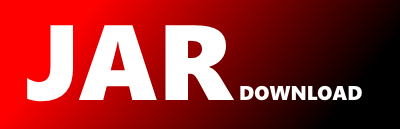
software.amazon.awscdk.services.gamelift.alpha.BuildFleet Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) A fleet contains Amazon Elastic Compute Cloud (Amazon EC2) instances that GameLift hosts.
*
* A fleet uses the configuration and scaling logic that you define to run your game server build. You can use a fleet directly without a queue.
* You can also associate multiple fleets with a GameLift queue.
*
* For example, you can use Spot Instance fleets configured with your preferred locations, along with a backup On-Demand Instance fleet with the same locations.
* Using multiple Spot Instance fleets of different instance types reduces the chance of needing On-Demand Instance placement.
*
* Example:
*
*
* Build build;
* // Server processes can be delcared in a declarative way through the constructor
* BuildFleet fleet = BuildFleet.Builder.create(this, "Game server fleet")
* .fleetName("test-fleet")
* .content(build)
* .instanceType(InstanceType.of(InstanceClass.C4, InstanceSize.LARGE))
* .runtimeConfiguration(RuntimeConfiguration.builder()
* .serverProcesses(List.of(ServerProcess.builder()
* .launchPath("/local/game/GameLiftExampleServer.x86_64")
* .parameters("-logFile /local/game/logs/myserver1935.log -port 1935")
* .concurrentExecutions(100)
* .build()))
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-27T17:02:12.093Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.BuildFleet")
public class BuildFleet extends software.amazon.awscdk.services.gamelift.alpha.FleetBase implements software.amazon.awscdk.services.gamelift.alpha.IBuildFleet {
protected BuildFleet(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected BuildFleet(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public BuildFleet(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.BuildFleetProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import an existing fleet from its ARN.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param buildFleetArn This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IBuildFleet fromBuildFleetArn(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String buildFleetArn) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.gamelift.alpha.BuildFleet.class, "fromBuildFleetArn", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IBuildFleet.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(buildFleetArn, "buildFleetArn is required") });
}
/**
* (experimental) Import an existing fleet from its identifier.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param buildFleetId This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IBuildFleet fromBuildFleetId(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String buildFleetId) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.gamelift.alpha.BuildFleet.class, "fromBuildFleetId", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IBuildFleet.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(buildFleetId, "buildFleetId is required") });
}
/**
* (experimental) Adds an ingress rule to allow inbound traffic to access game sessions on this fleet.
*
* @param source A range of allowed IP addresses. This parameter is required.
* @param port The port range used for ingress traffic. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addIngressRule(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IPeer source, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.Port port) {
software.amazon.jsii.Kernel.call(this, "addIngressRule", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(source, "source is required"), java.util.Objects.requireNonNull(port, "port is required") });
}
/**
* (experimental) The build content of the fleet.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IBuild getContent() {
return software.amazon.jsii.Kernel.get(this, "content", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IBuild.class));
}
/**
* (experimental) The ARN of the fleet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getFleetArn() {
return software.amazon.jsii.Kernel.get(this, "fleetArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The Identifier of the fleet.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getFleetId() {
return software.amazon.jsii.Kernel.get(this, "fleetId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The principal this GameLift fleet is using.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IPrincipal getGrantPrincipal() {
return software.amazon.jsii.Kernel.get(this, "grantPrincipal", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IPrincipal.class));
}
/**
* (experimental) The IAM role GameLift assumes by fleet instances to access AWS ressources.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole getRole() {
return software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.gamelift.alpha.BuildFleet}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.gamelift.alpha.BuildFleetProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.gamelift.alpha.BuildFleetProps.Builder();
}
/**
* (experimental) A descriptive label that is associated with a fleet.
*
* Fleet names do not need to be unique.
*
* @return {@code this}
* @param fleetName A descriptive label that is associated with a fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder fleetName(final java.lang.String fleetName) {
this.props.fleetName(fleetName);
return this;
}
/**
* (experimental) The GameLift-supported Amazon EC2 instance type to use for all fleet instances.
*
* Instance type determines the computing resources that will be used to host your game servers, including CPU, memory, storage, and networking capacity.
*
* @return {@code this}
* @see http://aws.amazon.com/ec2/instance-types/ for detailed descriptions of Amazon EC2 instance types.
* @param instanceType The GameLift-supported Amazon EC2 instance type to use for all fleet instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instanceType(final software.amazon.awscdk.services.ec2.InstanceType instanceType) {
this.props.instanceType(instanceType);
return this;
}
/**
* (experimental) A collection of server process configurations that describe the set of processes to run on each instance in a fleet.
*
* Server processes run either an executable in a custom game build or a Realtime Servers script.
* GameLift launches the configured processes, manages their life cycle, and replaces them as needed.
* Each instance checks regularly for an updated runtime configuration.
*
* A GameLift instance is limited to 50 processes running concurrently.
* To calculate the total number of processes in a runtime configuration, add the values of the ConcurrentExecutions parameter for each ServerProcess.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/fleets-multiprocess.html
* @param runtimeConfiguration A collection of server process configurations that describe the set of processes to run on each instance in a fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder runtimeConfiguration(final software.amazon.awscdk.services.gamelift.alpha.RuntimeConfiguration runtimeConfiguration) {
this.props.runtimeConfiguration(runtimeConfiguration);
return this;
}
/**
* (experimental) A human-readable description of the fleet.
*
* Default: no description is provided
*
* @return {@code this}
* @param description A human-readable description of the fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* (experimental) The number of EC2 instances that you want this fleet to host.
*
* When creating a new fleet, GameLift automatically sets this value to "1" and initiates a single instance.
* Once the fleet is active, update this value to trigger GameLift to add or remove instances from the fleet.
*
* Default: Default capacity is 0
*
* @return {@code this}
* @param desiredCapacity The number of EC2 instances that you want this fleet to host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder desiredCapacity(final java.lang.Number desiredCapacity) {
this.props.desiredCapacity(desiredCapacity);
return this;
}
/**
* (experimental) A set of remote locations to deploy additional instances to and manage as part of the fleet.
*
* This parameter can only be used when creating fleets in AWS Regions that support multiple locations.
* You can add any GameLift-supported AWS Region as a remote location, in the form of an AWS Region code such as us-west-2
.
* To create a fleet with instances in the home region only, omit this parameter.
*
* Default: Create a fleet with instances in the home region only
*
* @return {@code this}
* @param locations A set of remote locations to deploy additional instances to and manage as part of the fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder locations(final java.util.List extends software.amazon.awscdk.services.gamelift.alpha.Location> locations) {
this.props.locations(locations);
return this;
}
/**
* (experimental) The maximum number of instances that are allowed in the specified fleet location.
*
* Default: the default is 1
*
* @return {@code this}
* @param maxSize The maximum number of instances that are allowed in the specified fleet location. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder maxSize(final java.lang.Number maxSize) {
this.props.maxSize(maxSize);
return this;
}
/**
* (experimental) The name of an AWS CloudWatch metric group to add this fleet to.
*
* A metric group is used to aggregate the metrics for multiple fleets.
* You can specify an existing metric group name or set a new name to create a new metric group.
* A fleet can be included in only one metric group at a time.
*
* Default: Fleet metrics are aggregated with other fleets in the default metric group
*
* @return {@code this}
* @param metricGroup The name of an AWS CloudWatch metric group to add this fleet to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder metricGroup(final java.lang.String metricGroup) {
this.props.metricGroup(metricGroup);
return this;
}
/**
* (experimental) The minimum number of instances that are allowed in the specified fleet location.
*
* Default: the default is 0
*
* @return {@code this}
* @param minSize The minimum number of instances that are allowed in the specified fleet location. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder minSize(final java.lang.Number minSize) {
this.props.minSize(minSize);
return this;
}
/**
* (experimental) A VPC peering connection between your GameLift-hosted game servers and your other non-GameLift resources.
*
* Use Amazon Virtual Private Cloud (VPC) peering connections to enable your game servers to communicate directly and privately with your other AWS resources, such as a web service or a repository.
* You can establish VPC peering with any resources that run on AWS and are managed by an AWS account that you have access to.
* The VPC must be in the same Region as your fleet.
*
* Warning:
* Be sure to create a VPC Peering authorization through Gamelift Service API.
*
* Default: no vpc peering
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/vpc-peering.html
* @param peerVpc A VPC peering connection between your GameLift-hosted game servers and your other non-GameLift resources. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder peerVpc(final software.amazon.awscdk.services.ec2.IVpc peerVpc) {
this.props.peerVpc(peerVpc);
return this;
}
/**
* (experimental) The status of termination protection for active game sessions on the fleet.
*
* By default, new game sessions are protected and cannot be terminated during a scale-down event.
*
* Default: true - Game sessions in `ACTIVE` status cannot be terminated during a scale-down event.
*
* @return {@code this}
* @param protectNewGameSession The status of termination protection for active game sessions on the fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder protectNewGameSession(final java.lang.Boolean protectNewGameSession) {
this.props.protectNewGameSession(protectNewGameSession);
return this;
}
/**
* (experimental) A policy that limits the number of game sessions that an individual player can create on instances in this fleet within a specified span of time.
*
* Default: No resource creation limit policy
*
* @return {@code this}
* @param resourceCreationLimitPolicy A policy that limits the number of game sessions that an individual player can create on instances in this fleet within a specified span of time. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder resourceCreationLimitPolicy(final software.amazon.awscdk.services.gamelift.alpha.ResourceCreationLimitPolicy resourceCreationLimitPolicy) {
this.props.resourceCreationLimitPolicy(resourceCreationLimitPolicy);
return this;
}
/**
* (experimental) The IAM role assumed by GameLift fleet instances to access AWS ressources.
*
* With a role set, any application that runs on an instance in this fleet can assume the role, including install scripts, server processes, and daemons (background processes).
* If providing a custom role, it needs to trust the GameLift service principal (gamelift.amazonaws.com).
* No permission is required by default.
*
* This property cannot be changed after the fleet is created.
*
* Default: - a role will be created with default trust to Gamelift service principal.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-sdk-server-resources.html
* @param role The IAM role assumed by GameLift fleet instances to access AWS ressources. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* (experimental) Prompts GameLift to generate a TLS/SSL certificate for the fleet.
*
* GameLift uses the certificates to encrypt traffic between game clients and the game servers running on GameLift.
*
* You can't change this property after you create the fleet.
*
* Additionnal info:
* AWS Certificate Manager (ACM) certificates expire after 13 months.
* Certificate expiration can cause fleets to fail, preventing players from connecting to instances in the fleet.
* We recommend you replace fleets before 13 months, consider using fleet aliases for a smooth transition.
*
* Default: TLS/SSL certificate are generated for the fleet
*
* @return {@code this}
* @param useCertificate Prompts GameLift to generate a TLS/SSL certificate for the fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useCertificate(final java.lang.Boolean useCertificate) {
this.props.useCertificate(useCertificate);
return this;
}
/**
* (experimental) Indicates whether to use On-Demand or Spot instances for this fleet. By default, fleet use on demand capacity.
*
* This property cannot be changed after the fleet is created.
*
* Default: Gamelift fleet use on demand capacity
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-ec2-instances.html#gamelift-ec2-instances-spot
* @param useSpot Indicates whether to use On-Demand or Spot instances for this fleet. By default, fleet use on demand capacity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder useSpot(final java.lang.Boolean useSpot) {
this.props.useSpot(useSpot);
return this;
}
/**
* (experimental) A build to be deployed on the fleet.
*
* The build must have been successfully uploaded to Amazon GameLift and be in a READY
status.
*
* This fleet setting cannot be changed once the fleet is created.
*
* @return {@code this}
* @param content A build to be deployed on the fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder content(final software.amazon.awscdk.services.gamelift.alpha.IBuild content) {
this.props.content(content);
return this;
}
/**
* (experimental) The allowed IP address ranges and port settings that allow inbound traffic to access game sessions on this fleet.
*
* This property must be set before players can connect to game sessions.
*
* Default: no inbound traffic allowed
*
* @return {@code this}
* @param ingressRules The allowed IP address ranges and port settings that allow inbound traffic to access game sessions on this fleet. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ingressRules(final java.util.List extends software.amazon.awscdk.services.gamelift.alpha.IngressRule> ingressRules) {
this.props.ingressRules(ingressRules);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.gamelift.alpha.BuildFleet}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.gamelift.alpha.BuildFleet build() {
return new software.amazon.awscdk.services.gamelift.alpha.BuildFleet(
this.scope,
this.id,
this.props.build()
);
}
}
}