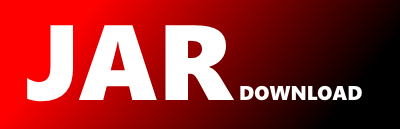
software.amazon.awscdk.services.elasticloadbalancingv2.actions.AuthenticateCognitoActionProps Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2-actions Show documentation
package software.amazon.awscdk.services.elasticloadbalancingv2.actions;
/**
* Properties for AuthenticateCognitoAction.
*
* Example:
*
*
* import software.amazon.awscdk.services.cognito.*;
* import software.amazon.awscdk.services.ec2.*;
* import software.amazon.awscdk.services.elasticloadbalancingv2.*;
* import software.amazon.awscdk.core.App;
* import software.amazon.awscdk.core.CfnOutput;
* import software.amazon.awscdk.core.Stack;
* import software.constructs.Construct;
* import software.amazon.awscdk.services.elasticloadbalancingv2.actions.*;
* CognitoStack extends Stack {CognitoStack(ApplicationLoadBalancer lb = ApplicationLoadBalancer.Builder.create(this, "LB")
* .vpc(vpc)
* .internetFacing(true)
* .build();
* UserPool userPool = new UserPool(this, "UserPool");
* UserPoolClient userPoolClient = UserPoolClient.Builder.create(this, "Client")
* .userPool(userPool)
* // Required minimal configuration for use with an ELB
* .generateSecret(true)
* .authFlows(AuthFlow.builder()
* .userPassword(true)
* .build())
* .oAuth(OAuthSettings.builder()
* .flows(OAuthFlows.builder()
* .authorizationCodeGrant(true)
* .build())
* .scopes(List.of(OAuthScope.EMAIL))
* .callbackUrls(List.of(String.format("https://%s/oauth2/idpresponse", lb.getLoadBalancerDnsName())))
* .build())
* .build();
* CfnUserPoolClient cfnClient = (CfnUserPoolClient)userPoolClient.getNode().getDefaultChild();
* cfnClient.addPropertyOverride("RefreshTokenValidity", 1);
* cfnClient.addPropertyOverride("SupportedIdentityProviders", List.of("COGNITO"));
* UserPoolDomain userPoolDomain = UserPoolDomain.Builder.create(this, "Domain")
* .userPool(userPool)
* .cognitoDomain(CognitoDomainOptions.builder()
* .domainPrefix("test-cdk-prefix")
* .build())
* .build();
* lb.addListener("Listener", BaseApplicationListenerProps.builder()
* .port(443)
* .certificates(List.of(certificate))
* .defaultAction(AuthenticateCognitoAction.Builder.create()
* .userPool(userPool)
* .userPoolClient(userPoolClient)
* .userPoolDomain(userPoolDomain)
* .next(ListenerAction.fixedResponse(200, FixedResponseOptions.builder()
* .contentType("text/plain")
* .messageBody("Authenticated")
* .build()))
* .build())
* .build());
* CfnOutput.Builder.create(this, "DNS")
* .value(lb.getLoadBalancerDnsName())
* .build();
* App app = new App();
* new CognitoStack(app, "integ-cognito");
* app.synth();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-31T18:44:17.075Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.elasticloadbalancingv2.actions.$Module.class, fqn = "@aws-cdk/aws-elasticloadbalancingv2-actions.AuthenticateCognitoActionProps")
@software.amazon.jsii.Jsii.Proxy(AuthenticateCognitoActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AuthenticateCognitoActionProps extends software.amazon.jsii.JsiiSerializable {
/**
* What action to execute next.
*
* Multiple actions form a linked chain; the chain must always terminate in a
* (weighted)forward, fixedResponse or redirect action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction getNext();
/**
* The Amazon Cognito user pool.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.IUserPool getUserPool();
/**
* The Amazon Cognito user pool client.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.IUserPoolClient getUserPoolClient();
/**
* The domain prefix or fully-qualified domain name of the Amazon Cognito user pool.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.IUserPoolDomain getUserPoolDomain();
/**
* The query parameters (up to 10) to include in the redirect request to the authorization endpoint.
*
* Default: - No extra parameters
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getAuthenticationRequestExtraParams() {
return null;
}
/**
* The behavior if the user is not authenticated.
*
* Default: UnauthenticatedAction.AUTHENTICATE
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction getOnUnauthenticatedRequest() {
return null;
}
/**
* The set of user claims to be requested from the IdP.
*
* To verify which scope values your IdP supports and how to separate multiple values, see the documentation for your IdP.
*
* Default: "openid"
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getScope() {
return null;
}
/**
* The name of the cookie used to maintain session information.
*
* Default: "AWSELBAuthSessionCookie"
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSessionCookieName() {
return null;
}
/**
* The maximum duration of the authentication session.
*
* Default: Duration.days(7)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getSessionTimeout() {
return null;
}
/**
* @return a {@link Builder} of {@link AuthenticateCognitoActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AuthenticateCognitoActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction next;
software.amazon.awscdk.services.cognito.IUserPool userPool;
software.amazon.awscdk.services.cognito.IUserPoolClient userPoolClient;
software.amazon.awscdk.services.cognito.IUserPoolDomain userPoolDomain;
java.util.Map authenticationRequestExtraParams;
software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction onUnauthenticatedRequest;
java.lang.String scope;
java.lang.String sessionCookieName;
software.amazon.awscdk.core.Duration sessionTimeout;
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getNext}
* @param next What action to execute next. This parameter is required.
* Multiple actions form a linked chain; the chain must always terminate in a
* (weighted)forward, fixedResponse or redirect action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder next(software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction next) {
this.next = next;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getUserPool}
* @param userPool The Amazon Cognito user pool. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userPool(software.amazon.awscdk.services.cognito.IUserPool userPool) {
this.userPool = userPool;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getUserPoolClient}
* @param userPoolClient The Amazon Cognito user pool client. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userPoolClient(software.amazon.awscdk.services.cognito.IUserPoolClient userPoolClient) {
this.userPoolClient = userPoolClient;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getUserPoolDomain}
* @param userPoolDomain The domain prefix or fully-qualified domain name of the Amazon Cognito user pool. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userPoolDomain(software.amazon.awscdk.services.cognito.IUserPoolDomain userPoolDomain) {
this.userPoolDomain = userPoolDomain;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getAuthenticationRequestExtraParams}
* @param authenticationRequestExtraParams The query parameters (up to 10) to include in the redirect request to the authorization endpoint.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authenticationRequestExtraParams(java.util.Map authenticationRequestExtraParams) {
this.authenticationRequestExtraParams = authenticationRequestExtraParams;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getOnUnauthenticatedRequest}
* @param onUnauthenticatedRequest The behavior if the user is not authenticated.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onUnauthenticatedRequest(software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction onUnauthenticatedRequest) {
this.onUnauthenticatedRequest = onUnauthenticatedRequest;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getScope}
* @param scope The set of user claims to be requested from the IdP.
* To verify which scope values your IdP supports and how to separate multiple values, see the documentation for your IdP.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scope(java.lang.String scope) {
this.scope = scope;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getSessionCookieName}
* @param sessionCookieName The name of the cookie used to maintain session information.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sessionCookieName(java.lang.String sessionCookieName) {
this.sessionCookieName = sessionCookieName;
return this;
}
/**
* Sets the value of {@link AuthenticateCognitoActionProps#getSessionTimeout}
* @param sessionTimeout The maximum duration of the authentication session.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sessionTimeout(software.amazon.awscdk.core.Duration sessionTimeout) {
this.sessionTimeout = sessionTimeout;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AuthenticateCognitoActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AuthenticateCognitoActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AuthenticateCognitoActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AuthenticateCognitoActionProps {
private final software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction next;
private final software.amazon.awscdk.services.cognito.IUserPool userPool;
private final software.amazon.awscdk.services.cognito.IUserPoolClient userPoolClient;
private final software.amazon.awscdk.services.cognito.IUserPoolDomain userPoolDomain;
private final java.util.Map authenticationRequestExtraParams;
private final software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction onUnauthenticatedRequest;
private final java.lang.String scope;
private final java.lang.String sessionCookieName;
private final software.amazon.awscdk.core.Duration sessionTimeout;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.next = software.amazon.jsii.Kernel.get(this, "next", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction.class));
this.userPool = software.amazon.jsii.Kernel.get(this, "userPool", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.IUserPool.class));
this.userPoolClient = software.amazon.jsii.Kernel.get(this, "userPoolClient", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.IUserPoolClient.class));
this.userPoolDomain = software.amazon.jsii.Kernel.get(this, "userPoolDomain", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.IUserPoolDomain.class));
this.authenticationRequestExtraParams = software.amazon.jsii.Kernel.get(this, "authenticationRequestExtraParams", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.onUnauthenticatedRequest = software.amazon.jsii.Kernel.get(this, "onUnauthenticatedRequest", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction.class));
this.scope = software.amazon.jsii.Kernel.get(this, "scope", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sessionCookieName = software.amazon.jsii.Kernel.get(this, "sessionCookieName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.sessionTimeout = software.amazon.jsii.Kernel.get(this, "sessionTimeout", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.next = java.util.Objects.requireNonNull(builder.next, "next is required");
this.userPool = java.util.Objects.requireNonNull(builder.userPool, "userPool is required");
this.userPoolClient = java.util.Objects.requireNonNull(builder.userPoolClient, "userPoolClient is required");
this.userPoolDomain = java.util.Objects.requireNonNull(builder.userPoolDomain, "userPoolDomain is required");
this.authenticationRequestExtraParams = builder.authenticationRequestExtraParams;
this.onUnauthenticatedRequest = builder.onUnauthenticatedRequest;
this.scope = builder.scope;
this.sessionCookieName = builder.sessionCookieName;
this.sessionTimeout = builder.sessionTimeout;
}
@Override
public final software.amazon.awscdk.services.elasticloadbalancingv2.ListenerAction getNext() {
return this.next;
}
@Override
public final software.amazon.awscdk.services.cognito.IUserPool getUserPool() {
return this.userPool;
}
@Override
public final software.amazon.awscdk.services.cognito.IUserPoolClient getUserPoolClient() {
return this.userPoolClient;
}
@Override
public final software.amazon.awscdk.services.cognito.IUserPoolDomain getUserPoolDomain() {
return this.userPoolDomain;
}
@Override
public final java.util.Map getAuthenticationRequestExtraParams() {
return this.authenticationRequestExtraParams;
}
@Override
public final software.amazon.awscdk.services.elasticloadbalancingv2.UnauthenticatedAction getOnUnauthenticatedRequest() {
return this.onUnauthenticatedRequest;
}
@Override
public final java.lang.String getScope() {
return this.scope;
}
@Override
public final java.lang.String getSessionCookieName() {
return this.sessionCookieName;
}
@Override
public final software.amazon.awscdk.core.Duration getSessionTimeout() {
return this.sessionTimeout;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("next", om.valueToTree(this.getNext()));
data.set("userPool", om.valueToTree(this.getUserPool()));
data.set("userPoolClient", om.valueToTree(this.getUserPoolClient()));
data.set("userPoolDomain", om.valueToTree(this.getUserPoolDomain()));
if (this.getAuthenticationRequestExtraParams() != null) {
data.set("authenticationRequestExtraParams", om.valueToTree(this.getAuthenticationRequestExtraParams()));
}
if (this.getOnUnauthenticatedRequest() != null) {
data.set("onUnauthenticatedRequest", om.valueToTree(this.getOnUnauthenticatedRequest()));
}
if (this.getScope() != null) {
data.set("scope", om.valueToTree(this.getScope()));
}
if (this.getSessionCookieName() != null) {
data.set("sessionCookieName", om.valueToTree(this.getSessionCookieName()));
}
if (this.getSessionTimeout() != null) {
data.set("sessionTimeout", om.valueToTree(this.getSessionTimeout()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-elasticloadbalancingv2-actions.AuthenticateCognitoActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AuthenticateCognitoActionProps.Jsii$Proxy that = (AuthenticateCognitoActionProps.Jsii$Proxy) o;
if (!next.equals(that.next)) return false;
if (!userPool.equals(that.userPool)) return false;
if (!userPoolClient.equals(that.userPoolClient)) return false;
if (!userPoolDomain.equals(that.userPoolDomain)) return false;
if (this.authenticationRequestExtraParams != null ? !this.authenticationRequestExtraParams.equals(that.authenticationRequestExtraParams) : that.authenticationRequestExtraParams != null) return false;
if (this.onUnauthenticatedRequest != null ? !this.onUnauthenticatedRequest.equals(that.onUnauthenticatedRequest) : that.onUnauthenticatedRequest != null) return false;
if (this.scope != null ? !this.scope.equals(that.scope) : that.scope != null) return false;
if (this.sessionCookieName != null ? !this.sessionCookieName.equals(that.sessionCookieName) : that.sessionCookieName != null) return false;
return this.sessionTimeout != null ? this.sessionTimeout.equals(that.sessionTimeout) : that.sessionTimeout == null;
}
@Override
public final int hashCode() {
int result = this.next.hashCode();
result = 31 * result + (this.userPool.hashCode());
result = 31 * result + (this.userPoolClient.hashCode());
result = 31 * result + (this.userPoolDomain.hashCode());
result = 31 * result + (this.authenticationRequestExtraParams != null ? this.authenticationRequestExtraParams.hashCode() : 0);
result = 31 * result + (this.onUnauthenticatedRequest != null ? this.onUnauthenticatedRequest.hashCode() : 0);
result = 31 * result + (this.scope != null ? this.scope.hashCode() : 0);
result = 31 * result + (this.sessionCookieName != null ? this.sessionCookieName.hashCode() : 0);
result = 31 * result + (this.sessionTimeout != null ? this.sessionTimeout.hashCode() : 0);
return result;
}
}
}