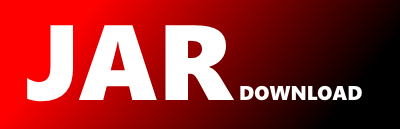
software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration Maven / Gradle / Ivy
Show all versions of gamelift-alpha Show documentation
package software.amazon.awscdk.services.gamelift.alpha;
/**
* (experimental) A FlexMatch matchmaker process does the work of building a game match.
*
* It manages the pool of matchmaking requests received, forms teams for a match, processes and selects players to find the best possible player groups, and initiates the process of placing and starting a game session for the match.
* This topic describes the key aspects of a matchmaker and how to configure one customized for your game.
*
* Example:
*
*
* GameSessionQueue queue;
* MatchmakingRuleSet ruleSet;
* QueuedMatchmakingConfiguration.Builder.create(this, "QueuedMatchmakingConfiguration")
* .matchmakingConfigurationName("test-queued-config-name")
* .gameSessionQueues(List.of(queue))
* .ruleSet(ruleSet)
* .build();
*
*
* @see https://docs.aws.amazon.com/gamelift/latest/flexmatchguide/match-configuration.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.104.0 (build e79254c)", date = "2024-12-27T17:02:12.138Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.gamelift.alpha.$Module.class, fqn = "@aws-cdk/aws-gamelift-alpha.QueuedMatchmakingConfiguration")
public class QueuedMatchmakingConfiguration extends software.amazon.awscdk.services.gamelift.alpha.MatchmakingConfigurationBase {
protected QueuedMatchmakingConfiguration(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected QueuedMatchmakingConfiguration(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public QueuedMatchmakingConfiguration(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfigurationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Import an existing matchmaking configuration from its ARN.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param matchmakingConfigurationArn This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IMatchmakingConfiguration fromQueuedMatchmakingConfigurationArn(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String matchmakingConfigurationArn) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration.class, "fromQueuedMatchmakingConfigurationArn", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IMatchmakingConfiguration.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(matchmakingConfigurationArn, "matchmakingConfigurationArn is required") });
}
/**
* (experimental) Import an existing matchmaking configuration from its name.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param matchmakingConfigurationName This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IMatchmakingConfiguration fromQueuedMatchmakingConfigurationName(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String matchmakingConfigurationName) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration.class, "fromQueuedMatchmakingConfigurationName", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.gamelift.alpha.IMatchmakingConfiguration.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(matchmakingConfigurationName, "matchmakingConfigurationName is required") });
}
/**
* (experimental) Adds a game session queue destination to the matchmaking configuration.
*
* @param gameSessionQueue A game session queue. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addGameSessionQueue(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.gamelift.alpha.IGameSessionQueue gameSessionQueue) {
software.amazon.jsii.Kernel.call(this, "addGameSessionQueue", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(gameSessionQueue, "gameSessionQueue is required") });
}
/**
* (experimental) The ARN of the matchmaking configuration.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getMatchmakingConfigurationArn() {
return software.amazon.jsii.Kernel.get(this, "matchmakingConfigurationArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The name of the matchmaking configuration.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull java.lang.String getMatchmakingConfigurationName() {
return software.amazon.jsii.Kernel.get(this, "matchmakingConfigurationName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* (experimental) The notification target for matchmaking events.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sns.ITopic getNotificationTarget() {
return software.amazon.jsii.Kernel.get(this, "notificationTarget", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.sns.ITopic.class));
}
/**
* (experimental) A fluent builder for {@link software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfigurationProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfigurationProps.Builder();
}
/**
* (experimental) A unique identifier for the matchmaking configuration.
*
* This name is used to identify the configuration associated with a matchmaking request or ticket.
*
* @return {@code this}
* @param matchmakingConfigurationName A unique identifier for the matchmaking configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder matchmakingConfigurationName(final java.lang.String matchmakingConfigurationName) {
this.props.matchmakingConfigurationName(matchmakingConfigurationName);
return this;
}
/**
* (experimental) A matchmaking rule set to use with this configuration.
*
* A matchmaking configuration can only use rule sets that are defined in the same Region.
*
* @return {@code this}
* @param ruleSet A matchmaking rule set to use with this configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder ruleSet(final software.amazon.awscdk.services.gamelift.alpha.IMatchmakingRuleSet ruleSet) {
this.props.ruleSet(ruleSet);
return this;
}
/**
* (experimental) The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required.
*
* Default: 300 seconds
*
* @return {@code this}
* @param acceptanceTimeout The length of time (in seconds) to wait for players to accept a proposed match, if acceptance is required. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder acceptanceTimeout(final software.amazon.awscdk.Duration acceptanceTimeout) {
this.props.acceptanceTimeout(acceptanceTimeout);
return this;
}
/**
* (experimental) Information to add to all events related to the matchmaking configuration.
*
* Default: no custom data added to events
*
* @return {@code this}
* @param customEventData Information to add to all events related to the matchmaking configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder customEventData(final java.lang.String customEventData) {
this.props.customEventData(customEventData);
return this;
}
/**
* (experimental) A human-readable description of the matchmaking configuration.
*
* Default: no description is provided
*
* @return {@code this}
* @param description A human-readable description of the matchmaking configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* (experimental) An SNS topic ARN that is set up to receive matchmaking notifications.
*
* Default: no notification target
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/flexmatchguide/match-notification.html
* @param notificationTarget An SNS topic ARN that is set up to receive matchmaking notifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder notificationTarget(final software.amazon.awscdk.services.sns.ITopic notificationTarget) {
this.props.notificationTarget(notificationTarget);
return this;
}
/**
* (experimental) The maximum duration, that a matchmaking ticket can remain in process before timing out.
*
* Requests that fail due to timing out can be resubmitted as needed.
*
* Default: 300 seconds
*
* @return {@code this}
* @param requestTimeout The maximum duration, that a matchmaking ticket can remain in process before timing out. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder requestTimeout(final software.amazon.awscdk.Duration requestTimeout) {
this.props.requestTimeout(requestTimeout);
return this;
}
/**
* (experimental) A flag that determines whether a match that was created with this configuration must be accepted by the matched players.
*
* With this option enabled, matchmaking tickets use the status REQUIRES_ACCEPTANCE
to indicate when a completed potential match is waiting for player acceptance.
*
* Default: Acceptance is not required
*
* @return {@code this}
* @param requireAcceptance A flag that determines whether a match that was created with this configuration must be accepted by the matched players. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder requireAcceptance(final java.lang.Boolean requireAcceptance) {
this.props.requireAcceptance(requireAcceptance);
return this;
}
/**
* (experimental) Queues are used to start new GameLift-hosted game sessions for matches that are created with this matchmaking configuration.
*
* Queues can be located in any Region.
*
* @return {@code this}
* @param gameSessionQueues Queues are used to start new GameLift-hosted game sessions for matches that are created with this matchmaking configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder gameSessionQueues(final java.util.List extends software.amazon.awscdk.services.gamelift.alpha.IGameSessionQueue> gameSessionQueues) {
this.props.gameSessionQueues(gameSessionQueues);
return this;
}
/**
* (experimental) The number of player slots in a match to keep open for future players.
*
* For example, if the configuration's rule set specifies a match for a single 12-person team, and the additional player count is set to 2, only 10 players are selected for the match.
*
* Default: no additional player slots
*
* @return {@code this}
* @param additionalPlayerCount The number of player slots in a match to keep open for future players. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder additionalPlayerCount(final java.lang.Number additionalPlayerCount) {
this.props.additionalPlayerCount(additionalPlayerCount);
return this;
}
/**
* (experimental) A set of custom properties for a game session, formatted as key-value pairs.
*
* These properties are passed to a game server process with a request to start a new game session.
*
* Default: no additional game properties
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-sdk-server-api.html#gamelift-sdk-server-startsession
* @param gameProperties A set of custom properties for a game session, formatted as key-value pairs. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder gameProperties(final java.util.List extends software.amazon.awscdk.services.gamelift.alpha.GameProperty> gameProperties) {
this.props.gameProperties(gameProperties);
return this;
}
/**
* (experimental) A set of custom game session properties, formatted as a single string value.
*
* This data is passed to a game server process with a request to start a new game session.
*
* Default: no additional game session data
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-sdk-server-api.html#gamelift-sdk-server-startsession
* @param gameSessionData A set of custom game session properties, formatted as a single string value. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder gameSessionData(final java.lang.String gameSessionData) {
this.props.gameSessionData(gameSessionData);
return this;
}
/**
* (experimental) The method used to backfill game sessions that are created with this matchmaking configuration.
*
*
* - Choose manual when your game manages backfill requests manually or does not use the match backfill feature.
* - Otherwise backfill is settled to automatic to have GameLift create a
StartMatchBackfill
request whenever a game session has one or more open slots.
*
*
* Default: automatic backfill mode
*
* @return {@code this}
* @see https://docs.aws.amazon.com/gamelift/latest/flexmatchguide/match-backfill.html
* @param manualBackfillMode The method used to backfill game sessions that are created with this matchmaking configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder manualBackfillMode(final java.lang.Boolean manualBackfillMode) {
this.props.manualBackfillMode(manualBackfillMode);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration build() {
return new software.amazon.awscdk.services.gamelift.alpha.QueuedMatchmakingConfiguration(
this.scope,
this.id,
this.props.build()
);
}
}
}