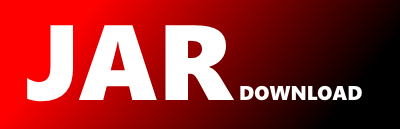
software.amazon.awscdk.services.elasticloadbalancingv2.package-info Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/**
* Amazon Elastic Load Balancing V2 Construct Library
*
* ---
*
*
*
*
*
*
*
*
*
* The @aws-cdk/aws-elasticloadbalancingv2
package provides constructs for
* configuring application and network load balancers.
*
* For more information, see the AWS documentation for
* Application Load Balancers
* and Network Load Balancers.
*
*
Defining an Application Load Balancer
*
* You define an application load balancer by creating an instance of
* ApplicationLoadBalancer
, adding a Listener to the load balancer
* and adding Targets to the Listener:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* import software.amazon.awscdk.services.ec2.*;
* import software.amazon.awscdk.services.elasticloadbalancingv2.*;
* import software.amazon.awscdk.services.autoscaling.AutoScalingGroup;
*
* // ...
*
* Vpc vpc = new Vpc(...);
*
* // Create the load balancer in a VPC. 'internetFacing' is 'false'
* // by default, which creates an internal load balancer.
* ApplicationLoadBalancer lb = new ApplicationLoadBalancer(this, "LB", new ApplicationLoadBalancerProps()
* .vpc(vpc)
* .internetFacing(true));
*
* // Add a listener and open up the load balancer's security group
* // to the world.
* ApplicationListener listener = lb.addListener("Listener", new BaseApplicationListenerProps()
* .port(80)
*
* // 'open: true' is the default, you can leave it out if you want. Set it
* // to 'false' and use `listener.connections` if you want to be selective
* // about who can access the load balancer.
* .open(true));
*
* // Create an AutoScaling group and add it as a load balancing
* // target to the listener.
* AutoScalingGroup asg = new AutoScalingGroup(...);
* listener.addTargets("ApplicationFleet", new AddApplicationTargetsProps()
* .port(8080)
* .targets(asList(asg)));
*
*
* The security groups of the load balancer and the target are automatically
* updated to allow the network traffic.
*
* One (or more) security groups can be associated with the load balancer;
* if a security group isn't provided, one will be automatically created.
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object securityGroup1 = SecurityGroup.Builder.create(stack, "SecurityGroup1").vpc(vpc).build();
* Object lb = ApplicationLoadBalancer.Builder.create(this, "LB")
* .vpc(vpc)
* .internetFacing(true)
* .securityGroup(securityGroup1)
* .build();
*
* Object securityGroup2 = SecurityGroup.Builder.create(stack, "SecurityGroup2").vpc(vpc).build();
* lb.addSecurityGroup(securityGroup2);
*
*
*
Conditions
*
* It's possible to route traffic to targets based on conditions in the incoming
* HTTP request. For example, the following will route requests to the indicated
* AutoScalingGroup only if the requested host in the request is either for
* example.com/ok
or example.com/path
:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* listener.addTargets("Example.Com Fleet", Map.of(
* "priority", 10,
* "conditions", asList(ListenerCondition.hostHeaders(asList("example.com")), ListenerCondition.pathPatterns(asList("/ok", "/path"))),
* "port", 8080,
* "targets", asList(asg)));
*
*
* A target with a condition contains either pathPatterns
or hostHeader
, or
* both. If both are specified, both conditions must be met for the requests to
* be routed to the given target. priority
is a required field when you add
* targets with conditions. The lowest number wins.
*
* Every listener must have at least one target without conditions, which is
* where all requests that didn't match any of the conditions will be sent.
*
*
Convenience methods and more complex Actions
*
* Routing traffic from a Load Balancer to a Target involves the following steps:
*
*
* - Create a Target Group, register the Target into the Target Group
* - Add an Action to the Listener which forwards traffic to the Target Group.
*
*
* Various methods on the Listener
take care of this work for you to a greater
* or lesser extent:
*
*
* addTargets()
performs both steps: automatically creates a Target Group and the
* required Action.
* addTargetGroups()
gives you more control: you create the Target Group (or
* Target Groups) yourself and the method creates Action that routes traffic to
* the Target Groups.
* addAction()
gives you full control: you supply the Action and wire it up
* to the Target Groups yourself (or access one of the other ELB routing features).
*
*
* Using addAction()
gives you access to some of the features of an Elastic Load
* Balancer that the other two convenience methods don't:
*
*
* - Routing stickiness: use
ListenerAction.forward()
and supply a
* stickinessDuration
to make sure requests are routed to the same target group
* for a given duration.
* - Weighted Target Groups: use
ListenerAction.weightedForward()
* to give different weights to different target groups.
* - Fixed Responses: use
ListenerAction.fixedResponse()
to serve
* a static response (ALB only).
* - Redirects: use
ListenerAction.redirect()
to serve an HTTP
* redirect response (ALB only).
* - Authentication: use
ListenerAction.authenticateOidc()
to
* perform OpenID authentication before serving a request (see the
* @aws-cdk/aws-elasticloadbalancingv2-actions
package for direct authentication
* integration with Cognito) (ALB only).
*
*
* Here's an example of serving a fixed response at the /ok
URL:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* listener.addAction("Fixed", Map.of(
* "priority", 10,
* "conditions", asList(ListenerCondition.pathPatterns(asList("/ok"))),
* "action", ListenerAction.fixedResponse(200, Map.of(
* "contentType", elbv2.ContentType.getTEXT_PLAIN(),
* "messageBody", "OK"))));
*
*
* Here's an example of using OIDC authentication before forwarding to a TargetGroup:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* listener.addAction("DefaultAction", Map.of(
* "action", ListenerAction.authenticateOidc(Map.of(
* "authorizationEndpoint", "https://example.com/openid",
* // Other OIDC properties here
* // ...
* "next", ListenerAction.forward(asList(myTargetGroup))))));
*
*
* If you just want to redirect all incoming traffic on one port to another port, you can use the following code:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* lb.addRedirect(Map.of(
* "sourceProtocol", elbv2.ApplicationProtocol.getHTTPS(),
* "sourcePort", 8443,
* "targetProtocol", elbv2.ApplicationProtocol.getHTTP(),
* "targetPort", 8080));
*
*
* If you do not provide any options for this method, it redirects HTTP port 80 to HTTPS port 443.
*
*
Defining a Network Load Balancer
*
* Network Load Balancers are defined in a similar way to Application Load
* Balancers:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* import software.amazon.awscdk.services.ec2.*;
* import software.amazon.awscdk.services.elasticloadbalancingv2.*;
* import software.amazon.awscdk.services.autoscaling.*;
*
* // Create the load balancer in a VPC. 'internetFacing' is 'false'
* // by default, which creates an internal load balancer.
* NetworkLoadBalancer lb = new NetworkLoadBalancer(this, "LB", new NetworkLoadBalancerProps()
* .vpc(vpc)
* .internetFacing(true));
*
* // Add a listener on a particular port.
* NetworkListener listener = lb.addListener("Listener", new BaseNetworkListenerProps()
* .port(443));
*
* // Add targets on a particular port.
* listener.addTargets("AppFleet", new AddNetworkTargetsProps()
* .port(443)
* .targets(asList(asg)));
*
*
* One thing to keep in mind is that network load balancers do not have security
* groups, and no automatic security group configuration is done for you. You will
* have to configure the security groups of the target yourself to allow traffic by
* clients and/or load balancer instances, depending on your target types. See
* Target Groups for your Network Load
* Balancers
* and Register targets with your Target
* Group
* for more information.
*
*
Targets and Target Groups
*
* Application and Network Load Balancers organize load balancing targets in Target
* Groups. If you add your balancing targets (such as AutoScalingGroups, ECS
* services or individual instances) to your listener directly, the appropriate
* TargetGroup
will be automatically created for you.
*
* If you need more control over the Target Groups created, create an instance of
* ApplicationTargetGroup
or NetworkTargetGroup
, add the members you desire,
* and add it to the listener by calling addTargetGroups
instead of addTargets
.
*
* addTargets()
will always return the Target Group it just created for you:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object group = listener.addTargets("AppFleet", Map.of(
* "port", 443,
* "targets", asList(asg1)));
*
* group.addTarget(asg2);
*
*
*
Using Lambda Targets
*
* To use a Lambda Function as a target, use the integration class in the
* @aws-cdk/aws-elasticloadbalancingv2-targets
package:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* import software.amazon.awscdk.services.lambda.*;
* import software.amazon.awscdk.services.elasticloadbalancingv2.*;
* import software.amazon.awscdk.services.elasticloadbalancingv2.targets.*;
*
* Function lambdaFunction = new Function(...);
* ApplicationLoadBalancer lb = new ApplicationLoadBalancer(...);
*
* ApplicationListener listener = lb.addListener("Listener", new BaseApplicationListenerProps().port(80));
* listener.addTargets("Targets", new AddApplicationTargetsProps()
* .targets(asList(new LambdaTarget(lambdaFunction)))
*
* // For Lambda Targets, you need to explicitly enable health checks if you
* // want them.
* .healthCheck(new HealthCheck()
* .enabled(true)));
*
*
* Only a single Lambda function can be added to a single listener rule.
*
*
Configuring Health Checks
*
* Health checks are configured upon creation of a target group:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* listener.addTargets("AppFleet", Map.of(
* "port", 8080,
* "targets", asList(asg),
* "healthCheck", Map.of(
* "path", "/ping",
* "interval", cdk.Duration.minutes(1))));
*
*
* The health check can also be configured after creation by calling
* configureHealthCheck()
on the created object.
*
* No attempts are made to configure security groups for the port you're
* configuring a health check for, but if the health check is on the same port
* you're routing traffic to, the security group already allows the traffic.
* If not, you will have to configure the security groups appropriately:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* listener.addTargets("AppFleet", Map.of(
* "port", 8080,
* "targets", asList(asg),
* "healthCheck", Map.of(
* "port", 8088)));
*
* listener.connections.allowFrom(lb, ec2.Port.tcp(8088));
*
*
*
Using a Load Balancer from a different Stack
*
* If you want to put your Load Balancer and the Targets it is load balancing to in
* different stacks, you may not be able to use the convenience methods
* loadBalancer.addListener()
and listener.addTargets()
.
*
* The reason is that these methods will create resources in the same Stack as the
* object they're called on, which may lead to cyclic references between stacks.
* Instead, you will have to create an ApplicationListener
in the target stack,
* or an empty TargetGroup
in the load balancer stack that you attach your
* service to.
*
* For an example of the alternatives while load balancing to an ECS service, see the
* ecs/cross-stack-load-balancer
* example.
*
*
Protocol for Load Balancer Targets
*
* Constructs that want to be a load balancer target should implement
* IApplicationLoadBalancerTarget
and/or INetworkLoadBalancerTarget
, and
* provide an implementation for the function attachToXxxTargetGroup()
, which can
* call functions on the load balancer and should return metadata about the
* load balancing target:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* attachToApplicationTargetGroup(targetGroup, ApplicationTargetGroup);LoadBalancerTargetProps;{
* targetGroup.registerConnectable(...);return Map.of(
* "targetType", TargetType.getInstance() | TargetType.getIp(),
* "targetJson", Map.of("id", , ..., "port", , ...))
* }
*
*
* targetType
should be one of Instance
or Ip
. If the target can be
* directly added to the target group, targetJson
should contain the id
of
* the target (either instance ID or IP address depending on the type) and
* optionally a port
or availabilityZone
override.
*
* Application load balancer targets can call registerConnectable()
on the
* target group to register themselves for addition to the load balancer's security
* group rules.
*
* If your load balancer target requires that the TargetGroup has been
* associated with a LoadBalancer before registration can happen (such as is the
* case for ECS Services for example), take a resource dependency on
* targetGroup.loadBalancerDependency()
as follows:
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* // Make sure that the listener has been created, and so the TargetGroup
* // has been associated with the LoadBalancer, before 'resource' is created.
* resourced.addDependency(targetGroup.loadBalancerDependency());
*
*
*
Looking up Load Balancers and Listeners
*
* You may look up load balancers and load balancer listeners by using one of the
* following lookup methods:
*
*
* ApplicationLoadBalancer.fromlookup(options)
- Look up an application load
* balancer.
* ApplicationListener.fromLookup(options)
- Look up an application load
* balancer listener.
* NetworkLoadBalancer.fromLookup(options)
- Look up a network load balancer.
* NetworkListener.fromLookup(options)
- Look up a network load balancer
* listener.
*
*
*
Load Balancer lookup options
*
* You may look up a load balancer by ARN or by associated tags. When you look a
* load balancer up by ARN, that load balancer will be returned unless CDK detects
* that the load balancer is of the wrong type. When you look up a load balancer by
* tags, CDK will return the load balancer matching all specified tags. If more
* than one load balancer matches, CDK will throw an error requesting that you
* provide more specific criteria.
*
* Look up a Application Load Balancer by ARN
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object loadBalancer = ApplicationLoadBalancer.fromLookup(stack, "ALB", Map.of(
* "loadBalancerArn", YOUR_ALB_ARN));
*
*
* Look up an Application Load Balancer by tags
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object loadBalancer = ApplicationLoadBalancer.fromLookup(stack, "ALB", Map.of(
* "loadBalancerTags", Map.of(
* // Finds a load balancer matching all tags.
* "some", "tag",
* "someother", "tag")));
*
*
*
Load Balancer Listener lookup options
*
* You may look up a load balancer listener by the following criteria:
*
*
* - Associated load balancer ARN
* - Associated load balancer tags
* - Listener ARN
* - Listener port
* - Listener protocol
*
*
* The lookup method will return the matching listener. If more than one listener
* matches, CDK will throw an error requesting that you specify additional
* criteria.
*
* Look up a Listener by associated Load Balancer, Port, and Protocol
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object listener = ApplicationListener.fromLookup(stack, "ALBListener", Map.of(
* "loadBalancerArn", YOUR_ALB_ARN,
* "listenerProtocol", ApplicationProtocol.getHTTPS(),
* "listenerPort", 443));
*
*
* Look up a Listener by associated Load Balancer Tag, Port, and Protocol
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object listener = ApplicationListener.fromLookup(stack, "ALBListener", Map.of(
* "loadBalancerTags", Map.of(
* "Cluster", "MyClusterName"),
* "listenerProtocol", ApplicationProtocol.getHTTPS(),
* "listenerPort", 443));
*
*
* Look up a Network Listener by associated Load Balancer Tag, Port, and Protocol
*
*
* // Example automatically generated without compilation. See https://github.com/aws/jsii/issues/826
* Object listener = NetworkListener.fromLookup(stack, "ALBListener", Map.of(
* "loadBalancerTags", Map.of(
* "Cluster", "MyClusterName"),
* "listenerProtocol", Protocol.getTCP(),
* "listenerPort", 12345));
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
package software.amazon.awscdk.services.elasticloadbalancingv2;