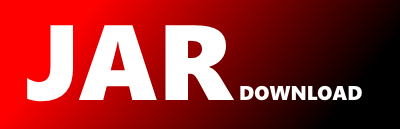
software.amazon.awscdk.services.kms.IKey Maven / Gradle / Ivy
package software.amazon.awscdk.services.kms;
/**
* A KMS Key, either managed by this CDK app, or imported.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.13.0 (build 385c325)", date = "2020-10-02T20:10:54.079Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.kms.$Module.class, fqn = "@aws-cdk/aws-kms.IKey")
@software.amazon.jsii.Jsii.Proxy(IKey.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface IKey extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.core.IResource {
/**
* The ARN of the key.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getKeyArn();
/**
* The ID of the key (the part that looks something like: 1234abcd-12ab-34cd-56ef-1234567890ab).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getKeyId();
/**
* Defines a new alias for the key.
*
* @param alias This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.kms.Alias addAlias(final @org.jetbrains.annotations.NotNull java.lang.String alias);
/**
* Adds a statement to the KMS key resource policy.
*
* @param statement The policy statement to add. This parameter is required.
* @param allowNoOp If this is set to `false` and there is no policy defined (i.e. external key), the operation will fail. Otherwise, it will no-op.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement, final @org.jetbrains.annotations.Nullable java.lang.Boolean allowNoOp);
/**
* Adds a statement to the KMS key resource policy.
*
* @param statement The policy statement to add. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement);
/**
* Grant the indicated permissions on this key to the given principal.
*
* @param grantee This parameter is required.
* @param actions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grant(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee, final @org.jetbrains.annotations.NotNull java.lang.String... actions);
/**
* Grant decryption permisisons using this key to the given principal.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grantDecrypt(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee);
/**
* Grant encryption permisisons using this key to the given principal.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grantEncrypt(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee);
/**
* Grant encryption and decryption permisisons using this key to the given principal.
*
* @param grantee This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grantEncryptDecrypt(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee);
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.kms.IKey {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
/**
* The ARN of the key.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getKeyArn() {
return this.jsiiGet("keyArn", java.lang.String.class);
}
/**
* The ID of the key (the part that looks something like: 1234abcd-12ab-34cd-56ef-1234567890ab).
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getKeyId() {
return this.jsiiGet("keyId", java.lang.String.class);
}
/**
* The environment this resource belongs to.
*
* For resources that are created and managed by the CDK
* (generally, those created by creating new class instances like Role, Bucket, etc.),
* this is always the same as the environment of the stack they belong to;
* however, for imported resources
* (those obtained from static methods like fromRoleArn, fromBucketName, etc.),
* that might be different than the stack they were imported into.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.ResourceEnvironment getEnv() {
return this.jsiiGet("env", software.amazon.awscdk.core.ResourceEnvironment.class);
}
/**
* The stack in which this resource is defined.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Stack getStack() {
return this.jsiiGet("stack", software.amazon.awscdk.core.Stack.class);
}
/**
* The construct tree node for this construct.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.ConstructNode getNode() {
return this.jsiiGet("node", software.amazon.awscdk.core.ConstructNode.class);
}
/**
* Defines a new alias for the key.
*
* @param alias This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.kms.Alias addAlias(final @org.jetbrains.annotations.NotNull java.lang.String alias) {
return this.jsiiCall("addAlias", software.amazon.awscdk.services.kms.Alias.class, new Object[] { java.util.Objects.requireNonNull(alias, "alias is required") });
}
/**
* Adds a statement to the KMS key resource policy.
*
* @param statement The policy statement to add. This parameter is required.
* @param allowNoOp If this is set to `false` and there is no policy defined (i.e. external key), the operation will fail. Otherwise, it will no-op.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement, final @org.jetbrains.annotations.Nullable java.lang.Boolean allowNoOp) {
return this.jsiiCall("addToResourcePolicy", software.amazon.awscdk.services.iam.AddToResourcePolicyResult.class, new Object[] { java.util.Objects.requireNonNull(statement, "statement is required"), allowNoOp });
}
/**
* Adds a statement to the KMS key resource policy.
*
* @param statement The policy statement to add. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.AddToResourcePolicyResult addToResourcePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement) {
return this.jsiiCall("addToResourcePolicy", software.amazon.awscdk.services.iam.AddToResourcePolicyResult.class, new Object[] { java.util.Objects.requireNonNull(statement, "statement is required") });
}
/**
* Grant the indicated permissions on this key to the given principal.
*
* @param grantee This parameter is required.
* @param actions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.Grant grant(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IGrantable grantee, final @org.jetbrains.annotations.NotNull java.lang.String... actions) {
return this.jsiiCall("grant", software.amazon.awscdk.services.iam.Grant.class, java.util.stream.Stream.concat(java.util.Arrays.