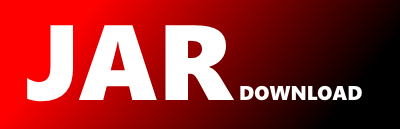
software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticbeanstalk Show documentation
Show all versions of elasticbeanstalk Show documentation
The CDK Construct Library for AWS::ElasticBeanstalk
package software.amazon.awscdk.services.elasticbeanstalk;
/**
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.7.15 (build e429c41)", date = "2019-03-07T22:11:43.634Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.elasticbeanstalk.$Module.class, fqn = "@aws-cdk/aws-elasticbeanstalk.CfnEnvironment")
public class CfnEnvironment extends software.amazon.awscdk.Resource {
protected CfnEnvironment(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
static {
RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironment.class, "resourceTypeName", java.lang.String.class);
}
/**
* Creates a new ``AWS::ElasticBeanstalk::Environment``.
* @param scope scope in which this resource is defined
* @param id scoped id of the resource
* @param props resource properties
*/
public CfnEnvironment(final software.amazon.awscdk.Construct scope, final java.lang.String id, final software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironmentProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, java.util.stream.Stream.concat(java.util.stream.Stream.concat(java.util.stream.Stream.of(java.util.Objects.requireNonNull(scope, "scope is required")), java.util.stream.Stream.of(java.util.Objects.requireNonNull(id, "id is required"))), java.util.stream.Stream.of(java.util.Objects.requireNonNull(props, "props is required"))).toArray());
}
@Override
protected java.util.Map renderProperties(final java.lang.Object properties) {
return this.jsiiCall("renderProperties", java.util.Map.class, java.util.stream.Stream.of(java.util.Objects.requireNonNull(properties, "properties is required")).toArray());
}
/**
* The CloudFormation resource type name for this resource class.
*/
public final static java.lang.String RESOURCE_TYPE_NAME;
/**
* @cloudformation_attribute EndpointURL
*/
public java.lang.String getEnvironmentEndpointUrl() {
return this.jsiiGet("environmentEndpointUrl", java.lang.String.class);
}
public java.lang.String getEnvironmentName() {
return this.jsiiGet("environmentName", java.lang.String.class);
}
public software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironmentProps getPropertyOverrides() {
return this.jsiiGet("propertyOverrides", software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironmentProps.class);
}
/**
* The ``TagManager`` handles setting, removing and formatting tags
*
* Tags should be managed either passing them as properties during
* initiation or by calling methods on this object. If both techniques are
* used only the tags from the TagManager will be used. ``Tag`` (aspect)
* will use the manager.
*/
public software.amazon.awscdk.TagManager getTags() {
return this.jsiiGet("tags", software.amazon.awscdk.TagManager.class);
}
/**
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html
*/
public static interface OptionSettingProperty extends software.amazon.jsii.JsiiSerializable {
/**
* ``CfnEnvironment.OptionSettingProperty.Namespace``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-namespace
*/
java.lang.String getNamespace();
/**
* ``CfnEnvironment.OptionSettingProperty.Namespace``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-namespace
*/
void setNamespace(final java.lang.String value);
/**
* ``CfnEnvironment.OptionSettingProperty.OptionName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-optionname
*/
java.lang.String getOptionName();
/**
* ``CfnEnvironment.OptionSettingProperty.OptionName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-optionname
*/
void setOptionName(final java.lang.String value);
/**
* ``CfnEnvironment.OptionSettingProperty.ResourceName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-elasticbeanstalk-environment-optionsetting-resourcename
*/
java.lang.String getResourceName();
/**
* ``CfnEnvironment.OptionSettingProperty.ResourceName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-elasticbeanstalk-environment-optionsetting-resourcename
*/
void setResourceName(final java.lang.String value);
/**
* ``CfnEnvironment.OptionSettingProperty.Value``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-value
*/
java.lang.String getValue();
/**
* ``CfnEnvironment.OptionSettingProperty.Value``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-value
*/
void setValue(final java.lang.String value);
/**
* @return a {@link Builder} of {@link OptionSettingProperty}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link OptionSettingProperty}
*/
final class Builder {
private java.lang.String _namespace;
private java.lang.String _optionName;
@javax.annotation.Nullable
private java.lang.String _resourceName;
@javax.annotation.Nullable
private java.lang.String _value;
/**
* Sets the value of Namespace
* @param value ``CfnEnvironment.OptionSettingProperty.Namespace``
* @return {@code this}
*/
public Builder withNamespace(final java.lang.String value) {
this._namespace = java.util.Objects.requireNonNull(value, "namespace is required");
return this;
}
/**
* Sets the value of OptionName
* @param value ``CfnEnvironment.OptionSettingProperty.OptionName``
* @return {@code this}
*/
public Builder withOptionName(final java.lang.String value) {
this._optionName = java.util.Objects.requireNonNull(value, "optionName is required");
return this;
}
/**
* Sets the value of ResourceName
* @param value ``CfnEnvironment.OptionSettingProperty.ResourceName``
* @return {@code this}
*/
public Builder withResourceName(@javax.annotation.Nullable final java.lang.String value) {
this._resourceName = value;
return this;
}
/**
* Sets the value of Value
* @param value ``CfnEnvironment.OptionSettingProperty.Value``
* @return {@code this}
*/
public Builder withValue(@javax.annotation.Nullable final java.lang.String value) {
this._value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link OptionSettingProperty}
* @throws NullPointerException if any required attribute was not provided
*/
public OptionSettingProperty build() {
return new OptionSettingProperty() {
private java.lang.String $namespace = java.util.Objects.requireNonNull(_namespace, "namespace is required");
private java.lang.String $optionName = java.util.Objects.requireNonNull(_optionName, "optionName is required");
@javax.annotation.Nullable
private java.lang.String $resourceName = _resourceName;
@javax.annotation.Nullable
private java.lang.String $value = _value;
@Override
public java.lang.String getNamespace() {
return this.$namespace;
}
@Override
public void setNamespace(final java.lang.String value) {
this.$namespace = java.util.Objects.requireNonNull(value, "namespace is required");
}
@Override
public java.lang.String getOptionName() {
return this.$optionName;
}
@Override
public void setOptionName(final java.lang.String value) {
this.$optionName = java.util.Objects.requireNonNull(value, "optionName is required");
}
@Override
public java.lang.String getResourceName() {
return this.$resourceName;
}
@Override
public void setResourceName(@javax.annotation.Nullable final java.lang.String value) {
this.$resourceName = value;
}
@Override
public java.lang.String getValue() {
return this.$value;
}
@Override
public void setValue(@javax.annotation.Nullable final java.lang.String value) {
this.$value = value;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironment.OptionSettingProperty {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* ``CfnEnvironment.OptionSettingProperty.Namespace``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-namespace
*/
@Override
public java.lang.String getNamespace() {
return this.jsiiGet("namespace", java.lang.String.class);
}
/**
* ``CfnEnvironment.OptionSettingProperty.Namespace``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-namespace
*/
@Override
public void setNamespace(final java.lang.String value) {
this.jsiiSet("namespace", java.util.Objects.requireNonNull(value, "namespace is required"));
}
/**
* ``CfnEnvironment.OptionSettingProperty.OptionName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-optionname
*/
@Override
public java.lang.String getOptionName() {
return this.jsiiGet("optionName", java.lang.String.class);
}
/**
* ``CfnEnvironment.OptionSettingProperty.OptionName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-optionname
*/
@Override
public void setOptionName(final java.lang.String value) {
this.jsiiSet("optionName", java.util.Objects.requireNonNull(value, "optionName is required"));
}
/**
* ``CfnEnvironment.OptionSettingProperty.ResourceName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-elasticbeanstalk-environment-optionsetting-resourcename
*/
@Override
@javax.annotation.Nullable
public java.lang.String getResourceName() {
return this.jsiiGet("resourceName", java.lang.String.class);
}
/**
* ``CfnEnvironment.OptionSettingProperty.ResourceName``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-elasticbeanstalk-environment-optionsetting-resourcename
*/
@Override
public void setResourceName(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("resourceName", value);
}
/**
* ``CfnEnvironment.OptionSettingProperty.Value``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-value
*/
@Override
@javax.annotation.Nullable
public java.lang.String getValue() {
return this.jsiiGet("value", java.lang.String.class);
}
/**
* ``CfnEnvironment.OptionSettingProperty.Value``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-option-settings.html#cfn-beanstalk-optionsettings-value
*/
@Override
public void setValue(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("value", value);
}
}
}
/**
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html
*/
public static interface TierProperty extends software.amazon.jsii.JsiiSerializable {
/**
* ``CfnEnvironment.TierProperty.Name``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-name
*/
java.lang.String getName();
/**
* ``CfnEnvironment.TierProperty.Name``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-name
*/
void setName(final java.lang.String value);
/**
* ``CfnEnvironment.TierProperty.Type``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-type
*/
java.lang.String getType();
/**
* ``CfnEnvironment.TierProperty.Type``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-type
*/
void setType(final java.lang.String value);
/**
* ``CfnEnvironment.TierProperty.Version``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-version
*/
java.lang.String getVersion();
/**
* ``CfnEnvironment.TierProperty.Version``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-version
*/
void setVersion(final java.lang.String value);
/**
* @return a {@link Builder} of {@link TierProperty}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link TierProperty}
*/
final class Builder {
@javax.annotation.Nullable
private java.lang.String _name;
@javax.annotation.Nullable
private java.lang.String _type;
@javax.annotation.Nullable
private java.lang.String _version;
/**
* Sets the value of Name
* @param value ``CfnEnvironment.TierProperty.Name``
* @return {@code this}
*/
public Builder withName(@javax.annotation.Nullable final java.lang.String value) {
this._name = value;
return this;
}
/**
* Sets the value of Type
* @param value ``CfnEnvironment.TierProperty.Type``
* @return {@code this}
*/
public Builder withType(@javax.annotation.Nullable final java.lang.String value) {
this._type = value;
return this;
}
/**
* Sets the value of Version
* @param value ``CfnEnvironment.TierProperty.Version``
* @return {@code this}
*/
public Builder withVersion(@javax.annotation.Nullable final java.lang.String value) {
this._version = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link TierProperty}
* @throws NullPointerException if any required attribute was not provided
*/
public TierProperty build() {
return new TierProperty() {
@javax.annotation.Nullable
private java.lang.String $name = _name;
@javax.annotation.Nullable
private java.lang.String $type = _type;
@javax.annotation.Nullable
private java.lang.String $version = _version;
@Override
public java.lang.String getName() {
return this.$name;
}
@Override
public void setName(@javax.annotation.Nullable final java.lang.String value) {
this.$name = value;
}
@Override
public java.lang.String getType() {
return this.$type;
}
@Override
public void setType(@javax.annotation.Nullable final java.lang.String value) {
this.$type = value;
}
@Override
public java.lang.String getVersion() {
return this.$version;
}
@Override
public void setVersion(@javax.annotation.Nullable final java.lang.String value) {
this.$version = value;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.elasticbeanstalk.CfnEnvironment.TierProperty {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* ``CfnEnvironment.TierProperty.Name``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-name
*/
@Override
@javax.annotation.Nullable
public java.lang.String getName() {
return this.jsiiGet("name", java.lang.String.class);
}
/**
* ``CfnEnvironment.TierProperty.Name``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-name
*/
@Override
public void setName(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("name", value);
}
/**
* ``CfnEnvironment.TierProperty.Type``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-type
*/
@Override
@javax.annotation.Nullable
public java.lang.String getType() {
return this.jsiiGet("type", java.lang.String.class);
}
/**
* ``CfnEnvironment.TierProperty.Type``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-type
*/
@Override
public void setType(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("type", value);
}
/**
* ``CfnEnvironment.TierProperty.Version``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-version
*/
@Override
@javax.annotation.Nullable
public java.lang.String getVersion() {
return this.jsiiGet("version", java.lang.String.class);
}
/**
* ``CfnEnvironment.TierProperty.Version``
* @link http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment-tier.html#cfn-beanstalk-env-tier-version
*/
@Override
public void setVersion(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("version", value);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy