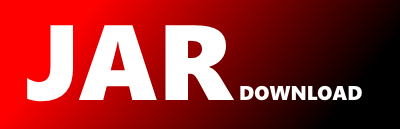
software.amazon.awscdk.services.iottwinmaker.CfnEntity Maven / Gradle / Ivy
package software.amazon.awscdk.services.iottwinmaker;
/**
* A CloudFormation `AWS::IoTTwinMaker::Entity`.
*
* Use the AWS::IoTTwinMaker::Entity
resource to declare an entity.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iottwinmaker.*;
* DataValueProperty dataValueProperty_;
* Object definition;
* Object error;
* Object relationshipValue;
* CfnEntity cfnEntity = CfnEntity.Builder.create(this, "MyCfnEntity")
* .entityName("entityName")
* .workspaceId("workspaceId")
* // the properties below are optional
* .components(Map.of(
* "componentsKey", ComponentProperty.builder()
* .componentName("componentName")
* .componentTypeId("componentTypeId")
* .definedIn("definedIn")
* .description("description")
* .properties(Map.of(
* "propertiesKey", PropertyProperty.builder()
* .definition(definition)
* .value(DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(dataValueProperty_))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", dataValueProperty_))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build())
* .build()))
* .status(StatusProperty.builder()
* .error(error)
* .state("state")
* .build())
* .build()))
* .description("description")
* .entityId("entityId")
* .parentEntityId("parentEntityId")
* .tags(Map.of(
* "tagsKey", "tags"))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.70.0 (build 03c2f6f)", date = "2022-10-27T14:10:40.859Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iottwinmaker.$Module.class, fqn = "@aws-cdk/aws-iottwinmaker.CfnEntity")
public class CfnEntity extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnEntity(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnEntity(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.iottwinmaker.CfnEntity.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::IoTTwinMaker::Entity`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnEntity(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iottwinmaker.CfnEntityProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The entity ARN.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrArn() {
return software.amazon.jsii.Kernel.get(this, "attrArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The date and time the entity was created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCreationDateTime() {
return software.amazon.jsii.Kernel.get(this, "attrCreationDateTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A boolean value that specifies whether the entity has child entities or not.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.IResolvable getAttrHasChildEntities() {
return software.amazon.jsii.Kernel.get(this, "attrHasChildEntities", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.IResolvable.class));
}
/**
* The date and time when the component type was last updated.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrUpdateDateTime() {
return software.amazon.jsii.Kernel.get(this, "attrUpdateDateTime", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* Metadata that you can use to manage the entity.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* The entity name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getEntityName() {
return software.amazon.jsii.Kernel.get(this, "entityName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The entity name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEntityName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "entityName", java.util.Objects.requireNonNull(value, "entityName is required"));
}
/**
* The ID of the workspace.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getWorkspaceId() {
return software.amazon.jsii.Kernel.get(this, "workspaceId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The ID of the workspace.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setWorkspaceId(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "workspaceId", java.util.Objects.requireNonNull(value, "workspaceId is required"));
}
/**
* An object that maps strings to the components in the entity.
*
* Each string in the mapping must be unique to this object.
*
* For information on the component object see the component API reference.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getComponents() {
return software.amazon.jsii.Kernel.get(this, "components", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* An object that maps strings to the components in the entity.
*
* Each string in the mapping must be unique to this object.
*
* For information on the component object see the component API reference.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setComponents(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "components", value);
}
/**
* An object that maps strings to the components in the entity.
*
* Each string in the mapping must be unique to this object.
*
* For information on the component object see the component API reference.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setComponents(final @org.jetbrains.annotations.Nullable java.util.Map value) {
if (software.amazon.jsii.Configuration.getRuntimeTypeChecking()) {
if (!(value.keySet().toArray()[0] instanceof String)) {
throw new IllegalArgumentException(
new java.lang.StringBuilder("Expected ")
.append("value").append(".keySet()")
.append(" to contain class String; received ")
.append(value.keySet().toArray()[0].getClass()).toString());
}
for (final java.util.Map.Entry __item_ac66f0: value.entrySet()) {
final java.lang.Object __val_ac66f0 = __item_ac66f0.getValue();
if (
!(__val_ac66f0 instanceof software.amazon.awscdk.core.IResolvable)
&& !(__val_ac66f0 instanceof software.amazon.awscdk.services.iottwinmaker.CfnEntity.ComponentProperty)
&& !(__val_ac66f0.getClass().equals(software.amazon.jsii.JsiiObject.class))
) {
throw new IllegalArgumentException(
new java.lang.StringBuilder("Expected ")
.append("value").append(".get(\"").append((__item_ac66f0.getKey())).append("\")")
.append(" to be one of: software.amazon.awscdk.core.IResolvable, software.amazon.awscdk.services.iottwinmaker.CfnEntity.ComponentProperty; received ")
.append(__val_ac66f0.getClass()).toString());
}
}
}
software.amazon.jsii.Kernel.set(this, "components", value);
}
/**
* The description of the entity.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The description of the entity.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setDescription(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "description", value);
}
/**
* The entity ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getEntityId() {
return software.amazon.jsii.Kernel.get(this, "entityId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The entity ID.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setEntityId(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "entityId", value);
}
/**
* The ID of the parent entity.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getParentEntityId() {
return software.amazon.jsii.Kernel.get(this, "parentEntityId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The ID of the parent entity.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setParentEntityId(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "parentEntityId", value);
}
/**
* The entity componenet.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iottwinmaker.*;
* DataValueProperty dataValueProperty_;
* Object definition;
* Object error;
* Object relationshipValue;
* ComponentProperty componentProperty = ComponentProperty.builder()
* .componentName("componentName")
* .componentTypeId("componentTypeId")
* .definedIn("definedIn")
* .description("description")
* .properties(Map.of(
* "propertiesKey", PropertyProperty.builder()
* .definition(definition)
* .value(DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(dataValueProperty_))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", dataValueProperty_))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build())
* .build()))
* .status(StatusProperty.builder()
* .error(error)
* .state("state")
* .build())
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iottwinmaker.$Module.class, fqn = "@aws-cdk/aws-iottwinmaker.CfnEntity.ComponentProperty")
@software.amazon.jsii.Jsii.Proxy(ComponentProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface ComponentProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the component.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getComponentName() {
return null;
}
/**
* The ID of the ComponentType.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getComponentTypeId() {
return null;
}
/**
* The name of the property definition set in the request.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDefinedIn() {
return null;
}
/**
* The description of the component.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* An object that maps strings to the properties to set in the component type.
*
* Each string in the mapping must be unique to this object.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getProperties() {
return null;
}
/**
* The status of the component.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getStatus() {
return null;
}
/**
* @return a {@link Builder} of {@link ComponentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ComponentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String componentName;
java.lang.String componentTypeId;
java.lang.String definedIn;
java.lang.String description;
java.lang.Object properties;
java.lang.Object status;
/**
* Sets the value of {@link ComponentProperty#getComponentName}
* @param componentName The name of the component.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder componentName(java.lang.String componentName) {
this.componentName = componentName;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getComponentTypeId}
* @param componentTypeId The ID of the ComponentType.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder componentTypeId(java.lang.String componentTypeId) {
this.componentTypeId = componentTypeId;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getDefinedIn}
* @param definedIn The name of the property definition set in the request.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder definedIn(java.lang.String definedIn) {
this.definedIn = definedIn;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getDescription}
* @param description The description of the component.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getProperties}
* @param properties An object that maps strings to the properties to set in the component type.
* Each string in the mapping must be unique to this object.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder properties(software.amazon.awscdk.core.IResolvable properties) {
this.properties = properties;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getProperties}
* @param properties An object that maps strings to the properties to set in the component type.
* Each string in the mapping must be unique to this object.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder properties(java.util.Map properties) {
this.properties = properties;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getStatus}
* @param status The status of the component.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder status(software.amazon.awscdk.core.IResolvable status) {
this.status = status;
return this;
}
/**
* Sets the value of {@link ComponentProperty#getStatus}
* @param status The status of the component.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder status(software.amazon.awscdk.services.iottwinmaker.CfnEntity.StatusProperty status) {
this.status = status;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ComponentProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ComponentProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ComponentProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ComponentProperty {
private final java.lang.String componentName;
private final java.lang.String componentTypeId;
private final java.lang.String definedIn;
private final java.lang.String description;
private final java.lang.Object properties;
private final java.lang.Object status;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.componentName = software.amazon.jsii.Kernel.get(this, "componentName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.componentTypeId = software.amazon.jsii.Kernel.get(this, "componentTypeId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.definedIn = software.amazon.jsii.Kernel.get(this, "definedIn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.properties = software.amazon.jsii.Kernel.get(this, "properties", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.status = software.amazon.jsii.Kernel.get(this, "status", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.componentName = builder.componentName;
this.componentTypeId = builder.componentTypeId;
this.definedIn = builder.definedIn;
this.description = builder.description;
this.properties = builder.properties;
this.status = builder.status;
}
@Override
public final java.lang.String getComponentName() {
return this.componentName;
}
@Override
public final java.lang.String getComponentTypeId() {
return this.componentTypeId;
}
@Override
public final java.lang.String getDefinedIn() {
return this.definedIn;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.Object getProperties() {
return this.properties;
}
@Override
public final java.lang.Object getStatus() {
return this.status;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getComponentName() != null) {
data.set("componentName", om.valueToTree(this.getComponentName()));
}
if (this.getComponentTypeId() != null) {
data.set("componentTypeId", om.valueToTree(this.getComponentTypeId()));
}
if (this.getDefinedIn() != null) {
data.set("definedIn", om.valueToTree(this.getDefinedIn()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getProperties() != null) {
data.set("properties", om.valueToTree(this.getProperties()));
}
if (this.getStatus() != null) {
data.set("status", om.valueToTree(this.getStatus()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iottwinmaker.CfnEntity.ComponentProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ComponentProperty.Jsii$Proxy that = (ComponentProperty.Jsii$Proxy) o;
if (this.componentName != null ? !this.componentName.equals(that.componentName) : that.componentName != null) return false;
if (this.componentTypeId != null ? !this.componentTypeId.equals(that.componentTypeId) : that.componentTypeId != null) return false;
if (this.definedIn != null ? !this.definedIn.equals(that.definedIn) : that.definedIn != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.properties != null ? !this.properties.equals(that.properties) : that.properties != null) return false;
return this.status != null ? this.status.equals(that.status) : that.status == null;
}
@Override
public final int hashCode() {
int result = this.componentName != null ? this.componentName.hashCode() : 0;
result = 31 * result + (this.componentTypeId != null ? this.componentTypeId.hashCode() : 0);
result = 31 * result + (this.definedIn != null ? this.definedIn.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.properties != null ? this.properties.hashCode() : 0);
result = 31 * result + (this.status != null ? this.status.hashCode() : 0);
return result;
}
}
}
/**
* An object that specifies a value for a property.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iottwinmaker.*;
* DataValueProperty dataValueProperty_;
* Object relationshipValue;
* DataValueProperty dataValueProperty = DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(dataValueProperty_))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", dataValueProperty_))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build()))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(dataValueProperty_))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", dataValueProperty_))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build()))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iottwinmaker.$Module.class, fqn = "@aws-cdk/aws-iottwinmaker.CfnEntity.DataValueProperty")
@software.amazon.jsii.Jsii.Proxy(DataValueProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface DataValueProperty extends software.amazon.jsii.JsiiSerializable {
/**
* A boolean value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getBooleanValue() {
return null;
}
/**
* A double value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getDoubleValue() {
return null;
}
/**
* An expression that produces the value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getExpression() {
return null;
}
/**
* An integer value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getIntegerValue() {
return null;
}
/**
* A list of multiple values.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getListValue() {
return null;
}
/**
* A long value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getLongValue() {
return null;
}
/**
* An object that maps strings to multiple DataValue objects.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getMapValue() {
return null;
}
/**
* A value that relates a component to another component.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getRelationshipValue() {
return null;
}
/**
* A string value.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getStringValue() {
return null;
}
/**
* @return a {@link Builder} of {@link DataValueProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DataValueProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object booleanValue;
java.lang.Number doubleValue;
java.lang.String expression;
java.lang.Number integerValue;
java.lang.Object listValue;
java.lang.Number longValue;
java.lang.Object mapValue;
java.lang.Object relationshipValue;
java.lang.String stringValue;
/**
* Sets the value of {@link DataValueProperty#getBooleanValue}
* @param booleanValue A boolean value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder booleanValue(java.lang.Boolean booleanValue) {
this.booleanValue = booleanValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getBooleanValue}
* @param booleanValue A boolean value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder booleanValue(software.amazon.awscdk.core.IResolvable booleanValue) {
this.booleanValue = booleanValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getDoubleValue}
* @param doubleValue A double value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder doubleValue(java.lang.Number doubleValue) {
this.doubleValue = doubleValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getExpression}
* @param expression An expression that produces the value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder expression(java.lang.String expression) {
this.expression = expression;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getIntegerValue}
* @param integerValue An integer value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder integerValue(java.lang.Number integerValue) {
this.integerValue = integerValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getListValue}
* @param listValue A list of multiple values.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder listValue(software.amazon.awscdk.core.IResolvable listValue) {
this.listValue = listValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getListValue}
* @param listValue A list of multiple values.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder listValue(java.util.List extends java.lang.Object> listValue) {
this.listValue = listValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getLongValue}
* @param longValue A long value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder longValue(java.lang.Number longValue) {
this.longValue = longValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getMapValue}
* @param mapValue An object that maps strings to multiple DataValue objects.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder mapValue(software.amazon.awscdk.core.IResolvable mapValue) {
this.mapValue = mapValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getMapValue}
* @param mapValue An object that maps strings to multiple DataValue objects.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder mapValue(java.util.Map mapValue) {
this.mapValue = mapValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getRelationshipValue}
* @param relationshipValue A value that relates a component to another component.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder relationshipValue(java.lang.Object relationshipValue) {
this.relationshipValue = relationshipValue;
return this;
}
/**
* Sets the value of {@link DataValueProperty#getStringValue}
* @param stringValue A string value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stringValue(java.lang.String stringValue) {
this.stringValue = stringValue;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DataValueProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DataValueProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DataValueProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DataValueProperty {
private final java.lang.Object booleanValue;
private final java.lang.Number doubleValue;
private final java.lang.String expression;
private final java.lang.Number integerValue;
private final java.lang.Object listValue;
private final java.lang.Number longValue;
private final java.lang.Object mapValue;
private final java.lang.Object relationshipValue;
private final java.lang.String stringValue;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.booleanValue = software.amazon.jsii.Kernel.get(this, "booleanValue", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.doubleValue = software.amazon.jsii.Kernel.get(this, "doubleValue", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.expression = software.amazon.jsii.Kernel.get(this, "expression", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.integerValue = software.amazon.jsii.Kernel.get(this, "integerValue", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.listValue = software.amazon.jsii.Kernel.get(this, "listValue", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.longValue = software.amazon.jsii.Kernel.get(this, "longValue", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.mapValue = software.amazon.jsii.Kernel.get(this, "mapValue", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.relationshipValue = software.amazon.jsii.Kernel.get(this, "relationshipValue", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.stringValue = software.amazon.jsii.Kernel.get(this, "stringValue", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.booleanValue = builder.booleanValue;
this.doubleValue = builder.doubleValue;
this.expression = builder.expression;
this.integerValue = builder.integerValue;
this.listValue = builder.listValue;
this.longValue = builder.longValue;
this.mapValue = builder.mapValue;
this.relationshipValue = builder.relationshipValue;
this.stringValue = builder.stringValue;
}
@Override
public final java.lang.Object getBooleanValue() {
return this.booleanValue;
}
@Override
public final java.lang.Number getDoubleValue() {
return this.doubleValue;
}
@Override
public final java.lang.String getExpression() {
return this.expression;
}
@Override
public final java.lang.Number getIntegerValue() {
return this.integerValue;
}
@Override
public final java.lang.Object getListValue() {
return this.listValue;
}
@Override
public final java.lang.Number getLongValue() {
return this.longValue;
}
@Override
public final java.lang.Object getMapValue() {
return this.mapValue;
}
@Override
public final java.lang.Object getRelationshipValue() {
return this.relationshipValue;
}
@Override
public final java.lang.String getStringValue() {
return this.stringValue;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getBooleanValue() != null) {
data.set("booleanValue", om.valueToTree(this.getBooleanValue()));
}
if (this.getDoubleValue() != null) {
data.set("doubleValue", om.valueToTree(this.getDoubleValue()));
}
if (this.getExpression() != null) {
data.set("expression", om.valueToTree(this.getExpression()));
}
if (this.getIntegerValue() != null) {
data.set("integerValue", om.valueToTree(this.getIntegerValue()));
}
if (this.getListValue() != null) {
data.set("listValue", om.valueToTree(this.getListValue()));
}
if (this.getLongValue() != null) {
data.set("longValue", om.valueToTree(this.getLongValue()));
}
if (this.getMapValue() != null) {
data.set("mapValue", om.valueToTree(this.getMapValue()));
}
if (this.getRelationshipValue() != null) {
data.set("relationshipValue", om.valueToTree(this.getRelationshipValue()));
}
if (this.getStringValue() != null) {
data.set("stringValue", om.valueToTree(this.getStringValue()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iottwinmaker.CfnEntity.DataValueProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DataValueProperty.Jsii$Proxy that = (DataValueProperty.Jsii$Proxy) o;
if (this.booleanValue != null ? !this.booleanValue.equals(that.booleanValue) : that.booleanValue != null) return false;
if (this.doubleValue != null ? !this.doubleValue.equals(that.doubleValue) : that.doubleValue != null) return false;
if (this.expression != null ? !this.expression.equals(that.expression) : that.expression != null) return false;
if (this.integerValue != null ? !this.integerValue.equals(that.integerValue) : that.integerValue != null) return false;
if (this.listValue != null ? !this.listValue.equals(that.listValue) : that.listValue != null) return false;
if (this.longValue != null ? !this.longValue.equals(that.longValue) : that.longValue != null) return false;
if (this.mapValue != null ? !this.mapValue.equals(that.mapValue) : that.mapValue != null) return false;
if (this.relationshipValue != null ? !this.relationshipValue.equals(that.relationshipValue) : that.relationshipValue != null) return false;
return this.stringValue != null ? this.stringValue.equals(that.stringValue) : that.stringValue == null;
}
@Override
public final int hashCode() {
int result = this.booleanValue != null ? this.booleanValue.hashCode() : 0;
result = 31 * result + (this.doubleValue != null ? this.doubleValue.hashCode() : 0);
result = 31 * result + (this.expression != null ? this.expression.hashCode() : 0);
result = 31 * result + (this.integerValue != null ? this.integerValue.hashCode() : 0);
result = 31 * result + (this.listValue != null ? this.listValue.hashCode() : 0);
result = 31 * result + (this.longValue != null ? this.longValue.hashCode() : 0);
result = 31 * result + (this.mapValue != null ? this.mapValue.hashCode() : 0);
result = 31 * result + (this.relationshipValue != null ? this.relationshipValue.hashCode() : 0);
result = 31 * result + (this.stringValue != null ? this.stringValue.hashCode() : 0);
return result;
}
}
}
/**
* An object that sets information about a property.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iottwinmaker.*;
* DataValueProperty dataValueProperty_;
* Object definition;
* Object relationshipValue;
* PropertyProperty propertyProperty = PropertyProperty.builder()
* .definition(definition)
* .value(DataValueProperty.builder()
* .booleanValue(false)
* .doubleValue(123)
* .expression("expression")
* .integerValue(123)
* .listValue(List.of(dataValueProperty_))
* .longValue(123)
* .mapValue(Map.of(
* "mapValueKey", dataValueProperty_))
* .relationshipValue(relationshipValue)
* .stringValue("stringValue")
* .build())
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iottwinmaker.$Module.class, fqn = "@aws-cdk/aws-iottwinmaker.CfnEntity.PropertyProperty")
@software.amazon.jsii.Jsii.Proxy(PropertyProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface PropertyProperty extends software.amazon.jsii.JsiiSerializable {
/**
* An object that specifies information about a property.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDefinition() {
return null;
}
/**
* An object that contains information about a value for a time series property.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getValue() {
return null;
}
/**
* @return a {@link Builder} of {@link PropertyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link PropertyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object definition;
java.lang.Object value;
/**
* Sets the value of {@link PropertyProperty#getDefinition}
* @param definition An object that specifies information about a property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder definition(java.lang.Object definition) {
this.definition = definition;
return this;
}
/**
* Sets the value of {@link PropertyProperty#getValue}
* @param value An object that contains information about a value for a time series property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(software.amazon.awscdk.core.IResolvable value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link PropertyProperty#getValue}
* @param value An object that contains information about a value for a time series property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(software.amazon.awscdk.services.iottwinmaker.CfnEntity.DataValueProperty value) {
this.value = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link PropertyProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public PropertyProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link PropertyProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements PropertyProperty {
private final java.lang.Object definition;
private final java.lang.Object value;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.definition = software.amazon.jsii.Kernel.get(this, "definition", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.definition = builder.definition;
this.value = builder.value;
}
@Override
public final java.lang.Object getDefinition() {
return this.definition;
}
@Override
public final java.lang.Object getValue() {
return this.value;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDefinition() != null) {
data.set("definition", om.valueToTree(this.getDefinition()));
}
if (this.getValue() != null) {
data.set("value", om.valueToTree(this.getValue()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iottwinmaker.CfnEntity.PropertyProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PropertyProperty.Jsii$Proxy that = (PropertyProperty.Jsii$Proxy) o;
if (this.definition != null ? !this.definition.equals(that.definition) : that.definition != null) return false;
return this.value != null ? this.value.equals(that.value) : that.value == null;
}
@Override
public final int hashCode() {
int result = this.definition != null ? this.definition.hashCode() : 0;
result = 31 * result + (this.value != null ? this.value.hashCode() : 0);
return result;
}
}
}
/**
* The current status of the entity.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.iottwinmaker.*;
* Object error;
* StatusProperty statusProperty = StatusProperty.builder()
* .error(error)
* .state("state")
* .build();
*
*/
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.iottwinmaker.$Module.class, fqn = "@aws-cdk/aws-iottwinmaker.CfnEntity.StatusProperty")
@software.amazon.jsii.Jsii.Proxy(StatusProperty.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static interface StatusProperty extends software.amazon.jsii.JsiiSerializable {
/**
* The error message.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getError() {
return null;
}
/**
* The current state of the entity, component, component type, or workspace.
*
* Valid Values: CREATING | UPDATING | DELETING | ACTIVE | ERROR
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getState() {
return null;
}
/**
* @return a {@link Builder} of {@link StatusProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link StatusProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object error;
java.lang.String state;
/**
* Sets the value of {@link StatusProperty#getError}
* @param error The error message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder error(java.lang.Object error) {
this.error = error;
return this;
}
/**
* Sets the value of {@link StatusProperty#getState}
* @param state The current state of the entity, component, component type, or workspace.
* Valid Values: CREATING | UPDATING | DELETING | ACTIVE | ERROR
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder state(java.lang.String state) {
this.state = state;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link StatusProperty}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public StatusProperty build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link StatusProperty}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements StatusProperty {
private final java.lang.Object error;
private final java.lang.String state;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.error = software.amazon.jsii.Kernel.get(this, "error", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.state = software.amazon.jsii.Kernel.get(this, "state", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.error = builder.error;
this.state = builder.state;
}
@Override
public final java.lang.Object getError() {
return this.error;
}
@Override
public final java.lang.String getState() {
return this.state;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getError() != null) {
data.set("error", om.valueToTree(this.getError()));
}
if (this.getState() != null) {
data.set("state", om.valueToTree(this.getState()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-iottwinmaker.CfnEntity.StatusProperty"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
StatusProperty.Jsii$Proxy that = (StatusProperty.Jsii$Proxy) o;
if (this.error != null ? !this.error.equals(that.error) : that.error != null) return false;
return this.state != null ? this.state.equals(that.state) : that.state == null;
}
@Override
public final int hashCode() {
int result = this.error != null ? this.error.hashCode() : 0;
result = 31 * result + (this.state != null ? this.state.hashCode() : 0);
return result;
}
}
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.iottwinmaker.CfnEntity}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.iottwinmaker.CfnEntityProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.iottwinmaker.CfnEntityProps.Builder();
}
/**
* The entity name.
*
* @return {@code this}
* @param entityName The entity name. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder entityName(final java.lang.String entityName) {
this.props.entityName(entityName);
return this;
}
/**
* The ID of the workspace.
*
* @return {@code this}
* @param workspaceId The ID of the workspace. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder workspaceId(final java.lang.String workspaceId) {
this.props.workspaceId(workspaceId);
return this;
}
/**
* An object that maps strings to the components in the entity.
*
* Each string in the mapping must be unique to this object.
*
* For information on the component object see the component API reference.
*
* @return {@code this}
* @param components An object that maps strings to the components in the entity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder components(final software.amazon.awscdk.core.IResolvable components) {
this.props.components(components);
return this;
}
/**
* An object that maps strings to the components in the entity.
*
* Each string in the mapping must be unique to this object.
*
* For information on the component object see the component API reference.
*
* @return {@code this}
* @param components An object that maps strings to the components in the entity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder components(final java.util.Map components) {
this.props.components(components);
return this;
}
/**
* The description of the entity.
*
* @return {@code this}
* @param description The description of the entity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(final java.lang.String description) {
this.props.description(description);
return this;
}
/**
* The entity ID.
*
* @return {@code this}
* @param entityId The entity ID. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder entityId(final java.lang.String entityId) {
this.props.entityId(entityId);
return this;
}
/**
* The ID of the parent entity.
*
* @return {@code this}
* @param parentEntityId The ID of the parent entity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parentEntityId(final java.lang.String parentEntityId) {
this.props.parentEntityId(parentEntityId);
return this;
}
/**
* Metadata that you can use to manage the entity.
*
* @return {@code this}
* @param tags Metadata that you can use to manage the entity. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.Map tags) {
this.props.tags(tags);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.iottwinmaker.CfnEntity}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.iottwinmaker.CfnEntity build() {
return new software.amazon.awscdk.services.iottwinmaker.CfnEntity(
this.scope,
this.id,
this.props.build()
);
}
}
}