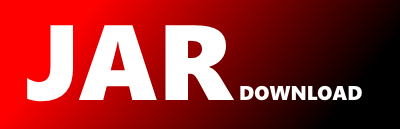
software.amazon.awscdk.services.kms.package-info Maven / Gradle / Ivy
/**
* AWS Key Management Service Construct Library
*
*
* 
*
*
* Define a KMS key:
* import kms = require('@aws-cdk/aws-kms');
*
* new kms.Key(this, 'MyKey', {
* enableKeyRotation: true
* });
*
* Add a couple of aliases:
* const key = new kms.Key(this, 'MyKey');
* key.addAlias('alias/foo');
* key.addAlias('alias/bar');
*
* Sharing keys between stacks
* To use a KMS key in a different stack in the same CDK application,
* pass the construct to the other stack:
*
* /**
* * Stack that defines the key
* *{@literal /}
* class KeyStack extends cdk.Stack {
* public readonly key: kms.Key;
*
* constructor(scope: cdk.App, id: string, props?: cdk.StackProps) {
* super(scope, id, props);
* this.key = new kms.Key(this, 'MyKey', { removalPolicy: RemovalPolicy.DESTROY });
* }
* }
*
* interface UseStackProps extends cdk.StackProps {
* key: kms.IKey; // Use IKey here
* }
*
* /**
* * Stack that uses the key
* *{@literal /}
* class UseStack extends cdk.Stack {
* constructor(scope: cdk.App, id: string, props: UseStackProps) {
* super(scope, id, props);
*
* // Use the IKey object here.
* props.key.addAlias('alias/foo');
* }
* }
*
* const keyStack = new KeyStack(app, 'KeyStack');
* new UseStack(app, 'UseStack', { key: keyStack.key });
*
* Importing existing keys
* To use a KMS key that is not defined in this CDK app, but is created through other means, use
* Key.fromKeyArn(parent, name, ref)
:
* const myKeyImported = kms.Key.fromKeyArn(this, 'MyImportedKey', 'arn:aws:...');
*
* // you can do stuff with this imported key.
* myKeyImported.addAlias('alias/foo');
*
* Note that a call to .addToPolicy(statement)
on myKeyImported
will not have
* an affect on the key's policy because it is not owned by your stack. The call
* will be a no-op.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
package software.amazon.awscdk.services.kms;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy