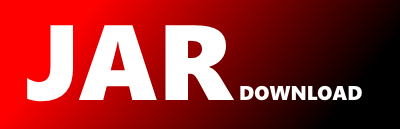
software.amazon.awscdk.services.gamelift.alpha.GameServerGroup Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) Creates a GameLift FleetIQ game server group for managing game hosting on a collection of Amazon EC2 instances for game hosting.
*
* This operation creates the game server group, creates an Auto Scaling group in your AWS account, and establishes a link between the two groups.
* You can view the status of your game server groups in the GameLift console.
* Game server group metrics and events are emitted to Amazon CloudWatch.
* Before creating a new game server group, you must have the following:
*
*
* - An Amazon EC2 launch template that specifies how to launch Amazon EC2 instances with your game server build.
* - An IAM role that extends limited access to your AWS account to allow GameLift FleetIQ to create and interact with the Auto Scaling group.
*
*
* To create a new game server group, specify a unique group name, IAM role and Amazon EC2 launch template, and provide a list of instance types that can be used in the group.
* You must also set initial maximum and minimum limits on the group's instance count.
* You can optionally set an Auto Scaling policy with target tracking based on a GameLift FleetIQ metric.
*
* Once the game server group and corresponding Auto Scaling group are created, you have full access to change the Auto Scaling group's configuration as needed.
* Several properties that are set when creating a game server group, including maximum/minimum size and auto-scaling policy settings, must be updated directly in the Auto Scaling group.
* Keep in mind that some Auto Scaling group properties are periodically updated by GameLift FleetIQ as part of its balancing activities to optimize for availability and cost.
*
* Example:
*
*
* ILaunchTemplate launchTemplate;
* IVpc vpc;
* GameServerGroup.Builder.create(this, "GameServerGroup")
* .gameServerGroupName("sample-gameservergroup-name")
* .instanceDefinitions(List.of(InstanceDefinition.builder()
* .instanceType(InstanceType.of(InstanceClass.C5, InstanceSize.LARGE))
* .build(), InstanceDefinition.builder()
* .instanceType(InstanceType.of(InstanceClass.C4, InstanceSize.LARGE))
* .build()))
* .launchTemplate(launchTemplate)
* .vpc(vpc)
* .vpcSubnets(SubnetSelection.builder().subnetType(SubnetType.PUBLIC).build())
* .build();
*
*
* @see https://docs.aws.amazon.com/gamelift/latest/fleetiqguide/gsg-intro.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-27T17:02:12.101Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.GameServerGroup")
public class GameServerGroup extends software.amazon.awscdk.services.gamelift.alpha.GameServerGroupBase {
protected GameServerGroup(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected GameServerGroup(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public GameServerGroup(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import an existing game server group from its attributes.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param attrs This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IGameServerGroup fromGameServerGroupAttributes(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.GameServerGroupAttributes attrs) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.gamelift.alpha.GameServerGroup.class, "fromGameServerGroupAttributes", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IGameServerGroup.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(attrs, "attrs is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.gamelift.CfnGameServerGroup.AutoScalingPolicyProperty parseAutoScalingPolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps props) {
return software.amazon.jsii.Kernel.call(this, "parseAutoScalingPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.CfnGameServerGroup.AutoScalingPolicyProperty.class), new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected @org.jetbrains.annotations.NotNull java.util.List parseInstanceDefinitions(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps props) {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.call(this, "parseInstanceDefinitions", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.CfnGameServerGroup.InstanceDefinitionProperty.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.CfnGameServerGroup.LaunchTemplateProperty parseLaunchTemplate(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps props) {
return software.amazon.jsii.Kernel.call(this, "parseLaunchTemplate", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.CfnGameServerGroup.LaunchTemplateProperty.class), new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) The ARN of the generated AutoScaling group.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getAutoScalingGroupArn() {
return software.amazon.jsii.Kernel.get(this, "autoScalingGroupArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The ARN of the game server group.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getGameServerGroupArn() {
return software.amazon.jsii.Kernel.get(this, "gameServerGroupArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The name of the game server group.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getGameServerGroupName() {
return software.amazon.jsii.Kernel.get(this, "gameServerGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The principal this GameLift game server group is using.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IPrincipal getGrantPrincipal() {
return software.amazon.jsii.Kernel.get(this, "grantPrincipal", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IPrincipal.class));
}
/**
* (experimental) The IAM role that allows Amazon GameLift to access your Amazon EC2 Auto Scaling groups.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole getRole() {
return software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
}
/**
* (experimental) The VPC network to place the game server group in.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IVpc getVpc() {
return software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
}
/**
* (experimental) The game server group's subnets.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.gamelift.alpha.GameServerGroup}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps.Builder();
}
/**
* (experimental) A developer-defined identifier for the game server group.
*
* The name is unique for each Region in each AWS account.
*
* @return {@code this}
* @param gameServerGroupName A developer-defined identifier for the game server group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder gameServerGroupName(final java.lang.String gameServerGroupName) {
this.props.gameServerGroupName(gameServerGroupName);
return this;
}
/**
* (experimental) The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling instances in the corresponding Auto Scaling group.
*
* @return {@code this}
* @param instanceDefinitions The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling instances in the corresponding Auto Scaling group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder instanceDefinitions(final java.util.List extends software.amazon.awscdk.services.gamelift.alpha.InstanceDefinition> instanceDefinitions) {
this.props.instanceDefinitions(instanceDefinitions);
return this;
}
/**
* (experimental) The Amazon EC2 launch template that contains configuration settings and game server code to be deployed to all instances in the game server group.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* NOTE:
* If you specify network interfaces in your launch template, you must explicitly set the property AssociatePublicIpAddress to true
.
* If no network interface is specified in the launch template, GameLift FleetIQ uses your account's default VPC.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/autoscaling/ec2/userguide/create-launch-template.html
* @param launchTemplate The Amazon EC2 launch template that contains configuration settings and game server code to be deployed to all instances in the game server group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder launchTemplate(final software.amazon.awscdk.services.ec2.ILaunchTemplate launchTemplate) {
this.props.launchTemplate(launchTemplate);
return this;
}
/**
* (experimental) The VPC network to place the game server group in.
*
* By default, all GameLift FleetIQ-supported Availability Zones are used.
*
* You can use this parameter to specify VPCs that you've set up.
*
* This property cannot be updated after the game server group is created,
* and the corresponding Auto Scaling group will always use the property value that is set with this request,
* even if the Auto Scaling group is updated directly.
*
* @return {@code this}
* @param vpc The VPC network to place the game server group in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(final software.amazon.awscdk.services.ec2.IVpc vpc) {
this.props.vpc(vpc);
return this;
}
/**
* (experimental) Configuration settings to define a scaling policy for the Auto Scaling group that is optimized for game hosting.
*
* The scaling policy uses the metric PercentUtilizedGameServers
to maintain a buffer of idle game servers that can immediately accommodate new games and players.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: no autoscaling policy settled
*
* @return {@code this}
* @param autoScalingPolicy Configuration settings to define a scaling policy for the Auto Scaling group that is optimized for game hosting. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder autoScalingPolicy(final software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy autoScalingPolicy) {
this.props.autoScalingPolicy(autoScalingPolicy);
return this;
}
/**
* (experimental) Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server group.
*
* Default: SPOT_PREFERRED
*
* @return {@code this}
* @param balancingStrategy Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder balancingStrategy(final software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy balancingStrategy) {
this.props.balancingStrategy(balancingStrategy);
return this;
}
/**
* (experimental) The type of delete to perform.
*
* To delete a game server group, specify the DeleteOption
*
* Default: SAFE_DELETE
*
* @return {@code this}
* @param deleteOption The type of delete to perform. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder deleteOption(final software.amazon.awscdk.services.gamelift.alpha.DeleteOption deleteOption) {
this.props.deleteOption(deleteOption);
return this;
}
/**
* (experimental) The maximum number of instances allowed in the Amazon EC2 Auto Scaling group.
*
* During automatic scaling events, GameLift FleetIQ and EC2 do not scale up the group above this maximum.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: the default is 1
*
* @return {@code this}
* @param maxSize The maximum number of instances allowed in the Amazon EC2 Auto Scaling group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder maxSize(final java.lang.Number maxSize) {
this.props.maxSize(maxSize);
return this;
}
/**
* (experimental) The minimum number of instances allowed in the Amazon EC2 Auto Scaling group.
*
* During automatic scaling events, GameLift FleetIQ and Amazon EC2 do not scale down the group below this minimum.
*
* In production, this value should be set to at least 1.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: the default is 0
*
* @return {@code this}
* @param minSize The minimum number of instances allowed in the Amazon EC2 Auto Scaling group. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder minSize(final java.lang.Number minSize) {
this.props.minSize(minSize);
return this;
}
/**
* (experimental) A flag that indicates whether instances in the game server group are protected from early termination.
*
* Unprotected instances that have active game servers running might be terminated during a scale-down event, causing players to be dropped from the game.
* Protected instances cannot be terminated while there are active game servers running except in the event of a forced game server group deletion.
*
* An exception to this is with Spot Instances, which can be terminated by AWS regardless of protection status.
*
* Default: game servers running might be terminated during a scale-down event
*
* @return {@code this}
* @param protectGameServer A flag that indicates whether instances in the game server group are protected from early termination. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder protectGameServer(final java.lang.Boolean protectGameServer) {
this.props.protectGameServer(protectGameServer);
return this;
}
/**
* (experimental) The IAM role that allows Amazon GameLift to access your Amazon EC2 Auto Scaling groups.
*
* Default: - a role will be created with default trust to Gamelift and Autoscaling service principal with a default policy `GameLiftGameServerGroupPolicy` attached.
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/fleetiqguide/gsg-iam-permissions-roles.html
* @param role The IAM role that allows Amazon GameLift to access your Amazon EC2 Auto Scaling groups. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* (experimental) Game server group subnet selection.
*
* Default: all GameLift FleetIQ-supported Availability Zones are used.
*
* @return {@code this}
* @param vpcSubnets Game server group subnet selection. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.props.vpcSubnets(vpcSubnets);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.gamelift.alpha.GameServerGroup}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.gamelift.alpha.GameServerGroup build() {
return new software.amazon.awscdk.services.gamelift.alpha.GameServerGroup(
this.scope,
this.id,
this.props.build()
);
}
}
}