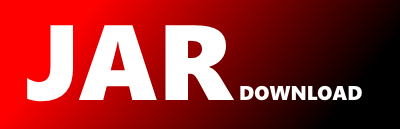
software.amazon.awscdk.services.ecs.GelfLogDriver Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A log driver that sends log information to journald Logs.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2021-01-06T15:18:00.606Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.GelfLogDriver")
public class GelfLogDriver extends software.amazon.awscdk.services.ecs.LogDriver {
protected GelfLogDriver(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected GelfLogDriver(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Constructs a new instance of the GelfLogDriver class.
*
* @param props the gelf log driver configuration options. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public GelfLogDriver(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.GelfLogDriverProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Called when the log driver is configured on a container.
*
* @param _scope This parameter is required.
* @param _containerDefinition This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.LogDriverConfig bind(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.ContainerDefinition _containerDefinition) {
return software.amazon.jsii.Kernel.call(this, "bind", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.LogDriverConfig.class), new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_containerDefinition, "_containerDefinition is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ecs.GelfLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.ecs.GelfLogDriverProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.ecs.GelfLogDriverProps.Builder();
}
/**
* The env option takes an array of keys.
*
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
*
* Default: - No env
*
* @return {@code this}
* @param env The env option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(final java.util.List env) {
this.props.env(env);
return this;
}
/**
* The env-regex option is similar to and compatible with env.
*
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
*
* Default: - No envRegex
*
* @return {@code this}
* @param envRegex The env-regex option is similar to and compatible with env. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(final java.lang.String envRegex) {
this.props.envRegex(envRegex);
return this;
}
/**
* The labels option takes an array of keys.
*
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
*
* Default: - No labels
*
* @return {@code this}
* @param labels The labels option takes an array of keys. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(final java.util.List labels) {
this.props.labels(labels);
return this;
}
/**
* By default, Docker uses the first 12 characters of the container ID to tag log messages.
*
* Refer to the log tag option documentation for customizing the
* log tag format.
*
* Default: - The first 12 characters of the container ID
*
* @return {@code this}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(final java.lang.String tag) {
this.props.tag(tag);
return this;
}
/**
* The address of the GELF server.
*
* tcp and udp are the only supported URI
* specifier and you must specify the port.
*
* @return {@code this}
* @param address The address of the GELF server. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder address(final java.lang.String address) {
this.props.address(address);
return this;
}
/**
* UDP Only The level of compression when gzip or zlib is the gelf-compression-type.
*
* An integer in the range of -1 to 9 (BestCompression). Higher levels provide more
* compression at lower speed. Either -1 or 0 disables compression.
*
* Default: - 1
*
* @return {@code this}
* @param compressionLevel UDP Only The level of compression when gzip or zlib is the gelf-compression-type. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compressionLevel(final java.lang.Number compressionLevel) {
this.props.compressionLevel(compressionLevel);
return this;
}
/**
* UDP Only The type of compression the GELF driver uses to compress each log message.
*
* Allowed values are gzip, zlib and none.
*
* Default: - gzip
*
* @return {@code this}
* @param compressionType UDP Only The type of compression the GELF driver uses to compress each log message. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder compressionType(final software.amazon.awscdk.services.ecs.GelfCompressionType compressionType) {
this.props.compressionType(compressionType);
return this;
}
/**
* TCP Only The maximum number of reconnection attempts when the connection drop.
*
* A positive integer.
*
* Default: - 3
*
* @return {@code this}
* @param tcpMaxReconnect TCP Only The maximum number of reconnection attempts when the connection drop. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tcpMaxReconnect(final java.lang.Number tcpMaxReconnect) {
this.props.tcpMaxReconnect(tcpMaxReconnect);
return this;
}
/**
* TCP Only The number of seconds to wait between reconnection attempts.
*
* A positive integer.
*
* Default: - 1
*
* @return {@code this}
* @param tcpReconnectDelay TCP Only The number of seconds to wait between reconnection attempts. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tcpReconnectDelay(final software.amazon.awscdk.core.Duration tcpReconnectDelay) {
this.props.tcpReconnectDelay(tcpReconnectDelay);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ecs.GelfLogDriver}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ecs.GelfLogDriver build() {
return new software.amazon.awscdk.services.ecs.GelfLogDriver(
this.props.build()
);
}
}
}