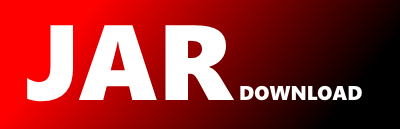
software.amazon.awscdk.services.ecs.SplunkLogDriverProps Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* Specifies the splunk log driver configuration options.
*
* Source
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2021-01-06T15:18:00.638Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.SplunkLogDriverProps")
@software.amazon.jsii.Jsii.Proxy(SplunkLogDriverProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface SplunkLogDriverProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.ecs.BaseLogDriverProps {
/**
* Splunk HTTP Event Collector token.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.core.SecretValue getToken();
/**
* Path to your Splunk Enterprise, self-service Splunk Cloud instance, or Splunk Cloud managed cluster (including port and scheme used by HTTP Event Collector) in one of the following formats: https://your_splunk_instance:8088 or https://input-prd-p-XXXXXXX.cloud.splunk.com:8088 or https://http-inputs-XXXXXXXX.splunkcloud.com.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getUrl();
/**
* Name to use for validating server certificate.
*
* Default: - The hostname of the splunk-url
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCaName() {
return null;
}
/**
* Path to root certificate.
*
* Default: - caPath not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCaPath() {
return null;
}
/**
* Message format.
*
* Can be inline, json or raw.
*
* Default: - inline
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.SplunkLogFormat getFormat() {
return null;
}
/**
* Enable/disable gzip compression to send events to Splunk Enterprise or Splunk Cloud instance.
*
* Default: - false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getGzip() {
return null;
}
/**
* Set compression level for gzip.
*
* Valid values are -1 (default), 0 (no compression),
* 1 (best speed) ... 9 (best compression).
*
* Default: - -1 (Default Compression)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getGzipLevel() {
return null;
}
/**
* Event index.
*
* Default: - index not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getIndex() {
return null;
}
/**
* Ignore server certificate validation.
*
* Default: - insecureSkipVerify not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getInsecureSkipVerify() {
return null;
}
/**
* Event source.
*
* Default: - source not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSource() {
return null;
}
/**
* Event source type.
*
* Default: - sourceType not set.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSourceType() {
return null;
}
/**
* Verify on start, that docker can connect to Splunk server.
*
* Default: - true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getVerifyConnection() {
return null;
}
/**
* @return a {@link Builder} of {@link SplunkLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SplunkLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private software.amazon.awscdk.core.SecretValue token;
private java.lang.String url;
private java.lang.String caName;
private java.lang.String caPath;
private software.amazon.awscdk.services.ecs.SplunkLogFormat format;
private java.lang.Boolean gzip;
private java.lang.Number gzipLevel;
private java.lang.String index;
private java.lang.String insecureSkipVerify;
private java.lang.String source;
private java.lang.String sourceType;
private java.lang.Boolean verifyConnection;
private java.util.List env;
private java.lang.String envRegex;
private java.util.List labels;
private java.lang.String tag;
/**
* Sets the value of {@link SplunkLogDriverProps#getToken}
* @param token Splunk HTTP Event Collector token. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder token(software.amazon.awscdk.core.SecretValue token) {
this.token = token;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getUrl}
* @param url Path to your Splunk Enterprise, self-service Splunk Cloud instance, or Splunk Cloud managed cluster (including port and scheme used by HTTP Event Collector) in one of the following formats: https://your_splunk_instance:8088 or https://input-prd-p-XXXXXXX.cloud.splunk.com:8088 or https://http-inputs-XXXXXXXX.splunkcloud.com. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder url(java.lang.String url) {
this.url = url;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getCaName}
* @param caName Name to use for validating server certificate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caName(java.lang.String caName) {
this.caName = caName;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getCaPath}
* @param caPath Path to root certificate.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caPath(java.lang.String caPath) {
this.caPath = caPath;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getFormat}
* @param format Message format.
* Can be inline, json or raw.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder format(software.amazon.awscdk.services.ecs.SplunkLogFormat format) {
this.format = format;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getGzip}
* @param gzip Enable/disable gzip compression to send events to Splunk Enterprise or Splunk Cloud instance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gzip(java.lang.Boolean gzip) {
this.gzip = gzip;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getGzipLevel}
* @param gzipLevel Set compression level for gzip.
* Valid values are -1 (default), 0 (no compression),
* 1 (best speed) ... 9 (best compression).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder gzipLevel(java.lang.Number gzipLevel) {
this.gzipLevel = gzipLevel;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getIndex}
* @param index Event index.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder index(java.lang.String index) {
this.index = index;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getInsecureSkipVerify}
* @param insecureSkipVerify Ignore server certificate validation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder insecureSkipVerify(java.lang.String insecureSkipVerify) {
this.insecureSkipVerify = insecureSkipVerify;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getSource}
* @param source Event source.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder source(java.lang.String source) {
this.source = source;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getSourceType}
* @param sourceType Event source type.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceType(java.lang.String sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getVerifyConnection}
* @param verifyConnection Verify on start, that docker can connect to Splunk server.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder verifyConnection(java.lang.Boolean verifyConnection) {
this.verifyConnection = verifyConnection;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getEnv}
* @param env The env option takes an array of keys.
* If there is collision between
* label and env keys, the value of the env takes precedence. Adds additional fields
* to the extra attributes of a logging message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder env(java.util.List env) {
this.env = env;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getEnvRegex}
* @param envRegex The env-regex option is similar to and compatible with env.
* Its value is a regular
* expression to match logging-related environment variables. It is used for advanced
* log tag options.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder envRegex(java.lang.String envRegex) {
this.envRegex = envRegex;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getLabels}
* @param labels The labels option takes an array of keys.
* If there is collision
* between label and env keys, the value of the env takes precedence. Adds additional
* fields to the extra attributes of a logging message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(java.util.List labels) {
this.labels = labels;
return this;
}
/**
* Sets the value of {@link SplunkLogDriverProps#getTag}
* @param tag By default, Docker uses the first 12 characters of the container ID to tag log messages.
* Refer to the log tag option documentation for customizing the
* log tag format.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tag(java.lang.String tag) {
this.tag = tag;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SplunkLogDriverProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public SplunkLogDriverProps build() {
return new Jsii$Proxy(token, url, caName, caPath, format, gzip, gzipLevel, index, insecureSkipVerify, source, sourceType, verifyConnection, env, envRegex, labels, tag);
}
}
/**
* An implementation for {@link SplunkLogDriverProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SplunkLogDriverProps {
private final software.amazon.awscdk.core.SecretValue token;
private final java.lang.String url;
private final java.lang.String caName;
private final java.lang.String caPath;
private final software.amazon.awscdk.services.ecs.SplunkLogFormat format;
private final java.lang.Boolean gzip;
private final java.lang.Number gzipLevel;
private final java.lang.String index;
private final java.lang.String insecureSkipVerify;
private final java.lang.String source;
private final java.lang.String sourceType;
private final java.lang.Boolean verifyConnection;
private final java.util.List env;
private final java.lang.String envRegex;
private final java.util.List labels;
private final java.lang.String tag;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.token = software.amazon.jsii.Kernel.get(this, "token", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.SecretValue.class));
this.url = software.amazon.jsii.Kernel.get(this, "url", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.caName = software.amazon.jsii.Kernel.get(this, "caName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.caPath = software.amazon.jsii.Kernel.get(this, "caPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.format = software.amazon.jsii.Kernel.get(this, "format", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.SplunkLogFormat.class));
this.gzip = software.amazon.jsii.Kernel.get(this, "gzip", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.gzipLevel = software.amazon.jsii.Kernel.get(this, "gzipLevel", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.index = software.amazon.jsii.Kernel.get(this, "index", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.insecureSkipVerify = software.amazon.jsii.Kernel.get(this, "insecureSkipVerify", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.source = software.amazon.jsii.Kernel.get(this, "source", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sourceType = software.amazon.jsii.Kernel.get(this, "sourceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.verifyConnection = software.amazon.jsii.Kernel.get(this, "verifyConnection", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.env = software.amazon.jsii.Kernel.get(this, "env", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.envRegex = software.amazon.jsii.Kernel.get(this, "envRegex", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.labels = software.amazon.jsii.Kernel.get(this, "labels", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.tag = software.amazon.jsii.Kernel.get(this, "tag", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final software.amazon.awscdk.core.SecretValue token, final java.lang.String url, final java.lang.String caName, final java.lang.String caPath, final software.amazon.awscdk.services.ecs.SplunkLogFormat format, final java.lang.Boolean gzip, final java.lang.Number gzipLevel, final java.lang.String index, final java.lang.String insecureSkipVerify, final java.lang.String source, final java.lang.String sourceType, final java.lang.Boolean verifyConnection, final java.util.List env, final java.lang.String envRegex, final java.util.List labels, final java.lang.String tag) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.token = java.util.Objects.requireNonNull(token, "token is required");
this.url = java.util.Objects.requireNonNull(url, "url is required");
this.caName = caName;
this.caPath = caPath;
this.format = format;
this.gzip = gzip;
this.gzipLevel = gzipLevel;
this.index = index;
this.insecureSkipVerify = insecureSkipVerify;
this.source = source;
this.sourceType = sourceType;
this.verifyConnection = verifyConnection;
this.env = env;
this.envRegex = envRegex;
this.labels = labels;
this.tag = tag;
}
@Override
public final software.amazon.awscdk.core.SecretValue getToken() {
return this.token;
}
@Override
public final java.lang.String getUrl() {
return this.url;
}
@Override
public final java.lang.String getCaName() {
return this.caName;
}
@Override
public final java.lang.String getCaPath() {
return this.caPath;
}
@Override
public final software.amazon.awscdk.services.ecs.SplunkLogFormat getFormat() {
return this.format;
}
@Override
public final java.lang.Boolean getGzip() {
return this.gzip;
}
@Override
public final java.lang.Number getGzipLevel() {
return this.gzipLevel;
}
@Override
public final java.lang.String getIndex() {
return this.index;
}
@Override
public final java.lang.String getInsecureSkipVerify() {
return this.insecureSkipVerify;
}
@Override
public final java.lang.String getSource() {
return this.source;
}
@Override
public final java.lang.String getSourceType() {
return this.sourceType;
}
@Override
public final java.lang.Boolean getVerifyConnection() {
return this.verifyConnection;
}
@Override
public final java.util.List getEnv() {
return this.env;
}
@Override
public final java.lang.String getEnvRegex() {
return this.envRegex;
}
@Override
public final java.util.List getLabels() {
return this.labels;
}
@Override
public final java.lang.String getTag() {
return this.tag;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("token", om.valueToTree(this.getToken()));
data.set("url", om.valueToTree(this.getUrl()));
if (this.getCaName() != null) {
data.set("caName", om.valueToTree(this.getCaName()));
}
if (this.getCaPath() != null) {
data.set("caPath", om.valueToTree(this.getCaPath()));
}
if (this.getFormat() != null) {
data.set("format", om.valueToTree(this.getFormat()));
}
if (this.getGzip() != null) {
data.set("gzip", om.valueToTree(this.getGzip()));
}
if (this.getGzipLevel() != null) {
data.set("gzipLevel", om.valueToTree(this.getGzipLevel()));
}
if (this.getIndex() != null) {
data.set("index", om.valueToTree(this.getIndex()));
}
if (this.getInsecureSkipVerify() != null) {
data.set("insecureSkipVerify", om.valueToTree(this.getInsecureSkipVerify()));
}
if (this.getSource() != null) {
data.set("source", om.valueToTree(this.getSource()));
}
if (this.getSourceType() != null) {
data.set("sourceType", om.valueToTree(this.getSourceType()));
}
if (this.getVerifyConnection() != null) {
data.set("verifyConnection", om.valueToTree(this.getVerifyConnection()));
}
if (this.getEnv() != null) {
data.set("env", om.valueToTree(this.getEnv()));
}
if (this.getEnvRegex() != null) {
data.set("envRegex", om.valueToTree(this.getEnvRegex()));
}
if (this.getLabels() != null) {
data.set("labels", om.valueToTree(this.getLabels()));
}
if (this.getTag() != null) {
data.set("tag", om.valueToTree(this.getTag()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.SplunkLogDriverProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SplunkLogDriverProps.Jsii$Proxy that = (SplunkLogDriverProps.Jsii$Proxy) o;
if (!token.equals(that.token)) return false;
if (!url.equals(that.url)) return false;
if (this.caName != null ? !this.caName.equals(that.caName) : that.caName != null) return false;
if (this.caPath != null ? !this.caPath.equals(that.caPath) : that.caPath != null) return false;
if (this.format != null ? !this.format.equals(that.format) : that.format != null) return false;
if (this.gzip != null ? !this.gzip.equals(that.gzip) : that.gzip != null) return false;
if (this.gzipLevel != null ? !this.gzipLevel.equals(that.gzipLevel) : that.gzipLevel != null) return false;
if (this.index != null ? !this.index.equals(that.index) : that.index != null) return false;
if (this.insecureSkipVerify != null ? !this.insecureSkipVerify.equals(that.insecureSkipVerify) : that.insecureSkipVerify != null) return false;
if (this.source != null ? !this.source.equals(that.source) : that.source != null) return false;
if (this.sourceType != null ? !this.sourceType.equals(that.sourceType) : that.sourceType != null) return false;
if (this.verifyConnection != null ? !this.verifyConnection.equals(that.verifyConnection) : that.verifyConnection != null) return false;
if (this.env != null ? !this.env.equals(that.env) : that.env != null) return false;
if (this.envRegex != null ? !this.envRegex.equals(that.envRegex) : that.envRegex != null) return false;
if (this.labels != null ? !this.labels.equals(that.labels) : that.labels != null) return false;
return this.tag != null ? this.tag.equals(that.tag) : that.tag == null;
}
@Override
public final int hashCode() {
int result = this.token.hashCode();
result = 31 * result + (this.url.hashCode());
result = 31 * result + (this.caName != null ? this.caName.hashCode() : 0);
result = 31 * result + (this.caPath != null ? this.caPath.hashCode() : 0);
result = 31 * result + (this.format != null ? this.format.hashCode() : 0);
result = 31 * result + (this.gzip != null ? this.gzip.hashCode() : 0);
result = 31 * result + (this.gzipLevel != null ? this.gzipLevel.hashCode() : 0);
result = 31 * result + (this.index != null ? this.index.hashCode() : 0);
result = 31 * result + (this.insecureSkipVerify != null ? this.insecureSkipVerify.hashCode() : 0);
result = 31 * result + (this.source != null ? this.source.hashCode() : 0);
result = 31 * result + (this.sourceType != null ? this.sourceType.hashCode() : 0);
result = 31 * result + (this.verifyConnection != null ? this.verifyConnection.hashCode() : 0);
result = 31 * result + (this.env != null ? this.env.hashCode() : 0);
result = 31 * result + (this.envRegex != null ? this.envRegex.hashCode() : 0);
result = 31 * result + (this.labels != null ? this.labels.hashCode() : 0);
result = 31 * result + (this.tag != null ? this.tag.hashCode() : 0);
return result;
}
}
}