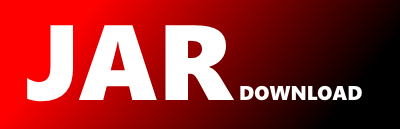
software.amazon.awscdk.services.lambda.EventSourceMappingProps Maven / Gradle / Ivy
package software.amazon.awscdk.services.lambda;
/**
* Properties for declaring a new event source mapping.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.lambda.*;
* import software.amazon.awscdk.core.*;
* IEventSourceDlq eventSourceDlq;
* Function function_;
* SourceAccessConfigurationType sourceAccessConfigurationType;
* EventSourceMappingProps eventSourceMappingProps = EventSourceMappingProps.builder()
* .target(function_)
* // the properties below are optional
* .batchSize(123)
* .bisectBatchOnError(false)
* .enabled(false)
* .eventSourceArn("eventSourceArn")
* .kafkaBootstrapServers(List.of("kafkaBootstrapServers"))
* .kafkaTopic("kafkaTopic")
* .maxBatchingWindow(Duration.minutes(30))
* .maxRecordAge(Duration.minutes(30))
* .onFailure(eventSourceDlq)
* .parallelizationFactor(123)
* .reportBatchItemFailures(false)
* .retryAttempts(123)
* .sourceAccessConfigurations(List.of(SourceAccessConfiguration.builder()
* .type(sourceAccessConfigurationType)
* .uri("uri")
* .build()))
* .startingPosition(StartingPosition.TRIM_HORIZON)
* .tumblingWindow(Duration.minutes(30))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.46.0 (build cd08c55)", date = "2021-11-23T14:40:40.064Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.EventSourceMappingProps")
@software.amazon.jsii.Jsii.Proxy(EventSourceMappingProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface EventSourceMappingProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.lambda.EventSourceMappingOptions {
/**
* The target AWS Lambda function.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.lambda.IFunction getTarget();
/**
* @return a {@link Builder} of {@link EventSourceMappingProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link EventSourceMappingProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.lambda.IFunction target;
java.lang.Number batchSize;
java.lang.Boolean bisectBatchOnError;
java.lang.Boolean enabled;
java.lang.String eventSourceArn;
java.util.List kafkaBootstrapServers;
java.lang.String kafkaTopic;
software.amazon.awscdk.core.Duration maxBatchingWindow;
software.amazon.awscdk.core.Duration maxRecordAge;
software.amazon.awscdk.services.lambda.IEventSourceDlq onFailure;
java.lang.Number parallelizationFactor;
java.lang.Boolean reportBatchItemFailures;
java.lang.Number retryAttempts;
java.util.List sourceAccessConfigurations;
software.amazon.awscdk.services.lambda.StartingPosition startingPosition;
software.amazon.awscdk.core.Duration tumblingWindow;
/**
* Sets the value of {@link EventSourceMappingProps#getTarget}
* @param target The target AWS Lambda function. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder target(software.amazon.awscdk.services.lambda.IFunction target) {
this.target = target;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getBatchSize}
* @param batchSize The largest number of records that AWS Lambda will retrieve from your event source at the time of invoking your function.
* Your function receives an
* event with all the retrieved records.
*
* Valid Range: Minimum value of 1. Maximum value of 10000.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder batchSize(java.lang.Number batchSize) {
this.batchSize = batchSize;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getBisectBatchOnError}
* @param bisectBatchOnError If the function returns an error, split the batch in two and retry.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bisectBatchOnError(java.lang.Boolean bisectBatchOnError) {
this.bisectBatchOnError = bisectBatchOnError;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getEnabled}
* @param enabled Set to false to disable the event source upon creation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getEventSourceArn}
* @param eventSourceArn The Amazon Resource Name (ARN) of the event source.
* Any record added to
* this stream can invoke the Lambda function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder eventSourceArn(java.lang.String eventSourceArn) {
this.eventSourceArn = eventSourceArn;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getKafkaBootstrapServers}
* @param kafkaBootstrapServers A list of host and port pairs that are the addresses of the Kafka brokers in a self managed "bootstrap" Kafka cluster that a Kafka client connects to initially to bootstrap itself.
* They are in the format abc.example.com:9096
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kafkaBootstrapServers(java.util.List kafkaBootstrapServers) {
this.kafkaBootstrapServers = kafkaBootstrapServers;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getKafkaTopic}
* @param kafkaTopic The name of the Kafka topic.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder kafkaTopic(java.lang.String kafkaTopic) {
this.kafkaTopic = kafkaTopic;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getMaxBatchingWindow}
* @param maxBatchingWindow The maximum amount of time to gather records before invoking the function.
* Maximum of Duration.minutes(5)
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxBatchingWindow(software.amazon.awscdk.core.Duration maxBatchingWindow) {
this.maxBatchingWindow = maxBatchingWindow;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getMaxRecordAge}
* @param maxRecordAge The maximum age of a record that Lambda sends to a function for processing.
* Valid Range:
*
*
* - Minimum value of 60 seconds
* - Maximum value of 7 days
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxRecordAge(software.amazon.awscdk.core.Duration maxRecordAge) {
this.maxRecordAge = maxRecordAge;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getOnFailure}
* @param onFailure An Amazon SQS queue or Amazon SNS topic destination for discarded records.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder onFailure(software.amazon.awscdk.services.lambda.IEventSourceDlq onFailure) {
this.onFailure = onFailure;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getParallelizationFactor}
* @param parallelizationFactor The number of batches to process from each shard concurrently.
* Valid Range:
*
*
* - Minimum value of 1
* - Maximum value of 10
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parallelizationFactor(java.lang.Number parallelizationFactor) {
this.parallelizationFactor = parallelizationFactor;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getReportBatchItemFailures}
* @param reportBatchItemFailures Allow functions to return partially successful responses for a batch of records.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder reportBatchItemFailures(java.lang.Boolean reportBatchItemFailures) {
this.reportBatchItemFailures = reportBatchItemFailures;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getRetryAttempts}
* @param retryAttempts The maximum number of times to retry when the function returns an error.
* Set to undefined
if you want lambda to keep retrying infinitely or until
* the record expires.
*
* Valid Range:
*
*
* - Minimum value of 0
* - Maximum value of 10000
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder retryAttempts(java.lang.Number retryAttempts) {
this.retryAttempts = retryAttempts;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getSourceAccessConfigurations}
* @param sourceAccessConfigurations Specific settings like the authentication protocol or the VPC components to secure access to your event source.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder sourceAccessConfigurations(java.util.List extends software.amazon.awscdk.services.lambda.SourceAccessConfiguration> sourceAccessConfigurations) {
this.sourceAccessConfigurations = (java.util.List)sourceAccessConfigurations;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getStartingPosition}
* @param startingPosition The position in the DynamoDB, Kinesis or MSK stream where AWS Lambda should start reading.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder startingPosition(software.amazon.awscdk.services.lambda.StartingPosition startingPosition) {
this.startingPosition = startingPosition;
return this;
}
/**
* Sets the value of {@link EventSourceMappingProps#getTumblingWindow}
* @param tumblingWindow The size of the tumbling windows to group records sent to DynamoDB or Kinesis.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tumblingWindow(software.amazon.awscdk.core.Duration tumblingWindow) {
this.tumblingWindow = tumblingWindow;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link EventSourceMappingProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public EventSourceMappingProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link EventSourceMappingProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements EventSourceMappingProps {
private final software.amazon.awscdk.services.lambda.IFunction target;
private final java.lang.Number batchSize;
private final java.lang.Boolean bisectBatchOnError;
private final java.lang.Boolean enabled;
private final java.lang.String eventSourceArn;
private final java.util.List kafkaBootstrapServers;
private final java.lang.String kafkaTopic;
private final software.amazon.awscdk.core.Duration maxBatchingWindow;
private final software.amazon.awscdk.core.Duration maxRecordAge;
private final software.amazon.awscdk.services.lambda.IEventSourceDlq onFailure;
private final java.lang.Number parallelizationFactor;
private final java.lang.Boolean reportBatchItemFailures;
private final java.lang.Number retryAttempts;
private final java.util.List sourceAccessConfigurations;
private final software.amazon.awscdk.services.lambda.StartingPosition startingPosition;
private final software.amazon.awscdk.core.Duration tumblingWindow;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.target = software.amazon.jsii.Kernel.get(this, "target", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.IFunction.class));
this.batchSize = software.amazon.jsii.Kernel.get(this, "batchSize", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.bisectBatchOnError = software.amazon.jsii.Kernel.get(this, "bisectBatchOnError", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.enabled = software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.eventSourceArn = software.amazon.jsii.Kernel.get(this, "eventSourceArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.kafkaBootstrapServers = software.amazon.jsii.Kernel.get(this, "kafkaBootstrapServers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.kafkaTopic = software.amazon.jsii.Kernel.get(this, "kafkaTopic", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.maxBatchingWindow = software.amazon.jsii.Kernel.get(this, "maxBatchingWindow", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.maxRecordAge = software.amazon.jsii.Kernel.get(this, "maxRecordAge", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.onFailure = software.amazon.jsii.Kernel.get(this, "onFailure", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.IEventSourceDlq.class));
this.parallelizationFactor = software.amazon.jsii.Kernel.get(this, "parallelizationFactor", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.reportBatchItemFailures = software.amazon.jsii.Kernel.get(this, "reportBatchItemFailures", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.retryAttempts = software.amazon.jsii.Kernel.get(this, "retryAttempts", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.sourceAccessConfigurations = software.amazon.jsii.Kernel.get(this, "sourceAccessConfigurations", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.SourceAccessConfiguration.class)));
this.startingPosition = software.amazon.jsii.Kernel.get(this, "startingPosition", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.StartingPosition.class));
this.tumblingWindow = software.amazon.jsii.Kernel.get(this, "tumblingWindow", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.target = java.util.Objects.requireNonNull(builder.target, "target is required");
this.batchSize = builder.batchSize;
this.bisectBatchOnError = builder.bisectBatchOnError;
this.enabled = builder.enabled;
this.eventSourceArn = builder.eventSourceArn;
this.kafkaBootstrapServers = builder.kafkaBootstrapServers;
this.kafkaTopic = builder.kafkaTopic;
this.maxBatchingWindow = builder.maxBatchingWindow;
this.maxRecordAge = builder.maxRecordAge;
this.onFailure = builder.onFailure;
this.parallelizationFactor = builder.parallelizationFactor;
this.reportBatchItemFailures = builder.reportBatchItemFailures;
this.retryAttempts = builder.retryAttempts;
this.sourceAccessConfigurations = (java.util.List)builder.sourceAccessConfigurations;
this.startingPosition = builder.startingPosition;
this.tumblingWindow = builder.tumblingWindow;
}
@Override
public final software.amazon.awscdk.services.lambda.IFunction getTarget() {
return this.target;
}
@Override
public final java.lang.Number getBatchSize() {
return this.batchSize;
}
@Override
public final java.lang.Boolean getBisectBatchOnError() {
return this.bisectBatchOnError;
}
@Override
public final java.lang.Boolean getEnabled() {
return this.enabled;
}
@Override
public final java.lang.String getEventSourceArn() {
return this.eventSourceArn;
}
@Override
public final java.util.List getKafkaBootstrapServers() {
return this.kafkaBootstrapServers;
}
@Override
public final java.lang.String getKafkaTopic() {
return this.kafkaTopic;
}
@Override
public final software.amazon.awscdk.core.Duration getMaxBatchingWindow() {
return this.maxBatchingWindow;
}
@Override
public final software.amazon.awscdk.core.Duration getMaxRecordAge() {
return this.maxRecordAge;
}
@Override
public final software.amazon.awscdk.services.lambda.IEventSourceDlq getOnFailure() {
return this.onFailure;
}
@Override
public final java.lang.Number getParallelizationFactor() {
return this.parallelizationFactor;
}
@Override
public final java.lang.Boolean getReportBatchItemFailures() {
return this.reportBatchItemFailures;
}
@Override
public final java.lang.Number getRetryAttempts() {
return this.retryAttempts;
}
@Override
public final java.util.List getSourceAccessConfigurations() {
return this.sourceAccessConfigurations;
}
@Override
public final software.amazon.awscdk.services.lambda.StartingPosition getStartingPosition() {
return this.startingPosition;
}
@Override
public final software.amazon.awscdk.core.Duration getTumblingWindow() {
return this.tumblingWindow;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("target", om.valueToTree(this.getTarget()));
if (this.getBatchSize() != null) {
data.set("batchSize", om.valueToTree(this.getBatchSize()));
}
if (this.getBisectBatchOnError() != null) {
data.set("bisectBatchOnError", om.valueToTree(this.getBisectBatchOnError()));
}
if (this.getEnabled() != null) {
data.set("enabled", om.valueToTree(this.getEnabled()));
}
if (this.getEventSourceArn() != null) {
data.set("eventSourceArn", om.valueToTree(this.getEventSourceArn()));
}
if (this.getKafkaBootstrapServers() != null) {
data.set("kafkaBootstrapServers", om.valueToTree(this.getKafkaBootstrapServers()));
}
if (this.getKafkaTopic() != null) {
data.set("kafkaTopic", om.valueToTree(this.getKafkaTopic()));
}
if (this.getMaxBatchingWindow() != null) {
data.set("maxBatchingWindow", om.valueToTree(this.getMaxBatchingWindow()));
}
if (this.getMaxRecordAge() != null) {
data.set("maxRecordAge", om.valueToTree(this.getMaxRecordAge()));
}
if (this.getOnFailure() != null) {
data.set("onFailure", om.valueToTree(this.getOnFailure()));
}
if (this.getParallelizationFactor() != null) {
data.set("parallelizationFactor", om.valueToTree(this.getParallelizationFactor()));
}
if (this.getReportBatchItemFailures() != null) {
data.set("reportBatchItemFailures", om.valueToTree(this.getReportBatchItemFailures()));
}
if (this.getRetryAttempts() != null) {
data.set("retryAttempts", om.valueToTree(this.getRetryAttempts()));
}
if (this.getSourceAccessConfigurations() != null) {
data.set("sourceAccessConfigurations", om.valueToTree(this.getSourceAccessConfigurations()));
}
if (this.getStartingPosition() != null) {
data.set("startingPosition", om.valueToTree(this.getStartingPosition()));
}
if (this.getTumblingWindow() != null) {
data.set("tumblingWindow", om.valueToTree(this.getTumblingWindow()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.EventSourceMappingProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EventSourceMappingProps.Jsii$Proxy that = (EventSourceMappingProps.Jsii$Proxy) o;
if (!target.equals(that.target)) return false;
if (this.batchSize != null ? !this.batchSize.equals(that.batchSize) : that.batchSize != null) return false;
if (this.bisectBatchOnError != null ? !this.bisectBatchOnError.equals(that.bisectBatchOnError) : that.bisectBatchOnError != null) return false;
if (this.enabled != null ? !this.enabled.equals(that.enabled) : that.enabled != null) return false;
if (this.eventSourceArn != null ? !this.eventSourceArn.equals(that.eventSourceArn) : that.eventSourceArn != null) return false;
if (this.kafkaBootstrapServers != null ? !this.kafkaBootstrapServers.equals(that.kafkaBootstrapServers) : that.kafkaBootstrapServers != null) return false;
if (this.kafkaTopic != null ? !this.kafkaTopic.equals(that.kafkaTopic) : that.kafkaTopic != null) return false;
if (this.maxBatchingWindow != null ? !this.maxBatchingWindow.equals(that.maxBatchingWindow) : that.maxBatchingWindow != null) return false;
if (this.maxRecordAge != null ? !this.maxRecordAge.equals(that.maxRecordAge) : that.maxRecordAge != null) return false;
if (this.onFailure != null ? !this.onFailure.equals(that.onFailure) : that.onFailure != null) return false;
if (this.parallelizationFactor != null ? !this.parallelizationFactor.equals(that.parallelizationFactor) : that.parallelizationFactor != null) return false;
if (this.reportBatchItemFailures != null ? !this.reportBatchItemFailures.equals(that.reportBatchItemFailures) : that.reportBatchItemFailures != null) return false;
if (this.retryAttempts != null ? !this.retryAttempts.equals(that.retryAttempts) : that.retryAttempts != null) return false;
if (this.sourceAccessConfigurations != null ? !this.sourceAccessConfigurations.equals(that.sourceAccessConfigurations) : that.sourceAccessConfigurations != null) return false;
if (this.startingPosition != null ? !this.startingPosition.equals(that.startingPosition) : that.startingPosition != null) return false;
return this.tumblingWindow != null ? this.tumblingWindow.equals(that.tumblingWindow) : that.tumblingWindow == null;
}
@Override
public final int hashCode() {
int result = this.target.hashCode();
result = 31 * result + (this.batchSize != null ? this.batchSize.hashCode() : 0);
result = 31 * result + (this.bisectBatchOnError != null ? this.bisectBatchOnError.hashCode() : 0);
result = 31 * result + (this.enabled != null ? this.enabled.hashCode() : 0);
result = 31 * result + (this.eventSourceArn != null ? this.eventSourceArn.hashCode() : 0);
result = 31 * result + (this.kafkaBootstrapServers != null ? this.kafkaBootstrapServers.hashCode() : 0);
result = 31 * result + (this.kafkaTopic != null ? this.kafkaTopic.hashCode() : 0);
result = 31 * result + (this.maxBatchingWindow != null ? this.maxBatchingWindow.hashCode() : 0);
result = 31 * result + (this.maxRecordAge != null ? this.maxRecordAge.hashCode() : 0);
result = 31 * result + (this.onFailure != null ? this.onFailure.hashCode() : 0);
result = 31 * result + (this.parallelizationFactor != null ? this.parallelizationFactor.hashCode() : 0);
result = 31 * result + (this.reportBatchItemFailures != null ? this.reportBatchItemFailures.hashCode() : 0);
result = 31 * result + (this.retryAttempts != null ? this.retryAttempts.hashCode() : 0);
result = 31 * result + (this.sourceAccessConfigurations != null ? this.sourceAccessConfigurations.hashCode() : 0);
result = 31 * result + (this.startingPosition != null ? this.startingPosition.hashCode() : 0);
result = 31 * result + (this.tumblingWindow != null ? this.tumblingWindow.hashCode() : 0);
return result;
}
}
}