.CapacityProviderStrategy`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-capacityproviderstrategy
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getCapacityProviderStrategy() {
return software.amazon.jsii.Kernel.get(this, "capacityProviderStrategy", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.CapacityProviderStrategy`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-capacityproviderstrategy
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCapacityProviderStrategy(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "capacityProviderStrategy", value);
}
/**
* `AWS::ECS::Service.CapacityProviderStrategy`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-capacityproviderstrategy
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCapacityProviderStrategy(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "capacityProviderStrategy", value);
}
/**
* `AWS::ECS::Service.Cluster`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-cluster
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getCluster() {
return software.amazon.jsii.Kernel.get(this, "cluster", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.Cluster`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-cluster
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setCluster(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "cluster", value);
}
/**
* `AWS::ECS::Service.DeploymentConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getDeploymentConfiguration() {
return software.amazon.jsii.Kernel.get(this, "deploymentConfiguration", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.DeploymentConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDeploymentConfiguration(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "deploymentConfiguration", value);
}
/**
* `AWS::ECS::Service.DeploymentConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDeploymentConfiguration(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.CfnService.DeploymentConfigurationProperty value) {
software.amazon.jsii.Kernel.set(this, "deploymentConfiguration", value);
}
/**
* `AWS::ECS::Service.DeploymentController`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentcontroller
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getDeploymentController() {
return software.amazon.jsii.Kernel.get(this, "deploymentController", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.DeploymentController`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentcontroller
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDeploymentController(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "deploymentController", value);
}
/**
* `AWS::ECS::Service.DeploymentController`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentcontroller
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDeploymentController(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.CfnService.DeploymentControllerProperty value) {
software.amazon.jsii.Kernel.set(this, "deploymentController", value);
}
/**
* `AWS::ECS::Service.DesiredCount`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-desiredcount
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Number getDesiredCount() {
return software.amazon.jsii.Kernel.get(this, "desiredCount", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* `AWS::ECS::Service.DesiredCount`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-desiredcount
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDesiredCount(final @org.jetbrains.annotations.Nullable java.lang.Number value) {
software.amazon.jsii.Kernel.set(this, "desiredCount", value);
}
/**
* `AWS::ECS::Service.EnableECSManagedTags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-enableecsmanagedtags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getEnableEcsManagedTags() {
return software.amazon.jsii.Kernel.get(this, "enableEcsManagedTags", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.EnableECSManagedTags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-enableecsmanagedtags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEnableEcsManagedTags(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "enableEcsManagedTags", value);
}
/**
* `AWS::ECS::Service.EnableECSManagedTags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-enableecsmanagedtags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEnableEcsManagedTags(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "enableEcsManagedTags", value);
}
/**
* `AWS::ECS::Service.HealthCheckGracePeriodSeconds`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-healthcheckgraceperiodseconds
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Number getHealthCheckGracePeriodSeconds() {
return software.amazon.jsii.Kernel.get(this, "healthCheckGracePeriodSeconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* `AWS::ECS::Service.HealthCheckGracePeriodSeconds`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-healthcheckgraceperiodseconds
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setHealthCheckGracePeriodSeconds(final @org.jetbrains.annotations.Nullable java.lang.Number value) {
software.amazon.jsii.Kernel.set(this, "healthCheckGracePeriodSeconds", value);
}
/**
* `AWS::ECS::Service.LaunchType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-launchtype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getLaunchType() {
return software.amazon.jsii.Kernel.get(this, "launchType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.LaunchType`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-launchtype
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLaunchType(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "launchType", value);
}
/**
* `AWS::ECS::Service.LoadBalancers`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-loadbalancers
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getLoadBalancers() {
return software.amazon.jsii.Kernel.get(this, "loadBalancers", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.LoadBalancers`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-loadbalancers
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLoadBalancers(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "loadBalancers", value);
}
/**
* `AWS::ECS::Service.LoadBalancers`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-loadbalancers
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLoadBalancers(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "loadBalancers", value);
}
/**
* `AWS::ECS::Service.NetworkConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-networkconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getNetworkConfiguration() {
return software.amazon.jsii.Kernel.get(this, "networkConfiguration", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.NetworkConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-networkconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setNetworkConfiguration(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "networkConfiguration", value);
}
/**
* `AWS::ECS::Service.NetworkConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-networkconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setNetworkConfiguration(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.CfnService.NetworkConfigurationProperty value) {
software.amazon.jsii.Kernel.set(this, "networkConfiguration", value);
}
/**
* `AWS::ECS::Service.PlacementConstraints`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementconstraints
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getPlacementConstraints() {
return software.amazon.jsii.Kernel.get(this, "placementConstraints", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.PlacementConstraints`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementconstraints
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementConstraints(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "placementConstraints", value);
}
/**
* `AWS::ECS::Service.PlacementConstraints`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementconstraints
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementConstraints(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "placementConstraints", value);
}
/**
* `AWS::ECS::Service.PlacementStrategies`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementstrategies
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getPlacementStrategies() {
return software.amazon.jsii.Kernel.get(this, "placementStrategies", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.PlacementStrategies`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementstrategies
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementStrategies(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "placementStrategies", value);
}
/**
* `AWS::ECS::Service.PlacementStrategies`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementstrategies
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementStrategies(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "placementStrategies", value);
}
/**
* `AWS::ECS::Service.PlatformVersion`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-platformversion
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getPlatformVersion() {
return software.amazon.jsii.Kernel.get(this, "platformVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.PlatformVersion`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-platformversion
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlatformVersion(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "platformVersion", value);
}
/**
* `AWS::ECS::Service.PropagateTags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-propagatetags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getPropagateTags() {
return software.amazon.jsii.Kernel.get(this, "propagateTags", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.PropagateTags`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-propagatetags
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPropagateTags(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "propagateTags", value);
}
/**
* `AWS::ECS::Service.Role`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-role
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getRole() {
return software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.Role`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-role
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setRole(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "role", value);
}
/**
* `AWS::ECS::Service.SchedulingStrategy`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-schedulingstrategy
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getSchedulingStrategy() {
return software.amazon.jsii.Kernel.get(this, "schedulingStrategy", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.SchedulingStrategy`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-schedulingstrategy
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSchedulingStrategy(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "schedulingStrategy", value);
}
/**
* `AWS::ECS::Service.ServiceArn`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicearn
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getServiceArn() {
return software.amazon.jsii.Kernel.get(this, "serviceArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.ServiceArn`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicearn
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setServiceArn(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "serviceArn", value);
}
/**
* `AWS::ECS::Service.ServiceName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicename
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getServiceName() {
return software.amazon.jsii.Kernel.get(this, "serviceName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.ServiceName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicename
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setServiceName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "serviceName", value);
}
/**
* `AWS::ECS::Service.ServiceRegistries`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-serviceregistries
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getServiceRegistries() {
return software.amazon.jsii.Kernel.get(this, "serviceRegistries", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* `AWS::ECS::Service.ServiceRegistries`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-serviceregistries
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setServiceRegistries(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "serviceRegistries", value);
}
/**
* `AWS::ECS::Service.ServiceRegistries`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-serviceregistries
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setServiceRegistries(final @org.jetbrains.annotations.Nullable java.util.List value) {
software.amazon.jsii.Kernel.set(this, "serviceRegistries", value);
}
/**
* `AWS::ECS::Service.TaskDefinition`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-taskdefinition
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getTaskDefinition() {
return software.amazon.jsii.Kernel.get(this, "taskDefinition", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* `AWS::ECS::Service.TaskDefinition`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-taskdefinition
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setTaskDefinition(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "taskDefinition", value);
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-awsvpcconfiguration.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.AwsVpcConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(AwsVpcConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface AwsVpcConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.AwsVpcConfigurationProperty.Subnets`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-awsvpcconfiguration.html#cfn-ecs-service-awsvpcconfiguration-subnets
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getSubnets();
/**
* `CfnService.AwsVpcConfigurationProperty.AssignPublicIp`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-awsvpcconfiguration.html#cfn-ecs-service-awsvpcconfiguration-assignpublicip
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAssignPublicIp() {
return null;
}
/**
* `CfnService.AwsVpcConfigurationProperty.SecurityGroups`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-awsvpcconfiguration.html#cfn-ecs-service-awsvpcconfiguration-securitygroups
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSecurityGroups() {
return null;
}
/**
* @return a {@link Builder} of {@link AwsVpcConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AwsVpcConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.util.List subnets;
private java.lang.String assignPublicIp;
private java.util.List securityGroups;
/**
* Sets the value of {@link AwsVpcConfigurationProperty#getSubnets}
* @param subnets `CfnService.AwsVpcConfigurationProperty.Subnets`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnets(java.util.List subnets) {
this.subnets = subnets;
return this;
}
/**
* Sets the value of {@link AwsVpcConfigurationProperty#getAssignPublicIp}
* @param assignPublicIp `CfnService.AwsVpcConfigurationProperty.AssignPublicIp`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder assignPublicIp(java.lang.String assignPublicIp) {
this.assignPublicIp = assignPublicIp;
return this;
}
/**
* Sets the value of {@link AwsVpcConfigurationProperty#getSecurityGroups}
* @param securityGroups `CfnService.AwsVpcConfigurationProperty.SecurityGroups`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityGroups(java.util.List securityGroups) {
this.securityGroups = securityGroups;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AwsVpcConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AwsVpcConfigurationProperty build() {
return new Jsii$Proxy(subnets, assignPublicIp, securityGroups);
}
}
/**
* An implementation for {@link AwsVpcConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AwsVpcConfigurationProperty {
private final java.util.List subnets;
private final java.lang.String assignPublicIp;
private final java.util.List securityGroups;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.subnets = software.amazon.jsii.Kernel.get(this, "subnets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.assignPublicIp = software.amazon.jsii.Kernel.get(this, "assignPublicIp", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.securityGroups = software.amazon.jsii.Kernel.get(this, "securityGroups", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.util.List subnets, final java.lang.String assignPublicIp, final java.util.List securityGroups) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.subnets = java.util.Objects.requireNonNull(subnets, "subnets is required");
this.assignPublicIp = assignPublicIp;
this.securityGroups = securityGroups;
}
@Override
public final java.util.List getSubnets() {
return this.subnets;
}
@Override
public final java.lang.String getAssignPublicIp() {
return this.assignPublicIp;
}
@Override
public final java.util.List getSecurityGroups() {
return this.securityGroups;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("subnets", om.valueToTree(this.getSubnets()));
if (this.getAssignPublicIp() != null) {
data.set("assignPublicIp", om.valueToTree(this.getAssignPublicIp()));
}
if (this.getSecurityGroups() != null) {
data.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.AwsVpcConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AwsVpcConfigurationProperty.Jsii$Proxy that = (AwsVpcConfigurationProperty.Jsii$Proxy) o;
if (!subnets.equals(that.subnets)) return false;
if (this.assignPublicIp != null ? !this.assignPublicIp.equals(that.assignPublicIp) : that.assignPublicIp != null) return false;
return this.securityGroups != null ? this.securityGroups.equals(that.securityGroups) : that.securityGroups == null;
}
@Override
public final int hashCode() {
int result = this.subnets.hashCode();
result = 31 * result + (this.assignPublicIp != null ? this.assignPublicIp.hashCode() : 0);
result = 31 * result + (this.securityGroups != null ? this.securityGroups.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-capacityproviderstrategyitem.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.CapacityProviderStrategyItemProperty")
@software.amazon.jsii.Jsii.Proxy(CapacityProviderStrategyItemProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface CapacityProviderStrategyItemProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.CapacityProviderStrategyItemProperty.Base`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-capacityproviderstrategyitem.html#cfn-ecs-service-capacityproviderstrategyitem-base
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getBase() {
return null;
}
/**
* `CfnService.CapacityProviderStrategyItemProperty.CapacityProvider`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-capacityproviderstrategyitem.html#cfn-ecs-service-capacityproviderstrategyitem-capacityprovider
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCapacityProvider() {
return null;
}
/**
* `CfnService.CapacityProviderStrategyItemProperty.Weight`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-capacityproviderstrategyitem.html#cfn-ecs-service-capacityproviderstrategyitem-weight
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getWeight() {
return null;
}
/**
* @return a {@link Builder} of {@link CapacityProviderStrategyItemProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CapacityProviderStrategyItemProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Number base;
private java.lang.String capacityProvider;
private java.lang.Number weight;
/**
* Sets the value of {@link CapacityProviderStrategyItemProperty#getBase}
* @param base `CfnService.CapacityProviderStrategyItemProperty.Base`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder base(java.lang.Number base) {
this.base = base;
return this;
}
/**
* Sets the value of {@link CapacityProviderStrategyItemProperty#getCapacityProvider}
* @param capacityProvider `CfnService.CapacityProviderStrategyItemProperty.CapacityProvider`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder capacityProvider(java.lang.String capacityProvider) {
this.capacityProvider = capacityProvider;
return this;
}
/**
* Sets the value of {@link CapacityProviderStrategyItemProperty#getWeight}
* @param weight `CfnService.CapacityProviderStrategyItemProperty.Weight`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder weight(java.lang.Number weight) {
this.weight = weight;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CapacityProviderStrategyItemProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CapacityProviderStrategyItemProperty build() {
return new Jsii$Proxy(base, capacityProvider, weight);
}
}
/**
* An implementation for {@link CapacityProviderStrategyItemProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CapacityProviderStrategyItemProperty {
private final java.lang.Number base;
private final java.lang.String capacityProvider;
private final java.lang.Number weight;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.base = software.amazon.jsii.Kernel.get(this, "base", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.capacityProvider = software.amazon.jsii.Kernel.get(this, "capacityProvider", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.weight = software.amazon.jsii.Kernel.get(this, "weight", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Number base, final java.lang.String capacityProvider, final java.lang.Number weight) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.base = base;
this.capacityProvider = capacityProvider;
this.weight = weight;
}
@Override
public final java.lang.Number getBase() {
return this.base;
}
@Override
public final java.lang.String getCapacityProvider() {
return this.capacityProvider;
}
@Override
public final java.lang.Number getWeight() {
return this.weight;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getBase() != null) {
data.set("base", om.valueToTree(this.getBase()));
}
if (this.getCapacityProvider() != null) {
data.set("capacityProvider", om.valueToTree(this.getCapacityProvider()));
}
if (this.getWeight() != null) {
data.set("weight", om.valueToTree(this.getWeight()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.CapacityProviderStrategyItemProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CapacityProviderStrategyItemProperty.Jsii$Proxy that = (CapacityProviderStrategyItemProperty.Jsii$Proxy) o;
if (this.base != null ? !this.base.equals(that.base) : that.base != null) return false;
if (this.capacityProvider != null ? !this.capacityProvider.equals(that.capacityProvider) : that.capacityProvider != null) return false;
return this.weight != null ? this.weight.equals(that.weight) : that.weight == null;
}
@Override
public final int hashCode() {
int result = this.base != null ? this.base.hashCode() : 0;
result = 31 * result + (this.capacityProvider != null ? this.capacityProvider.hashCode() : 0);
result = 31 * result + (this.weight != null ? this.weight.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentcircuitbreaker.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.DeploymentCircuitBreakerProperty")
@software.amazon.jsii.Jsii.Proxy(DeploymentCircuitBreakerProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DeploymentCircuitBreakerProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.DeploymentCircuitBreakerProperty.Enable`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentcircuitbreaker.html#cfn-ecs-service-deploymentcircuitbreaker-enable
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getEnable();
/**
* `CfnService.DeploymentCircuitBreakerProperty.Rollback`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentcircuitbreaker.html#cfn-ecs-service-deploymentcircuitbreaker-rollback
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getRollback();
/**
* @return a {@link Builder} of {@link DeploymentCircuitBreakerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DeploymentCircuitBreakerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Object enable;
private java.lang.Object rollback;
/**
* Sets the value of {@link DeploymentCircuitBreakerProperty#getEnable}
* @param enable `CfnService.DeploymentCircuitBreakerProperty.Enable`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enable(java.lang.Boolean enable) {
this.enable = enable;
return this;
}
/**
* Sets the value of {@link DeploymentCircuitBreakerProperty#getEnable}
* @param enable `CfnService.DeploymentCircuitBreakerProperty.Enable`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enable(software.amazon.awscdk.core.IResolvable enable) {
this.enable = enable;
return this;
}
/**
* Sets the value of {@link DeploymentCircuitBreakerProperty#getRollback}
* @param rollback `CfnService.DeploymentCircuitBreakerProperty.Rollback`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder rollback(java.lang.Boolean rollback) {
this.rollback = rollback;
return this;
}
/**
* Sets the value of {@link DeploymentCircuitBreakerProperty#getRollback}
* @param rollback `CfnService.DeploymentCircuitBreakerProperty.Rollback`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder rollback(software.amazon.awscdk.core.IResolvable rollback) {
this.rollback = rollback;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DeploymentCircuitBreakerProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DeploymentCircuitBreakerProperty build() {
return new Jsii$Proxy(enable, rollback);
}
}
/**
* An implementation for {@link DeploymentCircuitBreakerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DeploymentCircuitBreakerProperty {
private final java.lang.Object enable;
private final java.lang.Object rollback;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.enable = software.amazon.jsii.Kernel.get(this, "enable", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.rollback = software.amazon.jsii.Kernel.get(this, "rollback", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Object enable, final java.lang.Object rollback) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.enable = java.util.Objects.requireNonNull(enable, "enable is required");
this.rollback = java.util.Objects.requireNonNull(rollback, "rollback is required");
}
@Override
public final java.lang.Object getEnable() {
return this.enable;
}
@Override
public final java.lang.Object getRollback() {
return this.rollback;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("enable", om.valueToTree(this.getEnable()));
data.set("rollback", om.valueToTree(this.getRollback()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.DeploymentCircuitBreakerProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeploymentCircuitBreakerProperty.Jsii$Proxy that = (DeploymentCircuitBreakerProperty.Jsii$Proxy) o;
if (!enable.equals(that.enable)) return false;
return this.rollback.equals(that.rollback);
}
@Override
public final int hashCode() {
int result = this.enable.hashCode();
result = 31 * result + (this.rollback.hashCode());
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentconfiguration.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.DeploymentConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(DeploymentConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DeploymentConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.DeploymentConfigurationProperty.DeploymentCircuitBreaker`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentconfiguration.html#cfn-ecs-service-deploymentconfiguration-deploymentcircuitbreaker
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDeploymentCircuitBreaker() {
return null;
}
/**
* `CfnService.DeploymentConfigurationProperty.MaximumPercent`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentconfiguration.html#cfn-ecs-service-deploymentconfiguration-maximumpercent
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getMaximumPercent() {
return null;
}
/**
* `CfnService.DeploymentConfigurationProperty.MinimumHealthyPercent`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentconfiguration.html#cfn-ecs-service-deploymentconfiguration-minimumhealthypercent
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getMinimumHealthyPercent() {
return null;
}
/**
* @return a {@link Builder} of {@link DeploymentConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DeploymentConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Object deploymentCircuitBreaker;
private java.lang.Number maximumPercent;
private java.lang.Number minimumHealthyPercent;
/**
* Sets the value of {@link DeploymentConfigurationProperty#getDeploymentCircuitBreaker}
* @param deploymentCircuitBreaker `CfnService.DeploymentConfigurationProperty.DeploymentCircuitBreaker`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentCircuitBreaker(software.amazon.awscdk.core.IResolvable deploymentCircuitBreaker) {
this.deploymentCircuitBreaker = deploymentCircuitBreaker;
return this;
}
/**
* Sets the value of {@link DeploymentConfigurationProperty#getDeploymentCircuitBreaker}
* @param deploymentCircuitBreaker `CfnService.DeploymentConfigurationProperty.DeploymentCircuitBreaker`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentCircuitBreaker(software.amazon.awscdk.services.ecs.CfnService.DeploymentCircuitBreakerProperty deploymentCircuitBreaker) {
this.deploymentCircuitBreaker = deploymentCircuitBreaker;
return this;
}
/**
* Sets the value of {@link DeploymentConfigurationProperty#getMaximumPercent}
* @param maximumPercent `CfnService.DeploymentConfigurationProperty.MaximumPercent`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maximumPercent(java.lang.Number maximumPercent) {
this.maximumPercent = maximumPercent;
return this;
}
/**
* Sets the value of {@link DeploymentConfigurationProperty#getMinimumHealthyPercent}
* @param minimumHealthyPercent `CfnService.DeploymentConfigurationProperty.MinimumHealthyPercent`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder minimumHealthyPercent(java.lang.Number minimumHealthyPercent) {
this.minimumHealthyPercent = minimumHealthyPercent;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DeploymentConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DeploymentConfigurationProperty build() {
return new Jsii$Proxy(deploymentCircuitBreaker, maximumPercent, minimumHealthyPercent);
}
}
/**
* An implementation for {@link DeploymentConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DeploymentConfigurationProperty {
private final java.lang.Object deploymentCircuitBreaker;
private final java.lang.Number maximumPercent;
private final java.lang.Number minimumHealthyPercent;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.deploymentCircuitBreaker = software.amazon.jsii.Kernel.get(this, "deploymentCircuitBreaker", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.maximumPercent = software.amazon.jsii.Kernel.get(this, "maximumPercent", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.minimumHealthyPercent = software.amazon.jsii.Kernel.get(this, "minimumHealthyPercent", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Object deploymentCircuitBreaker, final java.lang.Number maximumPercent, final java.lang.Number minimumHealthyPercent) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.deploymentCircuitBreaker = deploymentCircuitBreaker;
this.maximumPercent = maximumPercent;
this.minimumHealthyPercent = minimumHealthyPercent;
}
@Override
public final java.lang.Object getDeploymentCircuitBreaker() {
return this.deploymentCircuitBreaker;
}
@Override
public final java.lang.Number getMaximumPercent() {
return this.maximumPercent;
}
@Override
public final java.lang.Number getMinimumHealthyPercent() {
return this.minimumHealthyPercent;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDeploymentCircuitBreaker() != null) {
data.set("deploymentCircuitBreaker", om.valueToTree(this.getDeploymentCircuitBreaker()));
}
if (this.getMaximumPercent() != null) {
data.set("maximumPercent", om.valueToTree(this.getMaximumPercent()));
}
if (this.getMinimumHealthyPercent() != null) {
data.set("minimumHealthyPercent", om.valueToTree(this.getMinimumHealthyPercent()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.DeploymentConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeploymentConfigurationProperty.Jsii$Proxy that = (DeploymentConfigurationProperty.Jsii$Proxy) o;
if (this.deploymentCircuitBreaker != null ? !this.deploymentCircuitBreaker.equals(that.deploymentCircuitBreaker) : that.deploymentCircuitBreaker != null) return false;
if (this.maximumPercent != null ? !this.maximumPercent.equals(that.maximumPercent) : that.maximumPercent != null) return false;
return this.minimumHealthyPercent != null ? this.minimumHealthyPercent.equals(that.minimumHealthyPercent) : that.minimumHealthyPercent == null;
}
@Override
public final int hashCode() {
int result = this.deploymentCircuitBreaker != null ? this.deploymentCircuitBreaker.hashCode() : 0;
result = 31 * result + (this.maximumPercent != null ? this.maximumPercent.hashCode() : 0);
result = 31 * result + (this.minimumHealthyPercent != null ? this.minimumHealthyPercent.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentcontroller.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.DeploymentControllerProperty")
@software.amazon.jsii.Jsii.Proxy(DeploymentControllerProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DeploymentControllerProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.DeploymentControllerProperty.Type`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-deploymentcontroller.html#cfn-ecs-service-deploymentcontroller-type
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getType() {
return null;
}
/**
* @return a {@link Builder} of {@link DeploymentControllerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DeploymentControllerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String type;
/**
* Sets the value of {@link DeploymentControllerProperty#getType}
* @param type `CfnService.DeploymentControllerProperty.Type`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DeploymentControllerProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DeploymentControllerProperty build() {
return new Jsii$Proxy(type);
}
}
/**
* An implementation for {@link DeploymentControllerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DeploymentControllerProperty {
private final java.lang.String type;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String type) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.type = type;
}
@Override
public final java.lang.String getType() {
return this.type;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.DeploymentControllerProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeploymentControllerProperty.Jsii$Proxy that = (DeploymentControllerProperty.Jsii$Proxy) o;
return this.type != null ? this.type.equals(that.type) : that.type == null;
}
@Override
public final int hashCode() {
int result = this.type != null ? this.type.hashCode() : 0;
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-loadbalancer.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.LoadBalancerProperty")
@software.amazon.jsii.Jsii.Proxy(LoadBalancerProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface LoadBalancerProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.LoadBalancerProperty.ContainerPort`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-loadbalancer.html#cfn-ecs-service-loadbalancer-containerport
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getContainerPort();
/**
* `CfnService.LoadBalancerProperty.ContainerName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-loadbalancer.html#cfn-ecs-service-loadbalancer-containername
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getContainerName() {
return null;
}
/**
* `CfnService.LoadBalancerProperty.LoadBalancerName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-loadbalancer.html#cfn-ecs-service-loadbalancer-loadbalancername
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getLoadBalancerName() {
return null;
}
/**
* `CfnService.LoadBalancerProperty.TargetGroupArn`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-loadbalancer.html#cfn-ecs-service-loadbalancer-targetgrouparn
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTargetGroupArn() {
return null;
}
/**
* @return a {@link Builder} of {@link LoadBalancerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link LoadBalancerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Number containerPort;
private java.lang.String containerName;
private java.lang.String loadBalancerName;
private java.lang.String targetGroupArn;
/**
* Sets the value of {@link LoadBalancerProperty#getContainerPort}
* @param containerPort `CfnService.LoadBalancerProperty.ContainerPort`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerPort(java.lang.Number containerPort) {
this.containerPort = containerPort;
return this;
}
/**
* Sets the value of {@link LoadBalancerProperty#getContainerName}
* @param containerName `CfnService.LoadBalancerProperty.ContainerName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerName(java.lang.String containerName) {
this.containerName = containerName;
return this;
}
/**
* Sets the value of {@link LoadBalancerProperty#getLoadBalancerName}
* @param loadBalancerName `CfnService.LoadBalancerProperty.LoadBalancerName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder loadBalancerName(java.lang.String loadBalancerName) {
this.loadBalancerName = loadBalancerName;
return this;
}
/**
* Sets the value of {@link LoadBalancerProperty#getTargetGroupArn}
* @param targetGroupArn `CfnService.LoadBalancerProperty.TargetGroupArn`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetGroupArn(java.lang.String targetGroupArn) {
this.targetGroupArn = targetGroupArn;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link LoadBalancerProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public LoadBalancerProperty build() {
return new Jsii$Proxy(containerPort, containerName, loadBalancerName, targetGroupArn);
}
}
/**
* An implementation for {@link LoadBalancerProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements LoadBalancerProperty {
private final java.lang.Number containerPort;
private final java.lang.String containerName;
private final java.lang.String loadBalancerName;
private final java.lang.String targetGroupArn;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerPort = software.amazon.jsii.Kernel.get(this, "containerPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.containerName = software.amazon.jsii.Kernel.get(this, "containerName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.loadBalancerName = software.amazon.jsii.Kernel.get(this, "loadBalancerName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.targetGroupArn = software.amazon.jsii.Kernel.get(this, "targetGroupArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Number containerPort, final java.lang.String containerName, final java.lang.String loadBalancerName, final java.lang.String targetGroupArn) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerPort = java.util.Objects.requireNonNull(containerPort, "containerPort is required");
this.containerName = containerName;
this.loadBalancerName = loadBalancerName;
this.targetGroupArn = targetGroupArn;
}
@Override
public final java.lang.Number getContainerPort() {
return this.containerPort;
}
@Override
public final java.lang.String getContainerName() {
return this.containerName;
}
@Override
public final java.lang.String getLoadBalancerName() {
return this.loadBalancerName;
}
@Override
public final java.lang.String getTargetGroupArn() {
return this.targetGroupArn;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("containerPort", om.valueToTree(this.getContainerPort()));
if (this.getContainerName() != null) {
data.set("containerName", om.valueToTree(this.getContainerName()));
}
if (this.getLoadBalancerName() != null) {
data.set("loadBalancerName", om.valueToTree(this.getLoadBalancerName()));
}
if (this.getTargetGroupArn() != null) {
data.set("targetGroupArn", om.valueToTree(this.getTargetGroupArn()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.LoadBalancerProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LoadBalancerProperty.Jsii$Proxy that = (LoadBalancerProperty.Jsii$Proxy) o;
if (!containerPort.equals(that.containerPort)) return false;
if (this.containerName != null ? !this.containerName.equals(that.containerName) : that.containerName != null) return false;
if (this.loadBalancerName != null ? !this.loadBalancerName.equals(that.loadBalancerName) : that.loadBalancerName != null) return false;
return this.targetGroupArn != null ? this.targetGroupArn.equals(that.targetGroupArn) : that.targetGroupArn == null;
}
@Override
public final int hashCode() {
int result = this.containerPort.hashCode();
result = 31 * result + (this.containerName != null ? this.containerName.hashCode() : 0);
result = 31 * result + (this.loadBalancerName != null ? this.loadBalancerName.hashCode() : 0);
result = 31 * result + (this.targetGroupArn != null ? this.targetGroupArn.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-networkconfiguration.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.NetworkConfigurationProperty")
@software.amazon.jsii.Jsii.Proxy(NetworkConfigurationProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface NetworkConfigurationProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.NetworkConfigurationProperty.AwsvpcConfiguration`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-networkconfiguration.html#cfn-ecs-service-networkconfiguration-awsvpcconfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getAwsvpcConfiguration() {
return null;
}
/**
* @return a {@link Builder} of {@link NetworkConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link NetworkConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Object awsvpcConfiguration;
/**
* Sets the value of {@link NetworkConfigurationProperty#getAwsvpcConfiguration}
* @param awsvpcConfiguration `CfnService.NetworkConfigurationProperty.AwsvpcConfiguration`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder awsvpcConfiguration(software.amazon.awscdk.core.IResolvable awsvpcConfiguration) {
this.awsvpcConfiguration = awsvpcConfiguration;
return this;
}
/**
* Sets the value of {@link NetworkConfigurationProperty#getAwsvpcConfiguration}
* @param awsvpcConfiguration `CfnService.NetworkConfigurationProperty.AwsvpcConfiguration`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder awsvpcConfiguration(software.amazon.awscdk.services.ecs.CfnService.AwsVpcConfigurationProperty awsvpcConfiguration) {
this.awsvpcConfiguration = awsvpcConfiguration;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link NetworkConfigurationProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public NetworkConfigurationProperty build() {
return new Jsii$Proxy(awsvpcConfiguration);
}
}
/**
* An implementation for {@link NetworkConfigurationProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements NetworkConfigurationProperty {
private final java.lang.Object awsvpcConfiguration;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.awsvpcConfiguration = software.amazon.jsii.Kernel.get(this, "awsvpcConfiguration", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Object awsvpcConfiguration) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.awsvpcConfiguration = awsvpcConfiguration;
}
@Override
public final java.lang.Object getAwsvpcConfiguration() {
return this.awsvpcConfiguration;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAwsvpcConfiguration() != null) {
data.set("awsvpcConfiguration", om.valueToTree(this.getAwsvpcConfiguration()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.NetworkConfigurationProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
NetworkConfigurationProperty.Jsii$Proxy that = (NetworkConfigurationProperty.Jsii$Proxy) o;
return this.awsvpcConfiguration != null ? this.awsvpcConfiguration.equals(that.awsvpcConfiguration) : that.awsvpcConfiguration == null;
}
@Override
public final int hashCode() {
int result = this.awsvpcConfiguration != null ? this.awsvpcConfiguration.hashCode() : 0;
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementconstraint.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.PlacementConstraintProperty")
@software.amazon.jsii.Jsii.Proxy(PlacementConstraintProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PlacementConstraintProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.PlacementConstraintProperty.Type`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementconstraint.html#cfn-ecs-service-placementconstraint-type
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getType();
/**
* `CfnService.PlacementConstraintProperty.Expression`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementconstraint.html#cfn-ecs-service-placementconstraint-expression
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getExpression() {
return null;
}
/**
* @return a {@link Builder} of {@link PlacementConstraintProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PlacementConstraintProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String type;
private java.lang.String expression;
/**
* Sets the value of {@link PlacementConstraintProperty#getType}
* @param type `CfnService.PlacementConstraintProperty.Type`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link PlacementConstraintProperty#getExpression}
* @param expression `CfnService.PlacementConstraintProperty.Expression`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder expression(java.lang.String expression) {
this.expression = expression;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PlacementConstraintProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PlacementConstraintProperty build() {
return new Jsii$Proxy(type, expression);
}
}
/**
* An implementation for {@link PlacementConstraintProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PlacementConstraintProperty {
private final java.lang.String type;
private final java.lang.String expression;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.expression = software.amazon.jsii.Kernel.get(this, "expression", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String type, final java.lang.String expression) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.type = java.util.Objects.requireNonNull(type, "type is required");
this.expression = expression;
}
@Override
public final java.lang.String getType() {
return this.type;
}
@Override
public final java.lang.String getExpression() {
return this.expression;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("type", om.valueToTree(this.getType()));
if (this.getExpression() != null) {
data.set("expression", om.valueToTree(this.getExpression()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.PlacementConstraintProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PlacementConstraintProperty.Jsii$Proxy that = (PlacementConstraintProperty.Jsii$Proxy) o;
if (!type.equals(that.type)) return false;
return this.expression != null ? this.expression.equals(that.expression) : that.expression == null;
}
@Override
public final int hashCode() {
int result = this.type.hashCode();
result = 31 * result + (this.expression != null ? this.expression.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementstrategy.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.PlacementStrategyProperty")
@software.amazon.jsii.Jsii.Proxy(PlacementStrategyProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PlacementStrategyProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.PlacementStrategyProperty.Type`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementstrategy.html#cfn-ecs-service-placementstrategy-type
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getType();
/**
* `CfnService.PlacementStrategyProperty.Field`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-placementstrategy.html#cfn-ecs-service-placementstrategy-field
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getField() {
return null;
}
/**
* @return a {@link Builder} of {@link PlacementStrategyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PlacementStrategyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String type;
private java.lang.String field;
/**
* Sets the value of {@link PlacementStrategyProperty#getType}
* @param type `CfnService.PlacementStrategyProperty.Type`. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(java.lang.String type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link PlacementStrategyProperty#getField}
* @param field `CfnService.PlacementStrategyProperty.Field`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder field(java.lang.String field) {
this.field = field;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PlacementStrategyProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PlacementStrategyProperty build() {
return new Jsii$Proxy(type, field);
}
}
/**
* An implementation for {@link PlacementStrategyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PlacementStrategyProperty {
private final java.lang.String type;
private final java.lang.String field;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.field = software.amazon.jsii.Kernel.get(this, "field", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String type, final java.lang.String field) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.type = java.util.Objects.requireNonNull(type, "type is required");
this.field = field;
}
@Override
public final java.lang.String getType() {
return this.type;
}
@Override
public final java.lang.String getField() {
return this.field;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("type", om.valueToTree(this.getType()));
if (this.getField() != null) {
data.set("field", om.valueToTree(this.getField()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.PlacementStrategyProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PlacementStrategyProperty.Jsii$Proxy that = (PlacementStrategyProperty.Jsii$Proxy) o;
if (!type.equals(that.type)) return false;
return this.field != null ? this.field.equals(that.field) : that.field == null;
}
@Override
public final int hashCode() {
int result = this.type.hashCode();
result = 31 * result + (this.field != null ? this.field.hashCode() : 0);
return result;
}
}
}
/**
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-serviceregistry.html
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.CfnService.ServiceRegistryProperty")
@software.amazon.jsii.Jsii.Proxy(ServiceRegistryProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ServiceRegistryProperty extends software.amazon.jsii.JsiiSerializable {
/**
* `CfnService.ServiceRegistryProperty.ContainerName`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-serviceregistry.html#cfn-ecs-service-serviceregistry-containername
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getContainerName() {
return null;
}
/**
* `CfnService.ServiceRegistryProperty.ContainerPort`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-serviceregistry.html#cfn-ecs-service-serviceregistry-containerport
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getContainerPort() {
return null;
}
/**
* `CfnService.ServiceRegistryProperty.Port`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-serviceregistry.html#cfn-ecs-service-serviceregistry-port
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getPort() {
return null;
}
/**
* `CfnService.ServiceRegistryProperty.RegistryArn`.
*
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-ecs-service-serviceregistry.html#cfn-ecs-service-serviceregistry-registryarn
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRegistryArn() {
return null;
}
/**
* @return a {@link Builder} of {@link ServiceRegistryProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ServiceRegistryProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String containerName;
private java.lang.Number containerPort;
private java.lang.Number port;
private java.lang.String registryArn;
/**
* Sets the value of {@link ServiceRegistryProperty#getContainerName}
* @param containerName `CfnService.ServiceRegistryProperty.ContainerName`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerName(java.lang.String containerName) {
this.containerName = containerName;
return this;
}
/**
* Sets the value of {@link ServiceRegistryProperty#getContainerPort}
* @param containerPort `CfnService.ServiceRegistryProperty.ContainerPort`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containerPort(java.lang.Number containerPort) {
this.containerPort = containerPort;
return this;
}
/**
* Sets the value of {@link ServiceRegistryProperty#getPort}
* @param port `CfnService.ServiceRegistryProperty.Port`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link ServiceRegistryProperty#getRegistryArn}
* @param registryArn `CfnService.ServiceRegistryProperty.RegistryArn`.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder registryArn(java.lang.String registryArn) {
this.registryArn = registryArn;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ServiceRegistryProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ServiceRegistryProperty build() {
return new Jsii$Proxy(containerName, containerPort, port, registryArn);
}
}
/**
* An implementation for {@link ServiceRegistryProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ServiceRegistryProperty {
private final java.lang.String containerName;
private final java.lang.Number containerPort;
private final java.lang.Number port;
private final java.lang.String registryArn;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.containerName = software.amazon.jsii.Kernel.get(this, "containerName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.containerPort = software.amazon.jsii.Kernel.get(this, "containerPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.registryArn = software.amazon.jsii.Kernel.get(this, "registryArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String containerName, final java.lang.Number containerPort, final java.lang.Number port, final java.lang.String registryArn) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.containerName = containerName;
this.containerPort = containerPort;
this.port = port;
this.registryArn = registryArn;
}
@Override
public final java.lang.String getContainerName() {
return this.containerName;
}
@Override
public final java.lang.Number getContainerPort() {
return this.containerPort;
}
@Override
public final java.lang.Number getPort() {
return this.port;
}
@Override
public final java.lang.String getRegistryArn() {
return this.registryArn;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getContainerName() != null) {
data.set("containerName", om.valueToTree(this.getContainerName()));
}
if (this.getContainerPort() != null) {
data.set("containerPort", om.valueToTree(this.getContainerPort()));
}
if (this.getPort() != null) {
data.set("port", om.valueToTree(this.getPort()));
}
if (this.getRegistryArn() != null) {
data.set("registryArn", om.valueToTree(this.getRegistryArn()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.CfnService.ServiceRegistryProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ServiceRegistryProperty.Jsii$Proxy that = (ServiceRegistryProperty.Jsii$Proxy) o;
if (this.containerName != null ? !this.containerName.equals(that.containerName) : that.containerName != null) return false;
if (this.containerPort != null ? !this.containerPort.equals(that.containerPort) : that.containerPort != null) return false;
if (this.port != null ? !this.port.equals(that.port) : that.port != null) return false;
return this.registryArn != null ? this.registryArn.equals(that.registryArn) : that.registryArn == null;
}
@Override
public final int hashCode() {
int result = this.containerName != null ? this.containerName.hashCode() : 0;
result = 31 * result + (this.containerPort != null ? this.containerPort.hashCode() : 0);
result = 31 * result + (this.port != null ? this.port.hashCode() : 0);
result = 31 * result + (this.registryArn != null ? this.registryArn.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.CfnService}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private software.amazon.awscdk.services.ecs.CfnServiceProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* `AWS::ECS::Service.CapacityProviderStrategy`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-capacityproviderstrategy
* @param capacityProviderStrategy `AWS::ECS::Service.CapacityProviderStrategy`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder capacityProviderStrategy(final software.amazon.awscdk.core.IResolvable capacityProviderStrategy) {
this.props().capacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
* `AWS::ECS::Service.CapacityProviderStrategy`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-capacityproviderstrategy
* @param capacityProviderStrategy `AWS::ECS::Service.CapacityProviderStrategy`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder capacityProviderStrategy(final java.util.List extends java.lang.Object> capacityProviderStrategy) {
this.props().capacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
* `AWS::ECS::Service.Cluster`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-cluster
* @param cluster `AWS::ECS::Service.Cluster`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cluster(final java.lang.String cluster) {
this.props().cluster(cluster);
return this;
}
/**
* `AWS::ECS::Service.DeploymentConfiguration`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentconfiguration
* @param deploymentConfiguration `AWS::ECS::Service.DeploymentConfiguration`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentConfiguration(final software.amazon.awscdk.core.IResolvable deploymentConfiguration) {
this.props().deploymentConfiguration(deploymentConfiguration);
return this;
}
/**
* `AWS::ECS::Service.DeploymentConfiguration`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentconfiguration
* @param deploymentConfiguration `AWS::ECS::Service.DeploymentConfiguration`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentConfiguration(final software.amazon.awscdk.services.ecs.CfnService.DeploymentConfigurationProperty deploymentConfiguration) {
this.props().deploymentConfiguration(deploymentConfiguration);
return this;
}
/**
* `AWS::ECS::Service.DeploymentController`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentcontroller
* @param deploymentController `AWS::ECS::Service.DeploymentController`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentController(final software.amazon.awscdk.core.IResolvable deploymentController) {
this.props().deploymentController(deploymentController);
return this;
}
/**
* `AWS::ECS::Service.DeploymentController`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-deploymentcontroller
* @param deploymentController `AWS::ECS::Service.DeploymentController`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentController(final software.amazon.awscdk.services.ecs.CfnService.DeploymentControllerProperty deploymentController) {
this.props().deploymentController(deploymentController);
return this;
}
/**
* `AWS::ECS::Service.DesiredCount`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-desiredcount
* @param desiredCount `AWS::ECS::Service.DesiredCount`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder desiredCount(final java.lang.Number desiredCount) {
this.props().desiredCount(desiredCount);
return this;
}
/**
* `AWS::ECS::Service.EnableECSManagedTags`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-enableecsmanagedtags
* @param enableEcsManagedTags `AWS::ECS::Service.EnableECSManagedTags`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableEcsManagedTags(final java.lang.Boolean enableEcsManagedTags) {
this.props().enableEcsManagedTags(enableEcsManagedTags);
return this;
}
/**
* `AWS::ECS::Service.EnableECSManagedTags`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-enableecsmanagedtags
* @param enableEcsManagedTags `AWS::ECS::Service.EnableECSManagedTags`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableEcsManagedTags(final software.amazon.awscdk.core.IResolvable enableEcsManagedTags) {
this.props().enableEcsManagedTags(enableEcsManagedTags);
return this;
}
/**
* `AWS::ECS::Service.HealthCheckGracePeriodSeconds`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-healthcheckgraceperiodseconds
* @param healthCheckGracePeriodSeconds `AWS::ECS::Service.HealthCheckGracePeriodSeconds`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckGracePeriodSeconds(final java.lang.Number healthCheckGracePeriodSeconds) {
this.props().healthCheckGracePeriodSeconds(healthCheckGracePeriodSeconds);
return this;
}
/**
* `AWS::ECS::Service.LaunchType`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-launchtype
* @param launchType `AWS::ECS::Service.LaunchType`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder launchType(final java.lang.String launchType) {
this.props().launchType(launchType);
return this;
}
/**
* `AWS::ECS::Service.LoadBalancers`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-loadbalancers
* @param loadBalancers `AWS::ECS::Service.LoadBalancers`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder loadBalancers(final software.amazon.awscdk.core.IResolvable loadBalancers) {
this.props().loadBalancers(loadBalancers);
return this;
}
/**
* `AWS::ECS::Service.LoadBalancers`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-loadbalancers
* @param loadBalancers `AWS::ECS::Service.LoadBalancers`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder loadBalancers(final java.util.List extends java.lang.Object> loadBalancers) {
this.props().loadBalancers(loadBalancers);
return this;
}
/**
* `AWS::ECS::Service.NetworkConfiguration`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-networkconfiguration
* @param networkConfiguration `AWS::ECS::Service.NetworkConfiguration`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkConfiguration(final software.amazon.awscdk.core.IResolvable networkConfiguration) {
this.props().networkConfiguration(networkConfiguration);
return this;
}
/**
* `AWS::ECS::Service.NetworkConfiguration`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-networkconfiguration
* @param networkConfiguration `AWS::ECS::Service.NetworkConfiguration`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder networkConfiguration(final software.amazon.awscdk.services.ecs.CfnService.NetworkConfigurationProperty networkConfiguration) {
this.props().networkConfiguration(networkConfiguration);
return this;
}
/**
* `AWS::ECS::Service.PlacementConstraints`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementconstraints
* @param placementConstraints `AWS::ECS::Service.PlacementConstraints`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementConstraints(final software.amazon.awscdk.core.IResolvable placementConstraints) {
this.props().placementConstraints(placementConstraints);
return this;
}
/**
* `AWS::ECS::Service.PlacementConstraints`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementconstraints
* @param placementConstraints `AWS::ECS::Service.PlacementConstraints`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementConstraints(final java.util.List extends java.lang.Object> placementConstraints) {
this.props().placementConstraints(placementConstraints);
return this;
}
/**
* `AWS::ECS::Service.PlacementStrategies`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementstrategies
* @param placementStrategies `AWS::ECS::Service.PlacementStrategies`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementStrategies(final software.amazon.awscdk.core.IResolvable placementStrategies) {
this.props().placementStrategies(placementStrategies);
return this;
}
/**
* `AWS::ECS::Service.PlacementStrategies`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-placementstrategies
* @param placementStrategies `AWS::ECS::Service.PlacementStrategies`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementStrategies(final java.util.List extends java.lang.Object> placementStrategies) {
this.props().placementStrategies(placementStrategies);
return this;
}
/**
* `AWS::ECS::Service.PlatformVersion`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-platformversion
* @param platformVersion `AWS::ECS::Service.PlatformVersion`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder platformVersion(final java.lang.String platformVersion) {
this.props().platformVersion(platformVersion);
return this;
}
/**
* `AWS::ECS::Service.PropagateTags`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-propagatetags
* @param propagateTags `AWS::ECS::Service.PropagateTags`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder propagateTags(final java.lang.String propagateTags) {
this.props().propagateTags(propagateTags);
return this;
}
/**
* `AWS::ECS::Service.Role`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-role
* @param role `AWS::ECS::Service.Role`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final java.lang.String role) {
this.props().role(role);
return this;
}
/**
* `AWS::ECS::Service.SchedulingStrategy`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-schedulingstrategy
* @param schedulingStrategy `AWS::ECS::Service.SchedulingStrategy`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder schedulingStrategy(final java.lang.String schedulingStrategy) {
this.props().schedulingStrategy(schedulingStrategy);
return this;
}
/**
* `AWS::ECS::Service.ServiceArn`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicearn
* @param serviceArn `AWS::ECS::Service.ServiceArn`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceArn(final java.lang.String serviceArn) {
this.props().serviceArn(serviceArn);
return this;
}
/**
* `AWS::ECS::Service.ServiceName`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-servicename
* @param serviceName `AWS::ECS::Service.ServiceName`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceName(final java.lang.String serviceName) {
this.props().serviceName(serviceName);
return this;
}
/**
* `AWS::ECS::Service.ServiceRegistries`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-serviceregistries
* @param serviceRegistries `AWS::ECS::Service.ServiceRegistries`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceRegistries(final software.amazon.awscdk.core.IResolvable serviceRegistries) {
this.props().serviceRegistries(serviceRegistries);
return this;
}
/**
* `AWS::ECS::Service.ServiceRegistries`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-serviceregistries
* @param serviceRegistries `AWS::ECS::Service.ServiceRegistries`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceRegistries(final java.util.List extends java.lang.Object> serviceRegistries) {
this.props().serviceRegistries(serviceRegistries);
return this;
}
/**
* `AWS::ECS::Service.Tags`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-tags
* @param tags `AWS::ECS::Service.Tags`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props().tags(tags);
return this;
}
/**
* `AWS::ECS::Service.TaskDefinition`.
*
* @return {@code this}
* @see http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-ecs-service.html#cfn-ecs-service-taskdefinition
* @param taskDefinition `AWS::ECS::Service.TaskDefinition`. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskDefinition(final java.lang.String taskDefinition) {
this.props().taskDefinition(taskDefinition);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.CfnService}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.CfnService build() {
return new software.amazon.awscdk.services.ecs.CfnService(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.ecs.CfnServiceProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.ecs.CfnServiceProps.Builder();
}
return this.props;
}
}
}