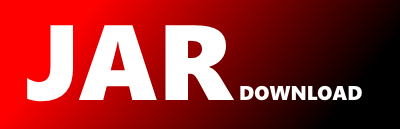
software.amazon.awscdk.services.lambda.FunctionAttributes Maven / Gradle / Ivy
package software.amazon.awscdk.services.lambda;
/**
* Represents a Lambda function defined outside of this stack.
*
* Example:
*
*
* IFunction fn = Function.fromFunctionAttributes(this, "Function", FunctionAttributes.builder()
* .functionArn("arn:aws:lambda:us-east-1:123456789012:function:MyFn")
* // The following are optional properties for specific use cases and should be used with caution:
* // Use Case: imported function is in the same account as the stack. This tells the CDK that it
* // can modify the function's permissions.
* .sameEnvironment(true)
* // Use Case: imported function is in a different account and user commits to ensuring that the
* // imported function has the correct permissions outside the CDK.
* .skipPermissions(true)
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-03-14T16:25:29.791Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.lambda.$Module.class, fqn = "@aws-cdk/aws-lambda.FunctionAttributes")
@software.amazon.jsii.Jsii.Proxy(FunctionAttributes.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface FunctionAttributes extends software.amazon.jsii.JsiiSerializable {
/**
* The ARN of the Lambda function.
*
* Format: arn::lambda:::function:
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getFunctionArn();
/**
* The architecture of this Lambda Function (this is an optional attribute and defaults to X86_64).
*
* Default: - Architecture.X86_64
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.lambda.Architecture getArchitecture() {
return null;
}
/**
* The IAM execution role associated with this function.
*
* If the role is not specified, any role-related operations will no-op.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.iam.IRole getRole() {
return null;
}
/**
* Setting this property informs the CDK that the imported function is in the same environment as the stack.
*
* This affects certain behaviours such as, whether this function's permission can be modified.
* When not configured, the CDK attempts to auto-determine this. For environment agnostic stacks, i.e., stacks
* where the account is not specified with the env
property, this is determined to be false.
*
* Set this to property ONLY IF the imported function is in the same account as the stack
* it's imported in.
*
* Default: - depends: true, if the Stack is configured with an explicit `env` (account and region) and the account is the same as this function.
* For environment-agnostic stacks this will default to `false`.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getSameEnvironment() {
return null;
}
/**
* The security group of this Lambda, if in a VPC.
*
* This needs to be given in order to support allowing connections
* to this Lambda.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return null;
}
/**
* (deprecated) Id of the security group of this Lambda, if in a VPC.
*
* This needs to be given in order to support allowing connections
* to this Lambda.
*
* @deprecated use `securityGroup` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
default @org.jetbrains.annotations.Nullable java.lang.String getSecurityGroupId() {
return null;
}
/**
* Setting this property informs the CDK that the imported function ALREADY HAS the necessary permissions for what you are trying to do.
*
* When not configured, the CDK attempts to auto-determine whether or not
* additional permissions are necessary on the function when grant APIs are used. If the CDK tried to add
* permissions on an imported lambda, it will fail.
*
* Set this property ONLY IF you are committing to manage the imported function's permissions outside of
* CDK. You are acknowledging that your CDK code alone will have insufficient permissions to access the
* imported function.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getSkipPermissions() {
return null;
}
/**
* @return a {@link Builder} of {@link FunctionAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link FunctionAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String functionArn;
software.amazon.awscdk.services.lambda.Architecture architecture;
software.amazon.awscdk.services.iam.IRole role;
java.lang.Boolean sameEnvironment;
software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
java.lang.String securityGroupId;
java.lang.Boolean skipPermissions;
/**
* Sets the value of {@link FunctionAttributes#getFunctionArn}
* @param functionArn The ARN of the Lambda function. This parameter is required.
* Format: arn::lambda:::function:
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder functionArn(java.lang.String functionArn) {
this.functionArn = functionArn;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getArchitecture}
* @param architecture The architecture of this Lambda Function (this is an optional attribute and defaults to X86_64).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder architecture(software.amazon.awscdk.services.lambda.Architecture architecture) {
this.architecture = architecture;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getRole}
* @param role The IAM execution role associated with this function.
* If the role is not specified, any role-related operations will no-op.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getSameEnvironment}
* @param sameEnvironment Setting this property informs the CDK that the imported function is in the same environment as the stack.
* This affects certain behaviours such as, whether this function's permission can be modified.
* When not configured, the CDK attempts to auto-determine this. For environment agnostic stacks, i.e., stacks
* where the account is not specified with the env
property, this is determined to be false.
*
* Set this to property ONLY IF the imported function is in the same account as the stack
* it's imported in.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sameEnvironment(java.lang.Boolean sameEnvironment) {
this.sameEnvironment = sameEnvironment;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getSecurityGroup}
* @param securityGroup The security group of this Lambda, if in a VPC.
* This needs to be given in order to support allowing connections
* to this Lambda.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder securityGroup(software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup) {
this.securityGroup = securityGroup;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getSecurityGroupId}
* @param securityGroupId Id of the security group of this Lambda, if in a VPC.
* This needs to be given in order to support allowing connections
* to this Lambda.
* @return {@code this}
* @deprecated use `securityGroup` instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder securityGroupId(java.lang.String securityGroupId) {
this.securityGroupId = securityGroupId;
return this;
}
/**
* Sets the value of {@link FunctionAttributes#getSkipPermissions}
* @param skipPermissions Setting this property informs the CDK that the imported function ALREADY HAS the necessary permissions for what you are trying to do.
* When not configured, the CDK attempts to auto-determine whether or not
* additional permissions are necessary on the function when grant APIs are used. If the CDK tried to add
* permissions on an imported lambda, it will fail.
*
* Set this property ONLY IF you are committing to manage the imported function's permissions outside of
* CDK. You are acknowledging that your CDK code alone will have insufficient permissions to access the
* imported function.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder skipPermissions(java.lang.Boolean skipPermissions) {
this.skipPermissions = skipPermissions;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link FunctionAttributes}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public FunctionAttributes build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link FunctionAttributes}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements FunctionAttributes {
private final java.lang.String functionArn;
private final software.amazon.awscdk.services.lambda.Architecture architecture;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.Boolean sameEnvironment;
private final software.amazon.awscdk.services.ec2.ISecurityGroup securityGroup;
private final java.lang.String securityGroupId;
private final java.lang.Boolean skipPermissions;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.functionArn = software.amazon.jsii.Kernel.get(this, "functionArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.architecture = software.amazon.jsii.Kernel.get(this, "architecture", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.lambda.Architecture.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.sameEnvironment = software.amazon.jsii.Kernel.get(this, "sameEnvironment", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.securityGroup = software.amazon.jsii.Kernel.get(this, "securityGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ec2.ISecurityGroup.class));
this.securityGroupId = software.amazon.jsii.Kernel.get(this, "securityGroupId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.skipPermissions = software.amazon.jsii.Kernel.get(this, "skipPermissions", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.functionArn = java.util.Objects.requireNonNull(builder.functionArn, "functionArn is required");
this.architecture = builder.architecture;
this.role = builder.role;
this.sameEnvironment = builder.sameEnvironment;
this.securityGroup = builder.securityGroup;
this.securityGroupId = builder.securityGroupId;
this.skipPermissions = builder.skipPermissions;
}
@Override
public final java.lang.String getFunctionArn() {
return this.functionArn;
}
@Override
public final software.amazon.awscdk.services.lambda.Architecture getArchitecture() {
return this.architecture;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.Boolean getSameEnvironment() {
return this.sameEnvironment;
}
@Override
public final software.amazon.awscdk.services.ec2.ISecurityGroup getSecurityGroup() {
return this.securityGroup;
}
@Override
public final java.lang.String getSecurityGroupId() {
return this.securityGroupId;
}
@Override
public final java.lang.Boolean getSkipPermissions() {
return this.skipPermissions;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("functionArn", om.valueToTree(this.getFunctionArn()));
if (this.getArchitecture() != null) {
data.set("architecture", om.valueToTree(this.getArchitecture()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
if (this.getSameEnvironment() != null) {
data.set("sameEnvironment", om.valueToTree(this.getSameEnvironment()));
}
if (this.getSecurityGroup() != null) {
data.set("securityGroup", om.valueToTree(this.getSecurityGroup()));
}
if (this.getSecurityGroupId() != null) {
data.set("securityGroupId", om.valueToTree(this.getSecurityGroupId()));
}
if (this.getSkipPermissions() != null) {
data.set("skipPermissions", om.valueToTree(this.getSkipPermissions()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-lambda.FunctionAttributes"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FunctionAttributes.Jsii$Proxy that = (FunctionAttributes.Jsii$Proxy) o;
if (!functionArn.equals(that.functionArn)) return false;
if (this.architecture != null ? !this.architecture.equals(that.architecture) : that.architecture != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (this.sameEnvironment != null ? !this.sameEnvironment.equals(that.sameEnvironment) : that.sameEnvironment != null) return false;
if (this.securityGroup != null ? !this.securityGroup.equals(that.securityGroup) : that.securityGroup != null) return false;
if (this.securityGroupId != null ? !this.securityGroupId.equals(that.securityGroupId) : that.securityGroupId != null) return false;
return this.skipPermissions != null ? this.skipPermissions.equals(that.skipPermissions) : that.skipPermissions == null;
}
@Override
public final int hashCode() {
int result = this.functionArn.hashCode();
result = 31 * result + (this.architecture != null ? this.architecture.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.sameEnvironment != null ? this.sameEnvironment.hashCode() : 0);
result = 31 * result + (this.securityGroup != null ? this.securityGroup.hashCode() : 0);
result = 31 * result + (this.securityGroupId != null ? this.securityGroupId.hashCode() : 0);
result = 31 * result + (this.skipPermissions != null ? this.skipPermissions.hashCode() : 0);
return result;
}
}
}