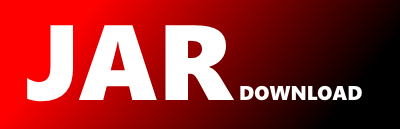
software.amazon.awscdk.services.ivs.CfnChannel Maven / Gradle / Ivy
package software.amazon.awscdk.services.ivs;
/**
* A CloudFormation `AWS::IVS::Channel`.
*
* The AWS::IVS::Channel
resource specifies an channel. A channel stores configuration information related to your live stream. For more information, see CreateChannel in the Amazon Interactive Video Service API Reference .
*
*
*
* By default, the IVS API CreateChannel endpoint creates a stream key in addition to a channel. The Channel resource does not create a stream key; to create a stream key, use the StreamKey resource instead.
*
*
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.ivs.*;
* CfnChannel cfnChannel = CfnChannel.Builder.create(this, "MyCfnChannel")
* .authorized(false)
* .latencyMode("latencyMode")
* .name("name")
* .recordingConfigurationArn("recordingConfigurationArn")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .type("type")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.72.0 (build 4b8828b)", date = "2022-12-30T16:29:40.724Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.ivs.$Module.class, fqn = "@aws-cdk/aws-ivs.CfnChannel")
public class CfnChannel extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnChannel(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnChannel(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.ivs.CfnChannel.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::IVS::Channel`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnChannel(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ivs.CfnChannelProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* Create a new `AWS::IVS::Channel`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnChannel(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* The channel ARN.
*
* For example: arn:aws:ivs:us-west-2:123456789012:channel/abcdABCDefgh
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrArn() {
return software.amazon.jsii.Kernel.get(this, "attrArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming software.
*
* For example: a1b2c3d4e5f6.global-contribute.live-video.net
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrIngestEndpoint() {
return software.amazon.jsii.Kernel.get(this, "attrIngestEndpoint", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Channel playback URL.
*
* For example: https://a1b2c3d4e5f6.us-west-2.playback.live-video.net/api/video/v1/us-west-2.123456789012.channel.abcdEFGH.m3u8
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrPlaybackUrl() {
return software.amazon.jsii.Kernel.get(this, "attrPlaybackUrl", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* An array of key-value pairs to apply to this resource.
*
* For more information, see Tag .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TagManager getTags() {
return software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.TagManager.class));
}
/**
* Whether the channel is authorized.
*
* Default : false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getAuthorized() {
return software.amazon.jsii.Kernel.get(this, "authorized", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Whether the channel is authorized.
*
* Default : false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAuthorized(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "authorized", value);
}
/**
* Whether the channel is authorized.
*
* Default : false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAuthorized(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "authorized", value);
}
/**
* Channel latency mode. Valid values:.
*
*
* NORMAL
: Use NORMAL to broadcast and deliver live video up to Full HD.
* LOW
: Use LOW for near real-time interactions with viewers.
*
*
*
*
* In the console, LOW
and NORMAL
correspond to Ultra-low
and Standard
, respectively.
*
*
*
* Default : LOW
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getLatencyMode() {
return software.amazon.jsii.Kernel.get(this, "latencyMode", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Channel latency mode. Valid values:.
*
*
* NORMAL
: Use NORMAL to broadcast and deliver live video up to Full HD.
* LOW
: Use LOW for near real-time interactions with viewers.
*
*
*
*
* In the console, LOW
and NORMAL
correspond to Ultra-low
and Standard
, respectively.
*
*
*
* Default : LOW
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLatencyMode(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "latencyMode", value);
}
/**
* Channel name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getName() {
return software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Channel name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "name", value);
}
/**
* The ARN of a RecordingConfiguration resource.
*
* An empty string indicates that recording is disabled for the channel. A RecordingConfiguration ARN indicates that recording is enabled using the specified recording configuration. See the RecordingConfiguration resource for more information and an example.
*
* Default : "" (empty string, recording is disabled)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getRecordingConfigurationArn() {
return software.amazon.jsii.Kernel.get(this, "recordingConfigurationArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The ARN of a RecordingConfiguration resource.
*
* An empty string indicates that recording is disabled for the channel. A RecordingConfiguration ARN indicates that recording is enabled using the specified recording configuration. See the RecordingConfiguration resource for more information and an example.
*
* Default : "" (empty string, recording is disabled)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setRecordingConfigurationArn(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "recordingConfigurationArn", value);
}
/**
* The channel type, which determines the allowable resolution and bitrate.
*
* If you exceed the allowable resolution or bitrate, the stream probably will disconnect immediately. Valid values:
*
*
* STANDARD
: Video is transcoded: multiple qualities are generated from the original input to automatically give viewers the best experience for their devices and network conditions. Transcoding allows higher playback quality across a range of download speeds. Resolution can be up to 1080p and bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
* BASIC
: Video is transmuxed: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is limited to the original input. Resolution can be up to 1080p and bitrate can be up to 1.5 Mbps for 480p and up to 3.5 Mbps for resolutions between 480p and 1080p.
*
*
* Default : STANDARD
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getType() {
return software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The channel type, which determines the allowable resolution and bitrate.
*
* If you exceed the allowable resolution or bitrate, the stream probably will disconnect immediately. Valid values:
*
*
* STANDARD
: Video is transcoded: multiple qualities are generated from the original input to automatically give viewers the best experience for their devices and network conditions. Transcoding allows higher playback quality across a range of download speeds. Resolution can be up to 1080p and bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
* BASIC
: Video is transmuxed: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is limited to the original input. Resolution can be up to 1080p and bitrate can be up to 1.5 Mbps for 480p and up to 3.5 Mbps for resolutions between 480p and 1080p.
*
*
* Default : STANDARD
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setType(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "type", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.ivs.CfnChannel}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private software.amazon.awscdk.services.ivs.CfnChannelProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* Whether the channel is authorized.
*
* Default : false
*
* @return {@code this}
* @param authorized Whether the channel is authorized. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authorized(final java.lang.Boolean authorized) {
this.props().authorized(authorized);
return this;
}
/**
* Whether the channel is authorized.
*
* Default : false
*
* @return {@code this}
* @param authorized Whether the channel is authorized. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authorized(final software.amazon.awscdk.core.IResolvable authorized) {
this.props().authorized(authorized);
return this;
}
/**
* Channel latency mode. Valid values:.
*
*
* NORMAL
: Use NORMAL to broadcast and deliver live video up to Full HD.
* LOW
: Use LOW for near real-time interactions with viewers.
*
*
*
*
* In the console, LOW
and NORMAL
correspond to Ultra-low
and Standard
, respectively.
*
*
*
* Default : LOW
*
* @return {@code this}
* @param latencyMode Channel latency mode. Valid values:. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder latencyMode(final java.lang.String latencyMode) {
this.props().latencyMode(latencyMode);
return this;
}
/**
* Channel name.
*
* @return {@code this}
* @param name Channel name. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(final java.lang.String name) {
this.props().name(name);
return this;
}
/**
* The ARN of a RecordingConfiguration resource.
*
* An empty string indicates that recording is disabled for the channel. A RecordingConfiguration ARN indicates that recording is enabled using the specified recording configuration. See the RecordingConfiguration resource for more information and an example.
*
* Default : "" (empty string, recording is disabled)
*
* @return {@code this}
* @param recordingConfigurationArn The ARN of a RecordingConfiguration resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder recordingConfigurationArn(final java.lang.String recordingConfigurationArn) {
this.props().recordingConfigurationArn(recordingConfigurationArn);
return this;
}
/**
* An array of key-value pairs to apply to this resource.
*
* For more information, see Tag .
*
* @return {@code this}
* @param tags An array of key-value pairs to apply to this resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tags(final java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.props().tags(tags);
return this;
}
/**
* The channel type, which determines the allowable resolution and bitrate.
*
* If you exceed the allowable resolution or bitrate, the stream probably will disconnect immediately. Valid values:
*
*
* STANDARD
: Video is transcoded: multiple qualities are generated from the original input to automatically give viewers the best experience for their devices and network conditions. Transcoding allows higher playback quality across a range of download speeds. Resolution can be up to 1080p and bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
* BASIC
: Video is transmuxed: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is limited to the original input. Resolution can be up to 1080p and bitrate can be up to 1.5 Mbps for 480p and up to 3.5 Mbps for resolutions between 480p and 1080p.
*
*
* Default : STANDARD
*
* @return {@code this}
* @param type The channel type, which determines the allowable resolution and bitrate. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(final java.lang.String type) {
this.props().type(type);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.ivs.CfnChannel}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.ivs.CfnChannel build() {
return new software.amazon.awscdk.services.ivs.CfnChannel(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.ivs.CfnChannelProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.ivs.CfnChannelProps.Builder();
}
return this.props;
}
}
}