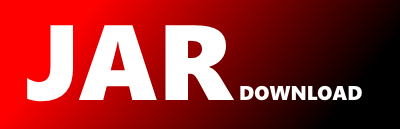
software.amazon.awscdk.services.iot1click.CfnProject Maven / Gradle / Ivy
Show all versions of iot1click Show documentation
package software.amazon.awscdk.services.iot1click;
/**
* A CloudFormation `AWS::IoT1Click::Project`.
*
* The AWS::IoT1Click::Project
resource creates an empty project with a placement template. A project contains zero or more placements that adhere to the placement template defined in the project. For more information, see CreateProject in the AWS IoT 1-Click Projects API Reference .
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iot1click.*;
* Object callbackOverrides;
* Object defaultAttributes;
* CfnProject cfnProject = CfnProject.Builder.create(this, "MyCfnProject")
* .placementTemplate(PlacementTemplateProperty.builder()
* .defaultAttributes(defaultAttributes)
* .deviceTemplates(Map.of(
* "deviceTemplatesKey", DeviceTemplateProperty.builder()
* .callbackOverrides(callbackOverrides)
* .deviceType("deviceType")
* .build()))
* .build())
* // the properties below are optional
* .description("description")
* .projectName("projectName")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.72.0 (build 4b8828b)", date = "2022-12-27T20:28:35.612Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iot1click.$Module.class, fqn = "@aws-cdk/aws-iot1click.CfnProject")
public class CfnProject extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnProject(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnProject(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.iot1click.CfnProject.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::IoT1Click::Project`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnProject(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iot1click.CfnProjectProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The Amazon Resource Name (ARN) of the project, such as `arn:aws:iot1click:us-east-1:123456789012:projects/project-a1bzhi` .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrArn() {
return software.amazon.jsii.Kernel.get(this, "attrArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the project, such as `project-a1bzhi` .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrProjectName() {
return software.amazon.jsii.Kernel.get(this, "attrProjectName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* An object describing the project's placement specifications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Object getPlacementTemplate() {
return software.amazon.jsii.Kernel.get(this, "placementTemplate", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* An object describing the project's placement specifications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementTemplate(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "placementTemplate", java.util.Objects.requireNonNull(value, "placementTemplate is required"));
}
/**
* An object describing the project's placement specifications.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setPlacementTemplate(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iot1click.CfnProject.PlacementTemplateProperty value) {
software.amazon.jsii.Kernel.set(this, "placementTemplate", java.util.Objects.requireNonNull(value, "placementTemplate is required"));
}
/**
* The description of the project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* The name of the project from which to obtain information.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getProjectName() {
return software.amazon.jsii.Kernel.get(this, "projectName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the project from which to obtain information.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setProjectName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "projectName", value);
}
/**
* In AWS CloudFormation , use the `DeviceTemplate` property type to define the template for an AWS IoT 1-Click project.
*
* DeviceTemplate
is a property of the AWS::IoT1Click::Project
resource.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iot1click.*;
* Object callbackOverrides;
* DeviceTemplateProperty deviceTemplateProperty = DeviceTemplateProperty.builder()
* .callbackOverrides(callbackOverrides)
* .deviceType("deviceType")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iot1click.$Module.class, fqn = "@aws-cdk/aws-iot1click.CfnProject.DeviceTemplateProperty")
@software.amazon.jsii.Jsii.Proxy(DeviceTemplateProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DeviceTemplateProperty extends software.amazon.jsii.JsiiSerializable {
/**
* An optional AWS Lambda function to invoke instead of the default AWS Lambda function provided by the placement template.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getCallbackOverrides() {
return null;
}
/**
* The device type, which currently must be `"button"` .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDeviceType() {
return null;
}
/**
* @return a {@link Builder} of {@link DeviceTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DeviceTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object callbackOverrides;
java.lang.String deviceType;
/**
* Sets the value of {@link DeviceTemplateProperty#getCallbackOverrides}
* @param callbackOverrides An optional AWS Lambda function to invoke instead of the default AWS Lambda function provided by the placement template.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder callbackOverrides(java.lang.Object callbackOverrides) {
this.callbackOverrides = callbackOverrides;
return this;
}
/**
* Sets the value of {@link DeviceTemplateProperty#getDeviceType}
* @param deviceType The device type, which currently must be `"button"` .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deviceType(java.lang.String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DeviceTemplateProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DeviceTemplateProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DeviceTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DeviceTemplateProperty {
private final java.lang.Object callbackOverrides;
private final java.lang.String deviceType;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.callbackOverrides = software.amazon.jsii.Kernel.get(this, "callbackOverrides", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.deviceType = software.amazon.jsii.Kernel.get(this, "deviceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.callbackOverrides = builder.callbackOverrides;
this.deviceType = builder.deviceType;
}
@Override
public final java.lang.Object getCallbackOverrides() {
return this.callbackOverrides;
}
@Override
public final java.lang.String getDeviceType() {
return this.deviceType;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCallbackOverrides() != null) {
data.set("callbackOverrides", om.valueToTree(this.getCallbackOverrides()));
}
if (this.getDeviceType() != null) {
data.set("deviceType", om.valueToTree(this.getDeviceType()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iot1click.CfnProject.DeviceTemplateProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeviceTemplateProperty.Jsii$Proxy that = (DeviceTemplateProperty.Jsii$Proxy) o;
if (this.callbackOverrides != null ? !this.callbackOverrides.equals(that.callbackOverrides) : that.callbackOverrides != null) return false;
return this.deviceType != null ? this.deviceType.equals(that.deviceType) : that.deviceType == null;
}
@Override
public final int hashCode() {
int result = this.callbackOverrides != null ? this.callbackOverrides.hashCode() : 0;
result = 31 * result + (this.deviceType != null ? this.deviceType.hashCode() : 0);
return result;
}
}
}
/**
* In AWS CloudFormation , use the `PlacementTemplate` property type to define the template for an AWS IoT 1-Click project.
*
* PlacementTemplate
is a property of the AWS::IoT1Click::Project
resource.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iot1click.*;
* Object callbackOverrides;
* Object defaultAttributes;
* PlacementTemplateProperty placementTemplateProperty = PlacementTemplateProperty.builder()
* .defaultAttributes(defaultAttributes)
* .deviceTemplates(Map.of(
* "deviceTemplatesKey", DeviceTemplateProperty.builder()
* .callbackOverrides(callbackOverrides)
* .deviceType("deviceType")
* .build()))
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iot1click.$Module.class, fqn = "@aws-cdk/aws-iot1click.CfnProject.PlacementTemplateProperty")
@software.amazon.jsii.Jsii.Proxy(PlacementTemplateProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PlacementTemplateProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The default attributes (key-value pairs) to be applied to all placements using this template.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDefaultAttributes() {
return null;
}
/**
* An object specifying the [DeviceTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_DeviceTemplate.html) for all placements using this ( [PlacementTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_PlacementTemplate.html) ) template.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDeviceTemplates() {
return null;
}
/**
* @return a {@link Builder} of {@link PlacementTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PlacementTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object defaultAttributes;
java.lang.Object deviceTemplates;
/**
* Sets the value of {@link PlacementTemplateProperty#getDefaultAttributes}
* @param defaultAttributes The default attributes (key-value pairs) to be applied to all placements using this template.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultAttributes(java.lang.Object defaultAttributes) {
this.defaultAttributes = defaultAttributes;
return this;
}
/**
* Sets the value of {@link PlacementTemplateProperty#getDeviceTemplates}
* @param deviceTemplates An object specifying the [DeviceTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_DeviceTemplate.html) for all placements using this ( [PlacementTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_PlacementTemplate.html) ) template.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deviceTemplates(software.amazon.awscdk.core.IResolvable deviceTemplates) {
this.deviceTemplates = deviceTemplates;
return this;
}
/**
* Sets the value of {@link PlacementTemplateProperty#getDeviceTemplates}
* @param deviceTemplates An object specifying the [DeviceTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_DeviceTemplate.html) for all placements using this ( [PlacementTemplate](https://docs.aws.amazon.com/iot-1-click/latest/projects-apireference/API_PlacementTemplate.html) ) template.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deviceTemplates(java.util.Map deviceTemplates) {
this.deviceTemplates = deviceTemplates;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PlacementTemplateProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PlacementTemplateProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PlacementTemplateProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PlacementTemplateProperty {
private final java.lang.Object defaultAttributes;
private final java.lang.Object deviceTemplates;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.defaultAttributes = software.amazon.jsii.Kernel.get(this, "defaultAttributes", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.deviceTemplates = software.amazon.jsii.Kernel.get(this, "deviceTemplates", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.defaultAttributes = builder.defaultAttributes;
this.deviceTemplates = builder.deviceTemplates;
}
@Override
public final java.lang.Object getDefaultAttributes() {
return this.defaultAttributes;
}
@Override
public final java.lang.Object getDeviceTemplates() {
return this.deviceTemplates;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDefaultAttributes() != null) {
data.set("defaultAttributes", om.valueToTree(this.getDefaultAttributes()));
}
if (this.getDeviceTemplates() != null) {
data.set("deviceTemplates", om.valueToTree(this.getDeviceTemplates()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iot1click.CfnProject.PlacementTemplateProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PlacementTemplateProperty.Jsii$Proxy that = (PlacementTemplateProperty.Jsii$Proxy) o;
if (this.defaultAttributes != null ? !this.defaultAttributes.equals(that.defaultAttributes) : that.defaultAttributes != null) return false;
return this.deviceTemplates != null ? this.deviceTemplates.equals(that.deviceTemplates) : that.deviceTemplates == null;
}
@Override
public final int hashCode() {
int result = this.defaultAttributes != null ? this.defaultAttributes.hashCode() : 0;
result = 31 * result + (this.deviceTemplates != null ? this.deviceTemplates.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.iot1click.CfnProject}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.iot1click.CfnProjectProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.iot1click.CfnProjectProps.Builder();
}
/**
* An object describing the project's placement specifications.
*
* @return {@code this}
* @param placementTemplate An object describing the project's placement specifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementTemplate(final software.amazon.awscdk.core.IResolvable placementTemplate) {
this.props.placementTemplate(placementTemplate);
return this;
}
/**
* An object describing the project's placement specifications.
*
* @return {@code this}
* @param placementTemplate An object describing the project's placement specifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder placementTemplate(final software.amazon.awscdk.services.iot1click.CfnProject.PlacementTemplateProperty placementTemplate) {
this.props.placementTemplate(placementTemplate);
return this;
}
/**
* The description of the project.
*
* @return {@code this}
* @param description The description of the project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The name of the project from which to obtain information.
*
* @return {@code this}
* @param projectName The name of the project from which to obtain information. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectName(final java.lang.String projectName) {
this.props.projectName(projectName);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.iot1click.CfnProject}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.iot1click.CfnProject build() {
return new software.amazon.awscdk.services.iot1click.CfnProject(
this.scope,
this.id,
this.props.build()
);
}
}
}