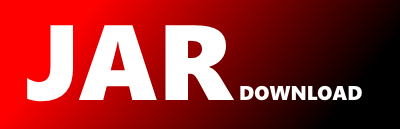
software.amazon.awscdk.services.globalaccelerator.EndpointGroupProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of globalaccelerator Show documentation
Show all versions of globalaccelerator Show documentation
The CDK Construct Library for AWS::GlobalAccelerator
package software.amazon.awscdk.services.globalaccelerator;
/**
* Property of the EndpointGroup.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.globalaccelerator.*;
* import software.amazon.awscdk.core.*;
* IEndpoint endpoint;
* Listener listener;
* EndpointGroupProps endpointGroupProps = EndpointGroupProps.builder()
* .listener(listener)
* // the properties below are optional
* .endpointGroupName("endpointGroupName")
* .endpoints(List.of(endpoint))
* .healthCheckInterval(Duration.minutes(30))
* .healthCheckPath("healthCheckPath")
* .healthCheckPort(123)
* .healthCheckProtocol(HealthCheckProtocol.TCP)
* .healthCheckThreshold(123)
* .portOverrides(List.of(PortOverride.builder()
* .endpointPort(123)
* .listenerPort(123)
* .build()))
* .region("region")
* .trafficDialPercentage(123)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-08T16:00:31.683Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.globalaccelerator.$Module.class, fqn = "@aws-cdk/aws-globalaccelerator.EndpointGroupProps")
@software.amazon.jsii.Jsii.Proxy(EndpointGroupProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface EndpointGroupProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.globalaccelerator.EndpointGroupOptions {
/**
* The Amazon Resource Name (ARN) of the listener.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.globalaccelerator.IListener getListener();
/**
* @return a {@link Builder} of {@link EndpointGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link EndpointGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.globalaccelerator.IListener listener;
java.lang.String endpointGroupName;
java.util.List endpoints;
software.amazon.awscdk.core.Duration healthCheckInterval;
java.lang.String healthCheckPath;
java.lang.Number healthCheckPort;
software.amazon.awscdk.services.globalaccelerator.HealthCheckProtocol healthCheckProtocol;
java.lang.Number healthCheckThreshold;
java.util.List portOverrides;
java.lang.String region;
java.lang.Number trafficDialPercentage;
/**
* Sets the value of {@link EndpointGroupProps#getListener}
* @param listener The Amazon Resource Name (ARN) of the listener. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder listener(software.amazon.awscdk.services.globalaccelerator.IListener listener) {
this.listener = listener;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getEndpointGroupName}
* @param endpointGroupName Name of the endpoint group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder endpointGroupName(java.lang.String endpointGroupName) {
this.endpointGroupName = endpointGroupName;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getEndpoints}
* @param endpoints Initial list of endpoints for this group.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder endpoints(java.util.List extends software.amazon.awscdk.services.globalaccelerator.IEndpoint> endpoints) {
this.endpoints = (java.util.List)endpoints;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getHealthCheckInterval}
* @param healthCheckInterval The time between health checks for each endpoint.
* Must be either 10 or 30 seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckInterval(software.amazon.awscdk.core.Duration healthCheckInterval) {
this.healthCheckInterval = healthCheckInterval;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getHealthCheckPath}
* @param healthCheckPath The ping path for health checks (if the protocol is HTTP(S)).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckPath(java.lang.String healthCheckPath) {
this.healthCheckPath = healthCheckPath;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getHealthCheckPort}
* @param healthCheckPort The port used to perform health checks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckPort(java.lang.Number healthCheckPort) {
this.healthCheckPort = healthCheckPort;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getHealthCheckProtocol}
* @param healthCheckProtocol The protocol used to perform health checks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckProtocol(software.amazon.awscdk.services.globalaccelerator.HealthCheckProtocol healthCheckProtocol) {
this.healthCheckProtocol = healthCheckProtocol;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getHealthCheckThreshold}
* @param healthCheckThreshold The number of consecutive health checks required to set the state of a healthy endpoint to unhealthy, or to set an unhealthy endpoint to healthy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckThreshold(java.lang.Number healthCheckThreshold) {
this.healthCheckThreshold = healthCheckThreshold;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getPortOverrides}
* @param portOverrides Override the destination ports used to route traffic to an endpoint.
* Unless overridden, the port used to hit the endpoint will be the same as the port
* that traffic arrives on at the listener.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder portOverrides(java.util.List extends software.amazon.awscdk.services.globalaccelerator.PortOverride> portOverrides) {
this.portOverrides = (java.util.List)portOverrides;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getRegion}
* @param region The AWS Region where the endpoint group is located.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of {@link EndpointGroupProps#getTrafficDialPercentage}
* @param trafficDialPercentage The percentage of traffic to send to this AWS Region.
* The percentage is applied to the traffic that would otherwise have been
* routed to the Region based on optimal routing. Additional traffic is
* distributed to other endpoint groups for this listener.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder trafficDialPercentage(java.lang.Number trafficDialPercentage) {
this.trafficDialPercentage = trafficDialPercentage;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link EndpointGroupProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public EndpointGroupProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link EndpointGroupProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements EndpointGroupProps {
private final software.amazon.awscdk.services.globalaccelerator.IListener listener;
private final java.lang.String endpointGroupName;
private final java.util.List endpoints;
private final software.amazon.awscdk.core.Duration healthCheckInterval;
private final java.lang.String healthCheckPath;
private final java.lang.Number healthCheckPort;
private final software.amazon.awscdk.services.globalaccelerator.HealthCheckProtocol healthCheckProtocol;
private final java.lang.Number healthCheckThreshold;
private final java.util.List portOverrides;
private final java.lang.String region;
private final java.lang.Number trafficDialPercentage;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.listener = software.amazon.jsii.Kernel.get(this, "listener", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.globalaccelerator.IListener.class));
this.endpointGroupName = software.amazon.jsii.Kernel.get(this, "endpointGroupName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.endpoints = software.amazon.jsii.Kernel.get(this, "endpoints", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.globalaccelerator.IEndpoint.class)));
this.healthCheckInterval = software.amazon.jsii.Kernel.get(this, "healthCheckInterval", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.healthCheckPath = software.amazon.jsii.Kernel.get(this, "healthCheckPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.healthCheckPort = software.amazon.jsii.Kernel.get(this, "healthCheckPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.healthCheckProtocol = software.amazon.jsii.Kernel.get(this, "healthCheckProtocol", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.globalaccelerator.HealthCheckProtocol.class));
this.healthCheckThreshold = software.amazon.jsii.Kernel.get(this, "healthCheckThreshold", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.portOverrides = software.amazon.jsii.Kernel.get(this, "portOverrides", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.globalaccelerator.PortOverride.class)));
this.region = software.amazon.jsii.Kernel.get(this, "region", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.trafficDialPercentage = software.amazon.jsii.Kernel.get(this, "trafficDialPercentage", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.listener = java.util.Objects.requireNonNull(builder.listener, "listener is required");
this.endpointGroupName = builder.endpointGroupName;
this.endpoints = (java.util.List)builder.endpoints;
this.healthCheckInterval = builder.healthCheckInterval;
this.healthCheckPath = builder.healthCheckPath;
this.healthCheckPort = builder.healthCheckPort;
this.healthCheckProtocol = builder.healthCheckProtocol;
this.healthCheckThreshold = builder.healthCheckThreshold;
this.portOverrides = (java.util.List)builder.portOverrides;
this.region = builder.region;
this.trafficDialPercentage = builder.trafficDialPercentage;
}
@Override
public final software.amazon.awscdk.services.globalaccelerator.IListener getListener() {
return this.listener;
}
@Override
public final java.lang.String getEndpointGroupName() {
return this.endpointGroupName;
}
@Override
public final java.util.List getEndpoints() {
return this.endpoints;
}
@Override
public final software.amazon.awscdk.core.Duration getHealthCheckInterval() {
return this.healthCheckInterval;
}
@Override
public final java.lang.String getHealthCheckPath() {
return this.healthCheckPath;
}
@Override
public final java.lang.Number getHealthCheckPort() {
return this.healthCheckPort;
}
@Override
public final software.amazon.awscdk.services.globalaccelerator.HealthCheckProtocol getHealthCheckProtocol() {
return this.healthCheckProtocol;
}
@Override
public final java.lang.Number getHealthCheckThreshold() {
return this.healthCheckThreshold;
}
@Override
public final java.util.List getPortOverrides() {
return this.portOverrides;
}
@Override
public final java.lang.String getRegion() {
return this.region;
}
@Override
public final java.lang.Number getTrafficDialPercentage() {
return this.trafficDialPercentage;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("listener", om.valueToTree(this.getListener()));
if (this.getEndpointGroupName() != null) {
data.set("endpointGroupName", om.valueToTree(this.getEndpointGroupName()));
}
if (this.getEndpoints() != null) {
data.set("endpoints", om.valueToTree(this.getEndpoints()));
}
if (this.getHealthCheckInterval() != null) {
data.set("healthCheckInterval", om.valueToTree(this.getHealthCheckInterval()));
}
if (this.getHealthCheckPath() != null) {
data.set("healthCheckPath", om.valueToTree(this.getHealthCheckPath()));
}
if (this.getHealthCheckPort() != null) {
data.set("healthCheckPort", om.valueToTree(this.getHealthCheckPort()));
}
if (this.getHealthCheckProtocol() != null) {
data.set("healthCheckProtocol", om.valueToTree(this.getHealthCheckProtocol()));
}
if (this.getHealthCheckThreshold() != null) {
data.set("healthCheckThreshold", om.valueToTree(this.getHealthCheckThreshold()));
}
if (this.getPortOverrides() != null) {
data.set("portOverrides", om.valueToTree(this.getPortOverrides()));
}
if (this.getRegion() != null) {
data.set("region", om.valueToTree(this.getRegion()));
}
if (this.getTrafficDialPercentage() != null) {
data.set("trafficDialPercentage", om.valueToTree(this.getTrafficDialPercentage()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-globalaccelerator.EndpointGroupProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EndpointGroupProps.Jsii$Proxy that = (EndpointGroupProps.Jsii$Proxy) o;
if (!listener.equals(that.listener)) return false;
if (this.endpointGroupName != null ? !this.endpointGroupName.equals(that.endpointGroupName) : that.endpointGroupName != null) return false;
if (this.endpoints != null ? !this.endpoints.equals(that.endpoints) : that.endpoints != null) return false;
if (this.healthCheckInterval != null ? !this.healthCheckInterval.equals(that.healthCheckInterval) : that.healthCheckInterval != null) return false;
if (this.healthCheckPath != null ? !this.healthCheckPath.equals(that.healthCheckPath) : that.healthCheckPath != null) return false;
if (this.healthCheckPort != null ? !this.healthCheckPort.equals(that.healthCheckPort) : that.healthCheckPort != null) return false;
if (this.healthCheckProtocol != null ? !this.healthCheckProtocol.equals(that.healthCheckProtocol) : that.healthCheckProtocol != null) return false;
if (this.healthCheckThreshold != null ? !this.healthCheckThreshold.equals(that.healthCheckThreshold) : that.healthCheckThreshold != null) return false;
if (this.portOverrides != null ? !this.portOverrides.equals(that.portOverrides) : that.portOverrides != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
return this.trafficDialPercentage != null ? this.trafficDialPercentage.equals(that.trafficDialPercentage) : that.trafficDialPercentage == null;
}
@Override
public final int hashCode() {
int result = this.listener.hashCode();
result = 31 * result + (this.endpointGroupName != null ? this.endpointGroupName.hashCode() : 0);
result = 31 * result + (this.endpoints != null ? this.endpoints.hashCode() : 0);
result = 31 * result + (this.healthCheckInterval != null ? this.healthCheckInterval.hashCode() : 0);
result = 31 * result + (this.healthCheckPath != null ? this.healthCheckPath.hashCode() : 0);
result = 31 * result + (this.healthCheckPort != null ? this.healthCheckPort.hashCode() : 0);
result = 31 * result + (this.healthCheckProtocol != null ? this.healthCheckProtocol.hashCode() : 0);
result = 31 * result + (this.healthCheckThreshold != null ? this.healthCheckThreshold.hashCode() : 0);
result = 31 * result + (this.portOverrides != null ? this.portOverrides.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.trafficDialPercentage != null ? this.trafficDialPercentage.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy