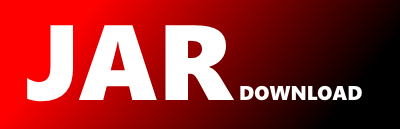
software.amazon.awscdk.services.eks.KubernetesManifest Maven / Gradle / Ivy
package software.amazon.awscdk.services.eks;
/**
* Represents a manifest within the Kubernetes system.
*
* Alternatively, you can use cluster.addManifest(resource[, resource, ...])
* to define resources on this cluster.
*
* Applies/deletes the manifest using kubectl
.
*
* Example:
*
*
* Cluster cluster;
* KubernetesManifest namespace = cluster.addManifest("my-namespace", Map.of(
* "apiVersion", "v1",
* "kind", "Namespace",
* "metadata", Map.of("name", "my-app")));
* KubernetesManifest service = cluster.addManifest("my-service", Map.of(
* "metadata", Map.of(
* "name", "myservice",
* "namespace", "my-app"),
* "spec", Map.of()));
* service.node.addDependency(namespace);
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-02T14:17:26.064Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.eks.$Module.class, fqn = "@aws-cdk/aws-eks.KubernetesManifest")
public class KubernetesManifest extends software.amazon.awscdk.core.Construct {
protected KubernetesManifest(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected KubernetesManifest(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
RESOURCE_TYPE = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.eks.KubernetesManifest.class, "RESOURCE_TYPE", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public KubernetesManifest(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.eks.KubernetesManifestProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation reosurce type.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String RESOURCE_TYPE;
/**
* A fluent builder for {@link software.amazon.awscdk.services.eks.KubernetesManifest}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.eks.KubernetesManifestProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.eks.KubernetesManifestProps.Builder();
}
/**
* Automatically detect `Ingress` resources in the manifest and annotate them so they are picked up by an ALB Ingress Controller.
*
* Default: false
*
* @return {@code this}
* @param ingressAlb Automatically detect `Ingress` resources in the manifest and annotate them so they are picked up by an ALB Ingress Controller. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ingressAlb(final java.lang.Boolean ingressAlb) {
this.props.ingressAlb(ingressAlb);
return this;
}
/**
* Specify the ALB scheme that should be applied to `Ingress` resources.
*
* Only applicable if ingressAlb
is set to true
.
*
* Default: AlbScheme.INTERNAL
*
* @return {@code this}
* @param ingressAlbScheme Specify the ALB scheme that should be applied to `Ingress` resources. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ingressAlbScheme(final software.amazon.awscdk.services.eks.AlbScheme ingressAlbScheme) {
this.props.ingressAlbScheme(ingressAlbScheme);
return this;
}
/**
* When a resource is removed from a Kubernetes manifest, it no longer appears in the manifest, and there is no way to know that this resource needs to be deleted.
*
* To address this, kubectl apply
has a --prune
option which will
* query the cluster for all resources with a specific label and will remove
* all the labeld resources that are not part of the applied manifest. If this
* option is disabled and a resource is removed, it will become "orphaned" and
* will not be deleted from the cluster.
*
* When this option is enabled (default), the construct will inject a label to
* all Kubernetes resources included in this manifest which will be used to
* prune resources when the manifest changes via kubectl apply --prune
.
*
* The label name will be aws.cdk.eks/prune-<ADDR>
where <ADDR>
is the
* 42-char unique address of this construct in the construct tree. Value is
* empty.
*
* Default: - based on the prune option of the cluster, which is `true` unless
* otherwise specified.
*
* @return {@code this}
* @see https://kubernetes.io/docs/tasks/manage-kubernetes-objects/declarative-config/#alternative-kubectl-apply-f-directory-prune-l-your-label
* @param prune When a resource is removed from a Kubernetes manifest, it no longer appears in the manifest, and there is no way to know that this resource needs to be deleted. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder prune(final java.lang.Boolean prune) {
this.props.prune(prune);
return this;
}
/**
* A flag to signify if the manifest validation should be skipped.
*
* Default: false
*
* @return {@code this}
* @param skipValidation A flag to signify if the manifest validation should be skipped. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder skipValidation(final java.lang.Boolean skipValidation) {
this.props.skipValidation(skipValidation);
return this;
}
/**
* The EKS cluster to apply this manifest to.
*
* [disable-awslint:ref-via-interface]
*
* @return {@code this}
* @param cluster The EKS cluster to apply this manifest to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cluster(final software.amazon.awscdk.services.eks.ICluster cluster) {
this.props.cluster(cluster);
return this;
}
/**
* The manifest to apply.
*
* Consists of any number of child resources.
*
* When the resources are created/updated, this manifest will be applied to the
* cluster through kubectl apply
and when the resources or the stack is
* deleted, the resources in the manifest will be deleted through kubectl delete
.
*
* Example:
*
*
* List.of(Map.of(
* "apiVersion", "v1",
* "kind", "Pod",
* "metadata", Map.of("name", "mypod"),
* "spec", Map.of(
* "containers", List.of(Map.of("name", "hello", "image", "paulbouwer/hello-kubernetes:1.5", "ports", List.of(Map.of("containerPort", 8080)))))));
*
*
* @return {@code this}
* @param manifest The manifest to apply. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder manifest(final java.util.List extends java.util.Map> manifest) {
this.props.manifest(manifest);
return this;
}
/**
* Overwrite any existing resources.
*
* If this is set, we will use kubectl apply
instead of kubectl create
* when the resource is created. Otherwise, if there is already a resource
* in the cluster with the same name, the operation will fail.
*
* Default: false
*
* @return {@code this}
* @param overwrite Overwrite any existing resources. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder overwrite(final java.lang.Boolean overwrite) {
this.props.overwrite(overwrite);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.eks.KubernetesManifest}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.eks.KubernetesManifest build() {
return new software.amazon.awscdk.services.eks.KubernetesManifest(
this.scope,
this.id,
this.props.build()
);
}
}
}