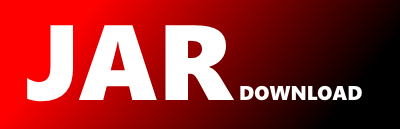
software.amazon.awscdk.services.ecs.HealthCheck Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* The health check command and associated configuration parameters for the container.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2021-01-06T15:18:00.620Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.HealthCheck")
@software.amazon.jsii.Jsii.Proxy(HealthCheck.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface HealthCheck extends software.amazon.jsii.JsiiSerializable {
/**
* A string array representing the command that the container runs to determine if it is healthy.
*
* The string array must start with CMD to execute the command arguments directly, or
* CMD-SHELL to run the command with the container's default shell.
*
* For example: [ "CMD-SHELL", "curl -f http://localhost/ || exit 1" ]
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getCommand();
/**
* The time period in seconds between each health check execution.
*
* You may specify between 5 and 300 seconds.
*
* Default: Duration.seconds(30)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getInterval() {
return null;
}
/**
* The number of times to retry a failed health check before the container is considered unhealthy.
*
* You may specify between 1 and 10 retries.
*
* Default: 3
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getRetries() {
return null;
}
/**
* The optional grace period within which to provide containers time to bootstrap before failed health checks count towards the maximum number of retries.
*
* You may specify between 0 and 300 seconds.
*
* Default: No start period
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getStartPeriod() {
return null;
}
/**
* The time period in seconds to wait for a health check to succeed before it is considered a failure.
*
* You may specify between 2 and 60 seconds.
*
* Default: Duration.seconds(5)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getTimeout() {
return null;
}
/**
* @return a {@link Builder} of {@link HealthCheck}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link HealthCheck}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.util.List command;
private software.amazon.awscdk.core.Duration interval;
private java.lang.Number retries;
private software.amazon.awscdk.core.Duration startPeriod;
private software.amazon.awscdk.core.Duration timeout;
/**
* Sets the value of {@link HealthCheck#getCommand}
* @param command A string array representing the command that the container runs to determine if it is healthy. This parameter is required.
* The string array must start with CMD to execute the command arguments directly, or
* CMD-SHELL to run the command with the container's default shell.
*
* For example: [ "CMD-SHELL", "curl -f http://localhost/ || exit 1" ]
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder command(java.util.List command) {
this.command = command;
return this;
}
/**
* Sets the value of {@link HealthCheck#getInterval}
* @param interval The time period in seconds between each health check execution.
* You may specify between 5 and 300 seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder interval(software.amazon.awscdk.core.Duration interval) {
this.interval = interval;
return this;
}
/**
* Sets the value of {@link HealthCheck#getRetries}
* @param retries The number of times to retry a failed health check before the container is considered unhealthy.
* You may specify between 1 and 10 retries.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder retries(java.lang.Number retries) {
this.retries = retries;
return this;
}
/**
* Sets the value of {@link HealthCheck#getStartPeriod}
* @param startPeriod The optional grace period within which to provide containers time to bootstrap before failed health checks count towards the maximum number of retries.
* You may specify between 0 and 300 seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder startPeriod(software.amazon.awscdk.core.Duration startPeriod) {
this.startPeriod = startPeriod;
return this;
}
/**
* Sets the value of {@link HealthCheck#getTimeout}
* @param timeout The time period in seconds to wait for a health check to succeed before it is considered a failure.
* You may specify between 2 and 60 seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timeout(software.amazon.awscdk.core.Duration timeout) {
this.timeout = timeout;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link HealthCheck}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public HealthCheck build() {
return new Jsii$Proxy(command, interval, retries, startPeriod, timeout);
}
}
/**
* An implementation for {@link HealthCheck}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements HealthCheck {
private final java.util.List command;
private final software.amazon.awscdk.core.Duration interval;
private final java.lang.Number retries;
private final software.amazon.awscdk.core.Duration startPeriod;
private final software.amazon.awscdk.core.Duration timeout;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.command = software.amazon.jsii.Kernel.get(this, "command", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.interval = software.amazon.jsii.Kernel.get(this, "interval", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.retries = software.amazon.jsii.Kernel.get(this, "retries", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.startPeriod = software.amazon.jsii.Kernel.get(this, "startPeriod", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.timeout = software.amazon.jsii.Kernel.get(this, "timeout", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.util.List command, final software.amazon.awscdk.core.Duration interval, final java.lang.Number retries, final software.amazon.awscdk.core.Duration startPeriod, final software.amazon.awscdk.core.Duration timeout) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.command = java.util.Objects.requireNonNull(command, "command is required");
this.interval = interval;
this.retries = retries;
this.startPeriod = startPeriod;
this.timeout = timeout;
}
@Override
public final java.util.List getCommand() {
return this.command;
}
@Override
public final software.amazon.awscdk.core.Duration getInterval() {
return this.interval;
}
@Override
public final java.lang.Number getRetries() {
return this.retries;
}
@Override
public final software.amazon.awscdk.core.Duration getStartPeriod() {
return this.startPeriod;
}
@Override
public final software.amazon.awscdk.core.Duration getTimeout() {
return this.timeout;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("command", om.valueToTree(this.getCommand()));
if (this.getInterval() != null) {
data.set("interval", om.valueToTree(this.getInterval()));
}
if (this.getRetries() != null) {
data.set("retries", om.valueToTree(this.getRetries()));
}
if (this.getStartPeriod() != null) {
data.set("startPeriod", om.valueToTree(this.getStartPeriod()));
}
if (this.getTimeout() != null) {
data.set("timeout", om.valueToTree(this.getTimeout()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.HealthCheck"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HealthCheck.Jsii$Proxy that = (HealthCheck.Jsii$Proxy) o;
if (!command.equals(that.command)) return false;
if (this.interval != null ? !this.interval.equals(that.interval) : that.interval != null) return false;
if (this.retries != null ? !this.retries.equals(that.retries) : that.retries != null) return false;
if (this.startPeriod != null ? !this.startPeriod.equals(that.startPeriod) : that.startPeriod != null) return false;
return this.timeout != null ? this.timeout.equals(that.timeout) : that.timeout == null;
}
@Override
public final int hashCode() {
int result = this.command.hashCode();
result = 31 * result + (this.interval != null ? this.interval.hashCode() : 0);
result = 31 * result + (this.retries != null ? this.retries.hashCode() : 0);
result = 31 * result + (this.startPeriod != null ? this.startPeriod.hashCode() : 0);
result = 31 * result + (this.timeout != null ? this.timeout.hashCode() : 0);
return result;
}
}
}