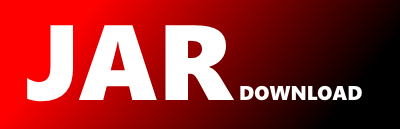
software.amazon.awscdk.services.ecs.Volume Maven / Gradle / Ivy
Show all versions of ecs Show documentation
package software.amazon.awscdk.services.ecs;
/**
* A data volume used in a task definition.
*
* For tasks that use a Docker volume, specify a DockerVolumeConfiguration.
* For tasks that use a bind mount host volume, specify a host and optional sourcePath.
*
* For more information, see Using Data Volumes in Tasks.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2021-01-06T15:18:00.655Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ecs.$Module.class, fqn = "@aws-cdk/aws-ecs.Volume")
@software.amazon.jsii.Jsii.Proxy(Volume.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface Volume extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the volume.
*
* Up to 255 letters (uppercase and lowercase), numbers, and hyphens are allowed.
* This name is referenced in the sourceVolume parameter of container definition mountPoints.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* This property is specified when you are using Docker volumes.
*
* Docker volumes are only supported when you are using the EC2 launch type.
* Windows containers only support the use of the local driver.
* To use bind mounts, specify a host instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.DockerVolumeConfiguration getDockerVolumeConfiguration() {
return null;
}
/**
* This property is specified when you are using Amazon EFS.
*
* When specifying Amazon EFS volumes in tasks using the Fargate launch type,
* Fargate creates a supervisor container that is responsible for managing the Amazon EFS volume.
* The supervisor container uses a small amount of the task's memory.
* The supervisor container is visible when querying the task metadata version 4 endpoint,
* but is not visible in CloudWatch Container Insights.
*
* Default: No Elastic FileSystem is setup
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.EfsVolumeConfiguration getEfsVolumeConfiguration() {
return null;
}
/**
* This property is specified when you are using bind mount host volumes.
*
* Bind mount host volumes are supported when you are using either the EC2 or Fargate launch types.
* The contents of the host parameter determine whether your bind mount host volume persists on the
* host container instance and where it is stored. If the host parameter is empty, then the Docker
* daemon assigns a host path for your data volume. However, the data is not guaranteed to persist
* after the containers associated with it stop running.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ecs.Host getHost() {
return null;
}
/**
* @return a {@link Builder} of {@link Volume}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link Volume}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String name;
private software.amazon.awscdk.services.ecs.DockerVolumeConfiguration dockerVolumeConfiguration;
private software.amazon.awscdk.services.ecs.EfsVolumeConfiguration efsVolumeConfiguration;
private software.amazon.awscdk.services.ecs.Host host;
/**
* Sets the value of {@link Volume#getName}
* @param name The name of the volume. This parameter is required.
* Up to 255 letters (uppercase and lowercase), numbers, and hyphens are allowed.
* This name is referenced in the sourceVolume parameter of container definition mountPoints.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link Volume#getDockerVolumeConfiguration}
* @param dockerVolumeConfiguration This property is specified when you are using Docker volumes.
* Docker volumes are only supported when you are using the EC2 launch type.
* Windows containers only support the use of the local driver.
* To use bind mounts, specify a host instead.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dockerVolumeConfiguration(software.amazon.awscdk.services.ecs.DockerVolumeConfiguration dockerVolumeConfiguration) {
this.dockerVolumeConfiguration = dockerVolumeConfiguration;
return this;
}
/**
* Sets the value of {@link Volume#getEfsVolumeConfiguration}
* @param efsVolumeConfiguration This property is specified when you are using Amazon EFS.
* When specifying Amazon EFS volumes in tasks using the Fargate launch type,
* Fargate creates a supervisor container that is responsible for managing the Amazon EFS volume.
* The supervisor container uses a small amount of the task's memory.
* The supervisor container is visible when querying the task metadata version 4 endpoint,
* but is not visible in CloudWatch Container Insights.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder efsVolumeConfiguration(software.amazon.awscdk.services.ecs.EfsVolumeConfiguration efsVolumeConfiguration) {
this.efsVolumeConfiguration = efsVolumeConfiguration;
return this;
}
/**
* Sets the value of {@link Volume#getHost}
* @param host This property is specified when you are using bind mount host volumes.
* Bind mount host volumes are supported when you are using either the EC2 or Fargate launch types.
* The contents of the host parameter determine whether your bind mount host volume persists on the
* host container instance and where it is stored. If the host parameter is empty, then the Docker
* daemon assigns a host path for your data volume. However, the data is not guaranteed to persist
* after the containers associated with it stop running.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder host(software.amazon.awscdk.services.ecs.Host host) {
this.host = host;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link Volume}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public Volume build() {
return new Jsii$Proxy(name, dockerVolumeConfiguration, efsVolumeConfiguration, host);
}
}
/**
* An implementation for {@link Volume}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements Volume {
private final java.lang.String name;
private final software.amazon.awscdk.services.ecs.DockerVolumeConfiguration dockerVolumeConfiguration;
private final software.amazon.awscdk.services.ecs.EfsVolumeConfiguration efsVolumeConfiguration;
private final software.amazon.awscdk.services.ecs.Host host;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.dockerVolumeConfiguration = software.amazon.jsii.Kernel.get(this, "dockerVolumeConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.DockerVolumeConfiguration.class));
this.efsVolumeConfiguration = software.amazon.jsii.Kernel.get(this, "efsVolumeConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.EfsVolumeConfiguration.class));
this.host = software.amazon.jsii.Kernel.get(this, "host", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.Host.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String name, final software.amazon.awscdk.services.ecs.DockerVolumeConfiguration dockerVolumeConfiguration, final software.amazon.awscdk.services.ecs.EfsVolumeConfiguration efsVolumeConfiguration, final software.amazon.awscdk.services.ecs.Host host) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.name = java.util.Objects.requireNonNull(name, "name is required");
this.dockerVolumeConfiguration = dockerVolumeConfiguration;
this.efsVolumeConfiguration = efsVolumeConfiguration;
this.host = host;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final software.amazon.awscdk.services.ecs.DockerVolumeConfiguration getDockerVolumeConfiguration() {
return this.dockerVolumeConfiguration;
}
@Override
public final software.amazon.awscdk.services.ecs.EfsVolumeConfiguration getEfsVolumeConfiguration() {
return this.efsVolumeConfiguration;
}
@Override
public final software.amazon.awscdk.services.ecs.Host getHost() {
return this.host;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("name", om.valueToTree(this.getName()));
if (this.getDockerVolumeConfiguration() != null) {
data.set("dockerVolumeConfiguration", om.valueToTree(this.getDockerVolumeConfiguration()));
}
if (this.getEfsVolumeConfiguration() != null) {
data.set("efsVolumeConfiguration", om.valueToTree(this.getEfsVolumeConfiguration()));
}
if (this.getHost() != null) {
data.set("host", om.valueToTree(this.getHost()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-ecs.Volume"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Volume.Jsii$Proxy that = (Volume.Jsii$Proxy) o;
if (!name.equals(that.name)) return false;
if (this.dockerVolumeConfiguration != null ? !this.dockerVolumeConfiguration.equals(that.dockerVolumeConfiguration) : that.dockerVolumeConfiguration != null) return false;
if (this.efsVolumeConfiguration != null ? !this.efsVolumeConfiguration.equals(that.efsVolumeConfiguration) : that.efsVolumeConfiguration != null) return false;
return this.host != null ? this.host.equals(that.host) : that.host == null;
}
@Override
public final int hashCode() {
int result = this.name.hashCode();
result = 31 * result + (this.dockerVolumeConfiguration != null ? this.dockerVolumeConfiguration.hashCode() : 0);
result = 31 * result + (this.efsVolumeConfiguration != null ? this.efsVolumeConfiguration.hashCode() : 0);
result = 31 * result + (this.host != null ? this.host.hashCode() : 0);
return result;
}
}
}