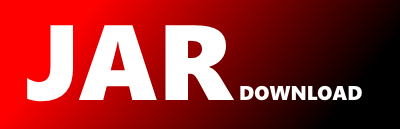
software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration Maven / Gradle / Ivy
Show all versions of iotevents-actions-alpha Show documentation
package software.amazon.awscdk.services.iotevents.actions.alpha;
/**
* (experimental) The duration of the timer.
*
* Example:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* import software.amazon.awscdk.services.iotevents.alpha.*;
* import software.amazon.awscdk.services.iotevents.actions.alpha.*;
* IInput input;
* State state = State.Builder.create()
* .stateName("MyState")
* .onEnter(List.of(Event.builder()
* .eventName("test-event")
* .condition(Expression.currentInput(input))
* .actions(List.of(
* new SetTimerAction("MyTimer", Map.of(
* "duration", cdk.Duration.seconds(60)))))
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.91.0 (build 1b1f239)", date = "2023-11-16T22:34:13.240Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iotevents.actions.alpha.$Module.class, fqn = "@aws-cdk/aws-iotevents-actions-alpha.TimerDuration")
public abstract class TimerDuration extends software.amazon.jsii.JsiiObject {
protected TimerDuration(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected TimerDuration(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
protected TimerDuration() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* (experimental) Create a timer-duration from Duration.
*
* The range of the duration is 60-31622400 seconds.
* The evaluated result of the duration expression is rounded down to the nearest whole number.
* For example, if you set the timer to 60.99 seconds, the evaluated result of the duration expression is 60 seconds.
*
* @param duration This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration fromDuration(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.Duration duration) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration.class, "fromDuration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration.class), new Object[] { java.util.Objects.requireNonNull(duration, "duration is required") });
}
/**
* (experimental) Create a timer-duration from Expression.
*
* You can use a string expression that includes numbers, variables ($variable.),
* and input values ($input..) as the duration.
*
* The range of the duration is 60-31622400 seconds.
* The evaluated result of the duration expression is rounded down to the nearest whole number.
* For example, if you set the timer to 60.99 seconds, the evaluated result of the duration expression is 60 seconds.
*
* @param expression This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration fromExpression(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iotevents.alpha.Expression expression) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration.class, "fromExpression", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration.class), new Object[] { java.util.Objects.requireNonNull(expression, "expression is required") });
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
@software.amazon.jsii.Internal
private static final class Jsii$Proxy extends software.amazon.awscdk.services.iotevents.actions.alpha.TimerDuration {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
}
}