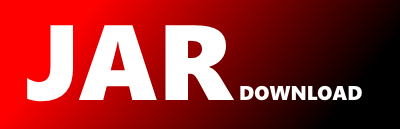
software.amazon.awscdk.services.elasticloadbalancingv2.AddApplicationTargetsProps Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
package software.amazon.awscdk.services.elasticloadbalancingv2;
/**
* Properties for adding new targets to a listener.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2020-12-12T01:56:36.174Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.elasticloadbalancingv2.$Module.class, fqn = "@aws-cdk/aws-elasticloadbalancingv2.AddApplicationTargetsProps")
@software.amazon.jsii.Jsii.Proxy(AddApplicationTargetsProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AddApplicationTargetsProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.elasticloadbalancingv2.AddRuleProps {
/**
* The amount of time for Elastic Load Balancing to wait before deregistering a target.
*
* The range is 0-3600 seconds.
*
* Default: Duration.minutes(5)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getDeregistrationDelay() {
return null;
}
/**
* Health check configuration.
*
* Default: No health check
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck getHealthCheck() {
return null;
}
/**
* The port on which the listener listens for requests.
*
* Default: Determined from protocol if known
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getPort() {
return null;
}
/**
* The protocol to use.
*
* Default: Determined from port if known
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol getProtocol() {
return null;
}
/**
* The time period during which the load balancer sends a newly registered target a linearly increasing share of the traffic to the target group.
*
* The range is 30-900 seconds (15 minutes).
*
* Default: 0
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getSlowStart() {
return null;
}
/**
* The stickiness cookie expiration period.
*
* Setting this value enables load balancer stickiness.
*
* After this period, the cookie is considered stale. The minimum value is
* 1 second and the maximum value is 7 days (604800 seconds).
*
* Default: Stickiness disabled
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getStickinessCookieDuration() {
return null;
}
/**
* The name of the target group.
*
* This name must be unique per region per account, can have a maximum of
* 32 characters, must contain only alphanumeric characters or hyphens, and
* must not begin or end with a hyphen.
*
* Default: Automatically generated
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getTargetGroupName() {
return null;
}
/**
* The targets to add to this target group.
*
* Can be Instance
, IPAddress
, or any self-registering load balancing
* target. All target must be of the same type.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTargets() {
return null;
}
/**
* @return a {@link Builder} of {@link AddApplicationTargetsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AddApplicationTargetsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private software.amazon.awscdk.core.Duration deregistrationDelay;
private software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck healthCheck;
private java.lang.Number port;
private software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol protocol;
private software.amazon.awscdk.core.Duration slowStart;
private software.amazon.awscdk.core.Duration stickinessCookieDuration;
private java.lang.String targetGroupName;
private java.util.List targets;
private java.util.List conditions;
private java.lang.String hostHeader;
private java.lang.String pathPattern;
private java.util.List pathPatterns;
private java.lang.Number priority;
/**
* Sets the value of {@link AddApplicationTargetsProps#getDeregistrationDelay}
* @param deregistrationDelay The amount of time for Elastic Load Balancing to wait before deregistering a target.
* The range is 0-3600 seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deregistrationDelay(software.amazon.awscdk.core.Duration deregistrationDelay) {
this.deregistrationDelay = deregistrationDelay;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getHealthCheck}
* @param healthCheck Health check configuration.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheck(software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck healthCheck) {
this.healthCheck = healthCheck;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getPort}
* @param port The port on which the listener listens for requests.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getProtocol}
* @param protocol The protocol to use.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder protocol(software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol protocol) {
this.protocol = protocol;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getSlowStart}
* @param slowStart The time period during which the load balancer sends a newly registered target a linearly increasing share of the traffic to the target group.
* The range is 30-900 seconds (15 minutes).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder slowStart(software.amazon.awscdk.core.Duration slowStart) {
this.slowStart = slowStart;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getStickinessCookieDuration}
* @param stickinessCookieDuration The stickiness cookie expiration period.
* Setting this value enables load balancer stickiness.
*
* After this period, the cookie is considered stale. The minimum value is
* 1 second and the maximum value is 7 days (604800 seconds).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stickinessCookieDuration(software.amazon.awscdk.core.Duration stickinessCookieDuration) {
this.stickinessCookieDuration = stickinessCookieDuration;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getTargetGroupName}
* @param targetGroupName The name of the target group.
* This name must be unique per region per account, can have a maximum of
* 32 characters, must contain only alphanumeric characters or hyphens, and
* must not begin or end with a hyphen.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder targetGroupName(java.lang.String targetGroupName) {
this.targetGroupName = targetGroupName;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getTargets}
* @param targets The targets to add to this target group.
* Can be Instance
, IPAddress
, or any self-registering load balancing
* target. All target must be of the same type.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder targets(java.util.List extends software.amazon.awscdk.services.elasticloadbalancingv2.IApplicationLoadBalancerTarget> targets) {
this.targets = (java.util.List)targets;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getConditions}
* @param conditions Rule applies if matches the conditions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder conditions(java.util.List extends software.amazon.awscdk.services.elasticloadbalancingv2.ListenerCondition> conditions) {
this.conditions = (java.util.List)conditions;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getHostHeader}
* @param hostHeader Rule applies if the requested host matches the indicated host.
* May contain up to three '*' wildcards.
*
* Requires that priority is set.
* @return {@code this}
* @deprecated Use `conditions` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder hostHeader(java.lang.String hostHeader) {
this.hostHeader = hostHeader;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getPathPattern}
* @param pathPattern Rule applies if the requested path matches the given path pattern.
* May contain up to three '*' wildcards.
*
* Requires that priority is set.
* @return {@code this}
* @deprecated Use `conditions` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder pathPattern(java.lang.String pathPattern) {
this.pathPattern = pathPattern;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getPathPatterns}
* @param pathPatterns Rule applies if the requested path matches any of the given patterns.
* May contain up to three '*' wildcards.
*
* Requires that priority is set.
* @return {@code this}
* @deprecated Use `conditions` instead.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder pathPatterns(java.util.List pathPatterns) {
this.pathPatterns = pathPatterns;
return this;
}
/**
* Sets the value of {@link AddApplicationTargetsProps#getPriority}
* @param priority Priority of this target group.
* The rule with the lowest priority will be used for every request.
* If priority is not given, these target groups will be added as
* defaults, and must not have conditions.
*
* Priorities must be unique.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder priority(java.lang.Number priority) {
this.priority = priority;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AddApplicationTargetsProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AddApplicationTargetsProps build() {
return new Jsii$Proxy(deregistrationDelay, healthCheck, port, protocol, slowStart, stickinessCookieDuration, targetGroupName, targets, conditions, hostHeader, pathPattern, pathPatterns, priority);
}
}
/**
* An implementation for {@link AddApplicationTargetsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AddApplicationTargetsProps {
private final software.amazon.awscdk.core.Duration deregistrationDelay;
private final software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck healthCheck;
private final java.lang.Number port;
private final software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol protocol;
private final software.amazon.awscdk.core.Duration slowStart;
private final software.amazon.awscdk.core.Duration stickinessCookieDuration;
private final java.lang.String targetGroupName;
private final java.util.List targets;
private final java.util.List conditions;
private final java.lang.String hostHeader;
private final java.lang.String pathPattern;
private final java.util.List pathPatterns;
private final java.lang.Number priority;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.deregistrationDelay = software.amazon.jsii.Kernel.get(this, "deregistrationDelay", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.healthCheck = software.amazon.jsii.Kernel.get(this, "healthCheck", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.protocol = software.amazon.jsii.Kernel.get(this, "protocol", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol.class));
this.slowStart = software.amazon.jsii.Kernel.get(this, "slowStart", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.stickinessCookieDuration = software.amazon.jsii.Kernel.get(this, "stickinessCookieDuration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.targetGroupName = software.amazon.jsii.Kernel.get(this, "targetGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.targets = software.amazon.jsii.Kernel.get(this, "targets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.IApplicationLoadBalancerTarget.class)));
this.conditions = software.amazon.jsii.Kernel.get(this, "conditions", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.elasticloadbalancingv2.ListenerCondition.class)));
this.hostHeader = software.amazon.jsii.Kernel.get(this, "hostHeader", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.pathPattern = software.amazon.jsii.Kernel.get(this, "pathPattern", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.pathPatterns = software.amazon.jsii.Kernel.get(this, "pathPatterns", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.priority = software.amazon.jsii.Kernel.get(this, "priority", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final software.amazon.awscdk.core.Duration deregistrationDelay, final software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck healthCheck, final java.lang.Number port, final software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol protocol, final software.amazon.awscdk.core.Duration slowStart, final software.amazon.awscdk.core.Duration stickinessCookieDuration, final java.lang.String targetGroupName, final java.util.List extends software.amazon.awscdk.services.elasticloadbalancingv2.IApplicationLoadBalancerTarget> targets, final java.util.List extends software.amazon.awscdk.services.elasticloadbalancingv2.ListenerCondition> conditions, final java.lang.String hostHeader, final java.lang.String pathPattern, final java.util.List pathPatterns, final java.lang.Number priority) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.deregistrationDelay = deregistrationDelay;
this.healthCheck = healthCheck;
this.port = port;
this.protocol = protocol;
this.slowStart = slowStart;
this.stickinessCookieDuration = stickinessCookieDuration;
this.targetGroupName = targetGroupName;
this.targets = (java.util.List)targets;
this.conditions = (java.util.List)conditions;
this.hostHeader = hostHeader;
this.pathPattern = pathPattern;
this.pathPatterns = pathPatterns;
this.priority = priority;
}
@Override
public final software.amazon.awscdk.core.Duration getDeregistrationDelay() {
return this.deregistrationDelay;
}
@Override
public final software.amazon.awscdk.services.elasticloadbalancingv2.HealthCheck getHealthCheck() {
return this.healthCheck;
}
@Override
public final java.lang.Number getPort() {
return this.port;
}
@Override
public final software.amazon.awscdk.services.elasticloadbalancingv2.ApplicationProtocol getProtocol() {
return this.protocol;
}
@Override
public final software.amazon.awscdk.core.Duration getSlowStart() {
return this.slowStart;
}
@Override
public final software.amazon.awscdk.core.Duration getStickinessCookieDuration() {
return this.stickinessCookieDuration;
}
@Override
public final java.lang.String getTargetGroupName() {
return this.targetGroupName;
}
@Override
public final java.util.List getTargets() {
return this.targets;
}
@Override
public final java.util.List getConditions() {
return this.conditions;
}
@Override
public final java.lang.String getHostHeader() {
return this.hostHeader;
}
@Override
public final java.lang.String getPathPattern() {
return this.pathPattern;
}
@Override
public final java.util.List getPathPatterns() {
return this.pathPatterns;
}
@Override
public final java.lang.Number getPriority() {
return this.priority;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDeregistrationDelay() != null) {
data.set("deregistrationDelay", om.valueToTree(this.getDeregistrationDelay()));
}
if (this.getHealthCheck() != null) {
data.set("healthCheck", om.valueToTree(this.getHealthCheck()));
}
if (this.getPort() != null) {
data.set("port", om.valueToTree(this.getPort()));
}
if (this.getProtocol() != null) {
data.set("protocol", om.valueToTree(this.getProtocol()));
}
if (this.getSlowStart() != null) {
data.set("slowStart", om.valueToTree(this.getSlowStart()));
}
if (this.getStickinessCookieDuration() != null) {
data.set("stickinessCookieDuration", om.valueToTree(this.getStickinessCookieDuration()));
}
if (this.getTargetGroupName() != null) {
data.set("targetGroupName", om.valueToTree(this.getTargetGroupName()));
}
if (this.getTargets() != null) {
data.set("targets", om.valueToTree(this.getTargets()));
}
if (this.getConditions() != null) {
data.set("conditions", om.valueToTree(this.getConditions()));
}
if (this.getHostHeader() != null) {
data.set("hostHeader", om.valueToTree(this.getHostHeader()));
}
if (this.getPathPattern() != null) {
data.set("pathPattern", om.valueToTree(this.getPathPattern()));
}
if (this.getPathPatterns() != null) {
data.set("pathPatterns", om.valueToTree(this.getPathPatterns()));
}
if (this.getPriority() != null) {
data.set("priority", om.valueToTree(this.getPriority()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-elasticloadbalancingv2.AddApplicationTargetsProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AddApplicationTargetsProps.Jsii$Proxy that = (AddApplicationTargetsProps.Jsii$Proxy) o;
if (this.deregistrationDelay != null ? !this.deregistrationDelay.equals(that.deregistrationDelay) : that.deregistrationDelay != null) return false;
if (this.healthCheck != null ? !this.healthCheck.equals(that.healthCheck) : that.healthCheck != null) return false;
if (this.port != null ? !this.port.equals(that.port) : that.port != null) return false;
if (this.protocol != null ? !this.protocol.equals(that.protocol) : that.protocol != null) return false;
if (this.slowStart != null ? !this.slowStart.equals(that.slowStart) : that.slowStart != null) return false;
if (this.stickinessCookieDuration != null ? !this.stickinessCookieDuration.equals(that.stickinessCookieDuration) : that.stickinessCookieDuration != null) return false;
if (this.targetGroupName != null ? !this.targetGroupName.equals(that.targetGroupName) : that.targetGroupName != null) return false;
if (this.targets != null ? !this.targets.equals(that.targets) : that.targets != null) return false;
if (this.conditions != null ? !this.conditions.equals(that.conditions) : that.conditions != null) return false;
if (this.hostHeader != null ? !this.hostHeader.equals(that.hostHeader) : that.hostHeader != null) return false;
if (this.pathPattern != null ? !this.pathPattern.equals(that.pathPattern) : that.pathPattern != null) return false;
if (this.pathPatterns != null ? !this.pathPatterns.equals(that.pathPatterns) : that.pathPatterns != null) return false;
return this.priority != null ? this.priority.equals(that.priority) : that.priority == null;
}
@Override
public final int hashCode() {
int result = this.deregistrationDelay != null ? this.deregistrationDelay.hashCode() : 0;
result = 31 * result + (this.healthCheck != null ? this.healthCheck.hashCode() : 0);
result = 31 * result + (this.port != null ? this.port.hashCode() : 0);
result = 31 * result + (this.protocol != null ? this.protocol.hashCode() : 0);
result = 31 * result + (this.slowStart != null ? this.slowStart.hashCode() : 0);
result = 31 * result + (this.stickinessCookieDuration != null ? this.stickinessCookieDuration.hashCode() : 0);
result = 31 * result + (this.targetGroupName != null ? this.targetGroupName.hashCode() : 0);
result = 31 * result + (this.targets != null ? this.targets.hashCode() : 0);
result = 31 * result + (this.conditions != null ? this.conditions.hashCode() : 0);
result = 31 * result + (this.hostHeader != null ? this.hostHeader.hashCode() : 0);
result = 31 * result + (this.pathPattern != null ? this.pathPattern.hashCode() : 0);
result = 31 * result + (this.pathPatterns != null ? this.pathPatterns.hashCode() : 0);
result = 31 * result + (this.priority != null ? this.priority.hashCode() : 0);
return result;
}
}
}