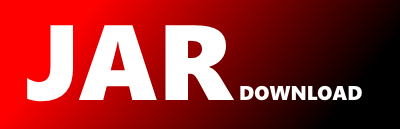
software.amazon.awscdk.services.gamelift.alpha.GameServerGroupProps Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) Properties for a new Gamelift FleetIQ Game server group.
*
* Example:
*
*
* ILaunchTemplate launchTemplate;
* IVpc vpc;
* GameServerGroup.Builder.create(this, "GameServerGroup")
* .gameServerGroupName("sample-gameservergroup-name")
* .instanceDefinitions(List.of(InstanceDefinition.builder()
* .instanceType(InstanceType.of(InstanceClass.C5, InstanceSize.LARGE))
* .build(), InstanceDefinition.builder()
* .instanceType(InstanceType.of(InstanceClass.C4, InstanceSize.LARGE))
* .build()))
* .launchTemplate(launchTemplate)
* .vpc(vpc)
* .vpcSubnets(SubnetSelection.builder().subnetType(SubnetType.PUBLIC).build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-27T17:02:12.104Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.GameServerGroupProps")
@software.amazon.jsii.Jsii.Proxy(GameServerGroupProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface GameServerGroupProps extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) A developer-defined identifier for the game server group.
*
* The name is unique for each Region in each AWS account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.lang.String getGameServerGroupName();
/**
* (experimental) The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling instances in the corresponding Auto Scaling group.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull java.util.List getInstanceDefinitions();
/**
* (experimental) The Amazon EC2 launch template that contains configuration settings and game server code to be deployed to all instances in the game server group.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* NOTE:
* If you specify network interfaces in your launch template, you must explicitly set the property AssociatePublicIpAddress to true
.
* If no network interface is specified in the launch template, GameLift FleetIQ uses your account's default VPC.
*
* @see https://docs.aws.amazon.com/autoscaling/ec2/userguide/create-launch-template.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.ILaunchTemplate getLaunchTemplate();
/**
* (experimental) The VPC network to place the game server group in.
*
* By default, all GameLift FleetIQ-supported Availability Zones are used.
*
* You can use this parameter to specify VPCs that you've set up.
*
* This property cannot be updated after the game server group is created,
* and the corresponding Auto Scaling group will always use the property value that is set with this request,
* even if the Auto Scaling group is updated directly.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ec2.IVpc getVpc();
/**
* (experimental) Configuration settings to define a scaling policy for the Auto Scaling group that is optimized for game hosting.
*
* The scaling policy uses the metric PercentUtilizedGameServers
to maintain a buffer of idle game servers that can immediately accommodate new games and players.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: no autoscaling policy settled
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy getAutoScalingPolicy() {
return null;
}
/**
* (experimental) Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server group.
*
* Default: SPOT_PREFERRED
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy getBalancingStrategy() {
return null;
}
/**
* (experimental) The type of delete to perform.
*
* To delete a game server group, specify the DeleteOption
*
* Default: SAFE_DELETE
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.gamelift.alpha.DeleteOption getDeleteOption() {
return null;
}
/**
* (experimental) The maximum number of instances allowed in the Amazon EC2 Auto Scaling group.
*
* During automatic scaling events, GameLift FleetIQ and EC2 do not scale up the group above this maximum.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: the default is 1
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getMaxSize() {
return null;
}
/**
* (experimental) The minimum number of instances allowed in the Amazon EC2 Auto Scaling group.
*
* During automatic scaling events, GameLift FleetIQ and Amazon EC2 do not scale down the group below this minimum.
*
* In production, this value should be set to at least 1.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* Default: the default is 0
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Number getMinSize() {
return null;
}
/**
* (experimental) A flag that indicates whether instances in the game server group are protected from early termination.
*
* Unprotected instances that have active game servers running might be terminated during a scale-down event, causing players to be dropped from the game.
* Protected instances cannot be terminated while there are active game servers running except in the event of a forced game server group deletion.
*
* An exception to this is with Spot Instances, which can be terminated by AWS regardless of protection status.
*
* Default: game servers running might be terminated during a scale-down event
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getProtectGameServer() {
return null;
}
/**
* (experimental) The IAM role that allows Amazon GameLift to access your Amazon EC2 Auto Scaling groups.
*
* Default: - a role will be created with default trust to Gamelift and Autoscaling service principal with a default policy `GameLiftGameServerGroupPolicy` attached.
*
* @see https://docs.aws.amazon.com/gamelift/latest/fleetiqguide/gsg-iam-permissions-roles.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.iam.IRole getRole() {
return null;
}
/**
* (experimental) Game server group subnet selection.
*
* Default: all GameLift FleetIQ-supported Availability Zones are used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return null;
}
/**
* @return a {@link Builder} of {@link GameServerGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link GameServerGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String gameServerGroupName;
java.util.List instanceDefinitions;
software.amazon.awscdk.services.ec2.ILaunchTemplate launchTemplate;
software.amazon.awscdk.services.ec2.IVpc vpc;
software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy autoScalingPolicy;
software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy balancingStrategy;
software.amazon.awscdk.services.gamelift.alpha.DeleteOption deleteOption;
java.lang.Number maxSize;
java.lang.Number minSize;
java.lang.Boolean protectGameServer;
software.amazon.awscdk.services.iam.IRole role;
software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Sets the value of {@link GameServerGroupProps#getGameServerGroupName}
* @param gameServerGroupName A developer-defined identifier for the game server group. This parameter is required.
* The name is unique for each Region in each AWS account.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder gameServerGroupName(java.lang.String gameServerGroupName) {
this.gameServerGroupName = gameServerGroupName;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getInstanceDefinitions}
* @param instanceDefinitions The set of Amazon EC2 instance types that GameLift FleetIQ can use when balancing and automatically scaling instances in the corresponding Auto Scaling group. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder instanceDefinitions(java.util.List extends software.amazon.awscdk.services.gamelift.alpha.InstanceDefinition> instanceDefinitions) {
this.instanceDefinitions = (java.util.List)instanceDefinitions;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getLaunchTemplate}
* @param launchTemplate The Amazon EC2 launch template that contains configuration settings and game server code to be deployed to all instances in the game server group. This parameter is required.
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
*
* NOTE:
* If you specify network interfaces in your launch template, you must explicitly set the property AssociatePublicIpAddress to true
.
* If no network interface is specified in the launch template, GameLift FleetIQ uses your account's default VPC.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder launchTemplate(software.amazon.awscdk.services.ec2.ILaunchTemplate launchTemplate) {
this.launchTemplate = launchTemplate;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getVpc}
* @param vpc The VPC network to place the game server group in. This parameter is required.
* By default, all GameLift FleetIQ-supported Availability Zones are used.
*
* You can use this parameter to specify VPCs that you've set up.
*
* This property cannot be updated after the game server group is created,
* and the corresponding Auto Scaling group will always use the property value that is set with this request,
* even if the Auto Scaling group is updated directly.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpc(software.amazon.awscdk.services.ec2.IVpc vpc) {
this.vpc = vpc;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getAutoScalingPolicy}
* @param autoScalingPolicy Configuration settings to define a scaling policy for the Auto Scaling group that is optimized for game hosting.
* The scaling policy uses the metric PercentUtilizedGameServers
to maintain a buffer of idle game servers that can immediately accommodate new games and players.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder autoScalingPolicy(software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy autoScalingPolicy) {
this.autoScalingPolicy = autoScalingPolicy;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getBalancingStrategy}
* @param balancingStrategy Indicates how GameLift FleetIQ balances the use of Spot Instances and On-Demand Instances in the game server group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder balancingStrategy(software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy balancingStrategy) {
this.balancingStrategy = balancingStrategy;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getDeleteOption}
* @param deleteOption The type of delete to perform.
* To delete a game server group, specify the DeleteOption
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder deleteOption(software.amazon.awscdk.services.gamelift.alpha.DeleteOption deleteOption) {
this.deleteOption = deleteOption;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getMaxSize}
* @param maxSize The maximum number of instances allowed in the Amazon EC2 Auto Scaling group.
* During automatic scaling events, GameLift FleetIQ and EC2 do not scale up the group above this maximum.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder maxSize(java.lang.Number maxSize) {
this.maxSize = maxSize;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getMinSize}
* @param minSize The minimum number of instances allowed in the Amazon EC2 Auto Scaling group.
* During automatic scaling events, GameLift FleetIQ and Amazon EC2 do not scale down the group below this minimum.
*
* In production, this value should be set to at least 1.
*
* After the Auto Scaling group is created, update this value directly in the Auto Scaling group using the AWS console or APIs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder minSize(java.lang.Number minSize) {
this.minSize = minSize;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getProtectGameServer}
* @param protectGameServer A flag that indicates whether instances in the game server group are protected from early termination.
* Unprotected instances that have active game servers running might be terminated during a scale-down event, causing players to be dropped from the game.
* Protected instances cannot be terminated while there are active game servers running except in the event of a forced game server group deletion.
*
* An exception to this is with Spot Instances, which can be terminated by AWS regardless of protection status.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder protectGameServer(java.lang.Boolean protectGameServer) {
this.protectGameServer = protectGameServer;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getRole}
* @param role The IAM role that allows Amazon GameLift to access your Amazon EC2 Auto Scaling groups.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link GameServerGroupProps#getVpcSubnets}
* @param vpcSubnets Game server group subnet selection.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder vpcSubnets(software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets) {
this.vpcSubnets = vpcSubnets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link GameServerGroupProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public GameServerGroupProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link GameServerGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements GameServerGroupProps {
private final java.lang.String gameServerGroupName;
private final java.util.List instanceDefinitions;
private final software.amazon.awscdk.services.ec2.ILaunchTemplate launchTemplate;
private final software.amazon.awscdk.services.ec2.IVpc vpc;
private final software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy autoScalingPolicy;
private final software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy balancingStrategy;
private final software.amazon.awscdk.services.gamelift.alpha.DeleteOption deleteOption;
private final java.lang.Number maxSize;
private final java.lang.Number minSize;
private final java.lang.Boolean protectGameServer;
private final software.amazon.awscdk.services.iam.IRole role;
private final software.amazon.awscdk.services.ec2.SubnetSelection vpcSubnets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.gameServerGroupName = software.amazon.jsii.Kernel.get(this, "gameServerGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.instanceDefinitions = software.amazon.jsii.Kernel.get(this, "instanceDefinitions", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.InstanceDefinition.class)));
this.launchTemplate = software.amazon.jsii.Kernel.get(this, "launchTemplate", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ILaunchTemplate.class));
this.vpc = software.amazon.jsii.Kernel.get(this, "vpc", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.IVpc.class));
this.autoScalingPolicy = software.amazon.jsii.Kernel.get(this, "autoScalingPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy.class));
this.balancingStrategy = software.amazon.jsii.Kernel.get(this, "balancingStrategy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy.class));
this.deleteOption = software.amazon.jsii.Kernel.get(this, "deleteOption", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.DeleteOption.class));
this.maxSize = software.amazon.jsii.Kernel.get(this, "maxSize", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.minSize = software.amazon.jsii.Kernel.get(this, "minSize", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.protectGameServer = software.amazon.jsii.Kernel.get(this, "protectGameServer", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.vpcSubnets = software.amazon.jsii.Kernel.get(this, "vpcSubnets", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.SubnetSelection.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.gameServerGroupName = java.util.Objects.requireNonNull(builder.gameServerGroupName, "gameServerGroupName is required");
this.instanceDefinitions = (java.util.List)java.util.Objects.requireNonNull(builder.instanceDefinitions, "instanceDefinitions is required");
this.launchTemplate = java.util.Objects.requireNonNull(builder.launchTemplate, "launchTemplate is required");
this.vpc = java.util.Objects.requireNonNull(builder.vpc, "vpc is required");
this.autoScalingPolicy = builder.autoScalingPolicy;
this.balancingStrategy = builder.balancingStrategy;
this.deleteOption = builder.deleteOption;
this.maxSize = builder.maxSize;
this.minSize = builder.minSize;
this.protectGameServer = builder.protectGameServer;
this.role = builder.role;
this.vpcSubnets = builder.vpcSubnets;
}
@Override
public final java.lang.String getGameServerGroupName() {
return this.gameServerGroupName;
}
@Override
public final java.util.List getInstanceDefinitions() {
return this.instanceDefinitions;
}
@Override
public final software.amazon.awscdk.services.ec2.ILaunchTemplate getLaunchTemplate() {
return this.launchTemplate;
}
@Override
public final software.amazon.awscdk.services.ec2.IVpc getVpc() {
return this.vpc;
}
@Override
public final software.amazon.awscdk.services.gamelift.alpha.AutoScalingPolicy getAutoScalingPolicy() {
return this.autoScalingPolicy;
}
@Override
public final software.amazon.awscdk.services.gamelift.alpha.BalancingStrategy getBalancingStrategy() {
return this.balancingStrategy;
}
@Override
public final software.amazon.awscdk.services.gamelift.alpha.DeleteOption getDeleteOption() {
return this.deleteOption;
}
@Override
public final java.lang.Number getMaxSize() {
return this.maxSize;
}
@Override
public final java.lang.Number getMinSize() {
return this.minSize;
}
@Override
public final java.lang.Boolean getProtectGameServer() {
return this.protectGameServer;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final software.amazon.awscdk.services.ec2.SubnetSelection getVpcSubnets() {
return this.vpcSubnets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("gameServerGroupName", om.valueToTree(this.getGameServerGroupName()));
data.set("instanceDefinitions", om.valueToTree(this.getInstanceDefinitions()));
data.set("launchTemplate", om.valueToTree(this.getLaunchTemplate()));
data.set("vpc", om.valueToTree(this.getVpc()));
if (this.getAutoScalingPolicy() != null) {
data.set("autoScalingPolicy", om.valueToTree(this.getAutoScalingPolicy()));
}
if (this.getBalancingStrategy() != null) {
data.set("balancingStrategy", om.valueToTree(this.getBalancingStrategy()));
}
if (this.getDeleteOption() != null) {
data.set("deleteOption", om.valueToTree(this.getDeleteOption()));
}
if (this.getMaxSize() != null) {
data.set("maxSize", om.valueToTree(this.getMaxSize()));
}
if (this.getMinSize() != null) {
data.set("minSize", om.valueToTree(this.getMinSize()));
}
if (this.getProtectGameServer() != null) {
data.set("protectGameServer", om.valueToTree(this.getProtectGameServer()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
if (this.getVpcSubnets() != null) {
data.set("vpcSubnets", om.valueToTree(this.getVpcSubnets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-gamelift-alpha.GameServerGroupProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GameServerGroupProps.Jsii$Proxy that = (GameServerGroupProps.Jsii$Proxy) o;
if (!gameServerGroupName.equals(that.gameServerGroupName)) return false;
if (!instanceDefinitions.equals(that.instanceDefinitions)) return false;
if (!launchTemplate.equals(that.launchTemplate)) return false;
if (!vpc.equals(that.vpc)) return false;
if (this.autoScalingPolicy != null ? !this.autoScalingPolicy.equals(that.autoScalingPolicy) : that.autoScalingPolicy != null) return false;
if (this.balancingStrategy != null ? !this.balancingStrategy.equals(that.balancingStrategy) : that.balancingStrategy != null) return false;
if (this.deleteOption != null ? !this.deleteOption.equals(that.deleteOption) : that.deleteOption != null) return false;
if (this.maxSize != null ? !this.maxSize.equals(that.maxSize) : that.maxSize != null) return false;
if (this.minSize != null ? !this.minSize.equals(that.minSize) : that.minSize != null) return false;
if (this.protectGameServer != null ? !this.protectGameServer.equals(that.protectGameServer) : that.protectGameServer != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
return this.vpcSubnets != null ? this.vpcSubnets.equals(that.vpcSubnets) : that.vpcSubnets == null;
}
@Override
public final int hashCode() {
int result = this.gameServerGroupName.hashCode();
result = 31 * result + (this.instanceDefinitions.hashCode());
result = 31 * result + (this.launchTemplate.hashCode());
result = 31 * result + (this.vpc.hashCode());
result = 31 * result + (this.autoScalingPolicy != null ? this.autoScalingPolicy.hashCode() : 0);
result = 31 * result + (this.balancingStrategy != null ? this.balancingStrategy.hashCode() : 0);
result = 31 * result + (this.deleteOption != null ? this.deleteOption.hashCode() : 0);
result = 31 * result + (this.maxSize != null ? this.maxSize.hashCode() : 0);
result = 31 * result + (this.minSize != null ? this.minSize.hashCode() : 0);
result = 31 * result + (this.protectGameServer != null ? this.protectGameServer.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.vpcSubnets != null ? this.vpcSubnets.hashCode() : 0);
return result;
}
}
}