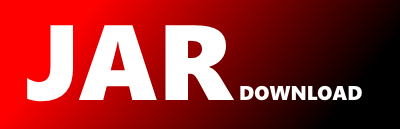
software.amazon.awscdk.services.gamelift.alpha.PriorityConfiguration Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) Custom prioritization settings for use by a game session queue when placing new game sessions with available game servers.
*
* When defined, this configuration replaces the default FleetIQ prioritization process, which is as follows:
*
*
* - If player latency data is included in a game session request, destinations and locations are prioritized first based on lowest average latency (1), then on lowest hosting cost (2), then on destination list order (3), and finally on location (alphabetical) (4).
* This approach ensures that the queue's top priority is to place game sessions where average player latency is lowest, and--if latency is the same--where the hosting cost is less, etc.
* - If player latency data is not included, destinations and locations are prioritized first on destination list order (1), and then on location (alphabetical) (2).
* This approach ensures that the queue's top priority is to place game sessions on the first destination fleet listed. If that fleet has multiple locations, the game session is placed on the first location (when listed alphabetically).
*
*
* Changing the priority order will affect how game sessions are placed.
*
* Example:
*
*
* BuildFleet fleet;
* Topic topic;
* GameSessionQueue.Builder.create(this, "MyGameSessionQueue")
* .gameSessionQueueName("test-gameSessionQueue")
* .customEventData("test-event-data")
* .allowedLocations(List.of("eu-west-1", "eu-west-2"))
* .destinations(List.of(fleet))
* .notificationTarget(topic)
* .playerLatencyPolicies(List.of(PlayerLatencyPolicy.builder()
* .maximumIndividualPlayerLatency(Duration.millis(100))
* .policyDuration(Duration.seconds(300))
* .build()))
* .priorityConfiguration(PriorityConfiguration.builder()
* .locationOrder(List.of("eu-west-1", "eu-west-2"))
* .priorityOrder(List.of(PriorityType.LATENCY, PriorityType.COST, PriorityType.DESTINATION, PriorityType.LOCATION))
* .build())
* .timeout(Duration.seconds(300))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.102.0 (build e354887)", date = "2024-08-23T05:56:25.541Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.PriorityConfiguration")
@software.amazon.jsii.Jsii.Proxy(PriorityConfiguration.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface PriorityConfiguration extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) The prioritization order to use for fleet locations, when the PriorityOrder property includes LOCATION.
*
* Locations are identified by AWS Region codes such as `us-west-2.
*
* Each location can only be listed once.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.util.List getLocationOrder();
/**
* (experimental) The recommended sequence to use when prioritizing where to place new game sessions.
*
* Each type can only be listed once.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.util.List getPriorityOrder();
/**
* @return a {@link Builder} of {@link PriorityConfiguration}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PriorityConfiguration}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List locationOrder;
java.util.List priorityOrder;
/**
* Sets the value of {@link PriorityConfiguration#getLocationOrder}
* @param locationOrder The prioritization order to use for fleet locations, when the PriorityOrder property includes LOCATION. This parameter is required.
* Locations are identified by AWS Region codes such as `us-west-2.
*
* Each location can only be listed once.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder locationOrder(java.util.List locationOrder) {
this.locationOrder = locationOrder;
return this;
}
/**
* Sets the value of {@link PriorityConfiguration#getPriorityOrder}
* @param priorityOrder The recommended sequence to use when prioritizing where to place new game sessions. This parameter is required.
* Each type can only be listed once.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder priorityOrder(java.util.List extends software.amazon.awscdk.services.gamelift.alpha.PriorityType> priorityOrder) {
this.priorityOrder = (java.util.List)priorityOrder;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PriorityConfiguration}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public PriorityConfiguration build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PriorityConfiguration}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PriorityConfiguration {
private final java.util.List locationOrder;
private final java.util.List priorityOrder;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.locationOrder = software.amazon.jsii.Kernel.get(this, "locationOrder", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.priorityOrder = software.amazon.jsii.Kernel.get(this, "priorityOrder", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.PriorityType.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.locationOrder = java.util.Objects.requireNonNull(builder.locationOrder, "locationOrder is required");
this.priorityOrder = (java.util.List)java.util.Objects.requireNonNull(builder.priorityOrder, "priorityOrder is required");
}
@Override
public final java.util.List getLocationOrder() {
return this.locationOrder;
}
@Override
public final java.util.List getPriorityOrder() {
return this.priorityOrder;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("locationOrder", om.valueToTree(this.getLocationOrder()));
data.set("priorityOrder", om.valueToTree(this.getPriorityOrder()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-gamelift-alpha.PriorityConfiguration"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PriorityConfiguration.Jsii$Proxy that = (PriorityConfiguration.Jsii$Proxy) o;
if (!locationOrder.equals(that.locationOrder)) return false;
return this.priorityOrder.equals(that.priorityOrder);
}
@Override
public final int hashCode() {
int result = this.locationOrder.hashCode();
result = 31 * result + (this.priorityOrder.hashCode());
return result;
}
}
}