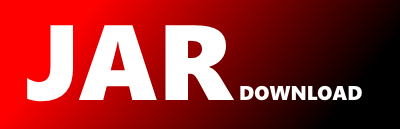
software.amazon.awscdk.services.eks.CfnFargateProfileProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.eks;
/**
* Properties for defining a `CfnFargateProfile`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.eks.*;
* CfnFargateProfileProps cfnFargateProfileProps = CfnFargateProfileProps.builder()
* .clusterName("clusterName")
* .podExecutionRoleArn("podExecutionRoleArn")
* .selectors(List.of(SelectorProperty.builder()
* .namespace("namespace")
* // the properties below are optional
* .labels(List.of(LabelProperty.builder()
* .key("key")
* .value("value")
* .build()))
* .build()))
* // the properties below are optional
* .fargateProfileName("fargateProfileName")
* .subnets(List.of("subnets"))
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-02T14:17:25.973Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.eks.$Module.class, fqn = "@aws-cdk/aws-eks.CfnFargateProfileProps")
@software.amazon.jsii.Jsii.Proxy(CfnFargateProfileProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnFargateProfileProps extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the Amazon EKS cluster to apply the Fargate profile to.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getClusterName();
/**
* The Amazon Resource Name (ARN) of the pod execution role to use for pods that match the selectors in the Fargate profile.
*
* The pod execution role allows Fargate infrastructure to register with your cluster as a node, and it provides read access to Amazon ECR image repositories. For more information, see Pod Execution Role in the Amazon EKS User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getPodExecutionRoleArn();
/**
* The selectors to match for pods to use this Fargate profile.
*
* Each selector must have an associated namespace. Optionally, you can also specify labels for a namespace. You may specify up to five selectors in a Fargate profile.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getSelectors();
/**
* The name of the Fargate profile.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getFargateProfileName() {
return null;
}
/**
* The IDs of subnets to launch your pods into.
*
* At this time, pods running on Fargate are not assigned public IP addresses, so only private subnets (with no direct route to an Internet Gateway) are accepted for this parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSubnets() {
return null;
}
/**
* The metadata to apply to the Fargate profile to assist with categorization and organization.
*
* Each tag consists of a key and an optional value. You define both. Fargate profile tags do not propagate to any other resources associated with the Fargate profile, such as the pods that are scheduled with it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnFargateProfileProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnFargateProfileProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String clusterName;
java.lang.String podExecutionRoleArn;
java.lang.Object selectors;
java.lang.String fargateProfileName;
java.util.List subnets;
java.util.List tags;
/**
* Sets the value of {@link CfnFargateProfileProps#getClusterName}
* @param clusterName The name of the Amazon EKS cluster to apply the Fargate profile to. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder clusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getPodExecutionRoleArn}
* @param podExecutionRoleArn The Amazon Resource Name (ARN) of the pod execution role to use for pods that match the selectors in the Fargate profile. This parameter is required.
* The pod execution role allows Fargate infrastructure to register with your cluster as a node, and it provides read access to Amazon ECR image repositories. For more information, see Pod Execution Role in the Amazon EKS User Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder podExecutionRoleArn(java.lang.String podExecutionRoleArn) {
this.podExecutionRoleArn = podExecutionRoleArn;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getSelectors}
* @param selectors The selectors to match for pods to use this Fargate profile. This parameter is required.
* Each selector must have an associated namespace. Optionally, you can also specify labels for a namespace. You may specify up to five selectors in a Fargate profile.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder selectors(software.amazon.awscdk.core.IResolvable selectors) {
this.selectors = selectors;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getSelectors}
* @param selectors The selectors to match for pods to use this Fargate profile. This parameter is required.
* Each selector must have an associated namespace. Optionally, you can also specify labels for a namespace. You may specify up to five selectors in a Fargate profile.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder selectors(java.util.List extends java.lang.Object> selectors) {
this.selectors = selectors;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getFargateProfileName}
* @param fargateProfileName The name of the Fargate profile.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fargateProfileName(java.lang.String fargateProfileName) {
this.fargateProfileName = fargateProfileName;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getSubnets}
* @param subnets The IDs of subnets to launch your pods into.
* At this time, pods running on Fargate are not assigned public IP addresses, so only private subnets (with no direct route to an Internet Gateway) are accepted for this parameter.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder subnets(java.util.List subnets) {
this.subnets = subnets;
return this;
}
/**
* Sets the value of {@link CfnFargateProfileProps#getTags}
* @param tags The metadata to apply to the Fargate profile to assist with categorization and organization.
* Each tag consists of a key and an optional value. You define both. Fargate profile tags do not propagate to any other resources associated with the Fargate profile, such as the pods that are scheduled with it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnFargateProfileProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnFargateProfileProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnFargateProfileProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnFargateProfileProps {
private final java.lang.String clusterName;
private final java.lang.String podExecutionRoleArn;
private final java.lang.Object selectors;
private final java.lang.String fargateProfileName;
private final java.util.List subnets;
private final java.util.List tags;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.clusterName = software.amazon.jsii.Kernel.get(this, "clusterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.podExecutionRoleArn = software.amazon.jsii.Kernel.get(this, "podExecutionRoleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.selectors = software.amazon.jsii.Kernel.get(this, "selectors", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.fargateProfileName = software.amazon.jsii.Kernel.get(this, "fargateProfileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.subnets = software.amazon.jsii.Kernel.get(this, "subnets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.clusterName = java.util.Objects.requireNonNull(builder.clusterName, "clusterName is required");
this.podExecutionRoleArn = java.util.Objects.requireNonNull(builder.podExecutionRoleArn, "podExecutionRoleArn is required");
this.selectors = java.util.Objects.requireNonNull(builder.selectors, "selectors is required");
this.fargateProfileName = builder.fargateProfileName;
this.subnets = builder.subnets;
this.tags = (java.util.List)builder.tags;
}
@Override
public final java.lang.String getClusterName() {
return this.clusterName;
}
@Override
public final java.lang.String getPodExecutionRoleArn() {
return this.podExecutionRoleArn;
}
@Override
public final java.lang.Object getSelectors() {
return this.selectors;
}
@Override
public final java.lang.String getFargateProfileName() {
return this.fargateProfileName;
}
@Override
public final java.util.List getSubnets() {
return this.subnets;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("clusterName", om.valueToTree(this.getClusterName()));
data.set("podExecutionRoleArn", om.valueToTree(this.getPodExecutionRoleArn()));
data.set("selectors", om.valueToTree(this.getSelectors()));
if (this.getFargateProfileName() != null) {
data.set("fargateProfileName", om.valueToTree(this.getFargateProfileName()));
}
if (this.getSubnets() != null) {
data.set("subnets", om.valueToTree(this.getSubnets()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-eks.CfnFargateProfileProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnFargateProfileProps.Jsii$Proxy that = (CfnFargateProfileProps.Jsii$Proxy) o;
if (!clusterName.equals(that.clusterName)) return false;
if (!podExecutionRoleArn.equals(that.podExecutionRoleArn)) return false;
if (!selectors.equals(that.selectors)) return false;
if (this.fargateProfileName != null ? !this.fargateProfileName.equals(that.fargateProfileName) : that.fargateProfileName != null) return false;
if (this.subnets != null ? !this.subnets.equals(that.subnets) : that.subnets != null) return false;
return this.tags != null ? this.tags.equals(that.tags) : that.tags == null;
}
@Override
public final int hashCode() {
int result = this.clusterName.hashCode();
result = 31 * result + (this.podExecutionRoleArn.hashCode());
result = 31 * result + (this.selectors.hashCode());
result = 31 * result + (this.fargateProfileName != null ? this.fargateProfileName.hashCode() : 0);
result = 31 * result + (this.subnets != null ? this.subnets.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
return result;
}
}
}