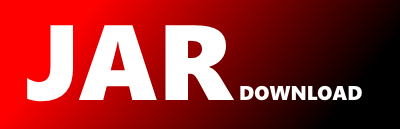
software.amazon.awscdk.services.gamelift.alpha.PlayerLatencyPolicy Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) The queue setting that determines the highest latency allowed for individual players when placing a game session.
*
* When a latency policy is in force, a game session cannot be placed with any fleet in a Region where a player reports latency higher than the cap.
*
* Latency policies are only enforced when the placement request contains player latency information.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.gamelift.alpha.*;
* import software.amazon.awscdk.*;
* PlayerLatencyPolicy playerLatencyPolicy = PlayerLatencyPolicy.builder()
* .maximumIndividualPlayerLatency(Duration.minutes(30))
* // the properties below are optional
* .policyDuration(Duration.minutes(30))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-27T17:02:12.136Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.PlayerLatencyPolicy")
@software.amazon.jsii.Jsii.Proxy(PlayerLatencyPolicy.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface PlayerLatencyPolicy extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) The maximum latency value that is allowed for any player, in milliseconds.
*
* All policies must have a value set for this property.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.Duration getMaximumIndividualPlayerLatency();
/**
* (experimental) The length of time, in seconds, that the policy is enforced while placing a new game session.
*
* Default: the policy is enforced until the queue times out.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.Duration getPolicyDuration() {
return null;
}
/**
* @return a {@link Builder} of {@link PlayerLatencyPolicy}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PlayerLatencyPolicy}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.Duration maximumIndividualPlayerLatency;
software.amazon.awscdk.Duration policyDuration;
/**
* Sets the value of {@link PlayerLatencyPolicy#getMaximumIndividualPlayerLatency}
* @param maximumIndividualPlayerLatency The maximum latency value that is allowed for any player, in milliseconds. This parameter is required.
* All policies must have a value set for this property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder maximumIndividualPlayerLatency(software.amazon.awscdk.Duration maximumIndividualPlayerLatency) {
this.maximumIndividualPlayerLatency = maximumIndividualPlayerLatency;
return this;
}
/**
* Sets the value of {@link PlayerLatencyPolicy#getPolicyDuration}
* @param policyDuration The length of time, in seconds, that the policy is enforced while placing a new game session.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder policyDuration(software.amazon.awscdk.Duration policyDuration) {
this.policyDuration = policyDuration;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PlayerLatencyPolicy}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public PlayerLatencyPolicy build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PlayerLatencyPolicy}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PlayerLatencyPolicy {
private final software.amazon.awscdk.Duration maximumIndividualPlayerLatency;
private final software.amazon.awscdk.Duration policyDuration;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.maximumIndividualPlayerLatency = software.amazon.jsii.Kernel.get(this, "maximumIndividualPlayerLatency", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.Duration.class));
this.policyDuration = software.amazon.jsii.Kernel.get(this, "policyDuration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.Duration.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.maximumIndividualPlayerLatency = java.util.Objects.requireNonNull(builder.maximumIndividualPlayerLatency, "maximumIndividualPlayerLatency is required");
this.policyDuration = builder.policyDuration;
}
@Override
public final software.amazon.awscdk.Duration getMaximumIndividualPlayerLatency() {
return this.maximumIndividualPlayerLatency;
}
@Override
public final software.amazon.awscdk.Duration getPolicyDuration() {
return this.policyDuration;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("maximumIndividualPlayerLatency", om.valueToTree(this.getMaximumIndividualPlayerLatency()));
if (this.getPolicyDuration() != null) {
data.set("policyDuration", om.valueToTree(this.getPolicyDuration()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-gamelift-alpha.PlayerLatencyPolicy"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PlayerLatencyPolicy.Jsii$Proxy that = (PlayerLatencyPolicy.Jsii$Proxy) o;
if (!maximumIndividualPlayerLatency.equals(that.maximumIndividualPlayerLatency)) return false;
return this.policyDuration != null ? this.policyDuration.equals(that.policyDuration) : that.policyDuration == null;
}
@Override
public final int hashCode() {
int result = this.maximumIndividualPlayerLatency.hashCode();
result = 31 * result + (this.policyDuration != null ? this.policyDuration.hashCode() : 0);
return result;
}
}
}