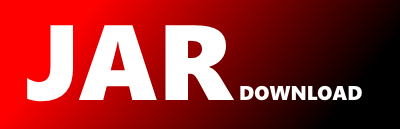
software.amazon.awscdk.services.events.targets.AwsApiInput Maven / Gradle / Ivy
Show all versions of events-targets Show documentation
package software.amazon.awscdk.services.events.targets;
/**
* Rule target input for an AwsApi target.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.30.0 (build adae23f)", date = "2021-07-09T19:18:30.457Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.events.targets.$Module.class, fqn = "@aws-cdk/aws-events-targets.AwsApiInput")
@software.amazon.jsii.Jsii.Proxy(AwsApiInput.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AwsApiInput extends software.amazon.jsii.JsiiSerializable {
/**
* The service action to call.
*
* @see https://docs.aws.amazon.com/AWSJavaScriptSDK/latest/index.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAction();
/**
* The service to call.
*
* @see https://docs.aws.amazon.com/AWSJavaScriptSDK/latest/index.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getService();
/**
* API version to use for the service.
*
* Default: - use latest available API version
*
* @see https://docs.aws.amazon.com/sdk-for-javascript/v2/developer-guide/locking-api-versions.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getApiVersion() {
return null;
}
/**
* The regex pattern to use to catch API errors.
*
* The code
property of the
* Error
object will be tested against this pattern. If there is a match an
* error will not be thrown.
*
* Default: - do not catch errors
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCatchErrorPattern() {
return null;
}
/**
* The parameters for the service action.
*
* Default: - no parameters
*
* @see https://docs.aws.amazon.com/AWSJavaScriptSDK/latest/index.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getParameters() {
return null;
}
/**
* @return a {@link Builder} of {@link AwsApiInput}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AwsApiInput}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String action;
private java.lang.String service;
private java.lang.String apiVersion;
private java.lang.String catchErrorPattern;
private java.lang.Object parameters;
/**
* Sets the value of {@link AwsApiInput#getAction}
* @param action The service action to call. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder action(java.lang.String action) {
this.action = action;
return this;
}
/**
* Sets the value of {@link AwsApiInput#getService}
* @param service The service to call. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder service(java.lang.String service) {
this.service = service;
return this;
}
/**
* Sets the value of {@link AwsApiInput#getApiVersion}
* @param apiVersion API version to use for the service.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder apiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* Sets the value of {@link AwsApiInput#getCatchErrorPattern}
* @param catchErrorPattern The regex pattern to use to catch API errors.
* The code
property of the
* Error
object will be tested against this pattern. If there is a match an
* error will not be thrown.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder catchErrorPattern(java.lang.String catchErrorPattern) {
this.catchErrorPattern = catchErrorPattern;
return this;
}
/**
* Sets the value of {@link AwsApiInput#getParameters}
* @param parameters The parameters for the service action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameters(java.lang.Object parameters) {
this.parameters = parameters;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AwsApiInput}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AwsApiInput build() {
return new Jsii$Proxy(action, service, apiVersion, catchErrorPattern, parameters);
}
}
/**
* An implementation for {@link AwsApiInput}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AwsApiInput {
private final java.lang.String action;
private final java.lang.String service;
private final java.lang.String apiVersion;
private final java.lang.String catchErrorPattern;
private final java.lang.Object parameters;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.action = software.amazon.jsii.Kernel.get(this, "action", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.service = software.amazon.jsii.Kernel.get(this, "service", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.apiVersion = software.amazon.jsii.Kernel.get(this, "apiVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.catchErrorPattern = software.amazon.jsii.Kernel.get(this, "catchErrorPattern", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.parameters = software.amazon.jsii.Kernel.get(this, "parameters", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.String action, final java.lang.String service, final java.lang.String apiVersion, final java.lang.String catchErrorPattern, final java.lang.Object parameters) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.action = java.util.Objects.requireNonNull(action, "action is required");
this.service = java.util.Objects.requireNonNull(service, "service is required");
this.apiVersion = apiVersion;
this.catchErrorPattern = catchErrorPattern;
this.parameters = parameters;
}
@Override
public final java.lang.String getAction() {
return this.action;
}
@Override
public final java.lang.String getService() {
return this.service;
}
@Override
public final java.lang.String getApiVersion() {
return this.apiVersion;
}
@Override
public final java.lang.String getCatchErrorPattern() {
return this.catchErrorPattern;
}
@Override
public final java.lang.Object getParameters() {
return this.parameters;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("action", om.valueToTree(this.getAction()));
data.set("service", om.valueToTree(this.getService()));
if (this.getApiVersion() != null) {
data.set("apiVersion", om.valueToTree(this.getApiVersion()));
}
if (this.getCatchErrorPattern() != null) {
data.set("catchErrorPattern", om.valueToTree(this.getCatchErrorPattern()));
}
if (this.getParameters() != null) {
data.set("parameters", om.valueToTree(this.getParameters()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-events-targets.AwsApiInput"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AwsApiInput.Jsii$Proxy that = (AwsApiInput.Jsii$Proxy) o;
if (!action.equals(that.action)) return false;
if (!service.equals(that.service)) return false;
if (this.apiVersion != null ? !this.apiVersion.equals(that.apiVersion) : that.apiVersion != null) return false;
if (this.catchErrorPattern != null ? !this.catchErrorPattern.equals(that.catchErrorPattern) : that.catchErrorPattern != null) return false;
return this.parameters != null ? this.parameters.equals(that.parameters) : that.parameters == null;
}
@Override
public final int hashCode() {
int result = this.action.hashCode();
result = 31 * result + (this.service.hashCode());
result = 31 * result + (this.apiVersion != null ? this.apiVersion.hashCode() : 0);
result = 31 * result + (this.catchErrorPattern != null ? this.catchErrorPattern.hashCode() : 0);
result = 31 * result + (this.parameters != null ? this.parameters.hashCode() : 0);
return result;
}
}
}